Hi there, we’re Harisystems
"Unlock your potential and soar to new heights with our exclusive online courses! Ignite your passion, acquire valuable skills, and embrace limitless possibilities. Don't miss out on our limited-time sale - invest in yourself today and embark on a journey of personal and professional growth. Enroll now and shape your future with knowledge that lasts a lifetime!".
For corporate trainings, projects, and real world experience reach us. We believe that education should be accessible to all, regardless of geographical location or background.
1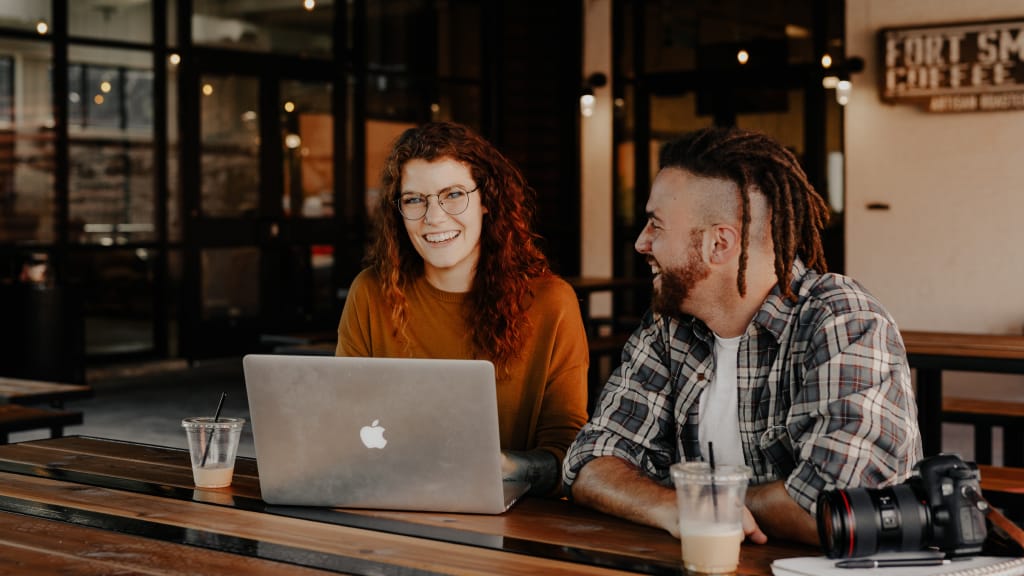
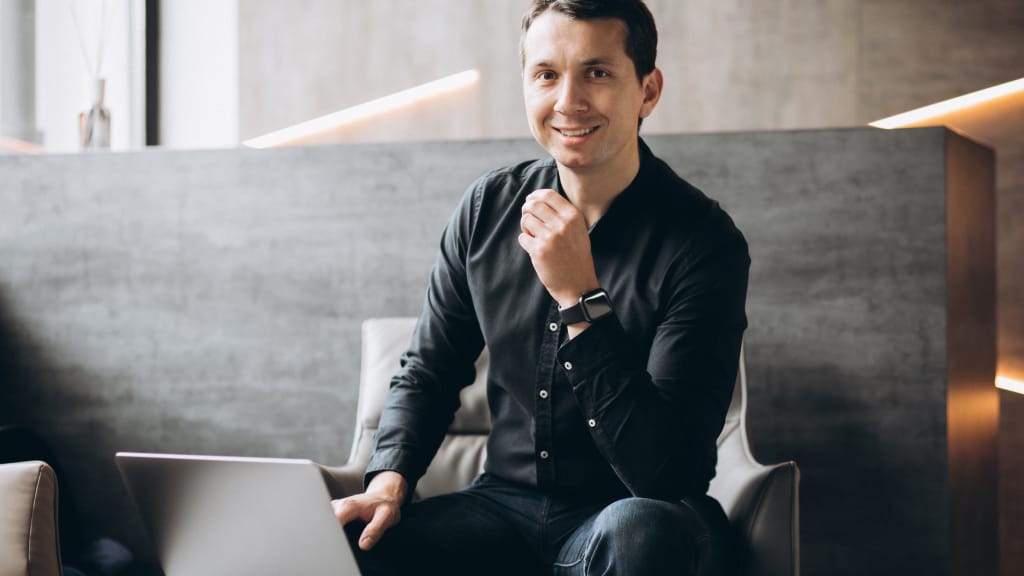
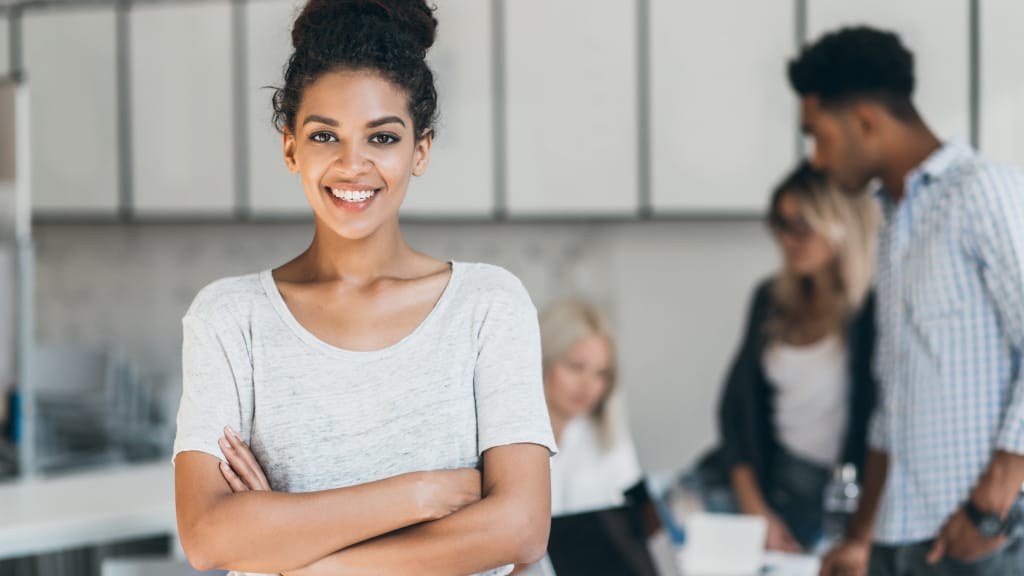
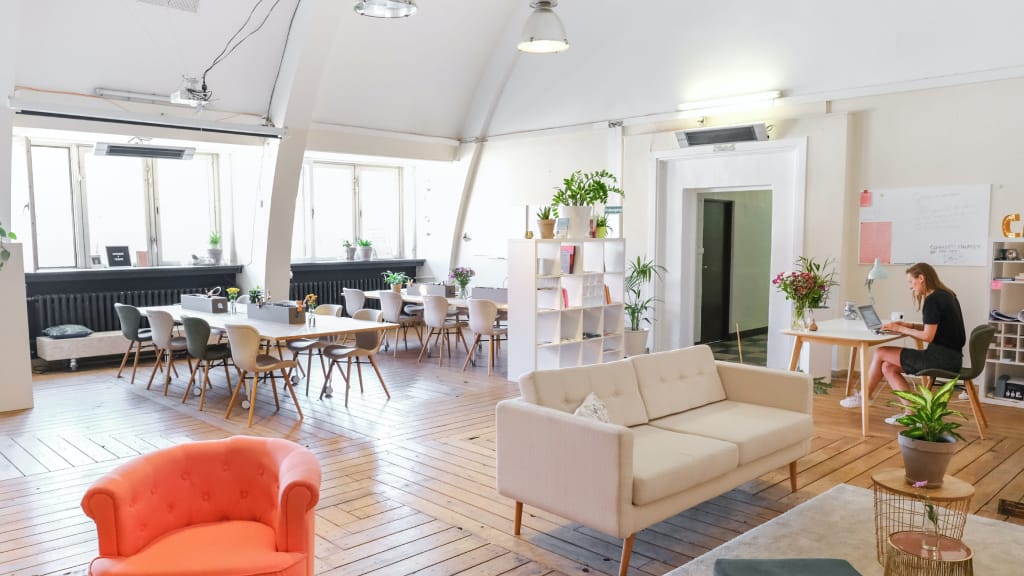
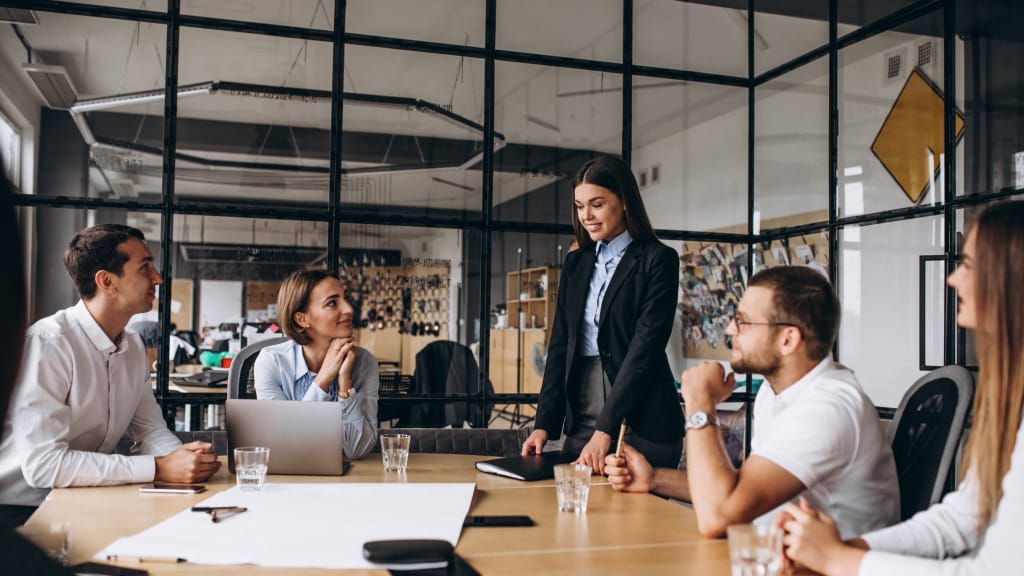
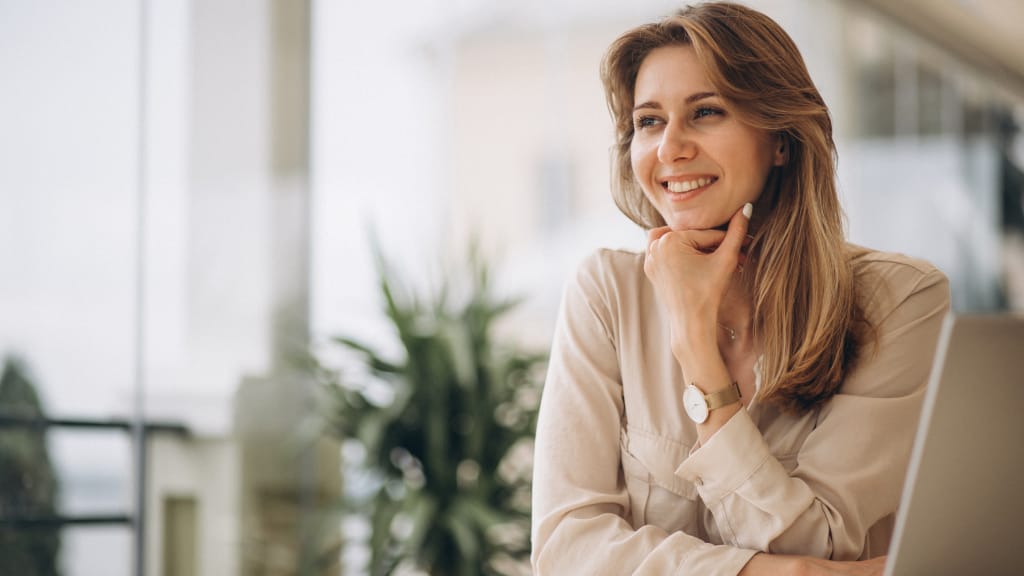
Web Development: Exploring XML in Web Development
XML (eXtensible Markup Language) is a widely used markup language that enables the structuring and representation of data in a human-readable and machine-readable format. Although XML is not typically used for web page content, it plays a vital role in various aspects of web development. In this article, we will explore the basics of XML and its applications in web development.
