Hi there, we’re Harisystems
"Unlock your potential and soar to new heights with our exclusive online courses! Ignite your passion, acquire valuable skills, and embrace limitless possibilities. Don't miss out on our limited-time sale - invest in yourself today and embark on a journey of personal and professional growth. Enroll now and shape your future with knowledge that lasts a lifetime!".
For corporate trainings, projects, and real world experience reach us. We believe that education should be accessible to all, regardless of geographical location or background.
1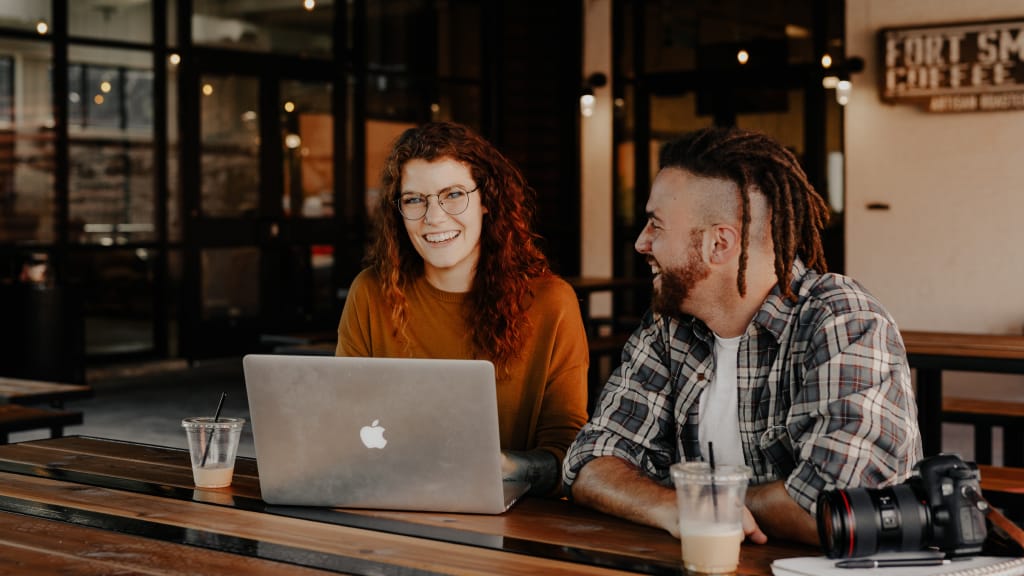
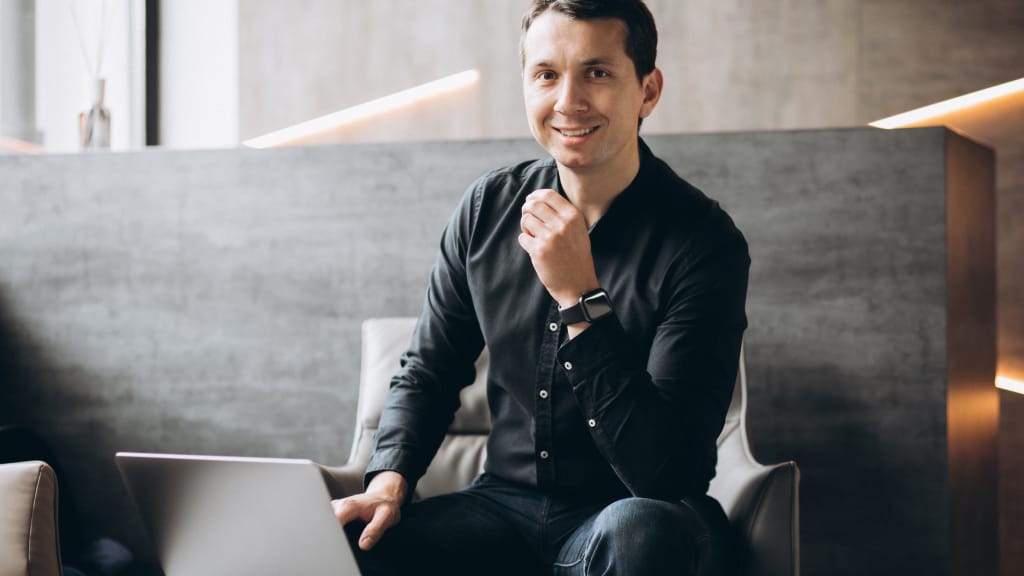
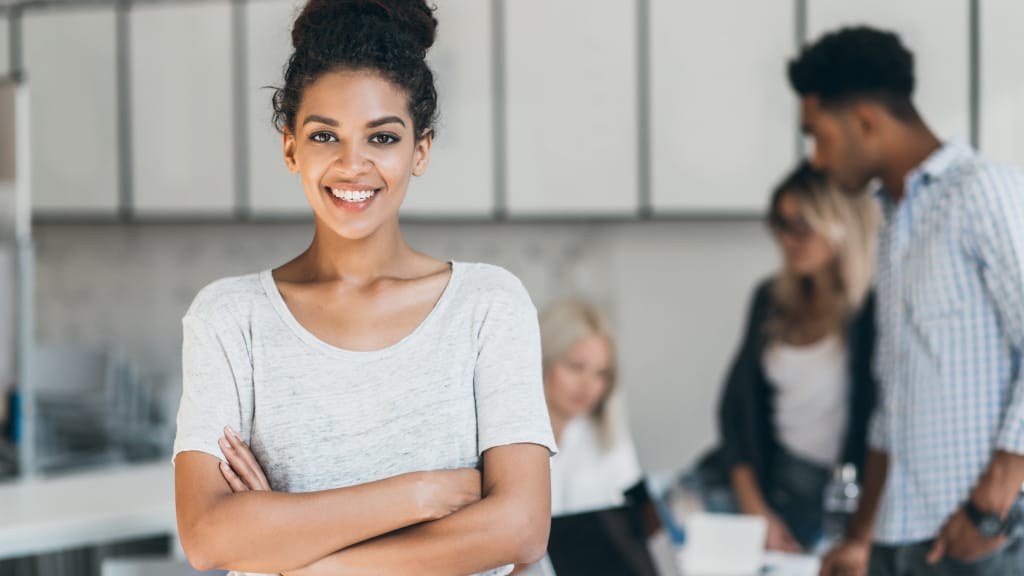
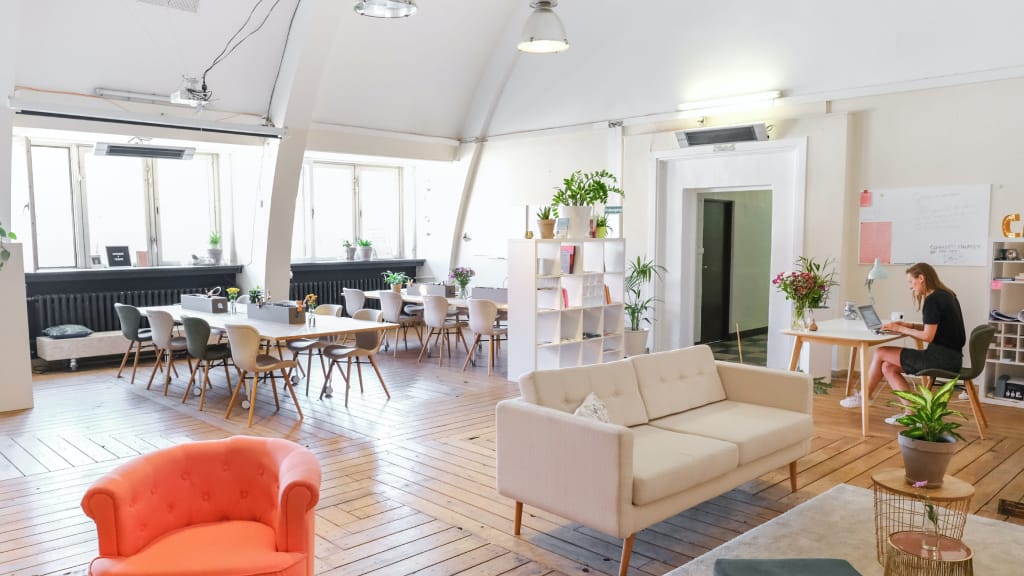
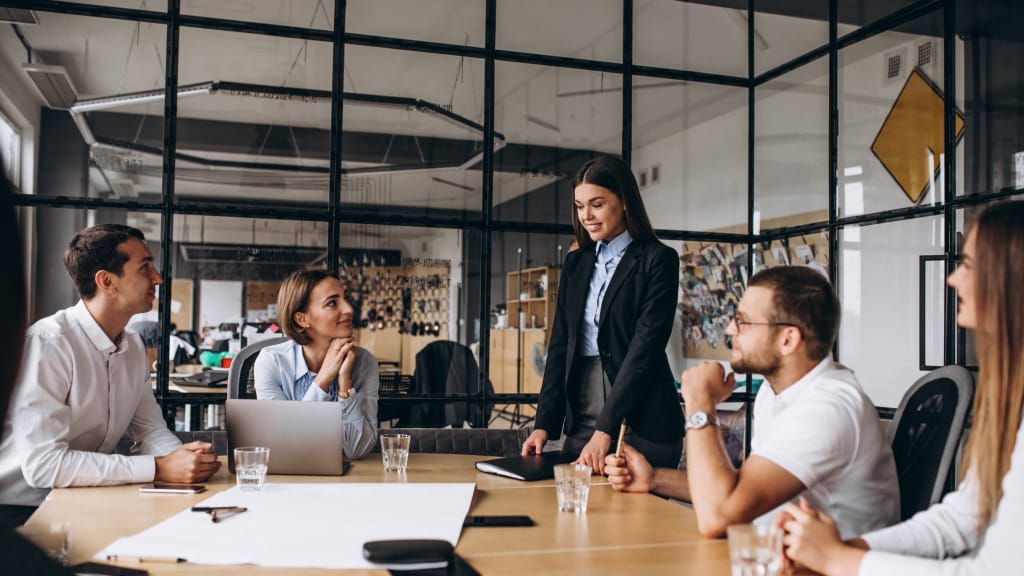
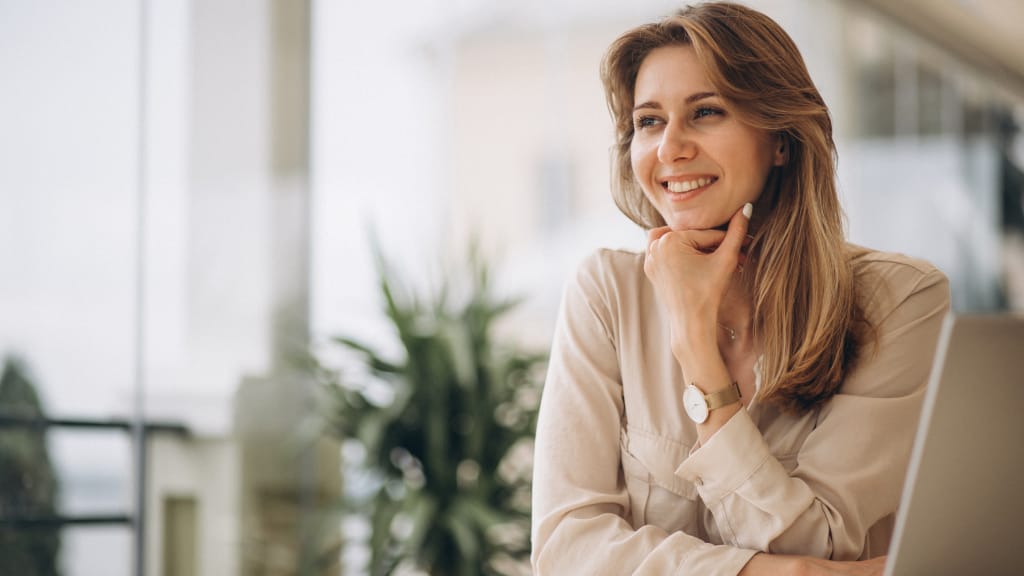
Python Abstract Classes
Abstract classes are an important concept in object-oriented programming (OOP) that provide a way to define common interfaces and enforce certain methods to be implemented by the subclasses. They serve as blueprints for other classes and cannot be instantiated directly. In this article, we'll explore the concept of abstract classes in Python and provide examples to help you understand their implementation and usage.
