Hi there, we’re Harisystems
"Unlock your potential and soar to new heights with our exclusive online courses! Ignite your passion, acquire valuable skills, and embrace limitless possibilities. Don't miss out on our limited-time sale - invest in yourself today and embark on a journey of personal and professional growth. Enroll now and shape your future with knowledge that lasts a lifetime!".
For corporate trainings, projects, and real world experience reach us. We believe that education should be accessible to all, regardless of geographical location or background.
1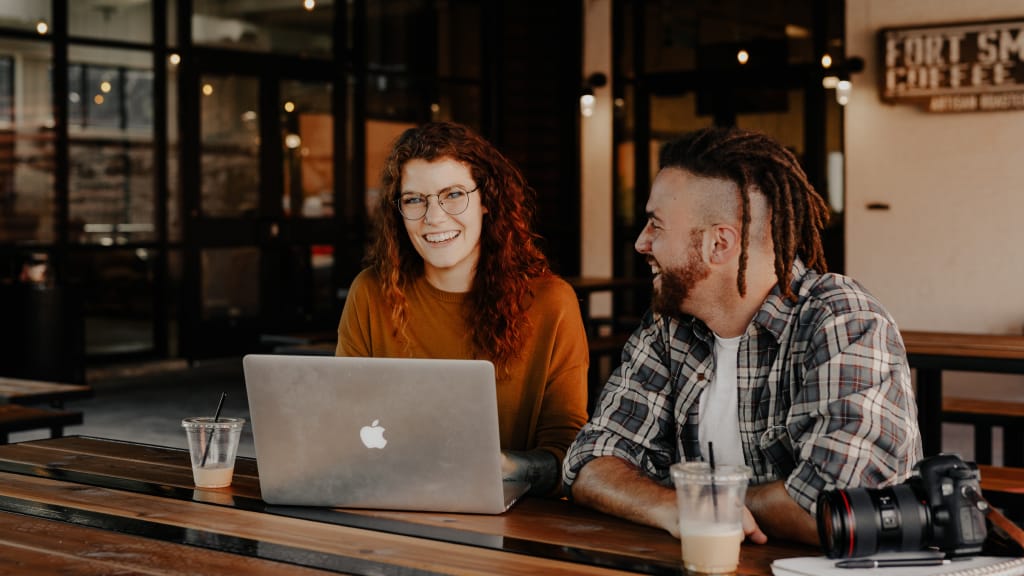
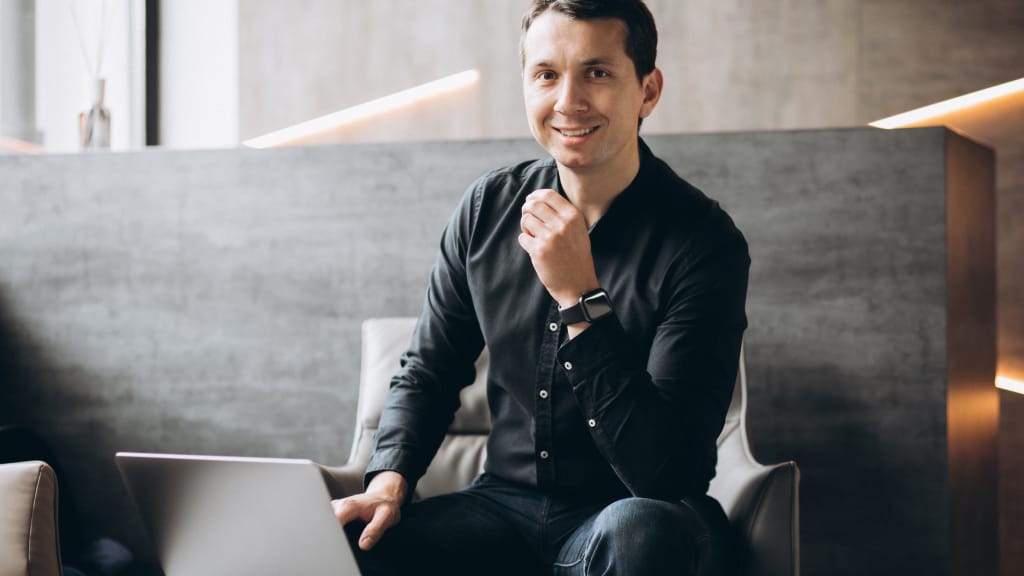
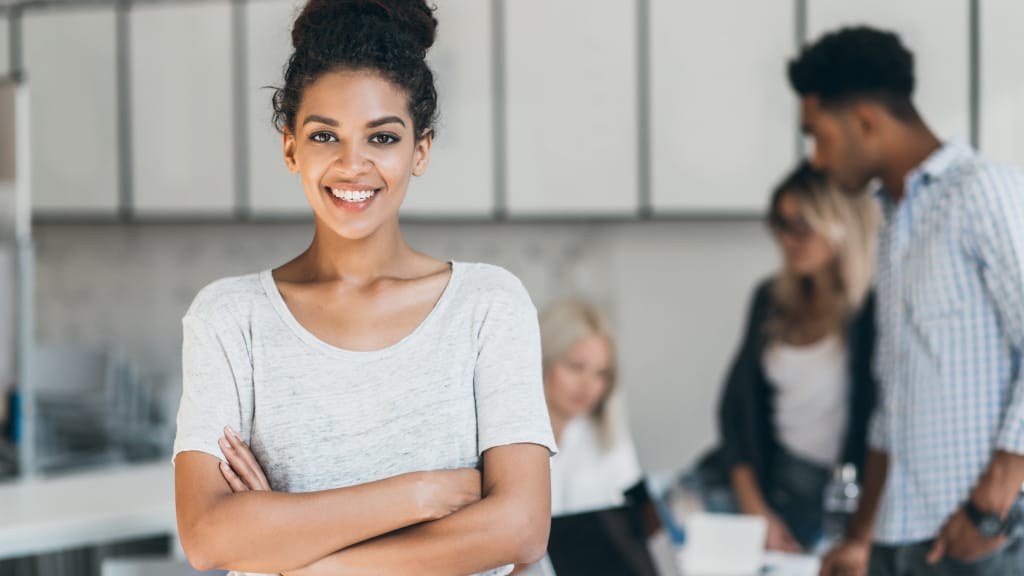
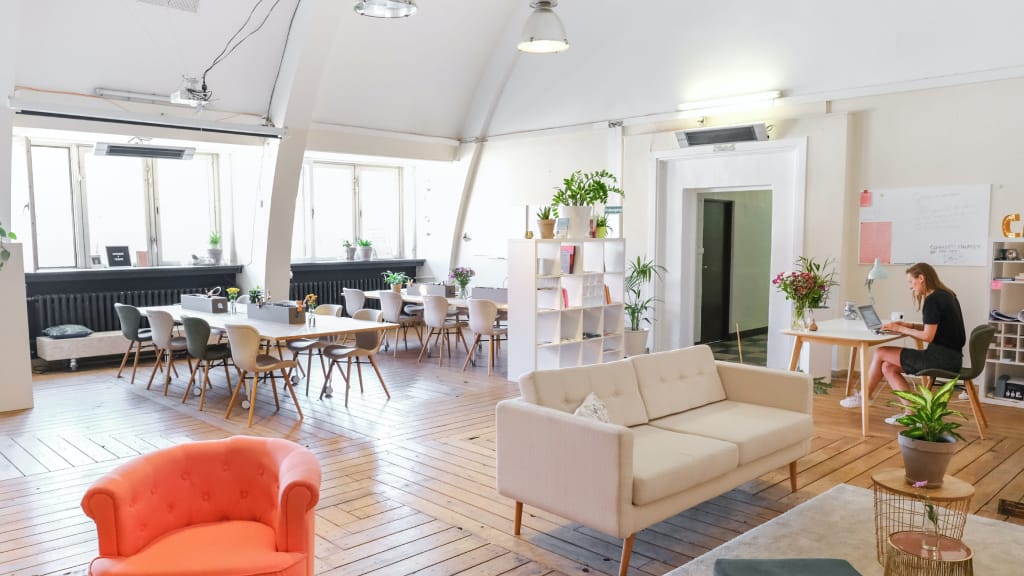
