Hi there, we’re Harisystems
"Unlock your potential and soar to new heights with our exclusive online courses! Ignite your passion, acquire valuable skills, and embrace limitless possibilities. Don't miss out on our limited-time sale - invest in yourself today and embark on a journey of personal and professional growth. Enroll now and shape your future with knowledge that lasts a lifetime!".
For corporate trainings, projects, and real world experience reach us. We believe that education should be accessible to all, regardless of geographical location or background.
1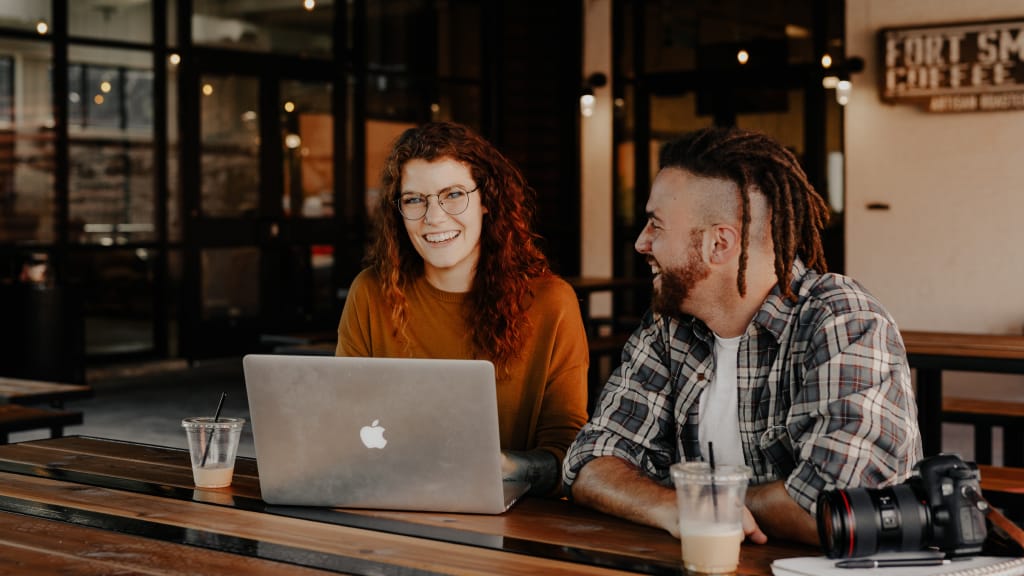
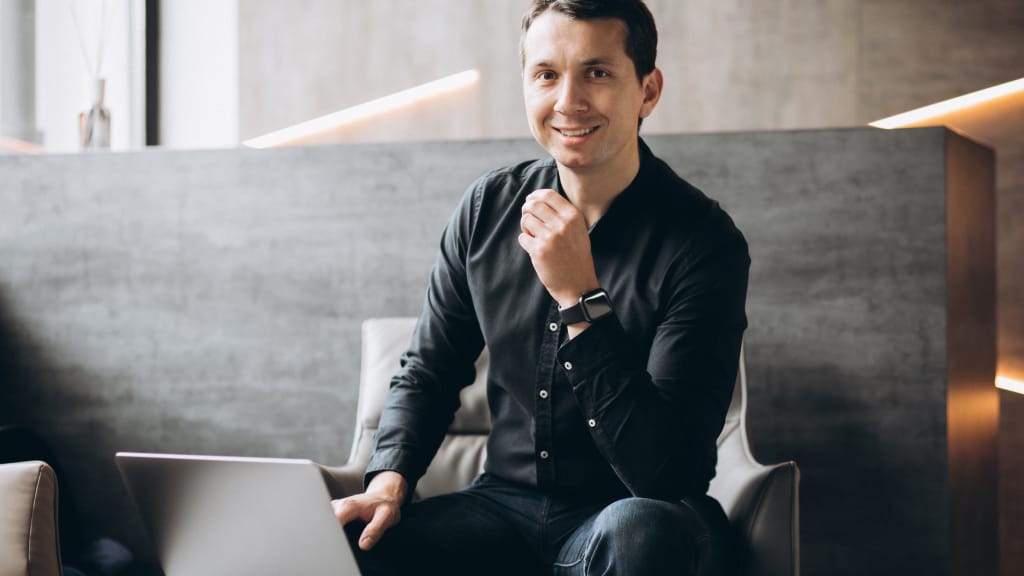
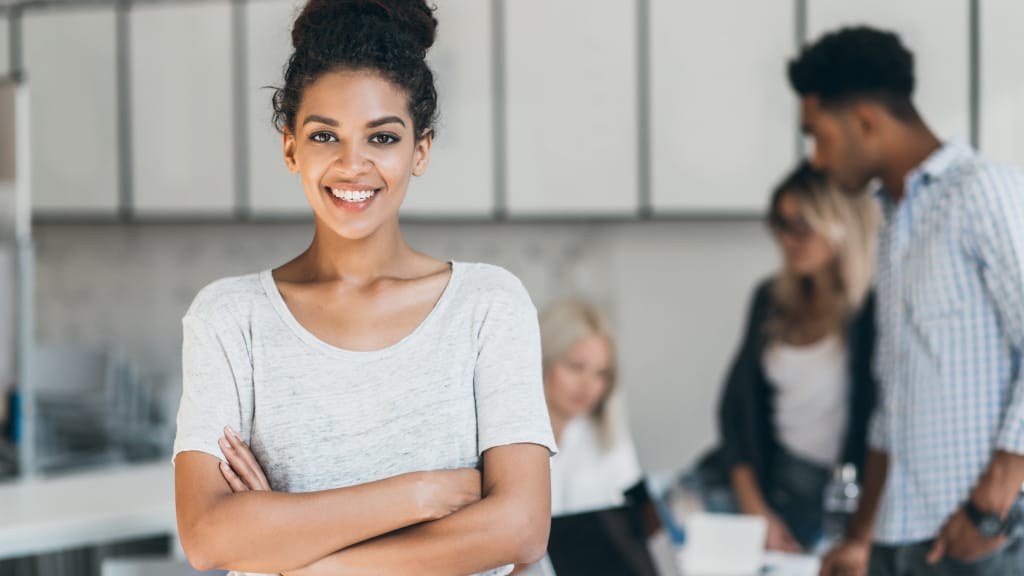
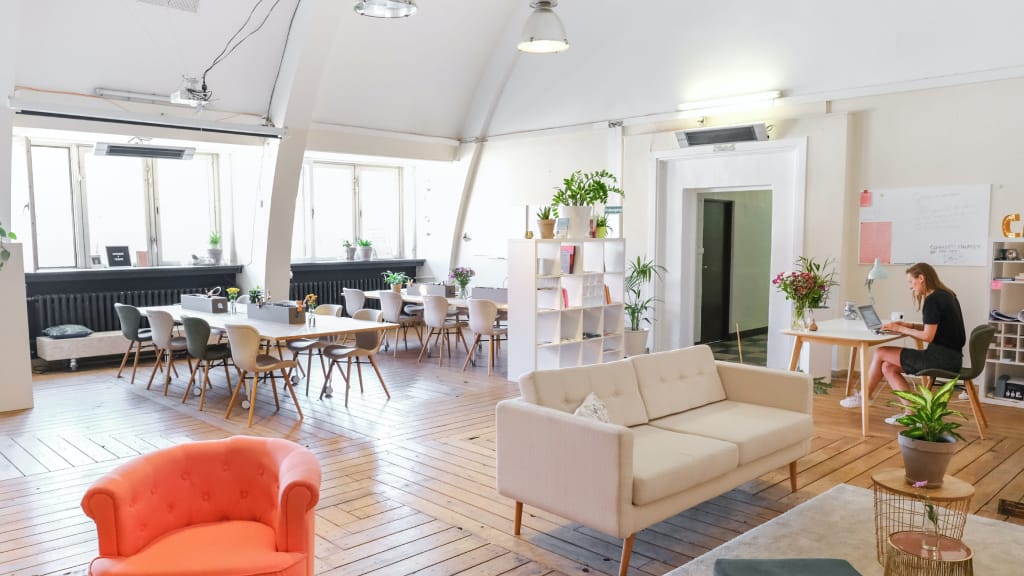
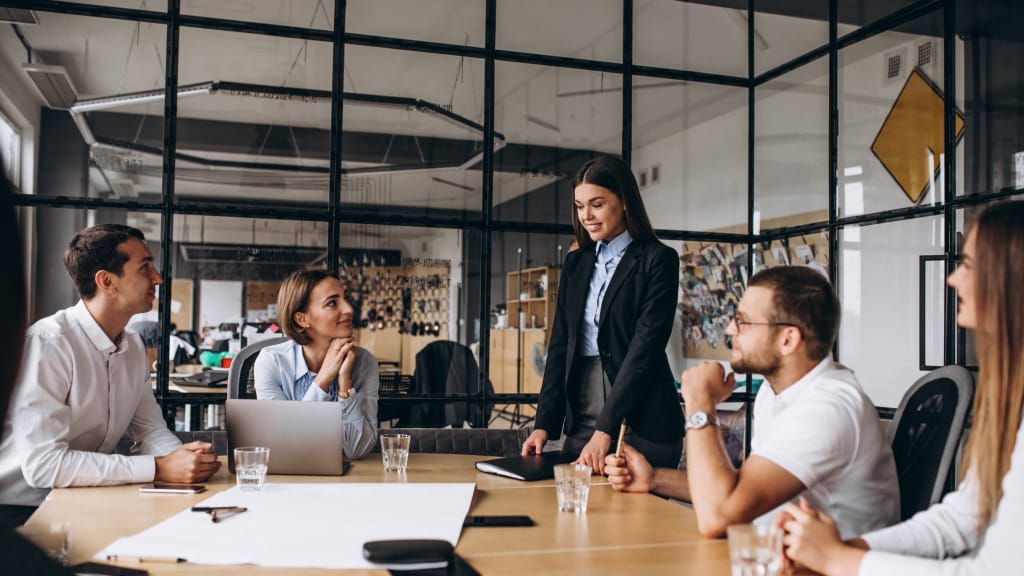
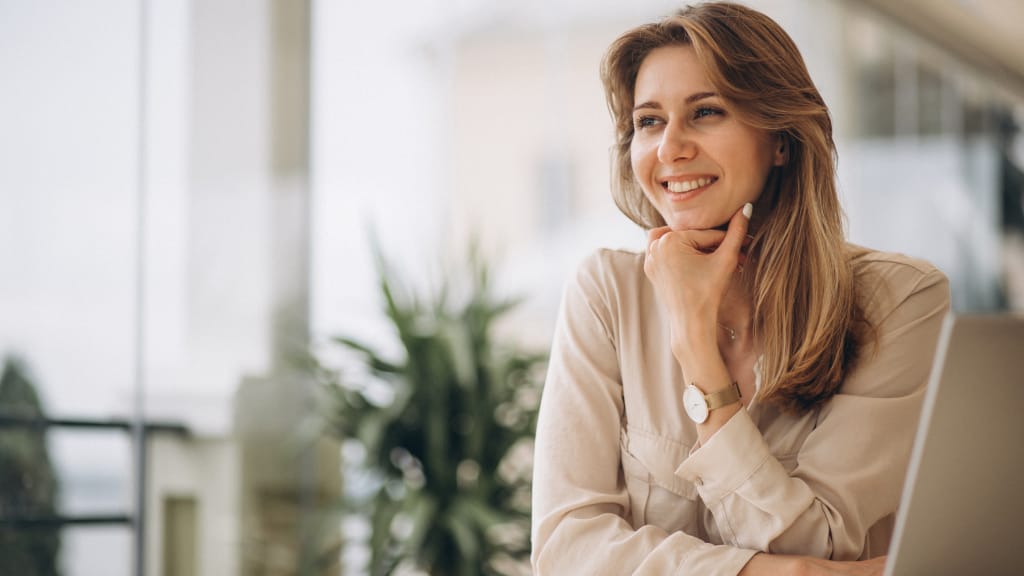
C++ Object-Oriented Programming
C++ is a powerful programming language that supports object-oriented programming (OOP) paradigm. OOP allows you to organize your code into objects, which are instances of classes. This approach promotes code reusability, modularity, and provides a structured way to design and implement complex systems. In this article, we will explore the fundamentals of object-oriented programming in C++ with examples.
