Hi there, we’re Harisystems
"Unlock your potential and soar to new heights with our exclusive online courses! Ignite your passion, acquire valuable skills, and embrace limitless possibilities. Don't miss out on our limited-time sale - invest in yourself today and embark on a journey of personal and professional growth. Enroll now and shape your future with knowledge that lasts a lifetime!".
For corporate trainings, projects, and real world experience reach us. We believe that education should be accessible to all, regardless of geographical location or background.
1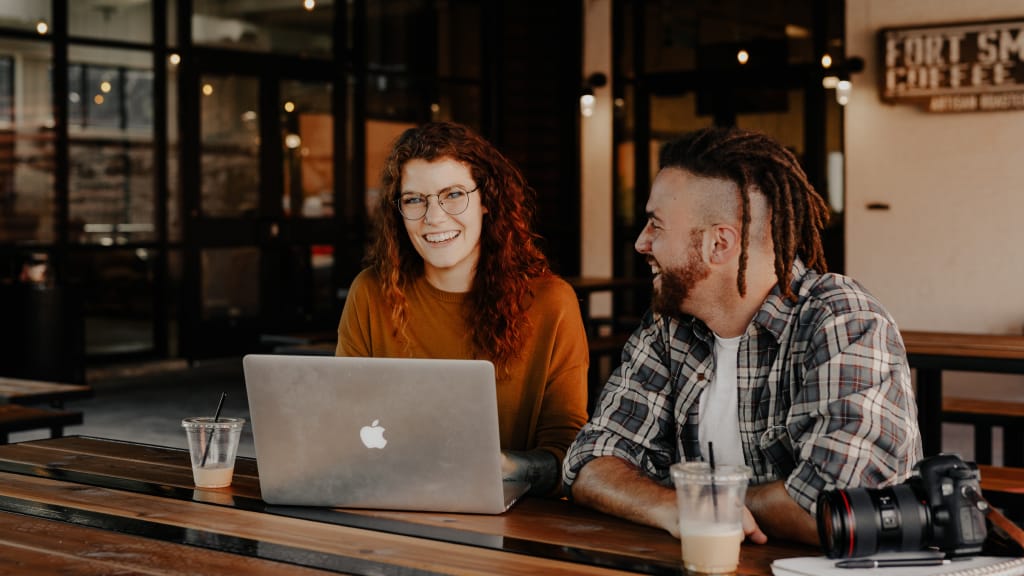
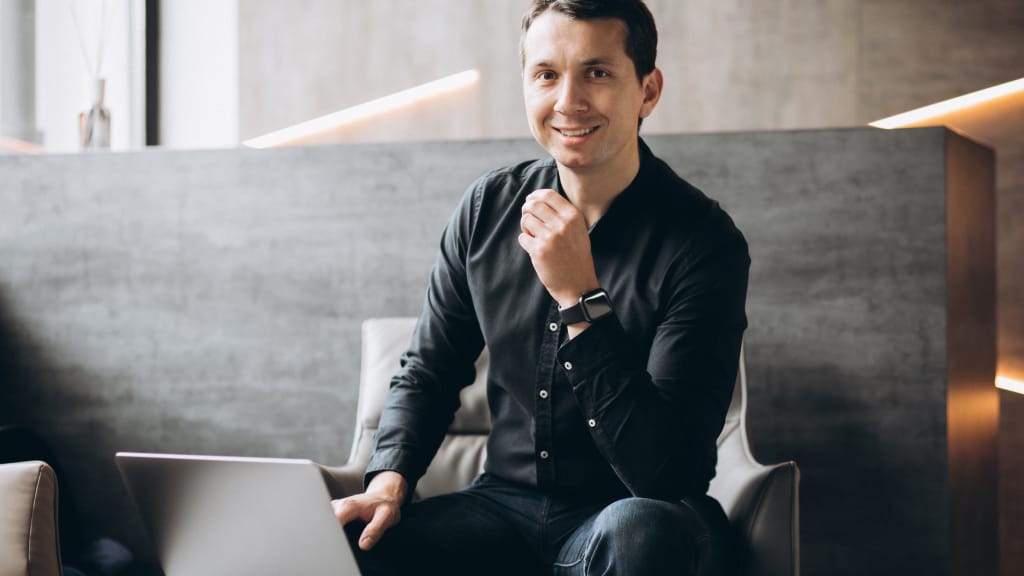
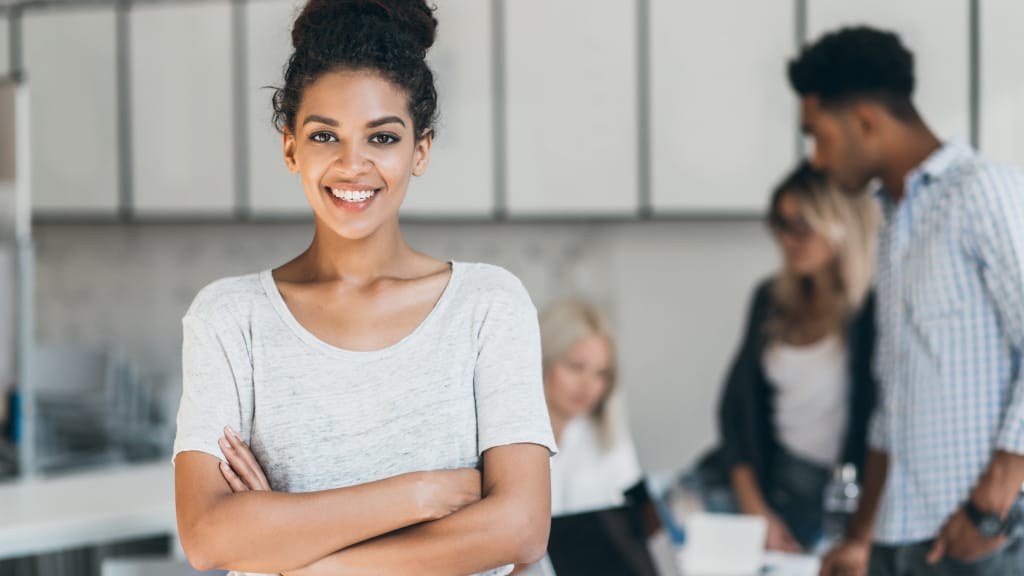
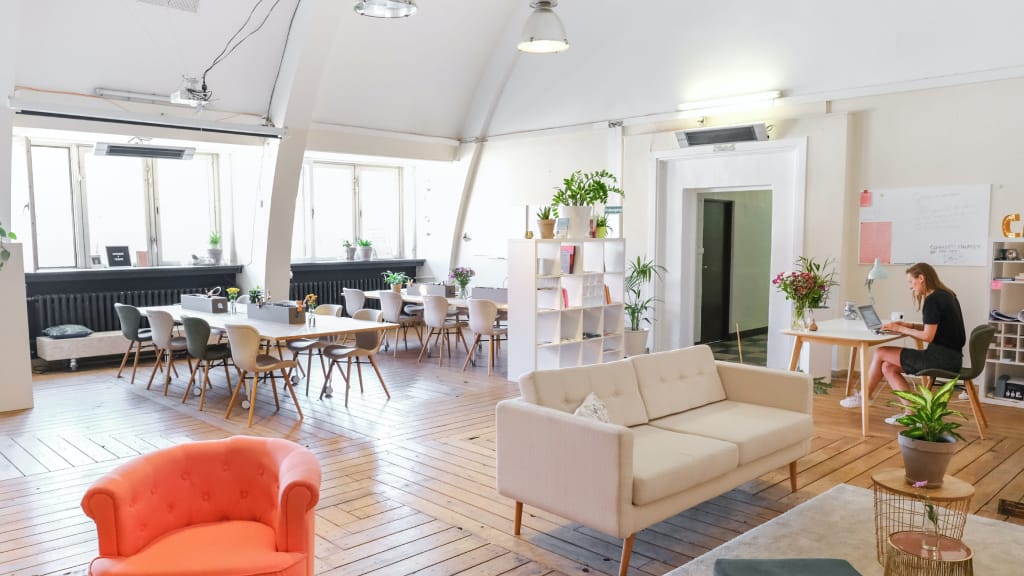
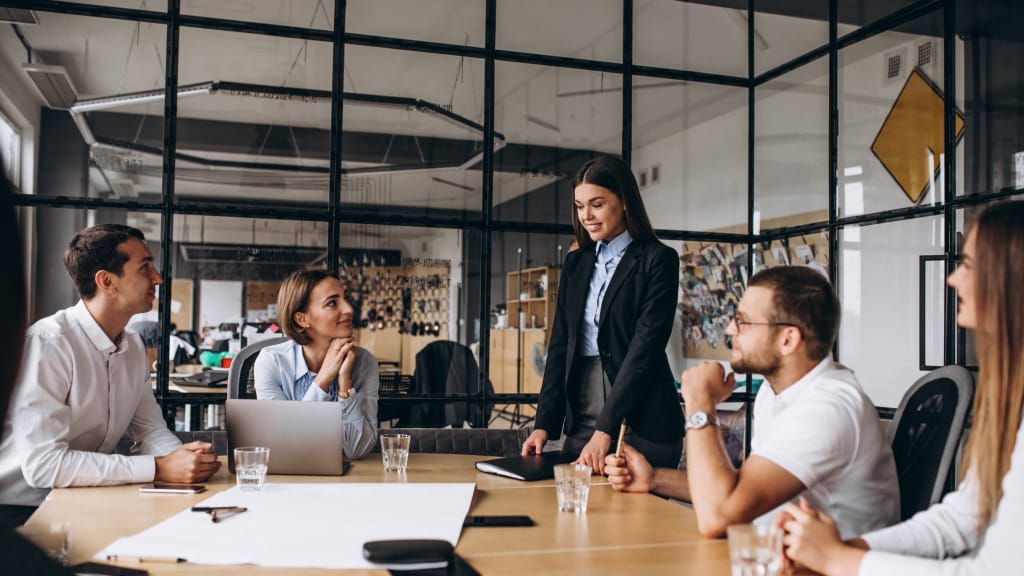
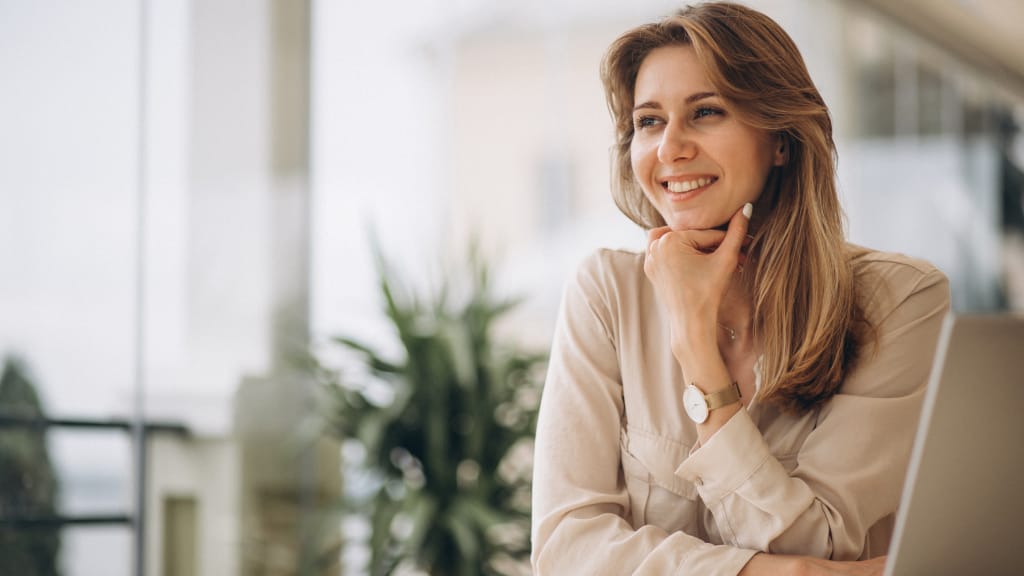
C++ Standard Libraries
C++ provides a rich collection of standard libraries that offer a wide range of functionalities to simplify and accelerate development. These libraries contain pre-defined classes and functions that can be utilized to perform common tasks, such as input/output operations, mathematical computations, string manipulations, and more. In this article, we will introduce you to some of the most commonly used C++ standard libraries with examples.
