Hi there, we’re Harisystems
"Unlock your potential and soar to new heights with our exclusive online courses! Ignite your passion, acquire valuable skills, and embrace limitless possibilities. Don't miss out on our limited-time sale - invest in yourself today and embark on a journey of personal and professional growth. Enroll now and shape your future with knowledge that lasts a lifetime!".
For corporate trainings, projects, and real world experience reach us. We believe that education should be accessible to all, regardless of geographical location or background.
1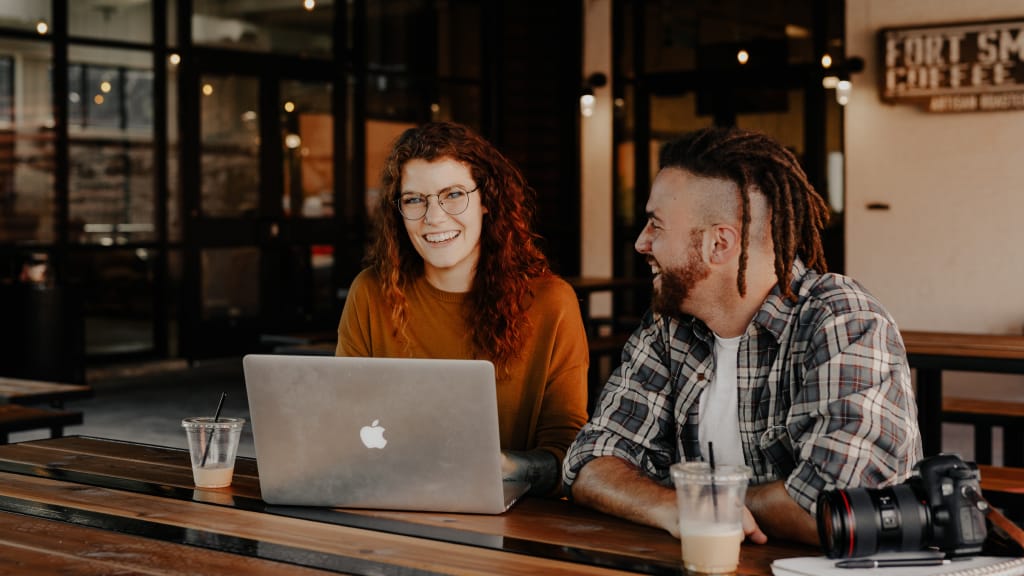
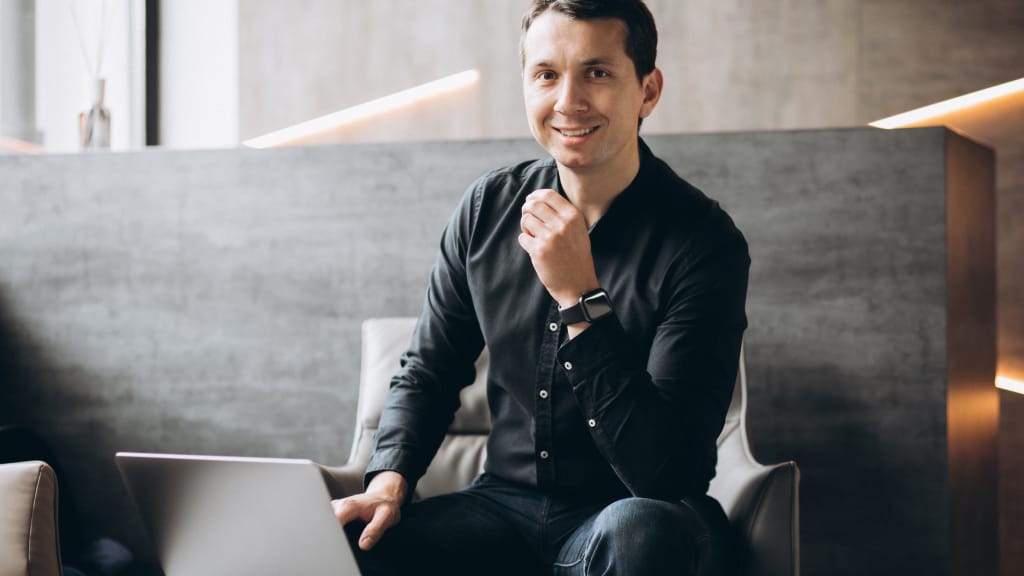
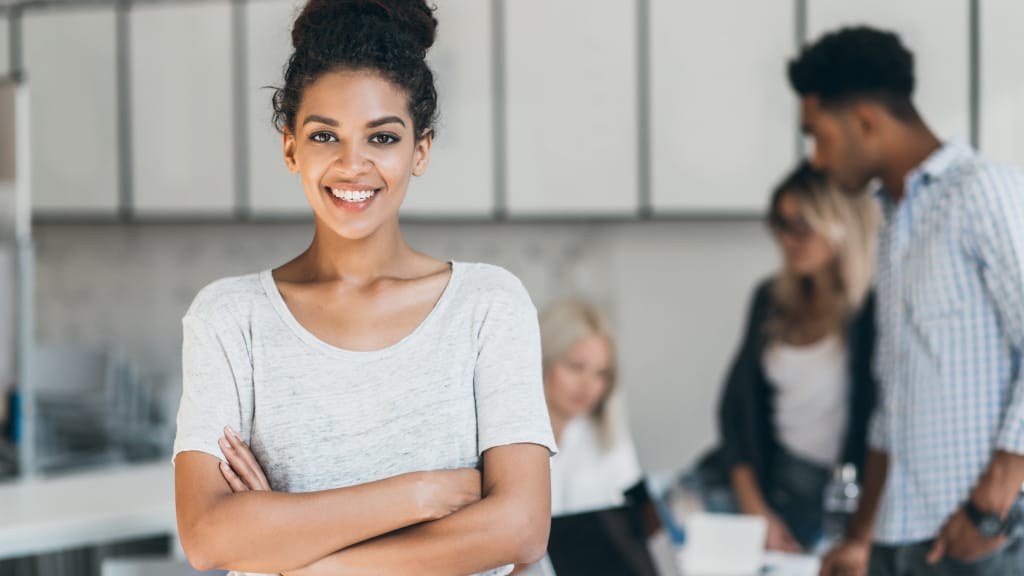
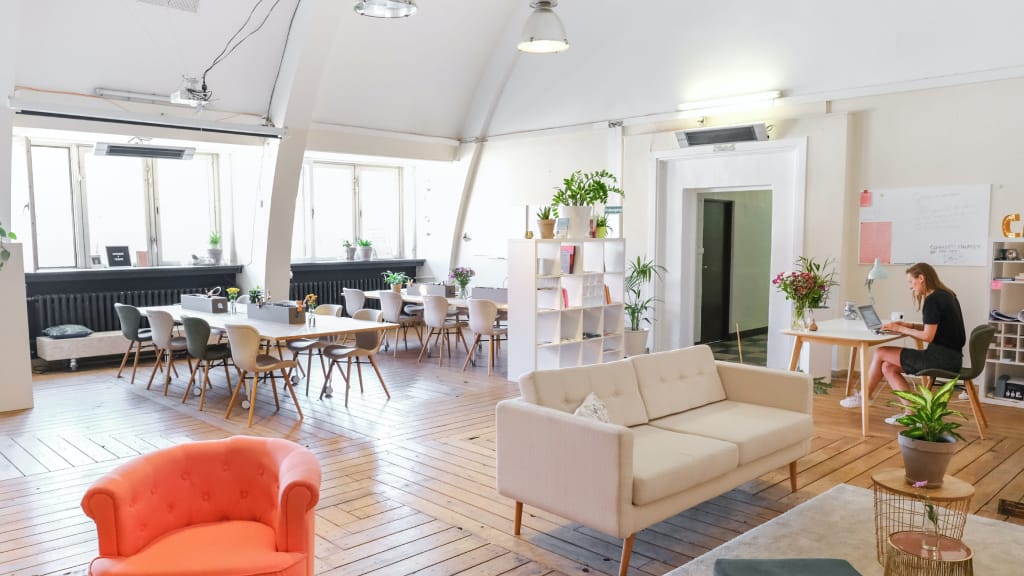
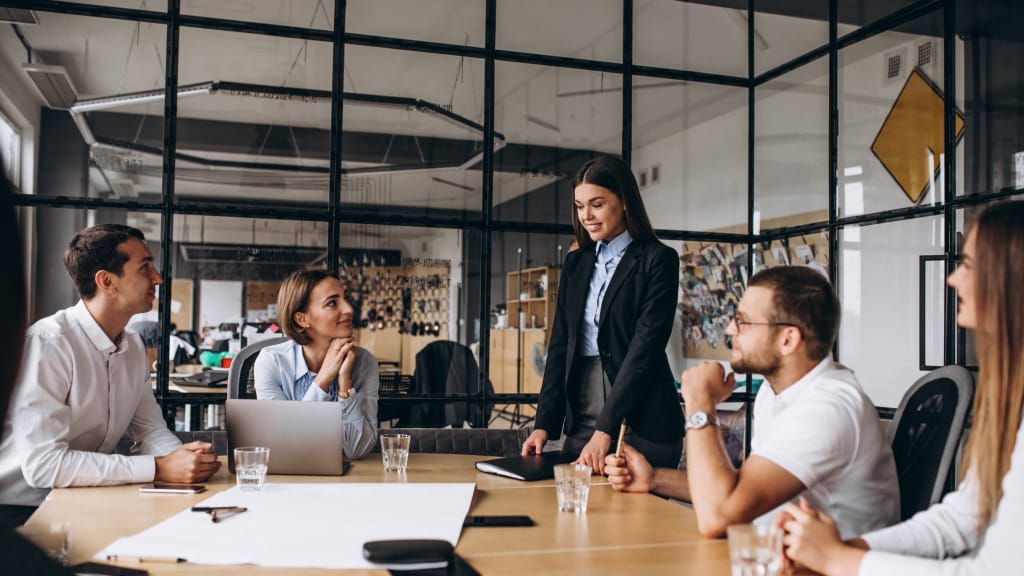
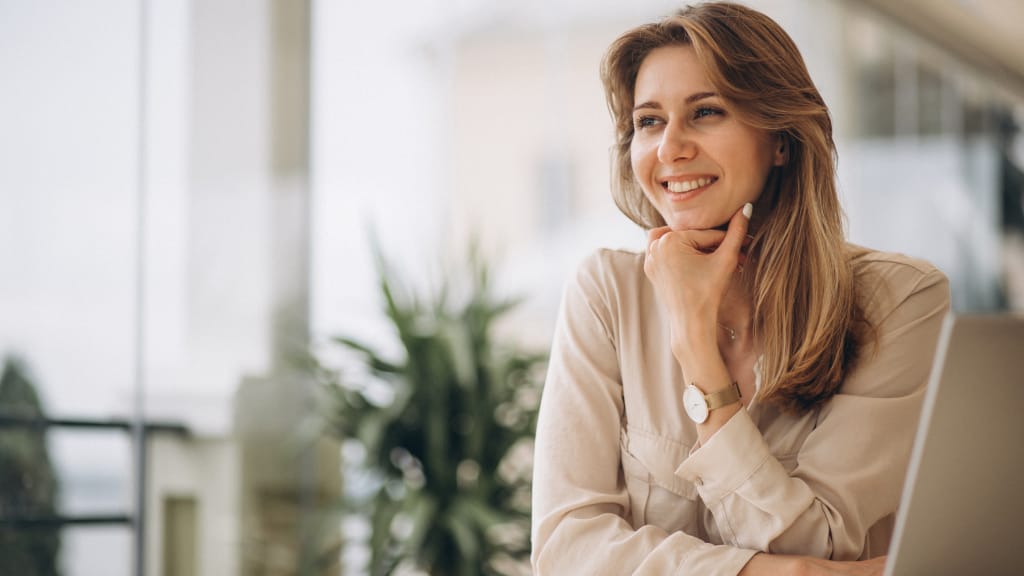
JavaScript Functions: Explained with Examples
Functions are an essential part of JavaScript that allow you to encapsulate reusable blocks of code. They enable you to organize and modularize your code, making it more maintainable and efficient. In this article, we will explore the concept of functions in JavaScript and provide examples of their usage.
Defining a Function
In JavaScript, you can define a function using the function
