Hi there, we’re Harisystems
"Unlock your potential and soar to new heights with our exclusive online courses! Ignite your passion, acquire valuable skills, and embrace limitless possibilities. Don't miss out on our limited-time sale - invest in yourself today and embark on a journey of personal and professional growth. Enroll now and shape your future with knowledge that lasts a lifetime!".
For corporate trainings, projects, and real world experience reach us. We believe that education should be accessible to all, regardless of geographical location or background.
1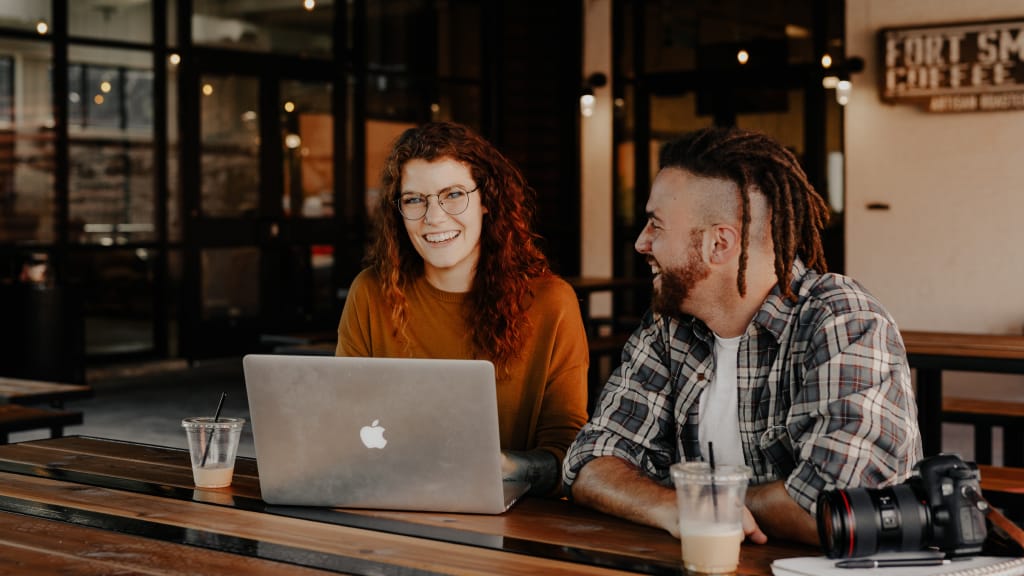
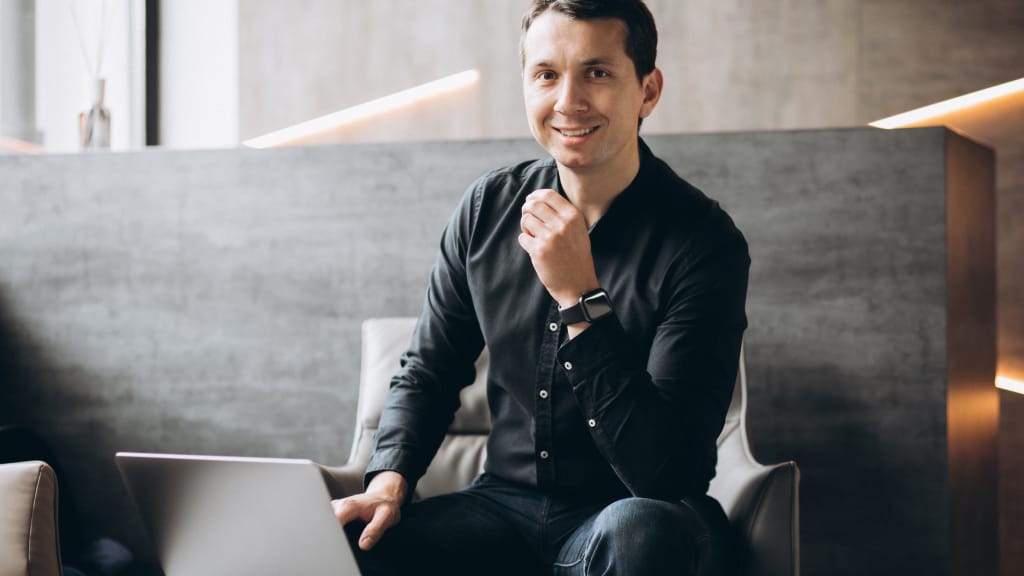
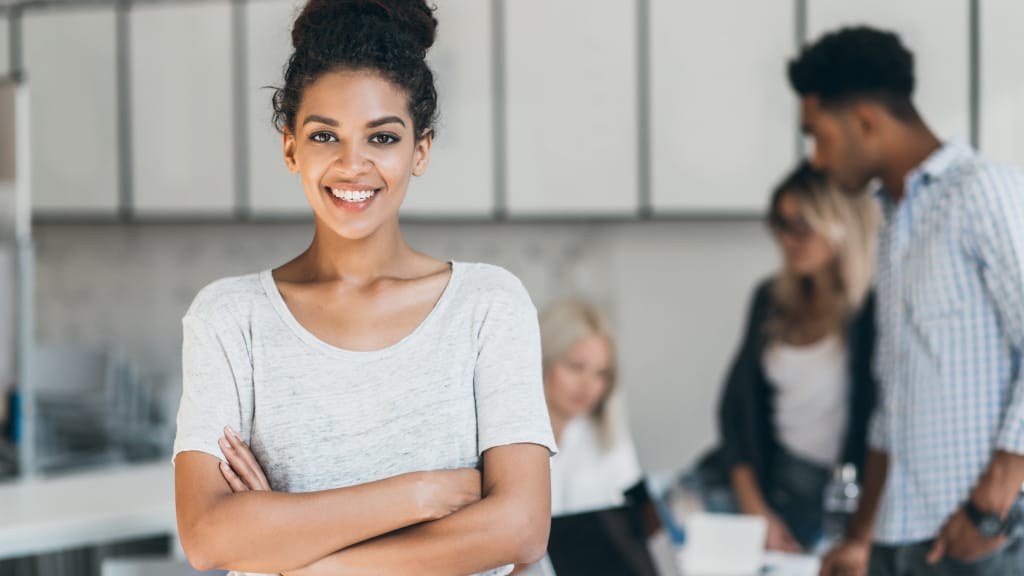
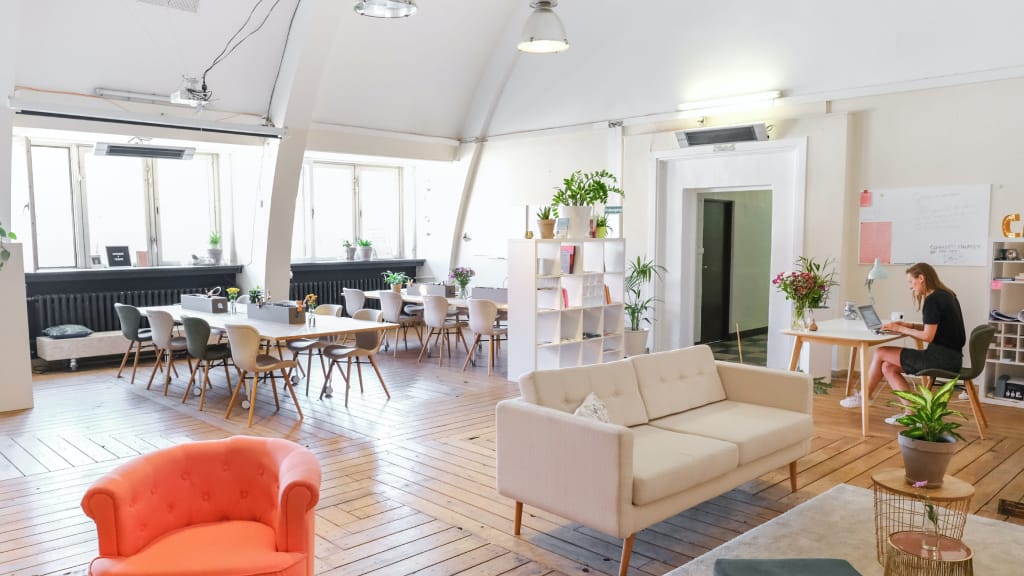
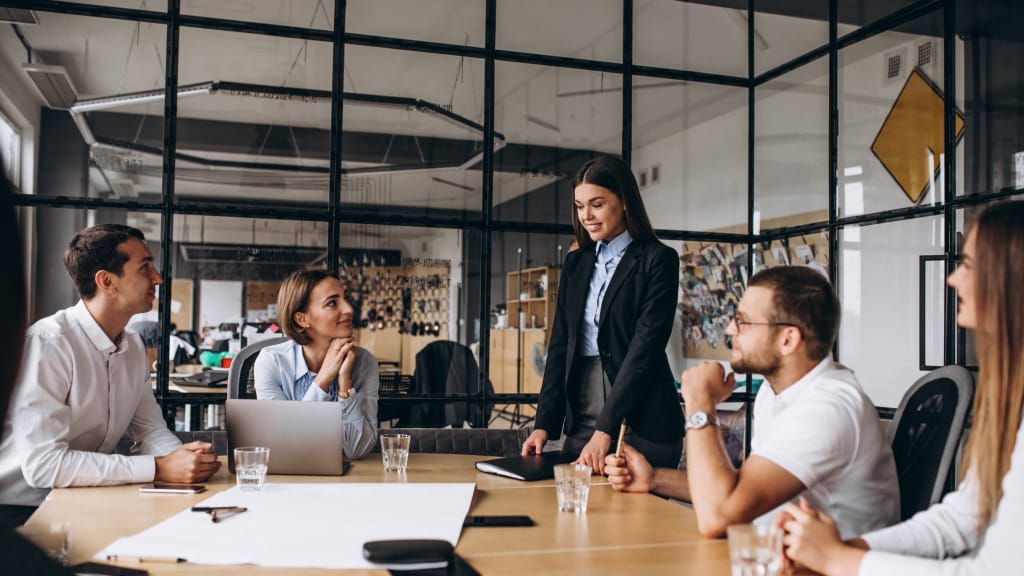
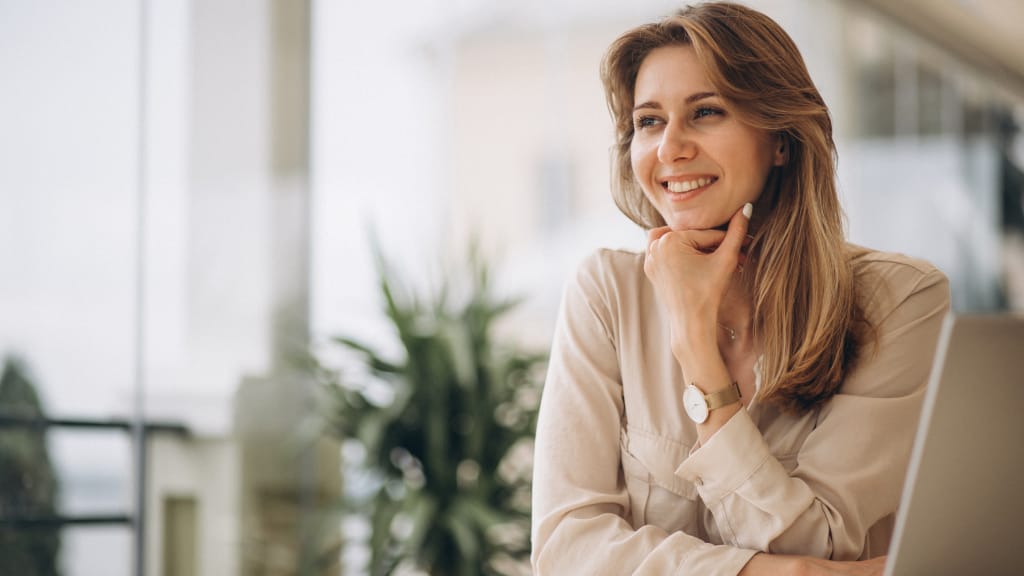
Java Booleans: Working with Logical Values
In Java, the boolean
type is used to represent logical values. A boolean
variable can have one of two values: true
or false
. Booleans are often used in decision-making and conditional statements to control the flow of a program. In this article, we will explore the concept of booleans in Java and provide examples to help you understand their usage.
