Hi there, we’re Harisystems
"Unlock your potential and soar to new heights with our exclusive online courses! Ignite your passion, acquire valuable skills, and embrace limitless possibilities. Don't miss out on our limited-time sale - invest in yourself today and embark on a journey of personal and professional growth. Enroll now and shape your future with knowledge that lasts a lifetime!".
For corporate trainings, projects, and real world experience reach us. We believe that education should be accessible to all, regardless of geographical location or background.
1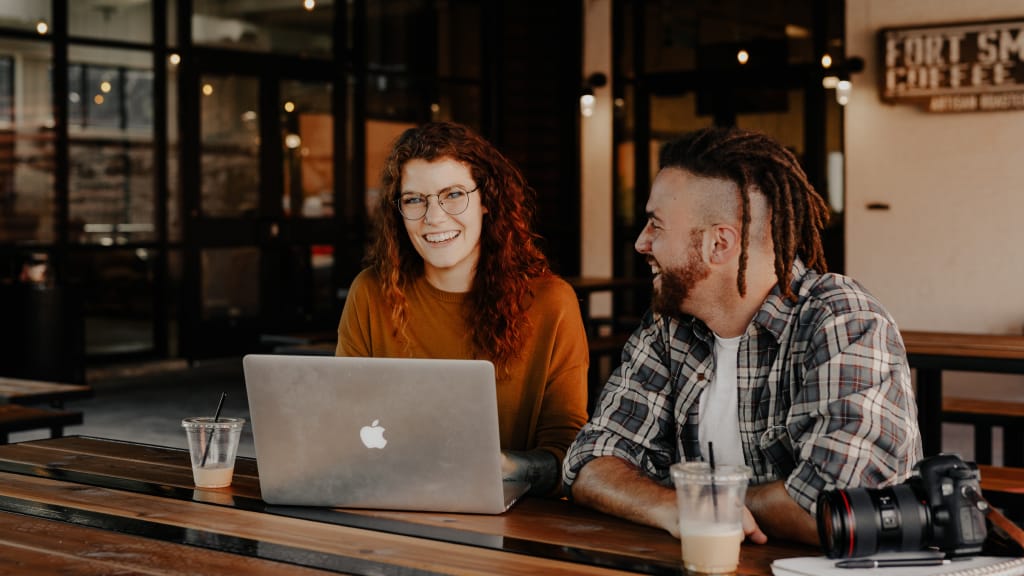
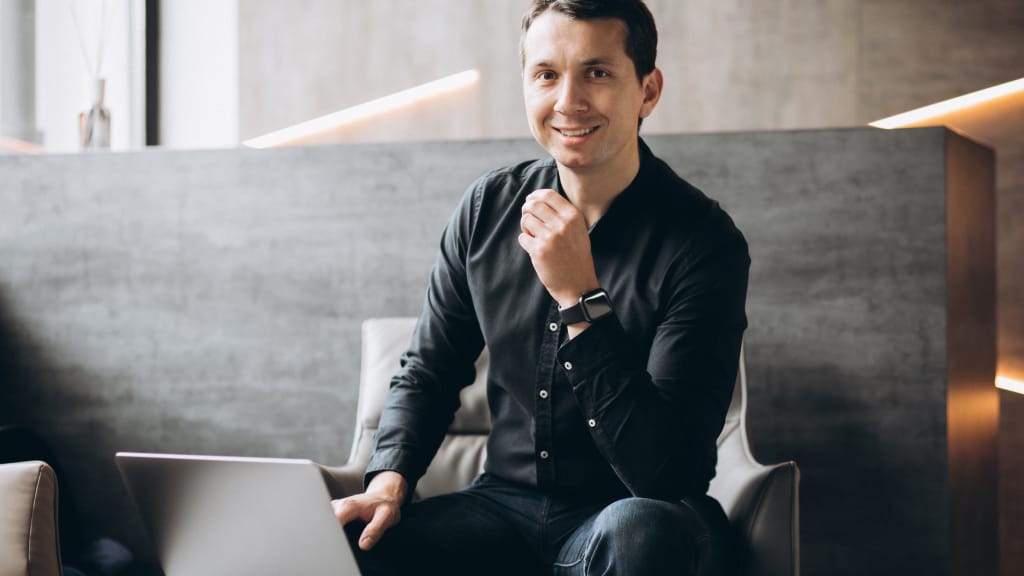
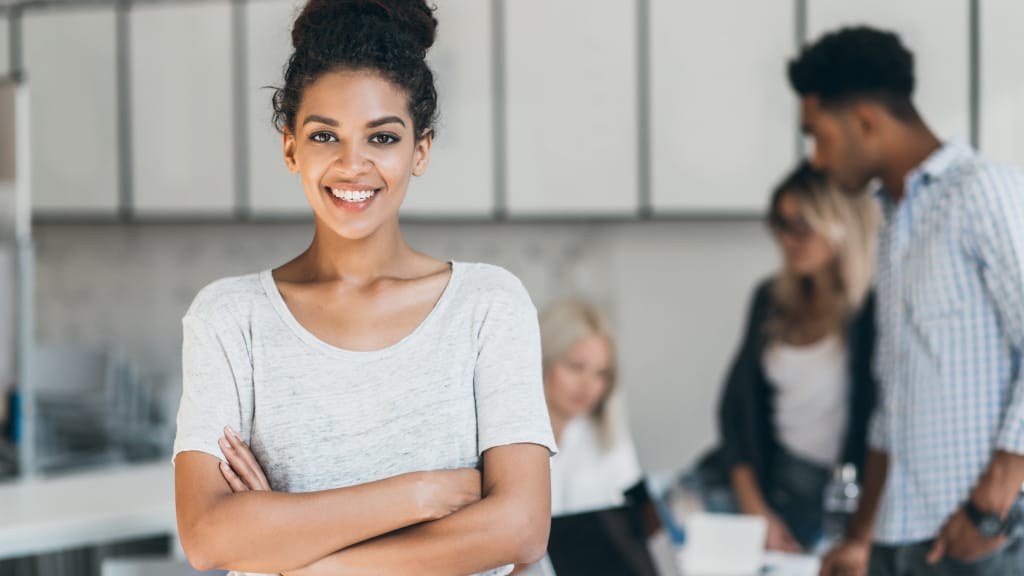
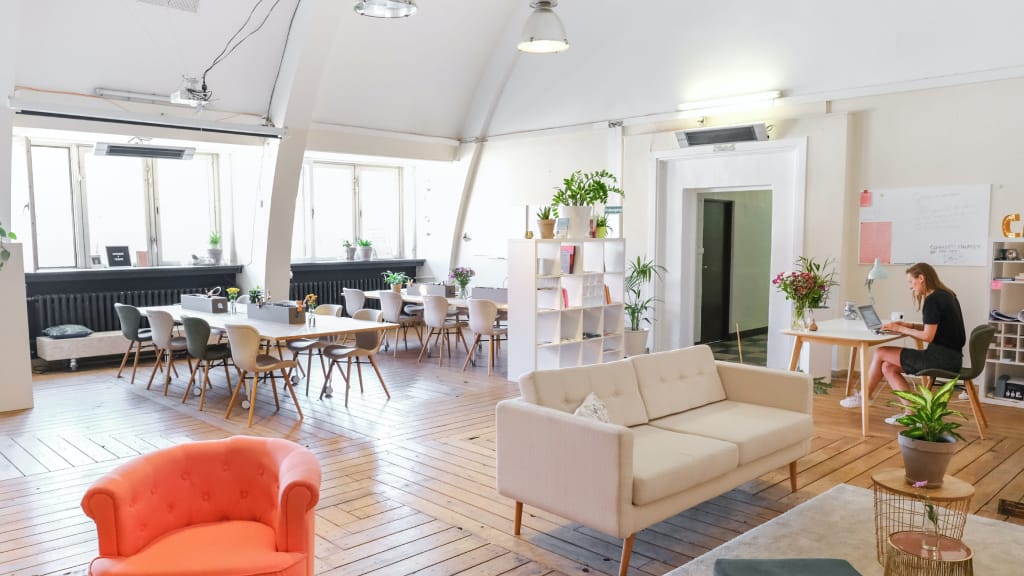
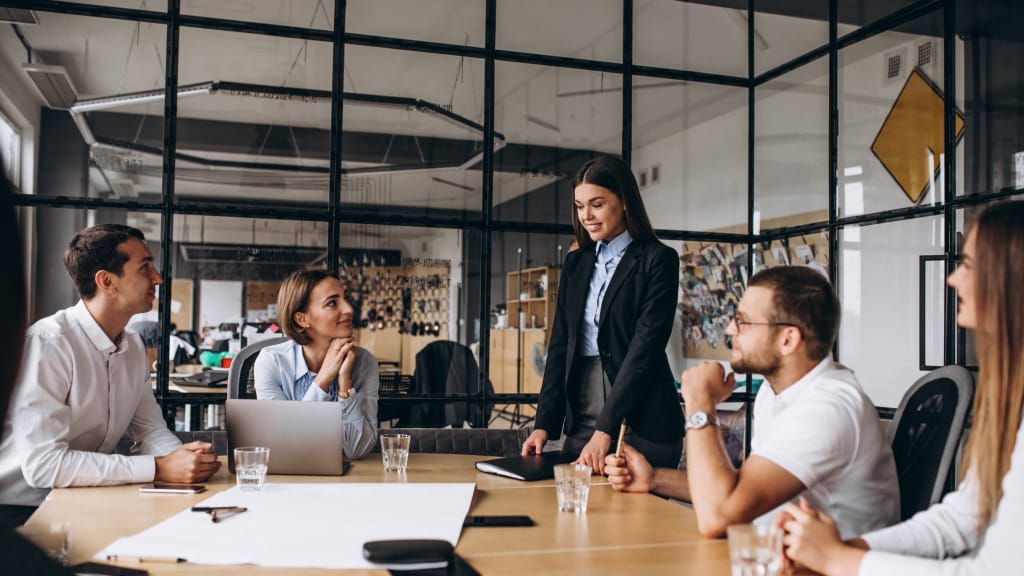
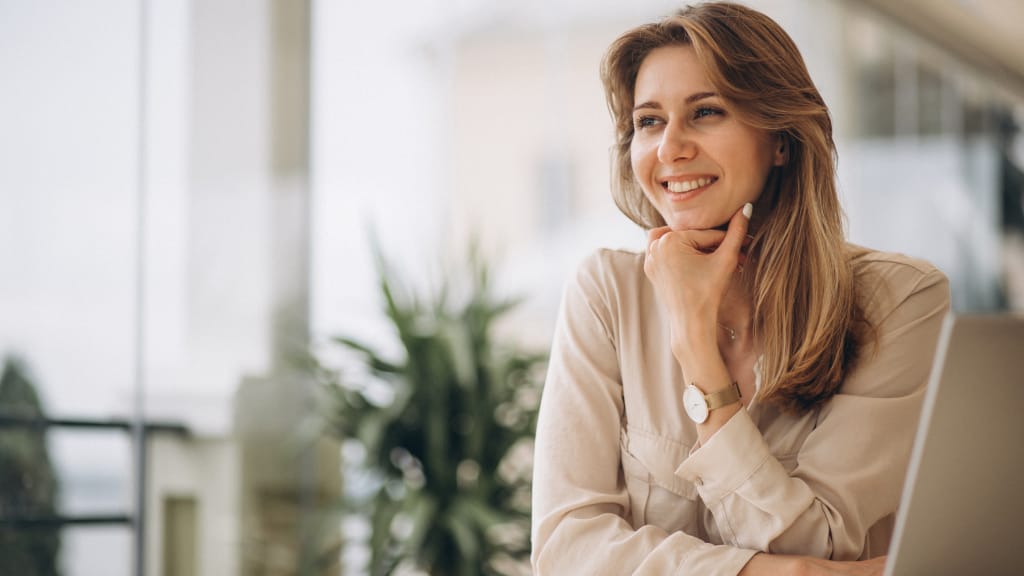
