Hi there, we’re Harisystems
"Unlock your potential and soar to new heights with our exclusive online courses! Ignite your passion, acquire valuable skills, and embrace limitless possibilities. Don't miss out on our limited-time sale - invest in yourself today and embark on a journey of personal and professional growth. Enroll now and shape your future with knowledge that lasts a lifetime!".
For corporate trainings, projects, and real world experience reach us. We believe that education should be accessible to all, regardless of geographical location or background.
1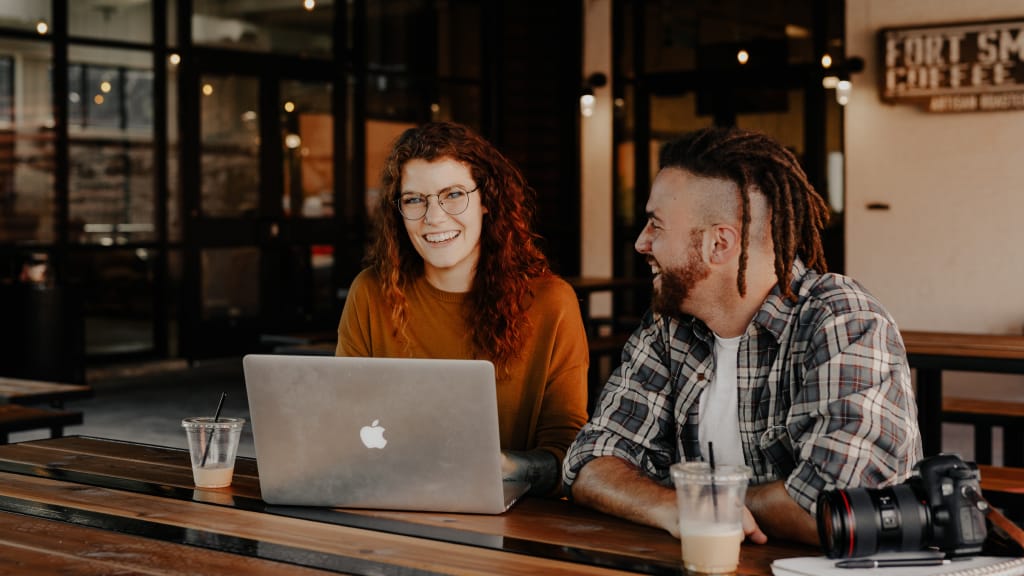
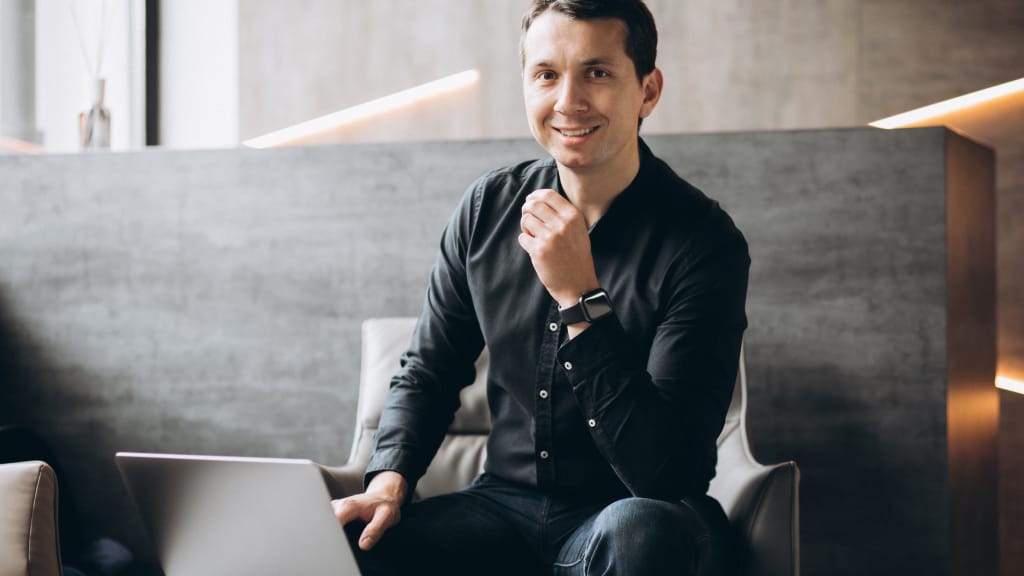
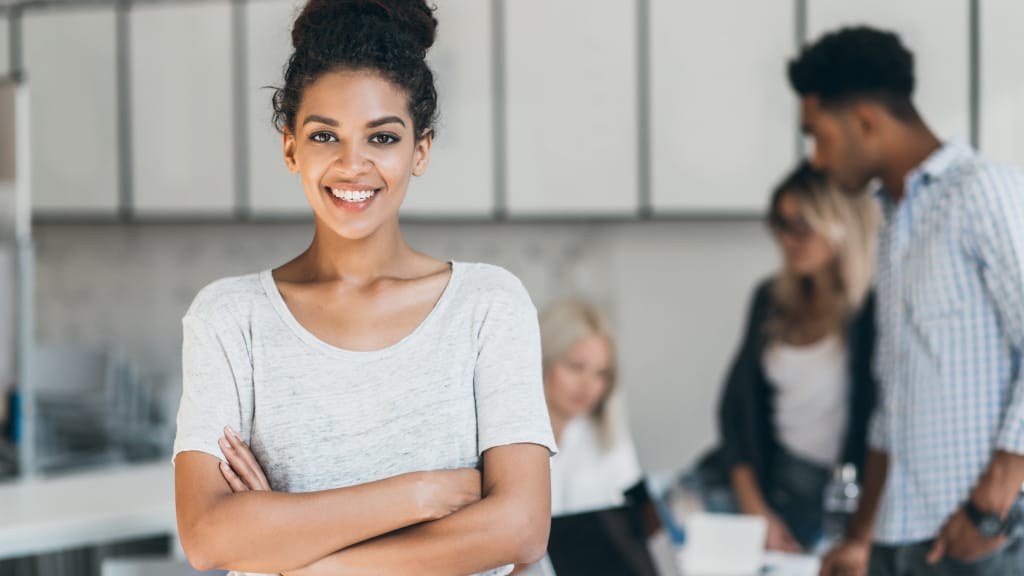
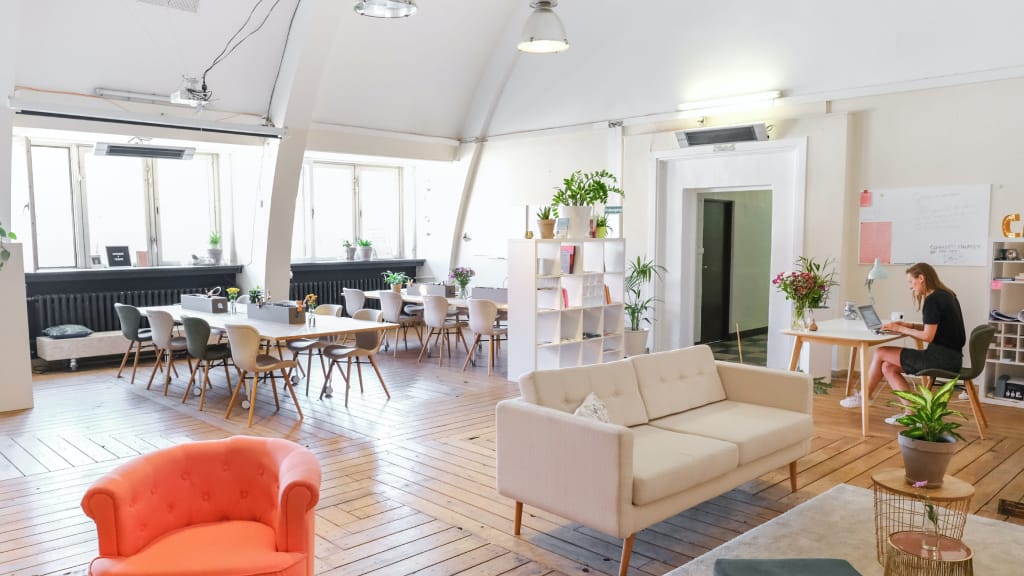
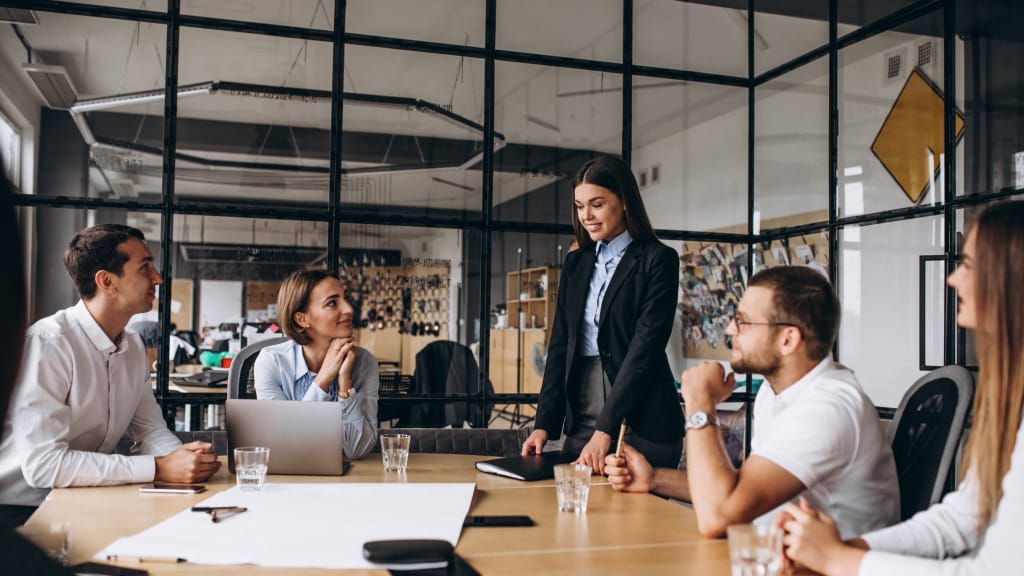
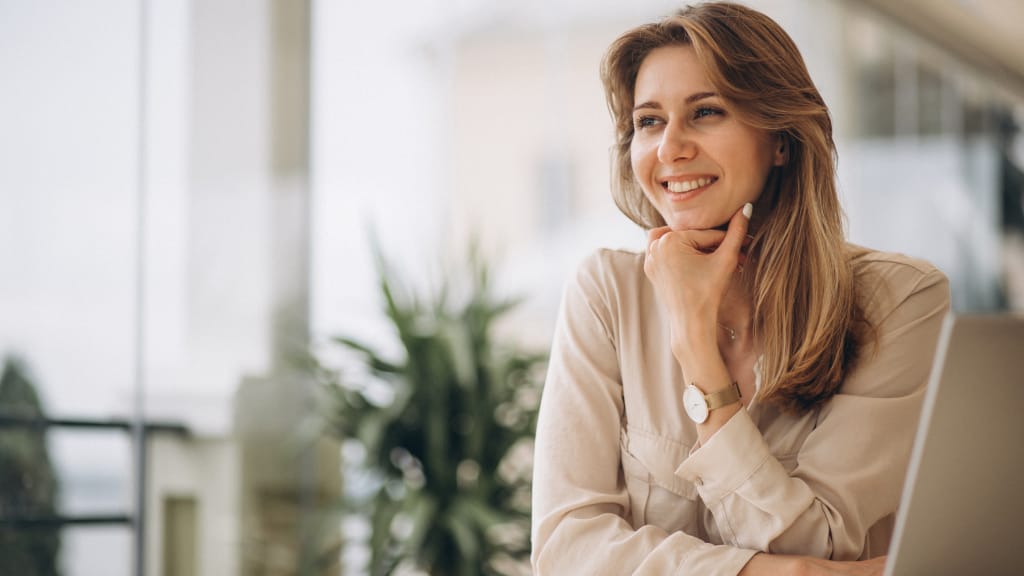
JavaScript Control Flow Statements: Explained with Examples
Control flow statements in JavaScript allow you to control the flow of your code by making decisions and executing code blocks based on certain conditions. They provide you with the ability to repeat code blocks or skip certain sections of code. In this article, we will explore different control flow statements in JavaScript and provide examples of their usage.