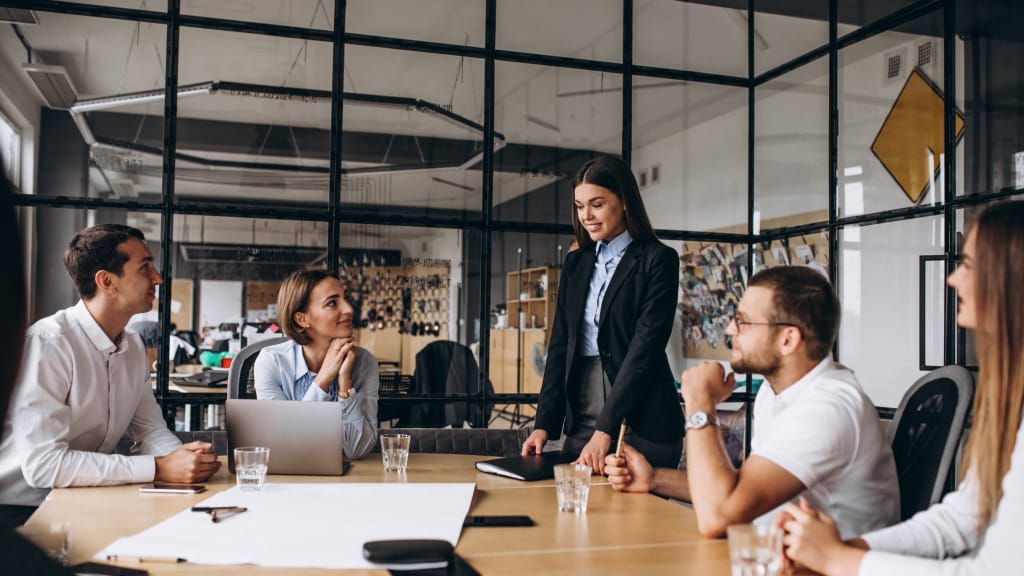
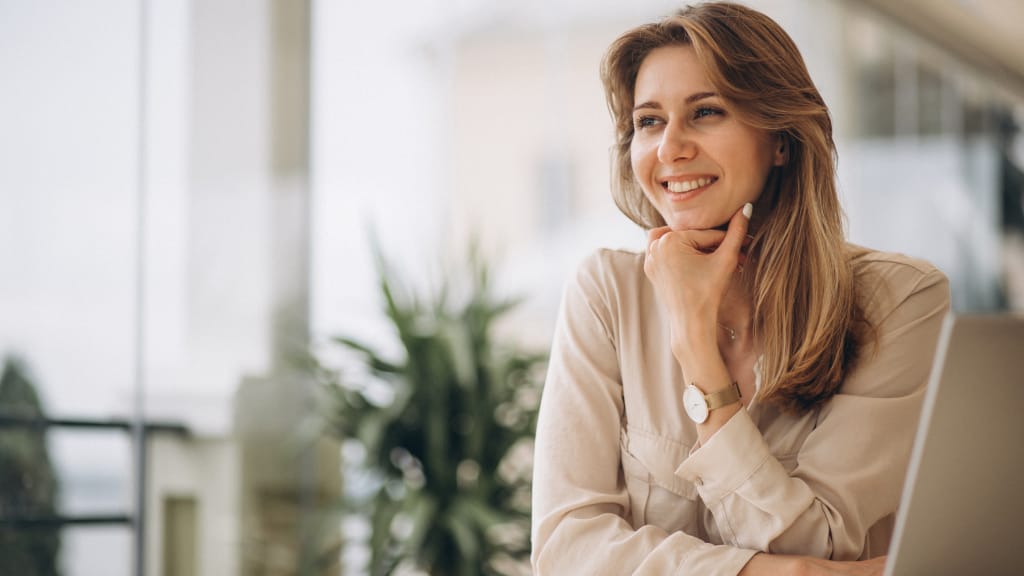
C++ Advanced Strings
In C++, strings are an essential component for working with textual data. The C++ standard library provides advanced features for manipulating strings, such as searching, modifying, and formatting. In this article, we will explore some advanced string concepts in C++ with examples.
