Hi there, we’re Harisystems
"Unlock your potential and soar to new heights with our exclusive online courses! Ignite your passion, acquire valuable skills, and embrace limitless possibilities. Don't miss out on our limited-time sale - invest in yourself today and embark on a journey of personal and professional growth. Enroll now and shape your future with knowledge that lasts a lifetime!".
For corporate trainings, projects, and real world experience reach us. We believe that education should be accessible to all, regardless of geographical location or background.
1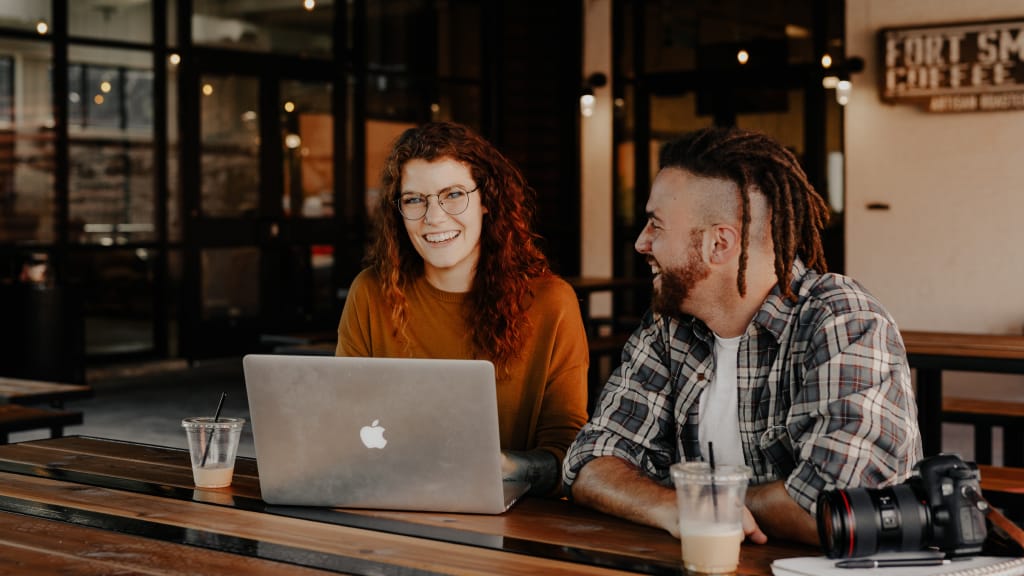
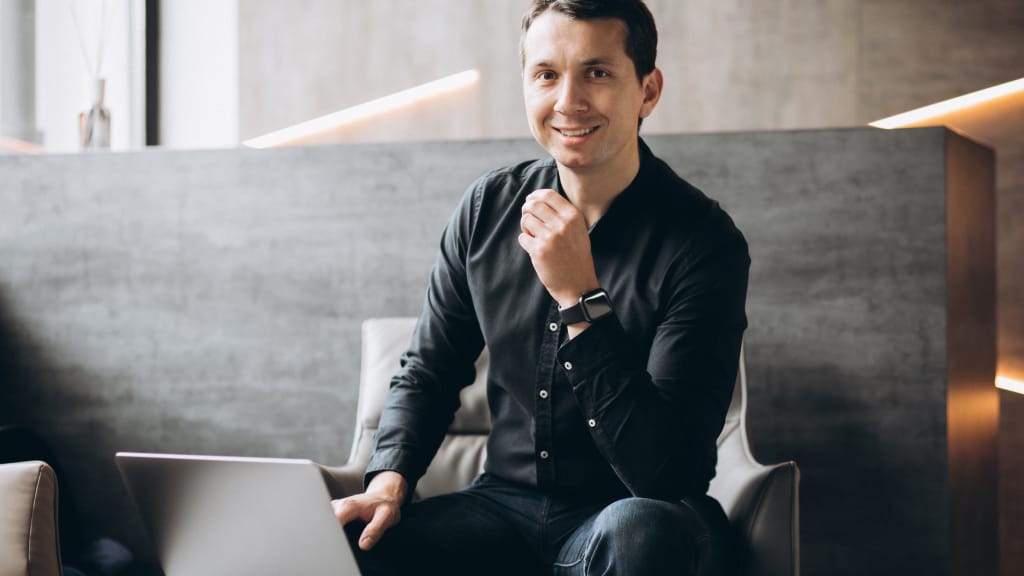
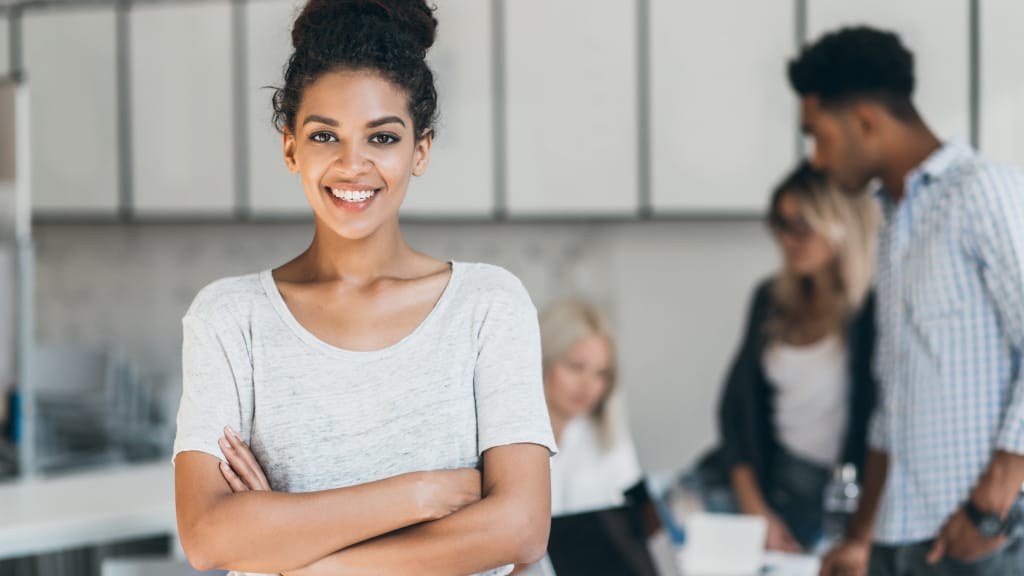
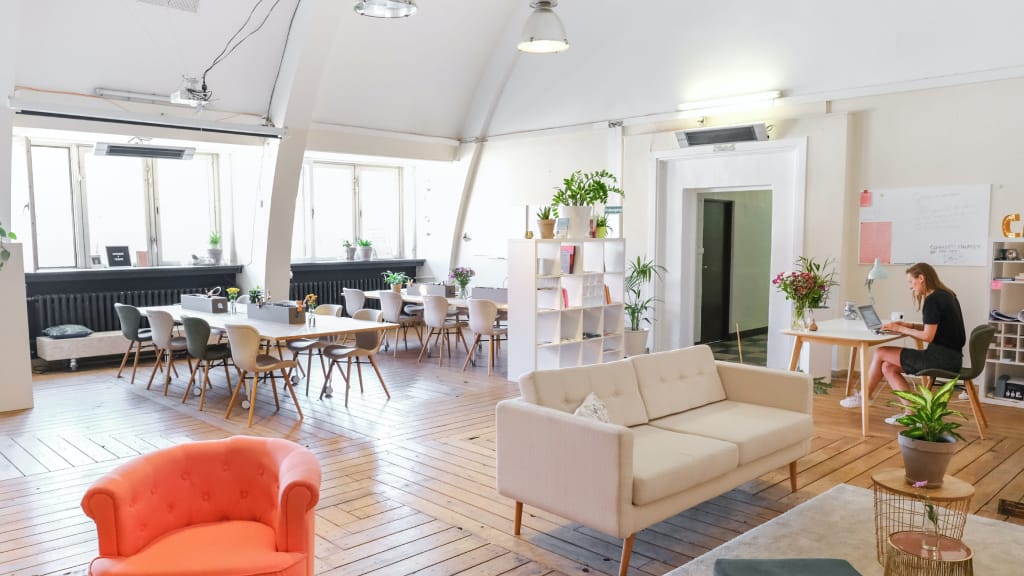
