Hi there, we’re Harisystems
"Unlock your potential and soar to new heights with our exclusive online courses! Ignite your passion, acquire valuable skills, and embrace limitless possibilities. Don't miss out on our limited-time sale - invest in yourself today and embark on a journey of personal and professional growth. Enroll now and shape your future with knowledge that lasts a lifetime!".
For corporate trainings, projects, and real world experience reach us. We believe that education should be accessible to all, regardless of geographical location or background.
1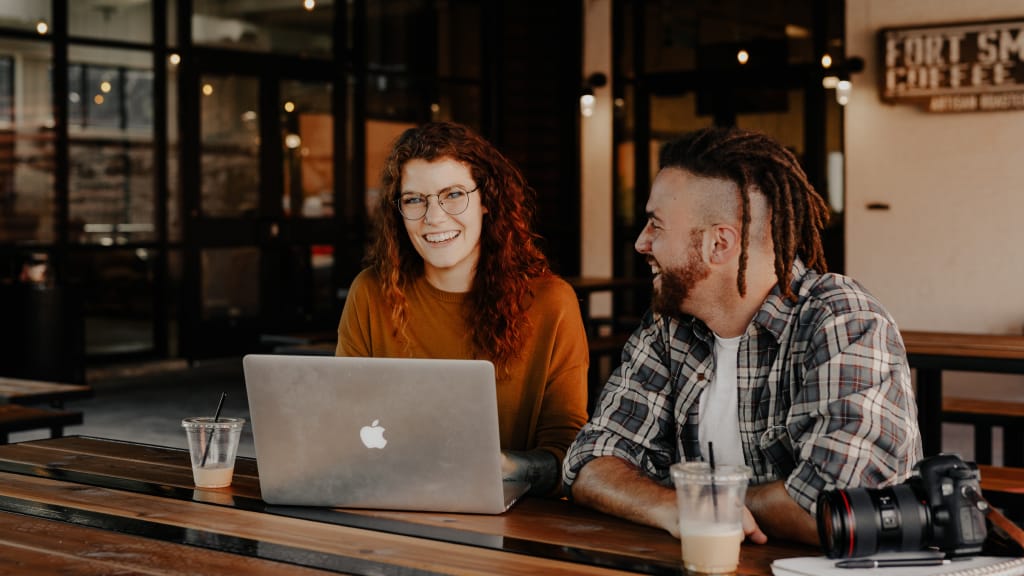
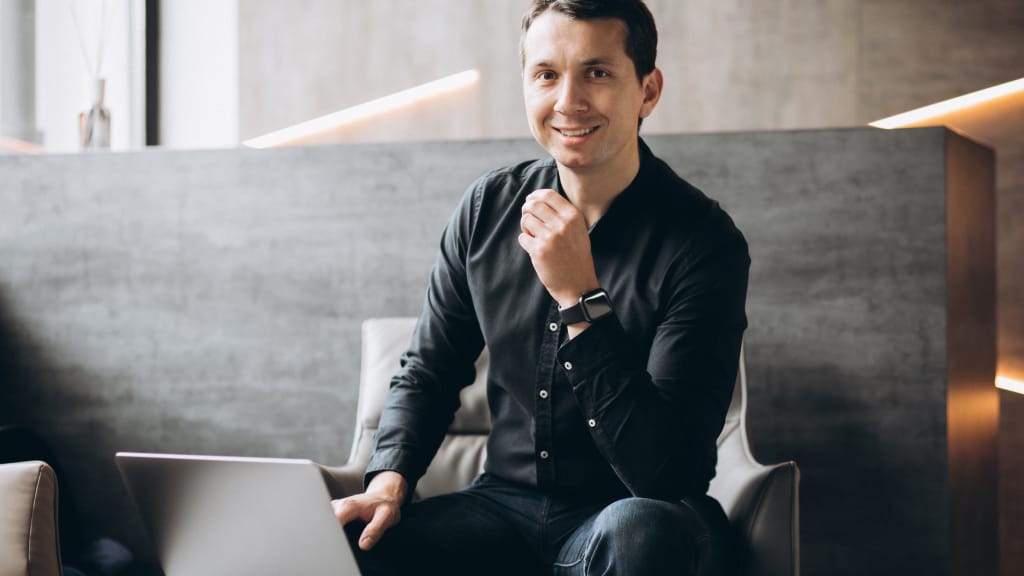
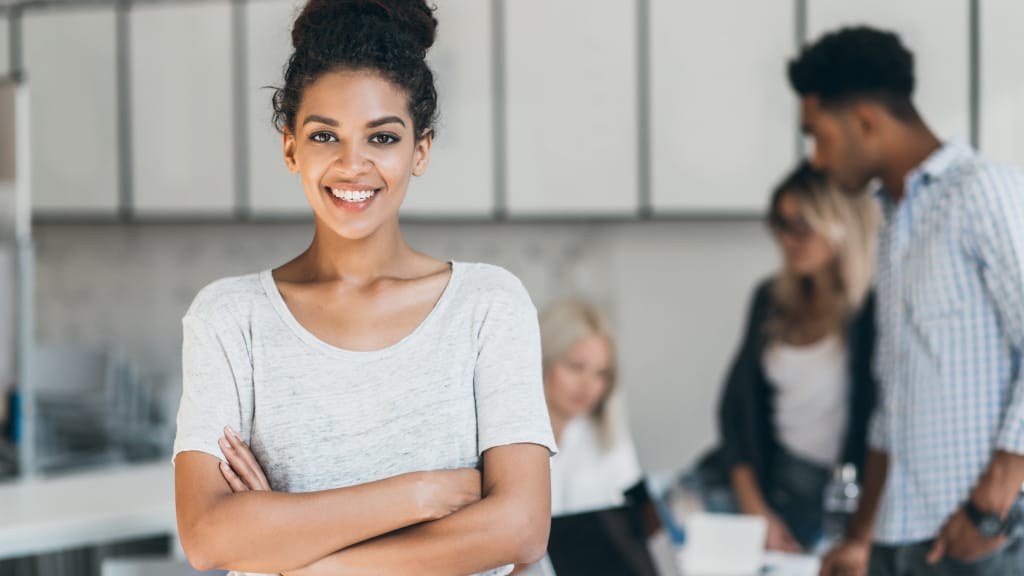
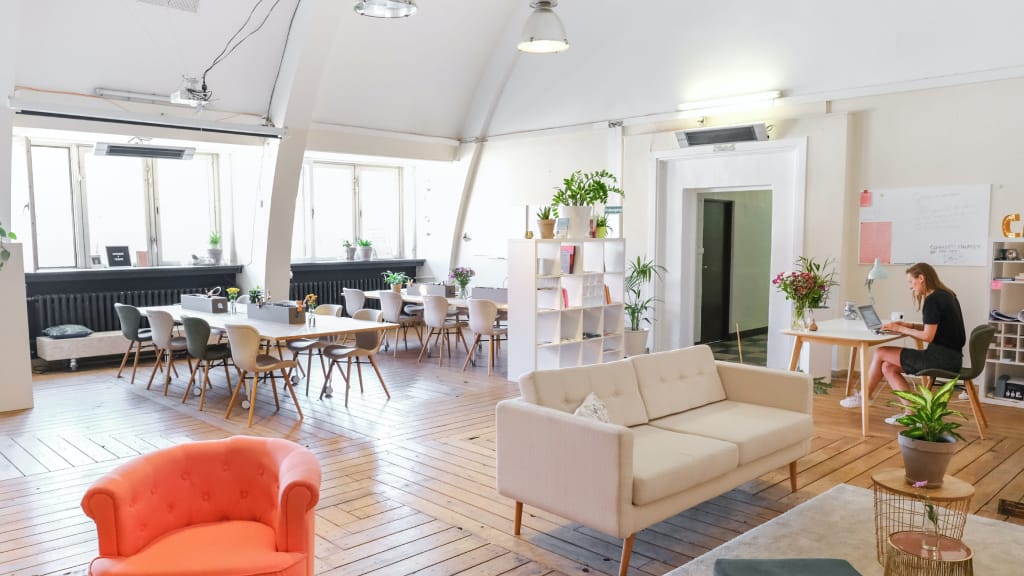
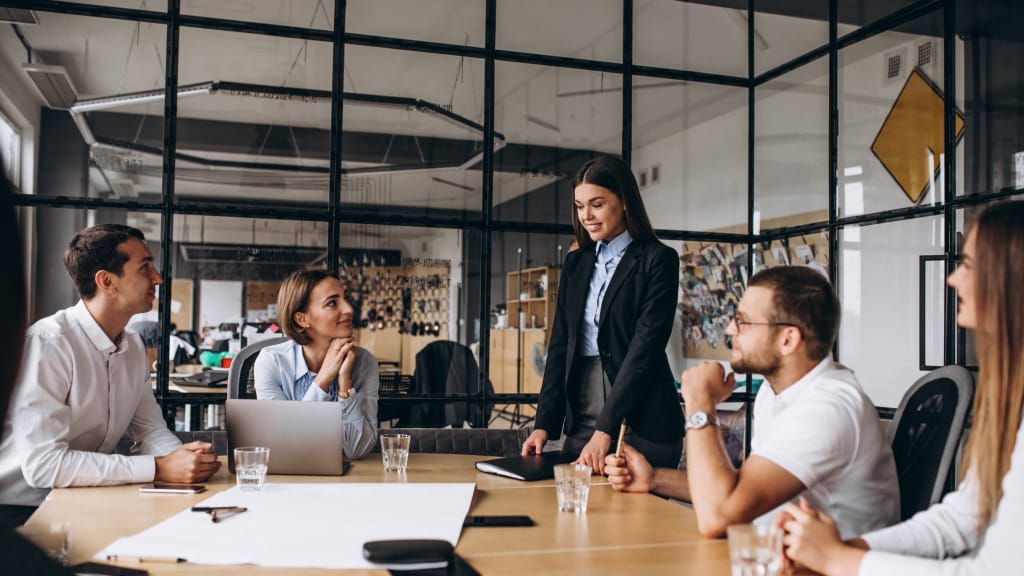
