Hi there, we’re Harisystems
"Unlock your potential and soar to new heights with our exclusive online courses! Ignite your passion, acquire valuable skills, and embrace limitless possibilities. Don't miss out on our limited-time sale - invest in yourself today and embark on a journey of personal and professional growth. Enroll now and shape your future with knowledge that lasts a lifetime!".
For corporate trainings, projects, and real world experience reach us. We believe that education should be accessible to all, regardless of geographical location or background.
1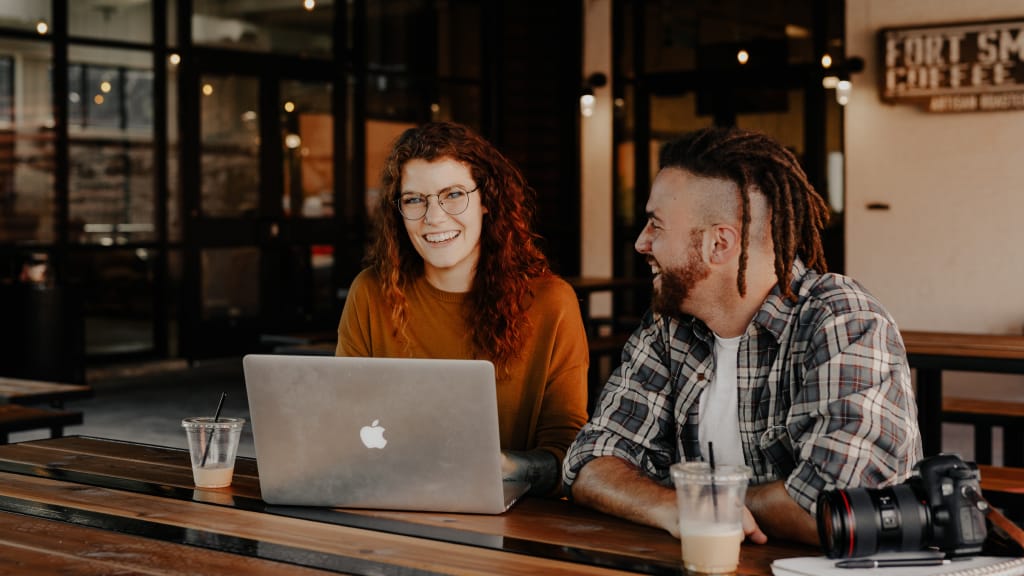
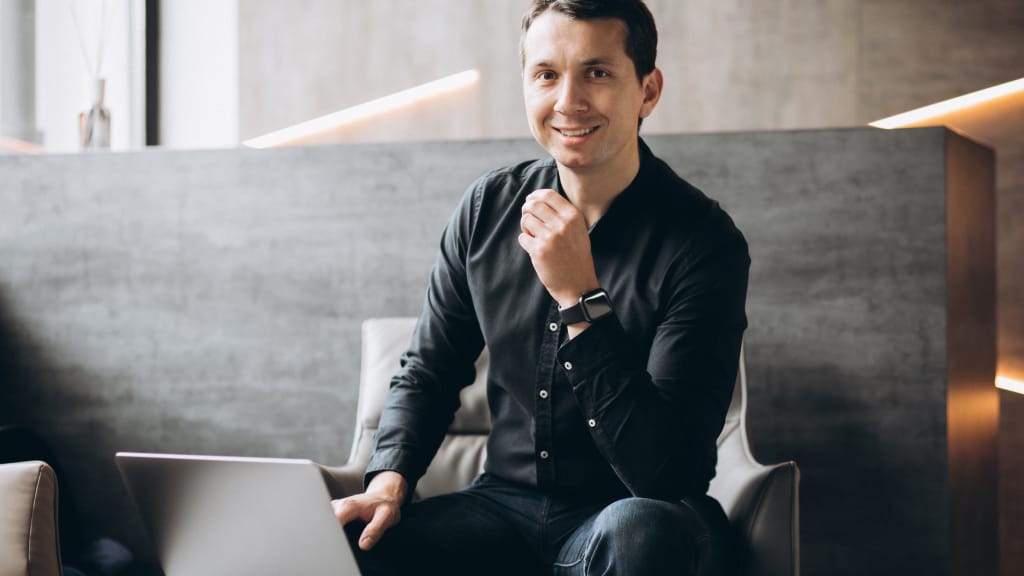
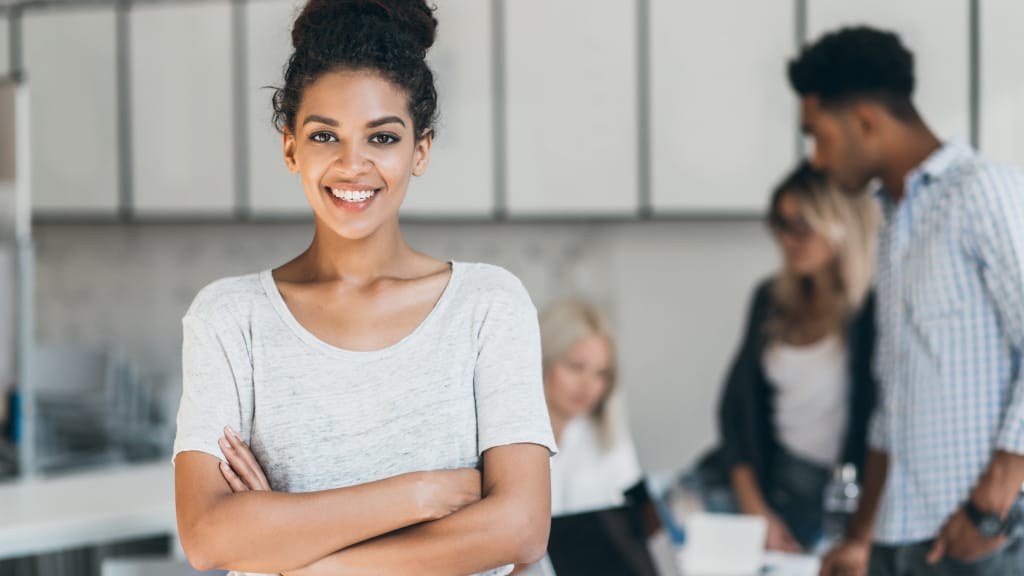
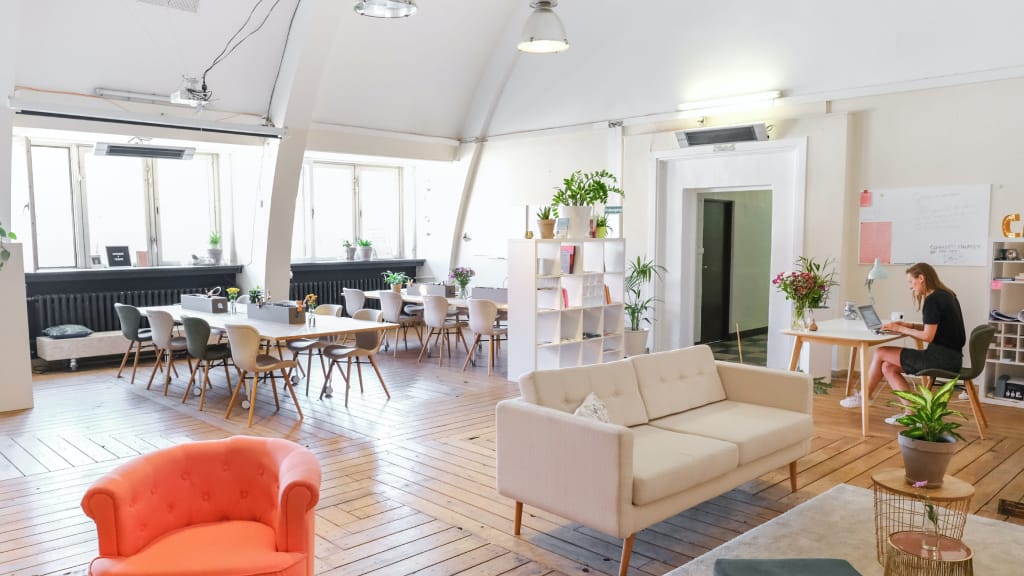
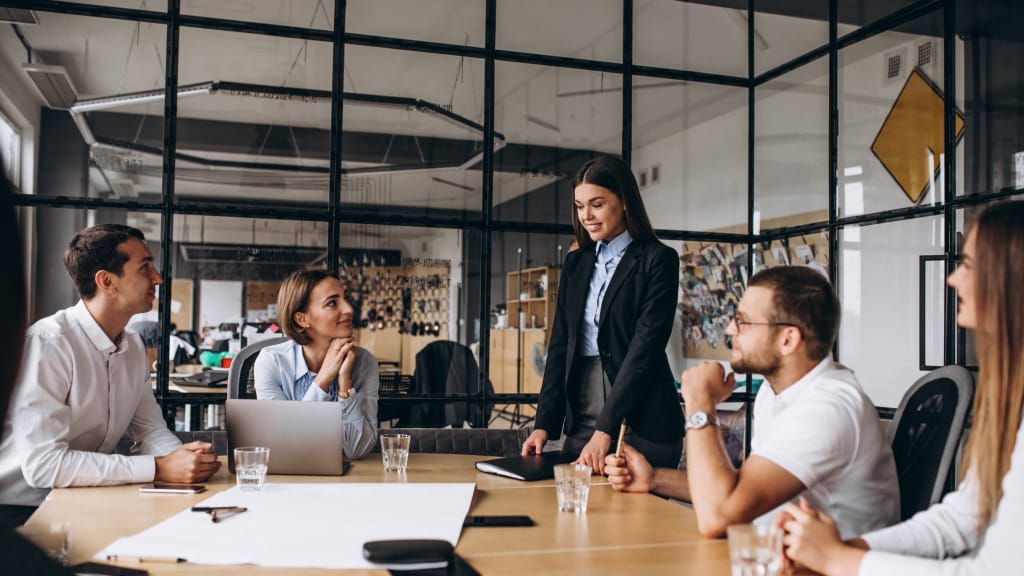
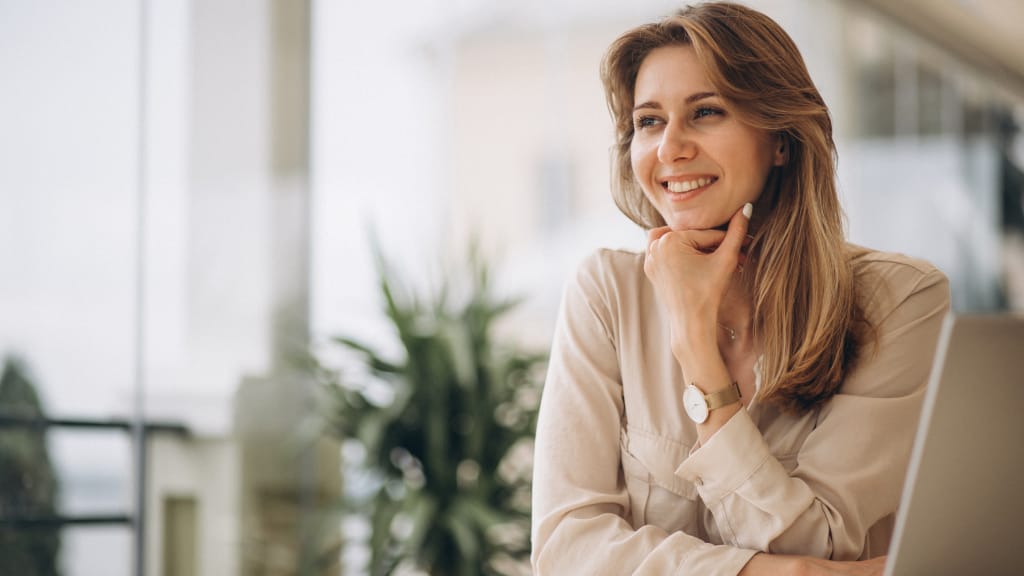
Python for Generative AI
Generative Artificial Intelligence (AI) refers to the use of machine learning algorithms and models to create new, original content such as images, music, and text. Python, with its extensive libraries and frameworks, provides a powerful platform for developing generative AI models. In this article, we'll explore how Python can be used for generative AI and provide example code snippets to showcase its capabilities.
Example 1: Generating Images with Deep Convolutional Generative Adversarial Networks (DCGAN)
Deep Convolutional Generative Adversarial Networks (DCGAN) is a popular architecture for generating realistic images. Here's an example of using Python and TensorFlow to implement a DCGAN model:
import tensorflow as tf
from tensorflow.keras import layers
# Define the generator model
def build_generator():
model = tf.keras.Sequential()