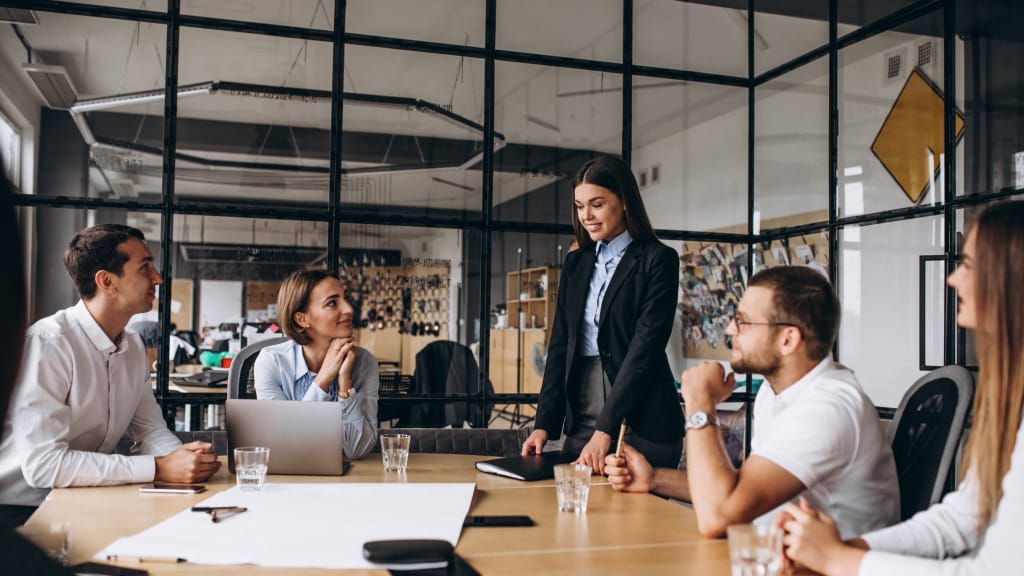
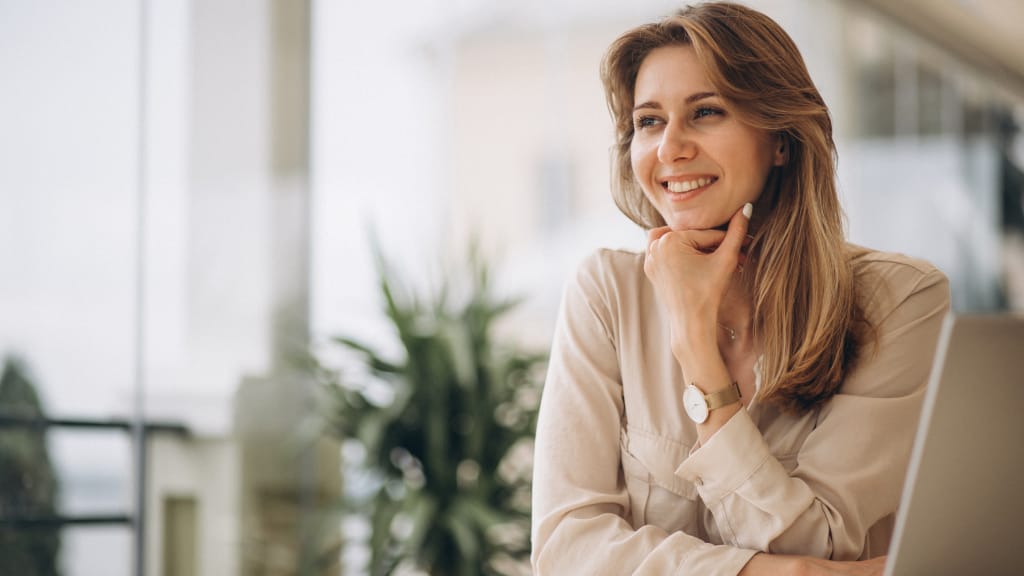
C++ Functions
In C++, a function is a reusable block of code that performs a specific task. Functions provide modularity, code reusability, and abstraction, making your code easier to understand and maintain. In this article, we will explore the usage of functions in C++ with examples.