1. Classes and Objects
In C++, a class is a blueprint or a template for creating objects. It defines the properties and behaviors that objects of the class will possess. Here's an example:
#include <iostream>
#include <string>
// Define a class
class Person {
private:
std::string name;
int age;
public:
// Constructor
Person(const std::string& _name, int _age) : name(_name), age(_age) {}
// Member function
void displayInfo() {
std::cout << "Name: " << name << std::endl;
std::cout << "Age: " << age << std::endl;
}
};
int main() {
// Create an object of the class
Person person("John Doe", 25);
// Call the member function
person.displayInfo();
return 0;
}
In the above code, we define a class named Person
that has two private member variables: name
and age
. We also have a constructor that initializes these variables. Additionally, we have a member function displayInfo()
that outputs the person's name and age. In the main()
function, we create an object of the class Person
and call its displayInfo()
member function.
2. Encapsulation and Access Modifiers
Encapsulation is a key concept in OOP that allows you to hide the internal implementation details of a class and provide a public interface for interacting with the object. In C++, you can achieve encapsulation using access modifiers: public
, private
, and protected
. Here's an example:
#include <iostream>
#include <string>
class Rectangle {
private:
double length;
double width;
public:
Rectangle(double _length, double _width) : length(_length), width(_width) {}
double calculateArea() {
return length * width;
}
};
int main() {
Rectangle rectangle(5.0, 3.0);
double area = rectangle.calculateArea();
std::cout << "Area: " << area << std::endl;
return 0;
}
In the above code, we define a class Rectangle
with two private member variables: length
and width
. We also have a constructor and a member function calculateArea()
that calculates and returns the area of the rectangle. In the main()
function, we create an object of the class Rectangle
and call its calculateArea()
member function.
3. Inheritance and Polymorphism
Inheritance is another important concept in OOP that allows you to create new classes based on existing classes. In C++, you can derive a class from a base class using the class
derived : access-specifier base
syntax. Here's an example:
#include <iostream>
#include <string>
class Animal {
public:
void makeSound() {
std::cout << "The animal makes a sound." << std::endl;
}
};
class Dog : public Animal {
public:
void makeSound() {
std::cout << "The dog barks." << std::endl;
}
};
int main() {
Animal animal;
animal.makeSound();
Dog dog;
dog.makeSound();
return 0;
}
In the above code, we define a base class Animal
with a member function makeSound()
. We then define a derived class Dog
that inherits from the Animal
class. The Dog
class overrides the makeSound()
member function. In the main()
function, we create objects of both classes and call the makeSound()
member function for each object.
Object-oriented programming in C++ provides a structured and organized approach to designing and implementing complex systems. By utilizing classes, objects, encapsulation, inheritance, and polymorphism, you can create modular, reusable, and extensible code. Embrace the power of OOP to build robust and flexible C++ applications.
4.5L
Learners
20+
Instructors
50+
Courses
6.0L
Course enrollments
Future Trending Courses
When selecting, a course, Here are a few areas that are expected to be in demand in the future:.
Future Learning for all
If you’re passionate and ready to dive in, we’d love to join 1:1 classes for you. We’re committed to support our learners and professionals their development and well-being.
View Courses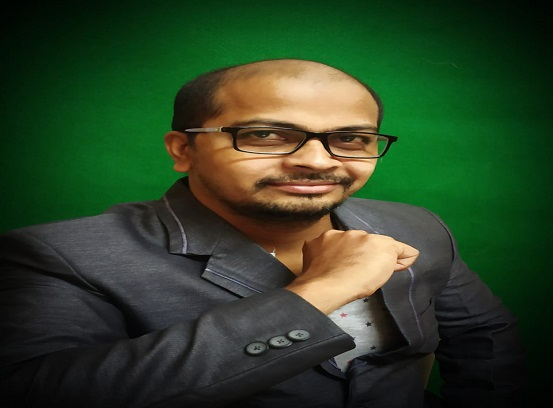
Most Popular Course topics
These are the most popular course topics among Software Courses for learners