model.add(layers.Dense(7*7*256, use_bias=False, input_shape=(100,)))
model.add(layers.BatchNormalization())
model.add(layers.LeakyReLU())
model.add(layers.Reshape((7, 7, 256)))
model.add(layers.Conv2DTranspose(128, (5, 5), strides=(1, 1), padding='same', use_bias=False))
model.add(layers.BatchNormalization())
model.add(layers.LeakyReLU())
model.add(layers.Conv2DTranspose(64, (5, 5), strides=(2, 2), padding='same', use_bias=False))
model.add(layers.BatchNormalization())
model.add(layers.LeakyReLU())
model.add(layers.Conv2DTranspose(1, (5, 5), strides=(2, 2), padding='same', use_bias=False, activation='tanh'))
return model
# Define the discriminator model
def build_discriminator():
model = tf.keras.Sequential()
model.add(layers.Conv2D(64, (5, 5), strides=(2, 2), padding='same',
input_shape=[28, 28, 1]))
model.add(layers.LeakyReLU())
model.add(layers.Dropout(0.3))
model.add(layers.Conv2D(128, (5, 5), strides=(2, 2), padding='same'))
model.add(layers.LeakyReLU())
model.add(layers.Dropout(0.3))
model.add(layers.Flatten())
model.add(layers.Dense(1))
return model
# Define the loss function
cross_entropy = tf.keras.losses.BinaryCrossentropy(from_logits=True)
# Define the generator and discriminator
generator = build_generator()
discriminator = build_discriminator()
# Define the generator loss
def generator_loss(fake_output):
return cross_entropy(tf.ones_like(fake_output), fake_output)
# Define the discriminator loss
def discriminator_loss(real_output, fake_output):
real_loss = cross_entropy(tf.ones_like(real_output), real_output)
fake_loss = cross_entropy(tf.zeros_like(fake_output), fake_output)
total_loss = real_loss + fake_loss
return total_loss
# Define the optimizers
generator_optimizer = tf.keras.optimizers.Adam(1e-4)
