Hi there, we’re Harisystems
"Unlock your potential and soar to new heights with our exclusive online courses! Ignite your passion, acquire valuable skills, and embrace limitless possibilities. Don't miss out on our limited-time sale - invest in yourself today and embark on a journey of personal and professional growth. Enroll now and shape your future with knowledge that lasts a lifetime!".
For corporate trainings, projects, and real world experience reach us. We believe that education should be accessible to all, regardless of geographical location or background.
1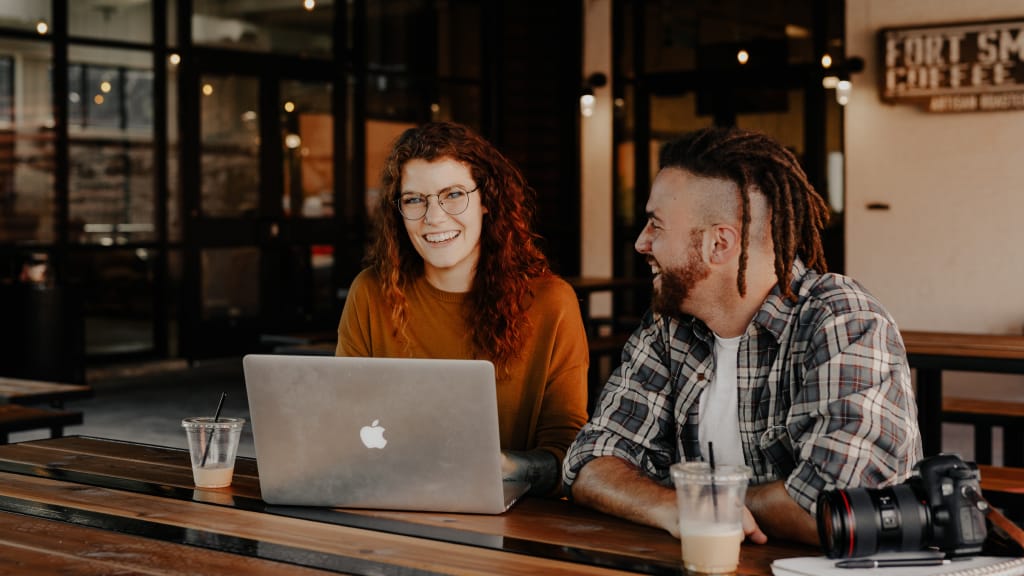
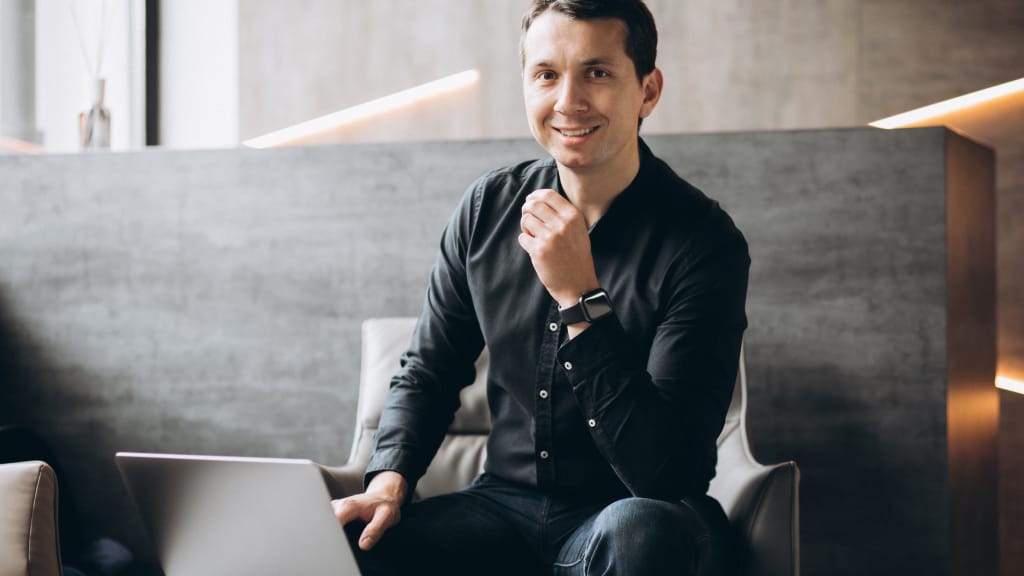
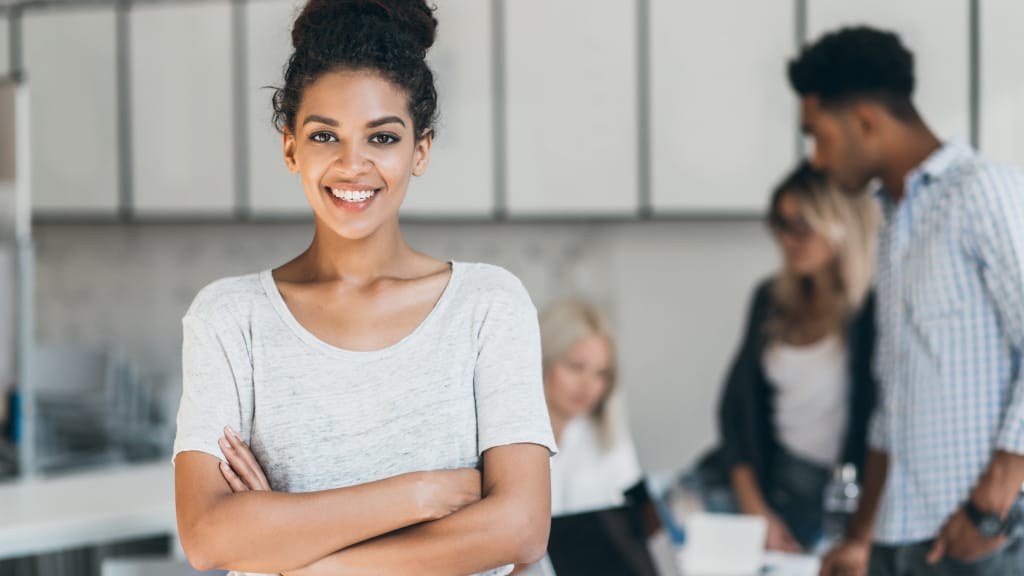
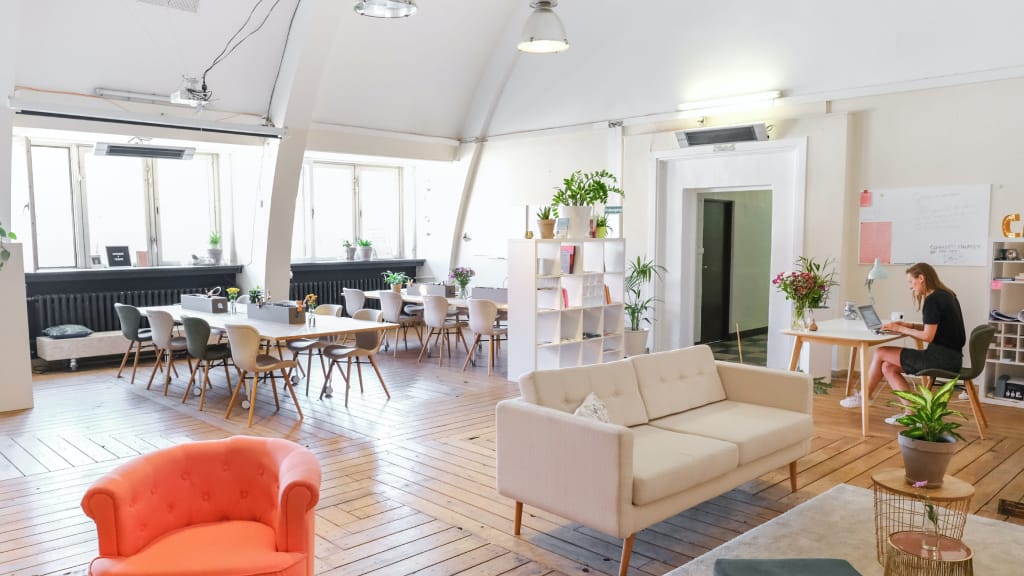
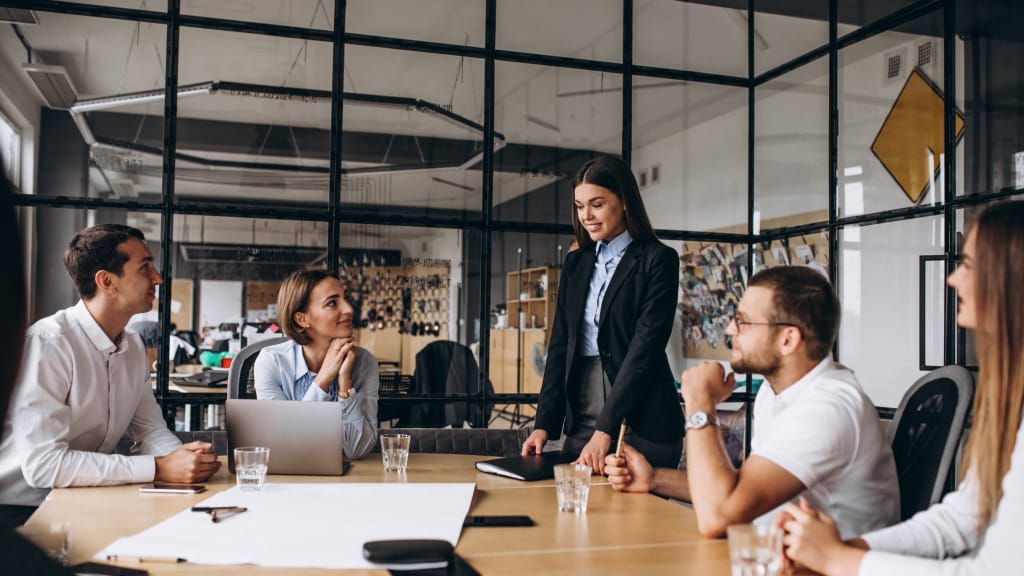
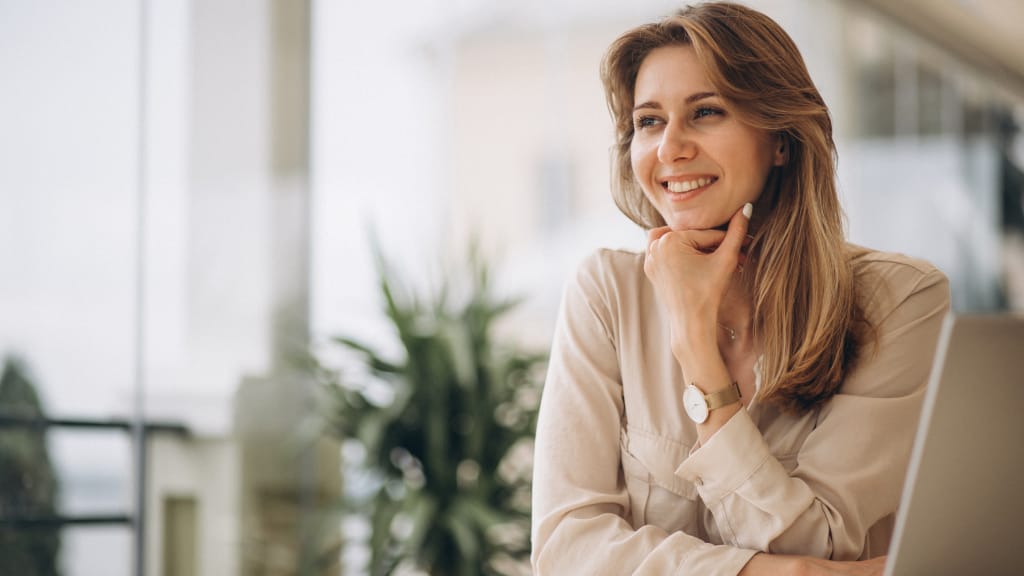
Python Django Project: Building Web Applications with Django
Introduction
Django is a high-level Python web framework that enables developers to build robust and scalable web applications. With its powerful features and elegant design, Django simplifies the development process and promotes best practices. In this guide, we will explore how to create a Python Django project from scratch with examples.
