Hi there, we’re Harisystems
"Unlock your potential and soar to new heights with our exclusive online courses! Ignite your passion, acquire valuable skills, and embrace limitless possibilities. Don't miss out on our limited-time sale - invest in yourself today and embark on a journey of personal and professional growth. Enroll now and shape your future with knowledge that lasts a lifetime!".
For corporate trainings, projects, and real world experience reach us. We believe that education should be accessible to all, regardless of geographical location or background.
1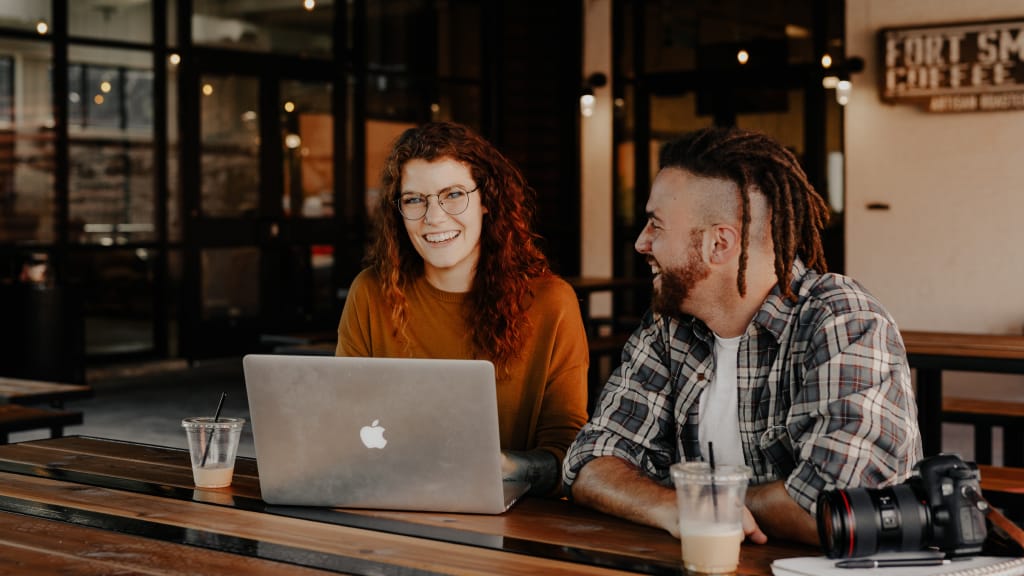
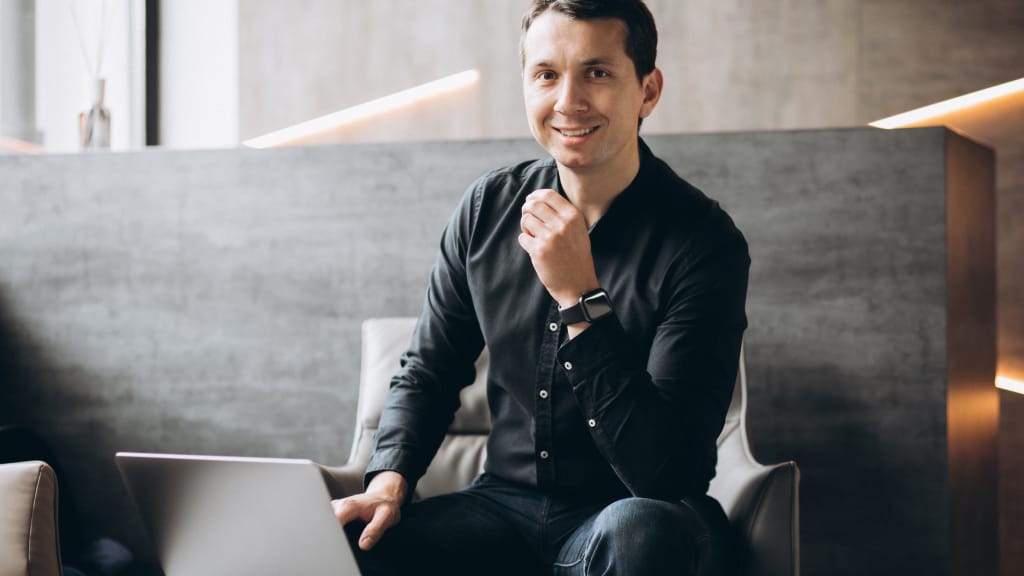
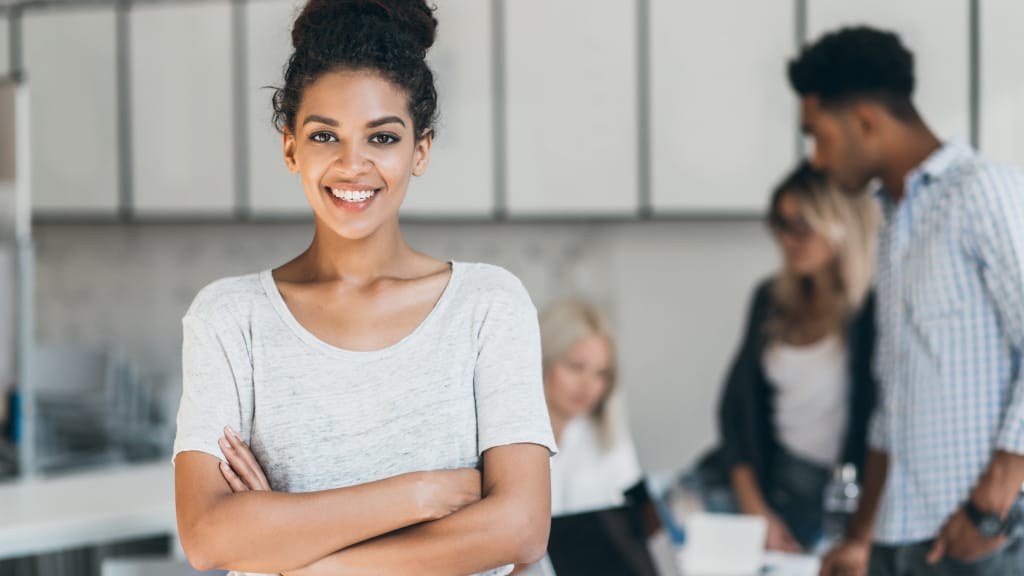
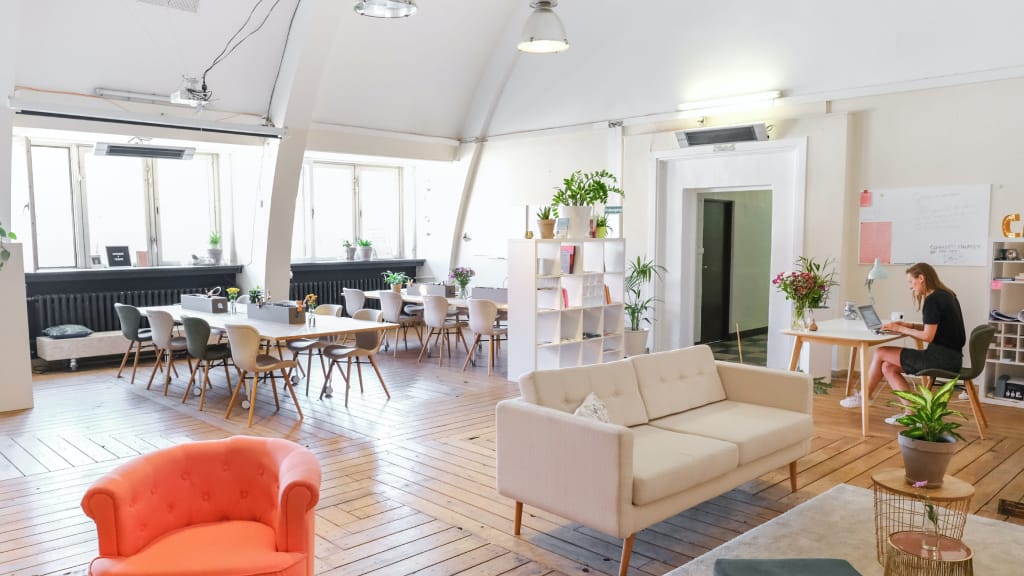
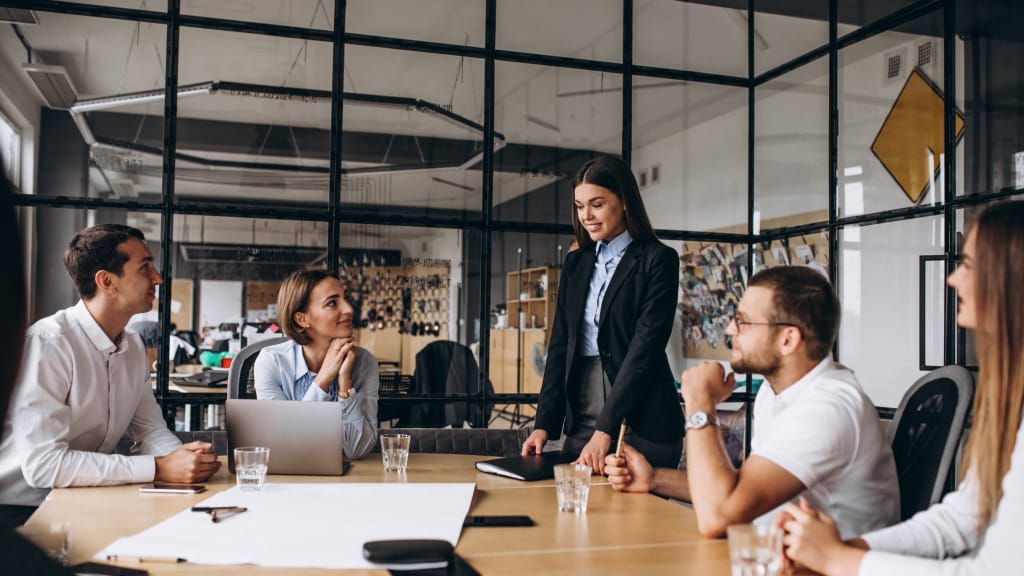
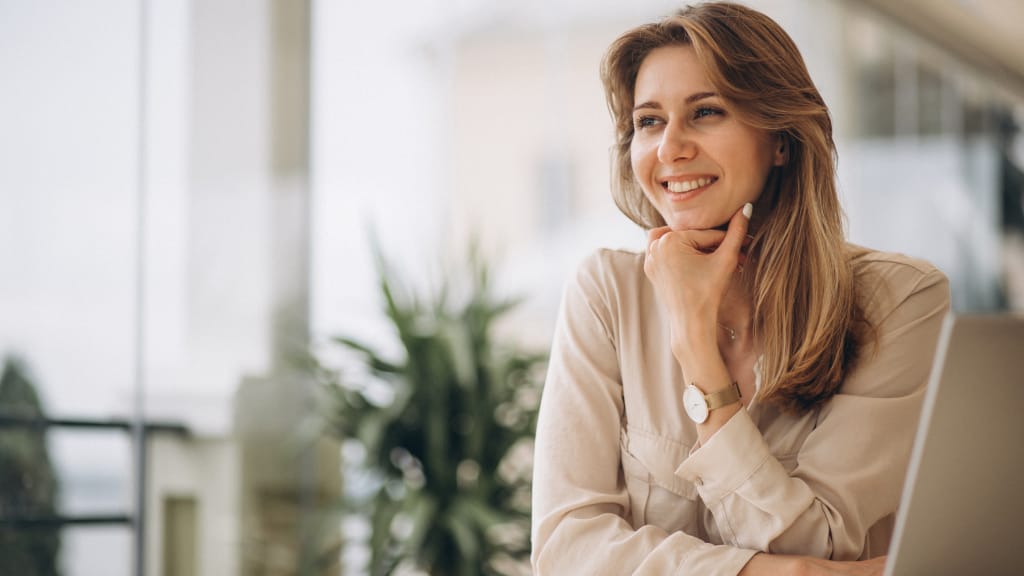
JavaScript Validations: Explained with Examples
Validations play a crucial role in ensuring the integrity and accuracy of data in web applications. JavaScript provides powerful tools and techniques to perform client-side validations, enhancing the user experience by providing immediate feedback. In this article, we will explore JavaScript validations and provide examples of their implementation.