1. iostream
The iostream
library is essential for input/output operations. It provides classes, such as iostream
, istream
, and ostream
, to handle input from the user and output to the console. Here's an example:
#include <iostream>
int main() {
int num;
std::cout << "Enter a number: ";
std::cin >> num;
std::cout << "You entered: " << num << std::endl;
return 0;
}
In the above code, we use std::cin
to read input from the user and std::cout
to display output on the console.
2. cmath
The cmath
library provides mathematical functions and constants. It includes functions for basic arithmetic operations, trigonometry, logarithms, and more. Here's an example:
#include <iostream>
#include <cmath>
int main() {
double radius = 2.5;
double area = M_PI * pow(radius, 2);
std::cout << "Area of the circle: " << area << std::endl;
return 0;
}
In the above code, we calculate the area of a circle using the M_PI
constant from the cmath
library and the pow
function to raise the radius to the power of 2.
3. string
The string
library provides classes and functions to handle strings in C++. It offers convenient ways to create, manipulate, and concatenate strings. Here's an example:
#include <iostream>
#include <string>
int main() {
std::string name = "John";
std::string greeting = "Hello, " + name;
std::cout << greeting << std::endl;
return 0;
}
In the above code, we use the std::string
class to create strings and the concatenation operator (+
) to join strings together.
4. vector
The vector
library provides a dynamic array implementation in C++. It allows you to create resizable arrays and provides functions for adding, removing, and accessing elements. Here's an example:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers;
numbers.push_back(1);
numbers.push_back(2);
numbers.push_back(3);
for (int num : numbers) {
std::cout << num << " ";
}
std::cout << std::endl;
return 0;
}
In the above code, we use the std::vector
class to create a dynamic array of integers. We add elements to the vector using push_back()
and then iterate over the vector to print the elements.
These are just a few examples of the many standard libraries available in C++. By leveraging these libraries, you can save time and effort in implementing common functionalities and focus on solving the core problems in your programs.
Explore the C++ standard libraries documentation to discover more features and functionalities. Experiment with different libraries and their functions to enhance your programming skills and efficiency.
4.5L
Learners
20+
Instructors
50+
Courses
6.0L
Course enrollments
Future Trending Courses
When selecting, a course, Here are a few areas that are expected to be in demand in the future:.
Future Learning for all
If you’re passionate and ready to dive in, we’d love to join 1:1 classes for you. We’re committed to support our learners and professionals their development and well-being.
View Courses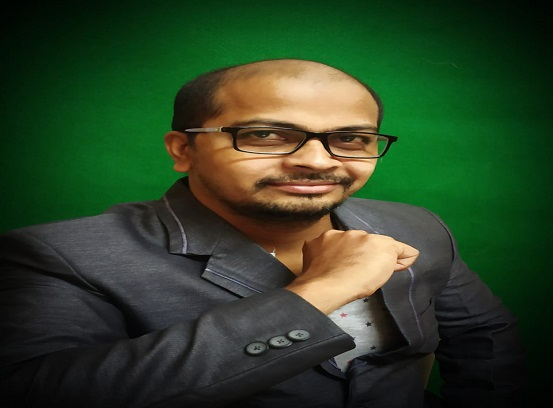
Most Popular Course topics
These are the most popular course topics among Software Courses for learners