C++ Polymorphism
Polymorphism is a fundamental concept in object-oriented programming (OOP) that allows objects of different classes to be treated as objects of a common base class. It promotes code flexibility, extensibility, and enables dynamic binding of functions at runtime. In this article, we will explore the usage of polymorphism in C++ with examples.
1. Virtual Functions
In C++, a virtual function is a member function of a base class that can be overridden by a derived class. Virtual functions enable dynamic binding, where the appropriate function is determined at runtime based on the actual object type. Here's an example:
#include <iostream>
class Shape {
public:
virtual void displayInfo() {
std::cout << "This is a shape." << std::endl;
}
};
class Circle : public Shape {
public:
void displayInfo() override {
std::cout << "This is a circle." << std::endl;
}
};
class Rectangle : public Shape {
public:
void displayInfo() override {
std::cout << "This is a rectangle." << std::endl;
}
};
int main() {
Shape* shape1 = new Circle();
Shape* shape2 = new Rectangle();
shape1->displayInfo();
shape2->displayInfo();
delete shape1;
delete shape2;
return 0;
}
In the above code, we define a base class Shape
with a virtual member function displayInfo()
. We then define two derived classes, Circle
and Rectangle
, that override the displayInfo()
function. In the main()
function, we create pointers of type Shape
and assign them to objects of the derived classes. We then call the displayInfo()
function using the pointers, which invokes the appropriate overridden function at runtime.