Declaring and Assigning Booleans
In Java, you can declare and assign boolean variables using the boolean
keyword. Here's an example:
boolean isRaining = true;
boolean isSunny = false;
In the above code, the variables isRaining
and isSunny
are declared as booleans and assigned the values true
and false
, respectively.
Boolean Expressions
Boolean expressions are expressions that evaluate to a boolean value (true
or false
). They are commonly used in conditional statements and logical operations. Here are some examples:
boolean isTrue = 5 > 3; // true
boolean isFalse = 10 == 5; // false
In the above code, the expressions 5 > 3
and 10 == 5
evaluate to true
and false
, respectively.
Logical Operators
Java provides several logical operators for working with boolean values. Here are the commonly used logical operators:
- AND (&&): Returns
true
if both operands aretrue
. Example:boolean result = true && false;
- OR (||): Returns
true
if at least one of the operands istrue
. Example:boolean result = true || false;
- NOT (!): Negates the value of the operand. Example:
boolean result = !true;
Here's an example that demonstrates the use of logical operators:
boolean isSunny = true;
boolean isWarm = false;
boolean isPerfectDay = isSunny && isWarm; // false
In the above code, the isPerfectDay
variable is assigned the value false
because the expression isSunny && isWarm
evaluates to false
(since isWarm
is false
).
Conditional Statements
Booleans are commonly used in conditional statements to control the flow of a program. The if
statement is a fundamental conditional statement in Java. Here's an example:
boolean isSunny = true;
if (isSunny) {
System.out.println("It's a sunny day!");
} else {
System.out.println("It's not sunny today.");
}
In the above code, if the isSunny
variable is true
, the message "It's a sunny day!"
is printed. Otherwise, the message "It's not sunny today."
is printed.
Conclusion
Booleans are fundamental to programming logic and decision-making. In this article, we explored the concept of booleans in Java and their usage. We learned how to declare and assign boolean variables, work with boolean expressions and logical operators, and use booleans in conditional statements. By understanding and effectively utilizing booleans, you can control the flow of your Java programs and make informed decisions based on logical conditions. Continuously practice using booleans and explore more advanced topics, such as boolean algebra and truth tables, to enhance your logical thinking and programming skills in Java.
4.5L
Learners
20+
Instructors
50+
Courses
6.0L
Course enrollments
Future Trending Courses
When selecting, a course, Here are a few areas that are expected to be in demand in the future:.
Future Learning for all
If you’re passionate and ready to dive in, we’d love to join 1:1 classes for you. We’re committed to support our learners and professionals their development and well-being.
View Courses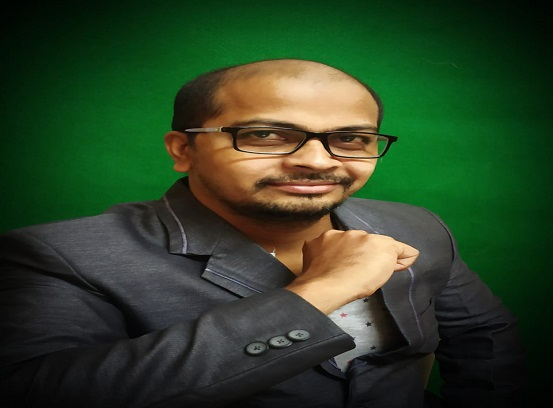
Most Popular Course topics
These are the most popular course topics among Software Courses for learners