if Statement
The if
statement is the most basic control flow statement. It allows you to execute a block of code only if a specified condition is true. Here's an example:
let x = 5;
if (x > 0) {
console.log("x is positive");
}
In this example, the code inside the if
block will only execute if the condition x > 0
is true. If the condition is false, the block will be skipped.
for Loop
The for
loop allows you to repeat a block of code a specific number of times. It consists of three parts: initialization, condition, and iteration. Here's an example:
for (let i = 0; i < 5; i++) {
console.log(i);
}
In this example, the code inside the loop will be executed five times. The loop starts with i = 0
, checks the condition i < 5
, and increments i
by 1 after each iteration.
while Loop
The while
loop allows you to repeat a block of code as long as a specified condition is true. Here's an example:
let i = 0;
while (i < 5) {
console.log(i);
i++;
}
In this example, the code inside the loop will be executed as long as the condition i < 5
is true. The value of i
starts at 0 and increments by 1 after each iteration.
do-while Loop
The do-while
loop is similar to the while
loop, but it executes the block of code at least once before checking the condition. Here's an example:
let i = 0;
do {
console.log(i);
i++;
} while (i < 5);
In this example, the code inside the loop will be executed at least once, and then it will continue to execute as long as the condition i < 5
is true. The value of i
starts at 0 and increments by 1 after each iteration.
break Statement
The break
statement allows you to
terminate a loop or switch statement. It is commonly used to exit a loop when a certain condition is met. Here's an example:
for (let i = 0; i < 5; i++) {
if (i === 3) {
break;
}
console.log(i);
}
In this example, the loop will terminate when i
is equal to 3 because of the break
statement. As a result, only the values 0, 1, and 2 will be printed.
continue Statement
The continue
statement allows you to skip the rest of the current iteration and move on to the next iteration of a loop. It is commonly used to skip certain iterations based on specific conditions. Here's an example:
for (let i = 0; i < 5; i++) {
if (i === 2) {
continue;
}
console.log(i);
}
In this example, when i
is equal to 2, the continue
statement is encountered, and the rest of the code in that iteration is skipped. As a result, the value 2 will be skipped, and the remaining values 0, 1, 3, and 4 will be printed.
Conclusion
JavaScript control flow statements provide you with the ability to control the flow and execution of your code based on specific conditions. In this article, we explored the if
statement, for
loop, while
loop, do-while
loop, break
statement, and continue
statement. Understanding and utilizing these control flow statements effectively will enable you to write more dynamic and flexible JavaScript code to handle various scenarios and make decisions based on specific conditions.
4.5L
Learners
20+
Instructors
50+
Courses
6.0L
Course enrollments
Future Trending Courses
When selecting, a course, Here are a few areas that are expected to be in demand in the future:.
Future Learning for all
If you’re passionate and ready to dive in, we’d love to join 1:1 classes for you. We’re committed to support our learners and professionals their development and well-being.
View Courses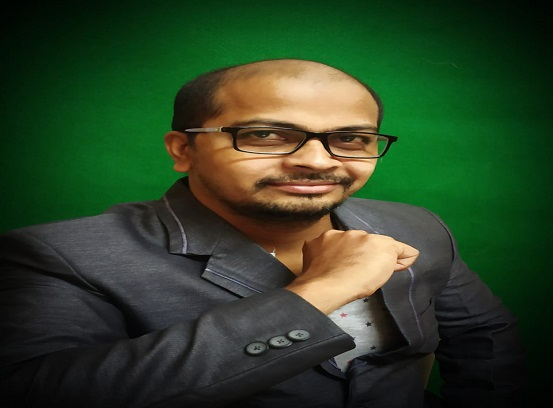
Most Popular Course topics
These are the most popular course topics among Software Courses for learners