Example 1: Creating an Abstract Class
Let's start with a basic example of creating an abstract class:
from abc import ABC, abstractmethod
class Shape(ABC):
@abstractmethod
def draw(self):
pass
class Circle(Shape):
def draw(self):
print("Drawing a circle.")
circle = Circle()
circle.draw() # Output: Drawing a circle.
In this code, we define an abstract class called "Shape" by inheriting from the ABC class provided by the "abc" module. We use the "@abstractmethod" decorator to indicate that the "draw" method must be implemented by any subclass of Shape. We then create a subclass called "Circle" that overrides the "draw" method and provides its own implementation. Although we cannot instantiate the Shape class directly, we can create an instance of the Circle class and call the "draw" method.
Example 2: Abstract Properties
Abstract classes can also have abstract properties, which are properties that must be implemented by the subclasses. Here's an example:
from abc import ABC, abstractproperty
class Vehicle(ABC):
@abstractproperty
def wheels(self):
pass
class Car(Vehicle):
@property
def wheels(self):
return 4
car = Car()
print(car.wheels) # Output: 4
In this code, we define an abstract class called "Vehicle" and an abstract property called "wheels". Any subclass of Vehicle must implement the "wheels" property. We create a subclass called "Car" that overrides the "wheels" property and provides its own implementation. We create an instance of the Car class and access the "wheels" property, which returns the value 4.
Example 3: Using Abstract Methods in Concrete Classes
Abstract methods can also be used in concrete classes to enforce the implementation of certain methods. Here's an example:
from abc import ABC, abstractmethod
class Animal:
def sound(self):
print("Animal sound.")
class Dog(Animal, ABC):
@abstractmethod
def sound(self):
pass
class LabradorRetriever(Dog):
def sound(self):
print("Bark!")
dog = LabradorRetriever()
dog.sound() # Output: Bark!
In this code, we have a concrete class called "Animal" that provides a default implementation for the "sound" method. We create a subclass called "Dog" that inherits from Animal and also inherits from the ABC class to make it an abstract class. We use the "@abstractmethod" decorator to enforce the implementation of the "sound" method in any subclass of Dog. We create a subclass called "LabradorRetriever" that overrides the "sound" method and provides its own implementation. We create an instance of the LabradorRetriever class and call the "sound" method, which outputs "Bark!".
Conclusion
Abstract classes provide a way to define common interfaces and enforce certain methods to be implemented by subclasses. They serve as blueprints for other classes and cannot be instantiated directly. By understanding and utilizing abstract classes in Python, you can design more structured and extensible code. Experiment with the examples provided and explore the possibilities of abstract classes in your Python projects.
4.5L
Learners
20+
Instructors
50+
Courses
6.0L
Course enrollments
Future Trending Courses
When selecting, a course, Here are a few areas that are expected to be in demand in the future:.
Future Learning for all
If you’re passionate and ready to dive in, we’d love to join 1:1 classes for you. We’re committed to support our learners and professionals their development and well-being.
View Courses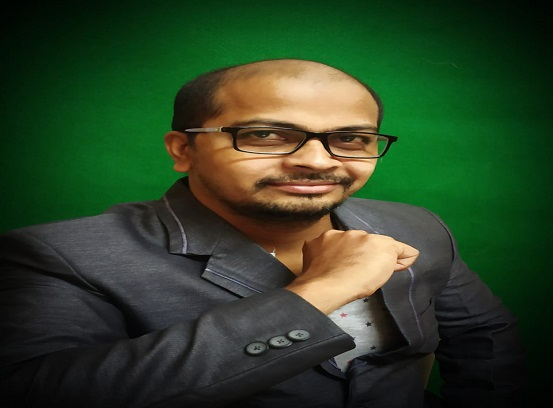
Most Popular Course topics
These are the most popular course topics among Software Courses for learners