Hi there, we’re Harisystems
"Unlock your potential and soar to new heights with our exclusive online courses! Ignite your passion, acquire valuable skills, and embrace limitless possibilities. Don't miss out on our limited-time sale - invest in yourself today and embark on a journey of personal and professional growth. Enroll now and shape your future with knowledge that lasts a lifetime!".
For corporate trainings, projects, and real world experience reach us. We believe that education should be accessible to all, regardless of geographical location or background.
1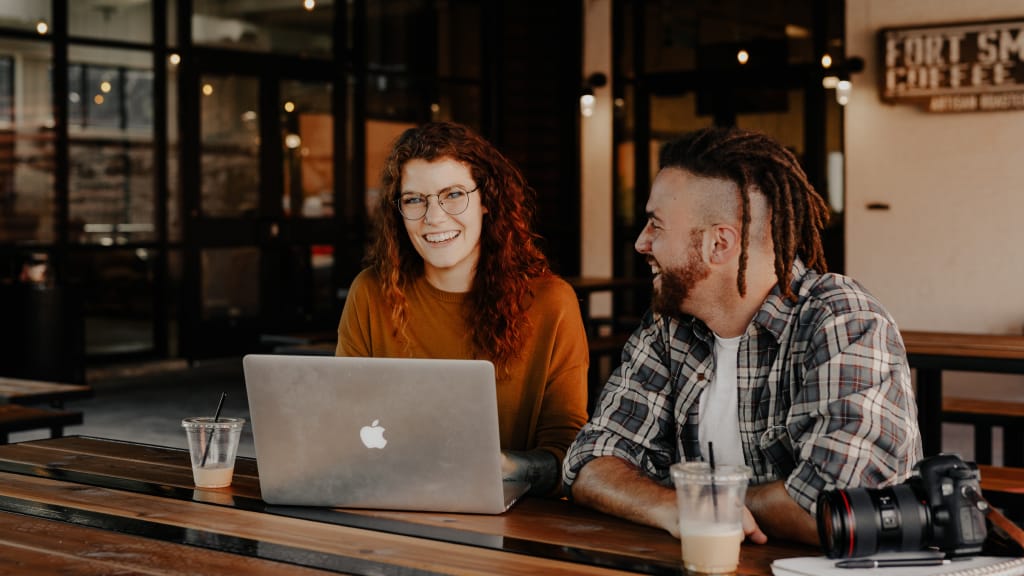
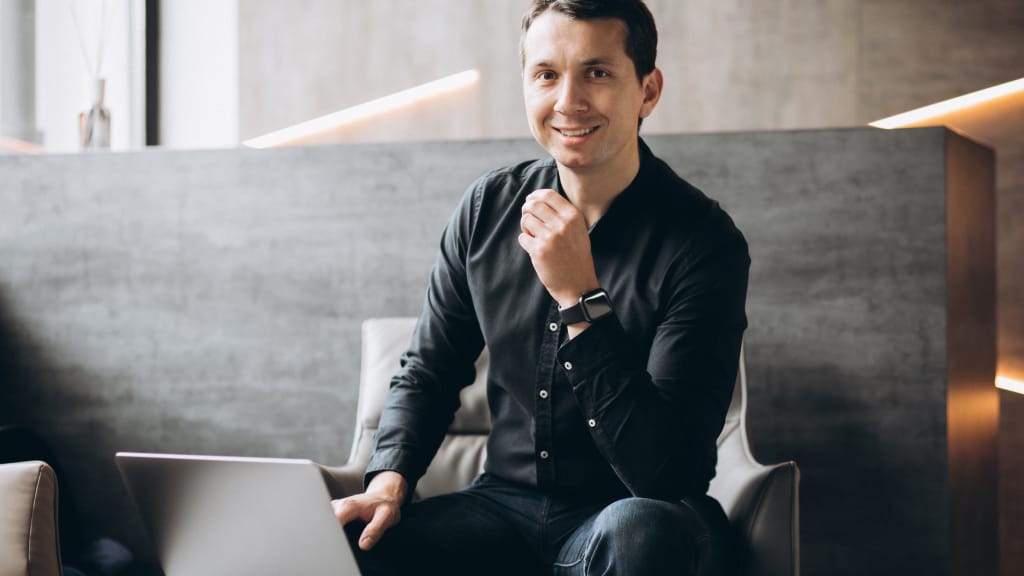
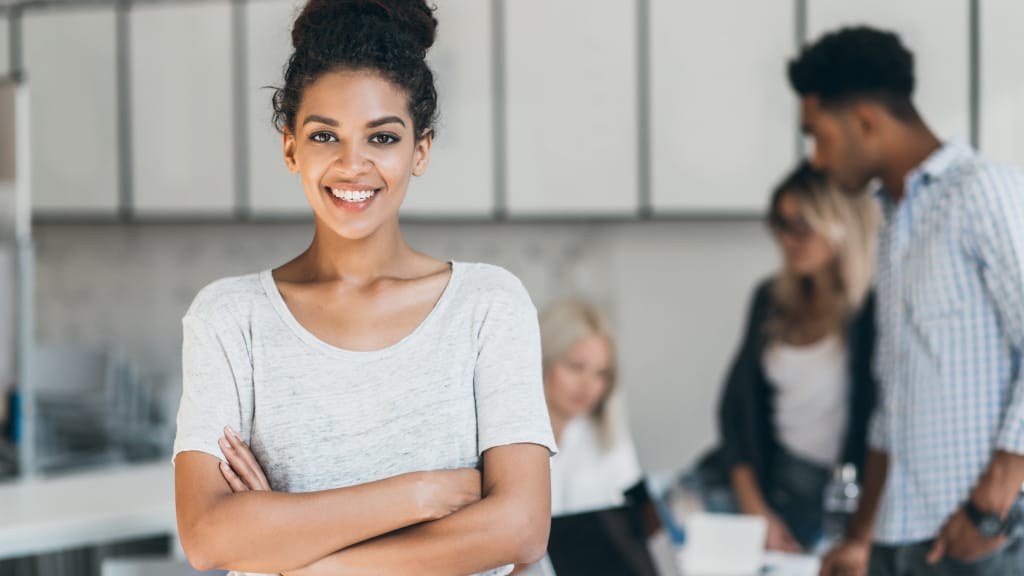
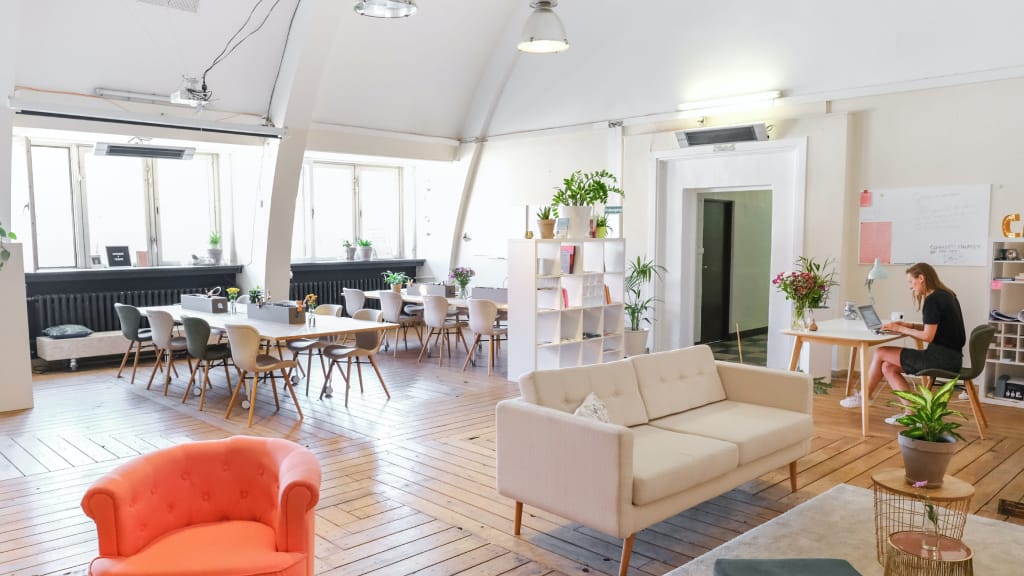
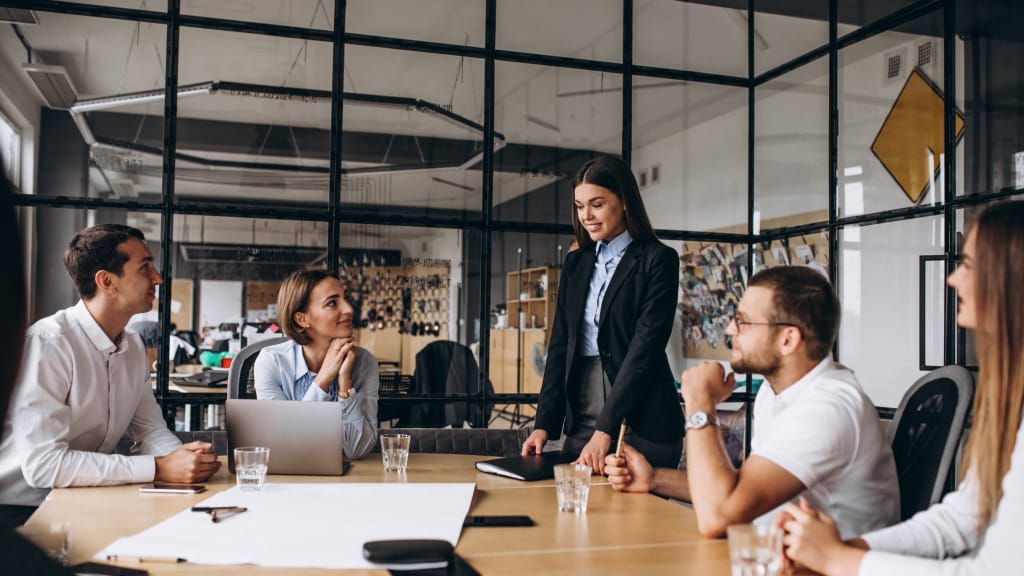
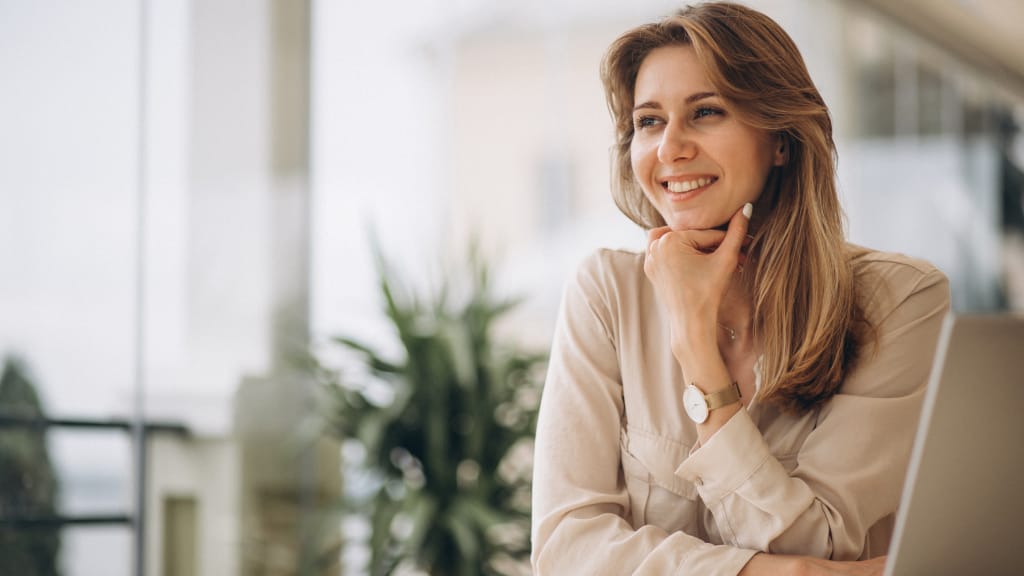
Python Encapsulation
Encapsulation is an important principle in object-oriented programming (OOP) that involves bundling data and methods together within a class and controlling their access from outside the class. It promotes data hiding, code organization, and provides a mechanism to protect the internal state of objects. In this article, we'll explore the concept of encapsulation in Python and provide examples to help you understand its implementation and benefits.