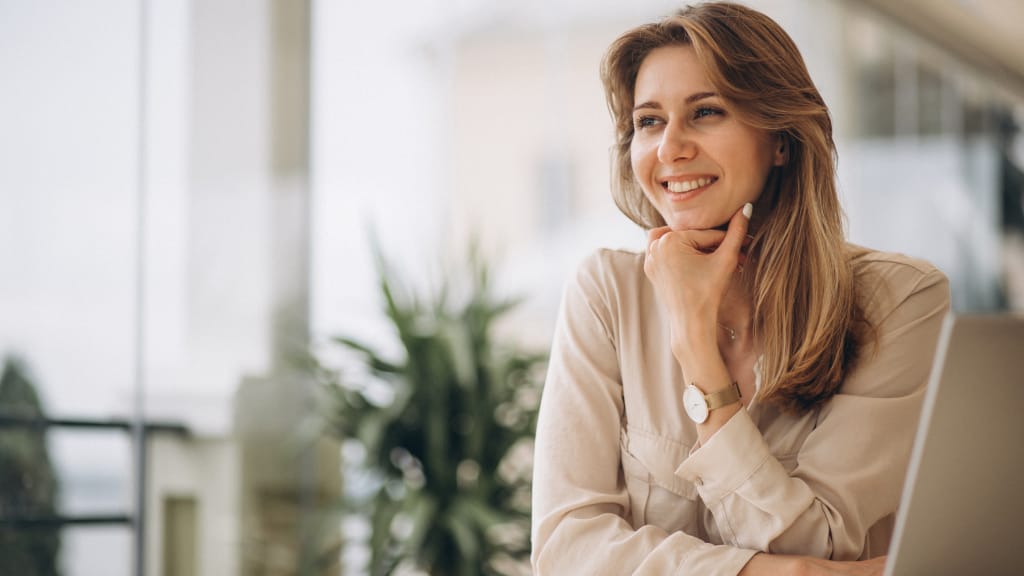
C++ Arrays
In C++, an array is a collection of elements of the same data type. It provides a convenient way to store and access multiple values using a single variable name. Arrays allow you to work with groups of related data and perform operations on them. In this article, we will explore the usage of arrays in C++ with examples.