1. Declaring and Defining Functions
To declare a function in C++, you need to specify the return type, the function name, and the parameters (if any). The function declaration tells the compiler about the existence of the function. Here's an example:
#include <iostream>
// Function declaration
int add(int a, int b);
int main() {
int result = add(10, 20);
std::cout << "Result: " << result << std::endl;
return 0;
}
// Function definition
int add(int a, int b) {
return a + b;
}
In the above code, we declare a function add
that takes two integer parameters and returns an integer. We then define the function after the main
function. The add
function adds the two parameters and returns the result.
2. Function Parameters
Functions can have parameters that allow you to pass values into the function for processing. Here's an example:
#include <iostream>
void greet(std::string name) {
std::cout << "Hello, " << name << "!" << std::endl;
}
int main() {
greet("John");
greet("Alice");
return 0;
}
In the above code, the function greet
takes a single parameter name
of type std::string
. It displays a greeting message with the provided name. The function is called multiple times with different arguments from the main
function.
3. Function Return Types
Functions can have different return types based on the data they produce. Here's an example:
#include <iostream>
bool isEven(int num) {
return num % 2 == 0;
}
int main() {
int number = 10;
if (isEven(number)) {
std::cout << number << " is even." << std::endl;
} else {
std::cout << number << " is odd." << std::endl;
}
return 0;
}
In the above code, the function isEven
takes an integer parameter num
and returns a boolean value indicating whether the number is even or not. The function is used within an if-else statement to display the appropriate message based on the return value.
4. Function Overloading
In C++, you can define multiple functions with the same name but different parameter lists. This is known as function overloading. Here's an example:
#include <iostream>
int add(int a, int b) {
return a + b;
}
double add(double a, double b) {
return a + b;
}
int main() {
int result1 = add(10, 20);
double result2 = add(2.5, 3.7);
std::cout << "Result 1: " << result1 << std::endl;
std::cout << "Result 2: " << result2 << std::endl;
return 0;
}
In the above code, we define two functions named add
with different parameter types. One function adds two integers, and the other function adds two doubles. The appropriate function is called based on the argument types provided.
Functions play a vital role in organizing and structuring your code in C++. They help encapsulate logic, promote code reuse, and enhance readability. Practice defining and calling functions with different return types and parameter lists to create modular and efficient code in your C++ programs.
4.5L
Learners
20+
Instructors
50+
Courses
6.0L
Course enrollments
Future Trending Courses
When selecting, a course, Here are a few areas that are expected to be in demand in the future:.
Future Learning for all
If you’re passionate and ready to dive in, we’d love to join 1:1 classes for you. We’re committed to support our learners and professionals their development and well-being.
View Courses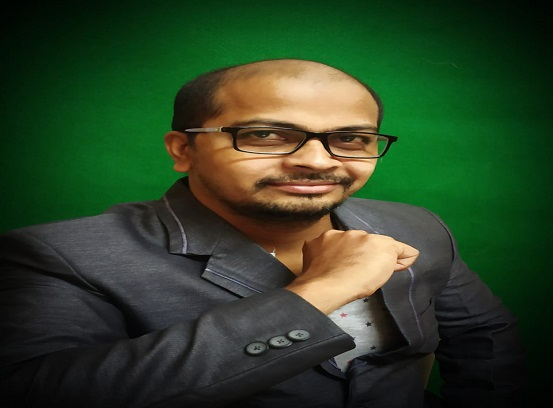
Most Popular Course topics
These are the most popular course topics among Software Courses for learners