Understanding XML
XML follows a hierarchical structure with nested elements, similar to HTML. It allows developers to define custom tags and attributes to structure and organize data. Here's an example of an XML document:
<?xml version="1.0" encoding="UTF-8"?>
<bookstore>
<book category="fiction">
<title>Harry Potter</title>
<author>J.K. Rowling</author>
<year>2005</year>
</book>
<book category="non-fiction">
<title>The Web Developer's Guide</title>
<author>John Smith</author>
<year>2020</year>
</book>
</bookstore>
XML in Web Development
XML has several applications in web development:
- Data Exchange: XML is commonly used for data exchange between systems and platforms. It provides a standardized format for sharing structured data.
- Configuration Files: XML files are often used to store configuration settings for web applications, such as database connections or application preferences.
- Web Services: XML is a fundamental part of web services protocols like SOAP (Simple Object Access Protocol) and REST (Representational State Transfer). It allows data to be exchanged between client and server in a standardized format.
Processing XML in JavaScript
JavaScript provides built-in functionality to parse and process XML data. The following example demonstrates how to parse and access XML data using JavaScript:
<script>
var xmlString = `
<?xml version="1.0" encoding="UTF-8"?>
<bookstore>
<book category="fiction">
<title>Harry Potter</title>
<author>J.K. Rowling</author>
<year>2005</year>
</book>
<book category="non-fiction">
<title>The Web Developer's Guide</title>
<author>John Smith</author>
<year>2020</year>
</book>
</bookstore>
`;
var parser = new DOMParser();
var xmlDoc = parser.parseFromString(xmlString, "text/xml");
var books = xmlDoc.getElementsByTagName("book");
for (var i = 0; i < books.length; i++) {
var title = books[i].getElementsByTagName("title")[0].textContent;
var author = books[i].getElementsByTagName("author")[0].textContent;
var year = books[i].getElementsByTagName("year")[0].textContent;
console.log("Title: " + title);
console.log("Author: " + author);
console.log("Year: " + year);
console.log("--------");
}
</script>
Conclusion
XML is a versatile markup language that finds applications in various aspects of web development. Whether it's for data exchange, configuration files, or web services, XML provides a standardized and structured format for storing and transmitting data. Understanding XML and its role in web development can greatly enhance your ability to work with data-driven applications and integrations in the web development landscape.
4.5L
Learners
20+
Instructors
50+
Courses
6.0L
Course enrollments
Future Trending Courses
When selecting, a course, Here are a few areas that are expected to be in demand in the future:.
Future Learning for all
If you’re passionate and ready to dive in, we’d love to join 1:1 classes for you. We’re committed to support our learners and professionals their development and well-being.
View Courses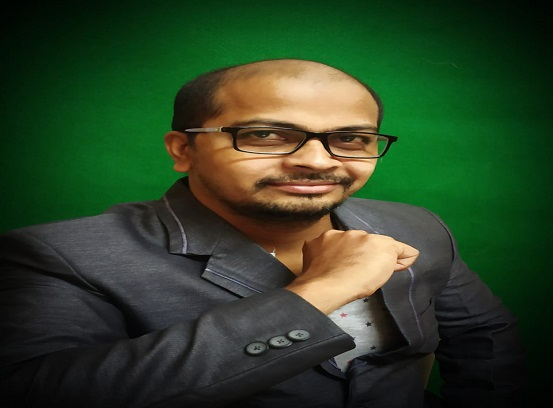
Most Popular Course topics
These are the most popular course topics among Software Courses for learners