Required Field Validation
One common validation is to ensure that a field is not left empty. Here's an example of how to perform a required field validation using JavaScript:
<form>
<input type="text" id="name" required>
<button type="submit" onclick="validateForm()">Submit</button>
</form>
<script>
function validateForm() {
var name = document.getElementById("name").value;
if (name === "") {
alert("Please enter your name.");
return false;
}
}
</script>
In this example, the required
attribute is added to the input field to ensure that it cannot be submitted empty. The validateForm
function is called when the form is submitted. It checks if the value of the name
field is empty, and if so, displays an alert message and returns false
to prevent form submission.
Email Validation
Another common validation is to ensure that an email address is entered in a valid format. Here's an example of how to perform an email validation using JavaScript:
<form>
<input type="email" id="email">
<button type="submit" onclick="validateForm()">Submit</button>
</form>
<script>
function validateForm() {
var email = document.getElementById("email").value;
var emailPattern = /^[^\s@]+@[^\s@]+\.[^\s@]+$/;
if (!emailPattern.test(email)) {
alert("Please enter a valid email address.");
return false;
}
}
</script>
In this example, the email
input field has its type set to email
to enforce email format validation. The validateForm
function is called when the form is submitted. It uses a regular expression (emailPattern
) to check if the entered email matches the required format. If the email is invalid, an alert message is displayed, and the form submission is prevented.
Custom Validations
JavaScript also allows you to perform custom validations based on specific requirements. Here's an example of how to perform a custom validation to check if a password meets certain criteria:
<form>
<input type="password" id="password">
<button type="submit" onclick="validateForm()">Submit</button>
</form>
<script>
function validateForm() {
var password = document.getElementById("password").value;
var minLength = 8;
if (password.length < minLength) {
alert("Password must be at least " + minLength + " characters long.");
return false;
}
}
</script>
In this example, the validateForm
function checks if the length of the entered password is less than the specified minLength
. If the password is too short, an alert message is displayed, and the form submission is prevented.
Conclusion
JavaScript validations are essential for ensuring data integrity and providing a smooth user experience in web applications. In this article, we explored examples of required field validation, email validation, and custom validations. By leveraging JavaScript's validation capabilities, you can create robust and user-friendly forms that enforce data accuracy and improve the overall quality of your web applications.
4.5L
Learners
20+
Instructors
50+
Courses
6.0L
Course enrollments
Future Trending Courses
When selecting, a course, Here are a few areas that are expected to be in demand in the future:.
Future Learning for all
If you’re passionate and ready to dive in, we’d love to join 1:1 classes for you. We’re committed to support our learners and professionals their development and well-being.
View Courses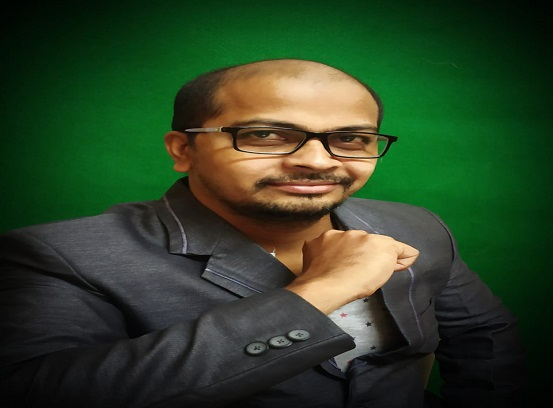
Most Popular Course topics
These are the most popular course topics among Software Courses for learners