keyword. Here's an example of a simple function that adds two numbers:
function addNumbers(a, b) {
return a + b;
}
In this example, we define a function called addNumbers
that takes two parameters a
and b
. It returns the sum of the two parameters using the return
statement.
Calling a Function
After defining a function, you can call it by using its name followed by parentheses. Here's an example of calling the addNumbers
function:
let result = addNumbers(5, 3);
console.log(result);
In this example, we call the addNumbers
function with arguments 5
and 3
. The returned value is assigned to the variable result
, and then it is logged to the console, which will display 8
.
Function Parameters
Functions can accept parameters, which are variables that hold values passed to the function. You can define parameters in the function declaration and use them within the function body. Here's an example:
function greet(name) {
console.log("Hello, " + name + "!");
}
greet("John");
In this example, the greet
function takes a name
parameter and logs a greeting message using the passed value. When we call the function with "John"
, it will output Hello, John!
.
Function Return Values
Functions can also return values using the return
statement. Here's an example:
function multiply(a, b) {
return a * b;
}
let result = multiply(4, 5);
console.log(result);
In this example, the multiply
function takes two parameters a
and b
and returns their product. The returned value is assigned to the variable result
, and then it is logged to the console, which will display 20
.
Conclusion
Functions are fundamental building blocks in JavaScript that enable you to encapsulate and reuse code. In this article, we explored the concept of functions, how to define and call them, work with parameters, and return values. By utilizing functions effectively, you can create modular and reusable code, improving the organization and efficiency of your JavaScript programs.
4.5L
Learners
20+
Instructors
50+
Courses
6.0L
Course enrollments
Future Trending Courses
When selecting, a course, Here are a few areas that are expected to be in demand in the future:.
Future Learning for all
If you’re passionate and ready to dive in, we’d love to join 1:1 classes for you. We’re committed to support our learners and professionals their development and well-being.
View Courses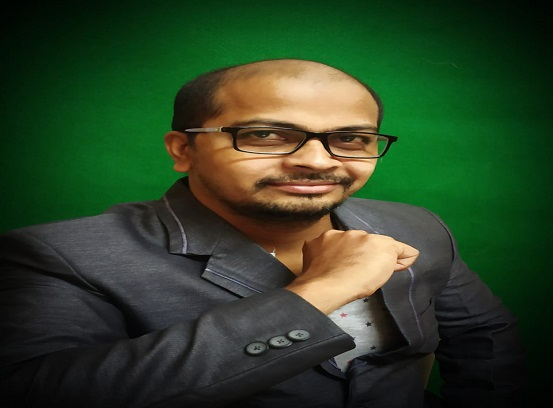
Most Popular Course topics
These are the most popular course topics among Software Courses for learners