Step 1: Setting Up a Django Project
To create a Django project, make sure you have Python installed on your system. Open a terminal or command prompt and run the following command to install Django:
pip install django
Once Django is installed, create a new Django project by running the following command:
django-admin startproject myproject
This command will create a new directory called "myproject" with the necessary files and directories for your Django project.
Step 2: Creating a Django App
In Django, apps are modular components that encapsulate specific functionality. To create a new app within your project, navigate to the project directory and run the following command:
python manage.py startapp myapp
This command will generate the necessary files and directories for your app within the project structure.
Step 3: Defining Models
In Django, models represent the structure and behavior of data in your application. Open the models.py
file within your app's directory and define your models using Django's model syntax. Here's an example:
from django.db import models
class MyModel(models.Model):
name = models.CharField(max_length=100)
description = models.TextField()
def __str__(self):
return self.name
In this example, we define a simple model called MyModel
with two fields: name
(a character field) and description
(a text field). We also override the __str__()
method to provide a human-readable representation of the model.
Step 4: Creating Views
Views in Django handle the logic and processing of requests. Open the views.py
file within your app's directory and define your views using Python functions or classes. Here's an example:
from django.shortcuts import render
from .models import MyModel
def my_view(request):
objects = MyModel.objects.all()
return render(request, 'myapp/my_template.html', {'objects': objects})
In this example, we define a view called my_view
that retrieves all objects from the MyModel
model and passes them to a template called my_template.html
for rendering.
Step 5: Creating Templates
Templates in Django define the structure and layout of your web pages. Create a templates
directory within your app's directory and add HTML templates. Here's an example template (my_template.html
):
<html>
<head>
<title>My App</title>
</head>
<body>
<h1>My App</h1>
<ul>
{% for object in objects %}
<li>{{ object.name }}: {{ object.description }}</li>
{% endfor %}
</ul>
</body>
</html>
In this example, we create a basic HTML structure with a heading and a list. We use a for loop to iterate over the objects passed from the view and display their names and descriptions within list items.
Step 6: Configuring URLs
URLs in Django define the routing and mapping of URLs to views. Open the urls.py
file within your project's directory and define the URL patterns for your app. Here's an example:
from django.urls import path
from myapp import views
urlpatterns = [
path('', views.my_view, name='my-view'),
]
In this example, we create a single URL pattern that maps the root URL to the my_view
view within the myapp
app.
Step 7: Running the Development Server
To test your Django project locally, start the development server by running the following command:
python manage.py runserver
This command will start the development server, and you can access your Django project by visiting http://localhost:8000/
in your web browser.
Conclusion
Building a Python Django project allows you to create powerful and scalable web applications. By following this guide, you have learned how to set up a Django project, create apps, define models, create views, design templates, configure URLs, and run the development server. With Django's robust framework and rich ecosystem, you can develop complex web applications with ease.
4.5L
Learners
20+
Instructors
50+
Courses
6.0L
Course enrollments
Future Trending Courses
When selecting, a course, Here are a few areas that are expected to be in demand in the future:.
Future Learning for all
If you’re passionate and ready to dive in, we’d love to join 1:1 classes for you. We’re committed to support our learners and professionals their development and well-being.
View Courses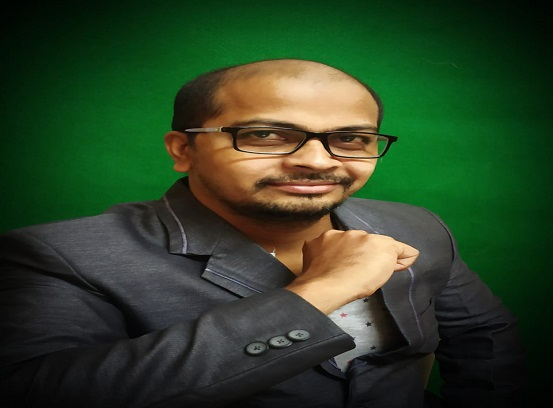
Most Popular Course topics
These are the most popular course topics among Software Courses for learners