1. Declaring and Accessing Arrays
To declare an array in C++, you need to specify the data type of the elements and the size of the array. Here's an example:
#include <iostream>
int main() {
int numbers[5];
numbers[0] = 10;
numbers[1] = 20;
numbers[2] = 30;
numbers[3] = 40;
numbers[4] = 50;
std::cout << "Element at index 0: " << numbers[0] << std::endl;
std::cout << "Element at index 2: " << numbers[2] << std::endl;
return 0;
}
In the above code, we declare an integer array numbers
with a size of 5. We then assign values to the individual elements of the array using index notation. Finally, we access and print the values of specific elements using their corresponding indices.
2. Initializing Arrays
You can initialize an array at the time of declaration. Here's an example:
#include <iostream>
int main() {
int numbers[] = {10, 20, 30, 40, 50};
std::cout << "Element at index 0: " << numbers[0] << std::endl;
std::cout << "Element at index 2: " << numbers[2] << std::endl;
return 0;
}
In the above code, we declare and initialize an integer array numbers
with the values specified inside the curly braces. The compiler automatically determines the size of the array based on the number of elements provided.
3. Array Traversal
You can use loops to traverse an array and perform operations on each element. Here's an example:
#include <iostream>
int main() {
int numbers[] = {10, 20, 30, 40, 50};
for (int i = 0; i < 5; i++) {
std::cout << "Element at index " << i << ": " << numbers[i] << std::endl;
}
return 0;
}
In the above code, we use a for loop to traverse the array numbers
and print each element along with its index.
4. Array Size and Bounds
In C++, arrays have a fixed size that is determined at the time of declaration. It is important to ensure that you do not access elements beyond the bounds of the array to avoid undefined behavior. Here's an example:
#include <iostream>
int main() {
int numbers[5] = {10, 20, 30, 40, 50};
std::cout << "Size of the array: " << sizeof(numbers) / sizeof(numbers[0]) << std::endl;
// Accessing an element beyond the array bounds
std::cout << "Element at index 10: " << numbers[10] << std::endl;
return 0;
}
In the above code, we calculate the size of the array numbers
using the sizeof
operator. We then attempt to access an element at index 10, which is beyond the bounds of the array. This results in undefined behavior and may produce unpredictable results.
Arrays are fundamental data structures in C++ that allow you to work with multiple values efficiently. Practice declaring, initializing, and accessing elements of arrays, and utilize loops for array traversal to manipulate and process data effectively in your C++ programs.
4.5L
Learners
20+
Instructors
50+
Courses
6.0L
Course enrollments
Future Trending Courses
When selecting, a course, Here are a few areas that are expected to be in demand in the future:.
Future Learning for all
If you’re passionate and ready to dive in, we’d love to join 1:1 classes for you. We’re committed to support our learners and professionals their development and well-being.
View Courses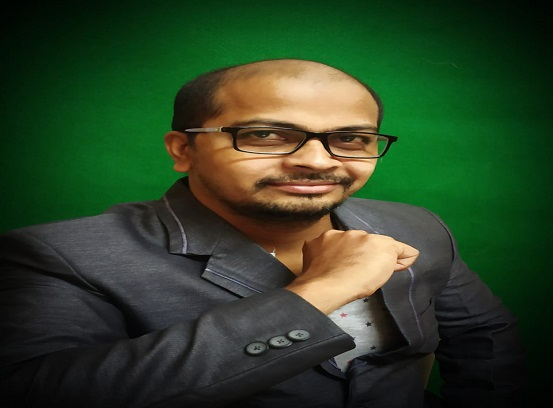
Most Popular Course topics
These are the most popular course topics among Software Courses for learners