discriminator_optimizer = tf.keras.optimizers.Adam(1e-4)
This code demonstrates the implementation of a DCGAN model using TensorFlow. We define the generator and discriminator models, as well as the loss functions and optimizers. The generator model takes a random noise vector as input and generates a new image. The discriminator model distinguishes between real images and generated images. The generator and discriminator are trained simultaneously in an adversarial manner, where the generator aims to fool the discriminator, and the discriminator aims to correctly classify real and fake images.
Example 2: Generating Text with Recurrent Neural Networks (RNNs)
Recurrent Neural Networks (RNNs) are widely used for generating text sequences. Here's an example of using Python and the Keras library to build an RNN model for text generation:
import tensorflow as tf
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Dense, LSTM, Embedding
# Define the model architecture
model = Sequential()
model.add(Embedding(input_dim=vocab_size, output_dim=embedding_dim, input_length=seq_length))
model.add(LSTM(units=128, return_sequences=True))
model.add(LSTM(units=128))
model.add(Dense(units=vocab_size, activation='softmax'))
# Compile the model
model.compile(loss='categorical_crossentropy', optimizer='adam', metrics=['accuracy'])
# Train the model
model.fit(X_train, y_train, epochs=10, batch_size=128)
# Generate text using the trained model
seed_text = "Once upon a time"
generated_text = seed_text
for _ in range(num_words):
encoded = tokenizer.texts_to_sequences([seed_text])[0]
encoded = pad_sequences([encoded], maxlen=seq_length, truncating='pre')
y_pred = np.argmax(model.predict(encoded), axis=-1)