Example 1: Creating a Class with Encapsulation
Let's start with a basic example of creating a class with encapsulated properties:
class BankAccount:
def __init__(self):
self.__balance = 0
def deposit(self, amount):
self.__balance += amount
def withdraw(self, amount):
if amount <= self.__balance:
self.__balance -= amount
else:
print("Insufficient funds.")
def get_balance(self):
return self.__balance
account = BankAccount()
account.deposit(100)
account.withdraw(50)
print(account.get_balance()) # Output: 50
In this code, we define a class called "BankAccount" that encapsulates the "balance" property and related methods. The "__balance" variable is prefixed with two underscores, indicating that it is a private attribute and should not be accessed directly from outside the class. The "deposit" and "withdraw" methods modify the balance, while the "get_balance" method returns the current balance. By encapsulating the "balance" property, we control its access and ensure that it can only be modified through the designated methods.
Example 2: Accessing Encapsulated Properties
Although encapsulated properties are intended to be private, Python provides a mechanism to access them indirectly. Here's an example:
class Person:
def __init__(self, name, age):
self.__name = name
self.__age = age
def get_name(self):
return self.__name
def get_age(self):
return self.__age
person = Person("John", 30)
print(person.get_name()) # Output: John
print(person.get_age()) # Output: 30
In this code, we define a class called "Person" with encapsulated properties "__name" and "__age". We provide getter methods, "get_name" and "get_age", to access these properties indirectly. By calling the getter methods, we can retrieve the values of the encapsulated properties, maintaining encapsulation while still providing access to the information.
Example 3: Encapsulation with Property Decorators
Python provides property decorators that allow us to define encapsulated properties with convenient access syntax. Here's an example:
class Circle:
def __init__(self, radius):
self.__radius = radius
@property
def radius(self):
return self.__radius
@radius.setter
def radius(self, value):
if value >= 0:
self.__radius = value
else:
print("Invalid radius value.")
circle = Circle(5)
print(circle.radius) # Output: 5
circle.radius = 10
print(circle.radius)
# Output: 10
circle.radius = -1 # Output: Invalid radius value.
In this code, we define a class called "Circle" with an encapsulated property "__radius". We use the property decorator to define a getter method for the "radius" property, and the "radius.setter" decorator to define a setter method. This allows us to access the property directly using the dot notation, while still maintaining encapsulation and adding validation logic to the setter method.
Conclusion
Encapsulation is a fundamental concept in Python that promotes data hiding and code organization. By encapsulating properties and controlling their access, you can ensure the integrity of your objects and protect their internal state. Experiment with the examples provided and explore the possibilities of encapsulation in your Python projects.
4.5L
Learners
20+
Instructors
50+
Courses
6.0L
Course enrollments
Future Trending Courses
When selecting, a course, Here are a few areas that are expected to be in demand in the future:.
Future Learning for all
If you’re passionate and ready to dive in, we’d love to join 1:1 classes for you. We’re committed to support our learners and professionals their development and well-being.
View Courses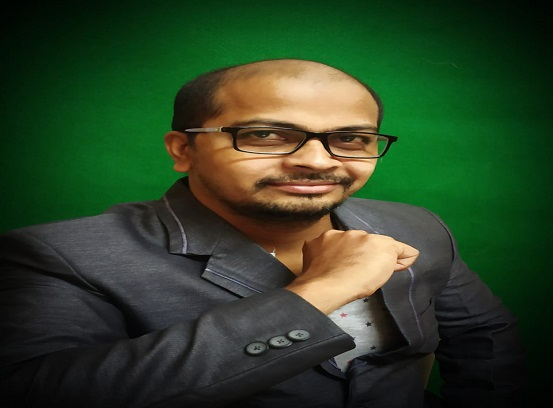
Most Popular Course topics
These are the most popular course topics among Software Courses for learners