1. Searching in Strings
The std::string
class provides various methods for searching within strings. Here are some commonly used functions:
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, World!";
// Find first occurrence of a substring
size_t found = str.find("World");
if (found != std::string::npos) {
std::cout << "Substring found at position: " << found << std::endl;
} else {
std::cout << "Substring not found." << std::endl;
}
// Find last occurrence of a character
found = str.find_last_of("l");
if (found != std::string::npos) {
std::cout << "Last occurrence found at position: " << found << std::endl;
} else {
std::cout << "Character not found." << std::endl;
}
// Check if a string starts with a substring
bool startsWith = str.compare(0, 5, "Hello") == 0;
std::cout << "Starts with 'Hello': " << std::boolalpha << startsWith << std::endl;
// Check if a string ends with a substring
bool endsWith = str.compare(str.length() - 6, 6, "World!") == 0;
std::cout << "Ends with 'World!': " << std::boolalpha << endsWith << std::endl;
return 0;
}
In the above code, we use the find()
function to search for a substring within the string. If the substring is found, the function returns its position; otherwise, it returns std::string::npos
. We also use the find_last_of()
function to find the last occurrence of a character within the string. Additionally, we use the compare()
function to check if the string starts with or ends with a specific substring.
2. Modifying Strings
The std::string
class allows you to modify strings in various ways. Here are some commonly used functions:
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, World!";
// Replace a substring
str.replace(7, 5, "Universe");
std::cout << "Modified string: " << str << std::endl;
// Insert a substring
str.insert(0, "Greeting: ");
std::cout << "Modified string: " << str << std::endl;
// Erase a substring
str.erase(0, 10);
std::cout << "Modified string: " << str << std::endl;
// Convert to uppercase
for (char& c : str) {
c = std::toupper(c);
}
std::cout << "Modified string: " << str << std::endl;
return 0;
}
In the above code, we use the replace()
function to replace a substring within the string. We use the insert()
function to insert a substring at a specified position. We use the erase()
function to remove a substring from the string. Finally, we convert the string to uppercase using the toupper()
function.
3. Formatting Strings
C++ provides powerful string formatting capabilities using std::stringstream
or the more modern std::format
. Here's an example using std::format
:
#include <iostream>
#include <format>
int main() {
std::string name = "Alice";
int age = 25;
std::string formattedString = std::format("My name is {} and I am {} years old.", name, age);
std::cout << formattedString << std::endl;
return 0;
}
In the above code, we use the std::format
function to create a formatted string. We provide placeholders ({}
) in the string and pass the corresponding values to the function. The function returns a formatted string with the provided values substituted.
Advanced string operations in C++ allow for powerful manipulation, searching, and formatting of strings. Utilize these features to handle complex string-related tasks effectively in your C++ programs.
4.5L
Learners
20+
Instructors
50+
Courses
6.0L
Course enrollments
Future Trending Courses
When selecting, a course, Here are a few areas that are expected to be in demand in the future:.
Future Learning for all
If you’re passionate and ready to dive in, we’d love to join 1:1 classes for you. We’re committed to support our learners and professionals their development and well-being.
View Courses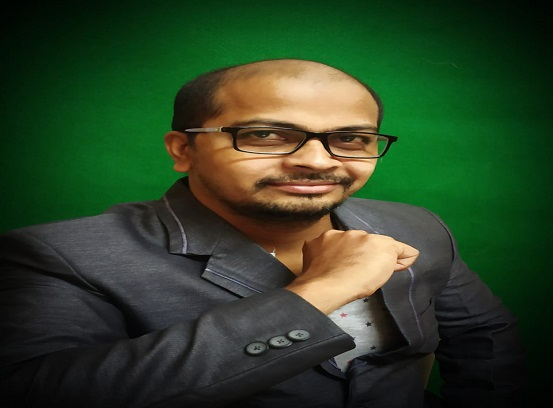
Most Popular Course topics
These are the most popular course topics among Software Courses for learners