predicted_word = reverse_word_index[y_pred[0]]
generated_text += " " + predicted_word
seed_text += " " + predicted_word
print(generated_text)
In this code, we define an RNN model using the Sequential API from Keras. The model includes an embedding layer, LSTM layers, and a dense layer with softmax activation. We compile the model with categorical cross-entropy loss and the Adam optimizer. We then train the model on a text dataset, and finally, we generate new text by providing a seed text and iteratively predicting the next word based on the model's output probabilities.
Conclusion
Python provides a powerful platform for developing generative AI models. The examples presented in this article demonstrate how Python, along with libraries like TensorFlow and Keras, can be used to generate images and text using deep learning techniques. Whether you're interested in image generation, text generation, or other forms of generative AI, Python's flexibility, extensive libraries, and supportive community will enable you to explore and create innovative generative AI applications. Feel free to explore these examples further and leverage Python for your own generative AI projects!
4.5L
Learners
20+
Instructors
50+
Courses
6.0L
Course enrollments
Future Trending Courses
When selecting, a course, Here are a few areas that are expected to be in demand in the future:.
Future Learning for all
If you’re passionate and ready to dive in, we’d love to join 1:1 classes for you. We’re committed to support our learners and professionals their development and well-being.
View Courses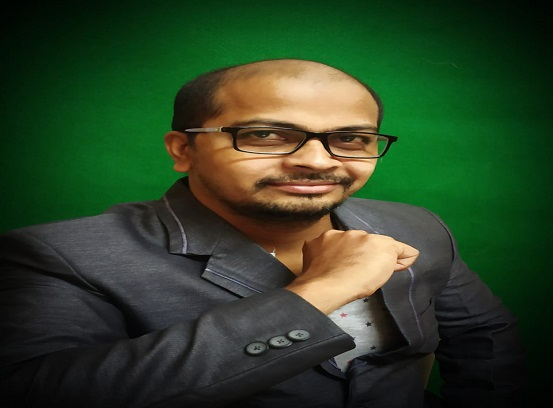
Most Popular Course topics
These are the most popular course topics among Software Courses for learners