2. Pure Virtual Functions
A pure virtual function is a virtual function that has no implementation in the base class and must be overridden by the derived classes. It serves as a placeholder for a function that must be implemented in the derived classes. Here's an example:
#include <iostream>
class Animal {
public:
virtual void makeSound() = 0;
};
class Dog : public Animal {
public:
void makeSound() override {
std::cout << "The dog barks." << std::endl;
}
};
class Cat : public Animal {
public:
void makeSound() override {
std::cout << "The cat meows." << std::endl;
}
};
int main() {
Animal* animal1 = new Dog();
Animal* animal2 = new Cat();
animal1->makeSound();
animal2->makeSound();
delete animal1;
delete animal2;
return 0;
}
In the above code, we define a base class Animal
with a pure virtual function makeSound()
. The derived classes Dog
and Cat
override the makeSound()
function with their respective implementations. In the main()
function, we create pointers of type Animal
and assign them to objects of the derived classes. We then call the makeSound()
function using the pointers, which invokes the appropriate overridden function at runtime.
3. Polymorphic Behavior
Polymorphism allows objects of different derived classes to be treated as objects of the base class. This enables writing code that can operate on objects of multiple types without the need for explicit type checking. Here's an example:
#include <iostream>
class Shape {
public:
virtual double calculateArea() = 0;
};
class Rectangle : public Shape {
private:
double length;
double width;
public:
Rectangle(double _length, double _width) : length(_length), width(_width) {}
double calculateArea() override {
return length * width;
}
};
class Circle : public Shape {
private:
double radius;
public:
Circle(double _radius) : radius(_radius) {}
double calculateArea() override {
return 3.14159 * radius * radius;
}
};
int main() {
Shape* shape1 = new Rectangle(5.0, 3.0);
Shape* shape2 = new Circle(2.5);
double area1 = shape1->calculateArea();
double area2 = shape2->calculateArea();
std::cout << "Area of rectangle: " << area1 << std::endl;
std::cout << "Area of circle: " << area2 << std::endl;
delete shape1;
delete shape2;
return 0;
}
In the above code, we define a base class Shape
with a pure virtual function calculateArea()
. The derived classes Rectangle
and Circle
override the calculateArea()
function with their respective implementations. In the main()
function, we create pointers of type Shape
and assign them to objects of the derived classes. We then call the calculateArea()
function using the pointers, which invokes the appropriate overridden function at runtime, giving us the polymorphic behavior.
Polymorphism is a powerful feature in C++ that promotes code flexibility, extensibility, and enables dynamic binding of functions at runtime. Utilize polymorphism to write modular, reusable, and flexible code that can handle objects of different types in a unified manner.
4.5L
Learners
20+
Instructors
50+
Courses
6.0L
Course enrollments
Future Trending Courses
When selecting, a course, Here are a few areas that are expected to be in demand in the future:.
Future Learning for all
If you’re passionate and ready to dive in, we’d love to join 1:1 classes for you. We’re committed to support our learners and professionals their development and well-being.
View Courses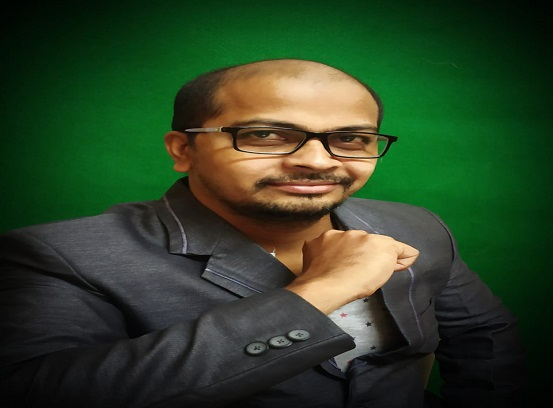
Most Popular Course topics
These are the most popular course topics among Software Courses for learners