Hi there, we’re Harisystems
"Unlock your potential and soar to new heights with our exclusive online courses! Ignite your passion, acquire valuable skills, and embrace limitless possibilities. Don't miss out on our limited-time sale - invest in yourself today and embark on a journey of personal and professional growth. Enroll now and shape your future with knowledge that lasts a lifetime!".
For corporate trainings, projects, and real world experience reach us. We believe that education should be accessible to all, regardless of geographical location or background.
1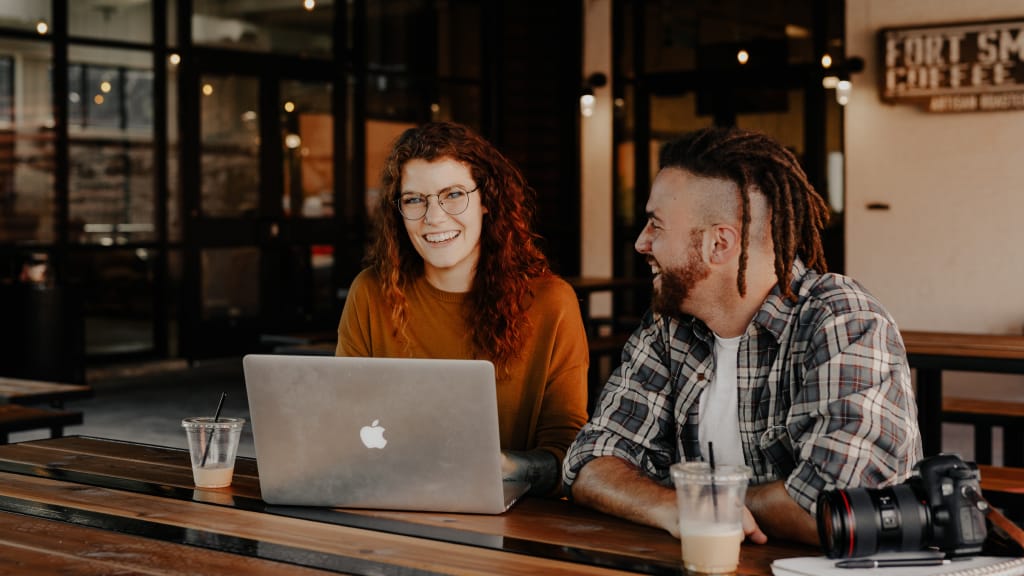
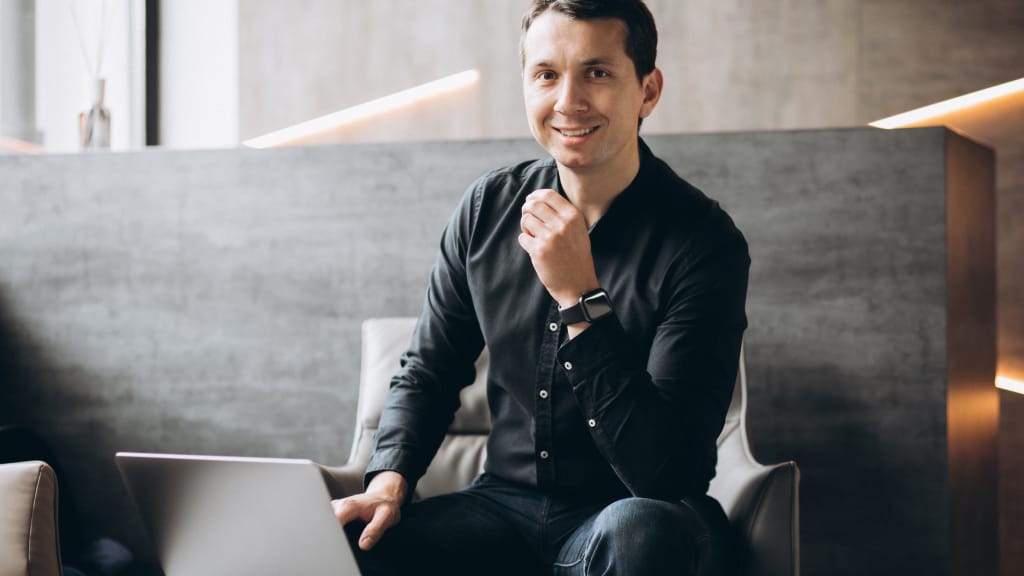
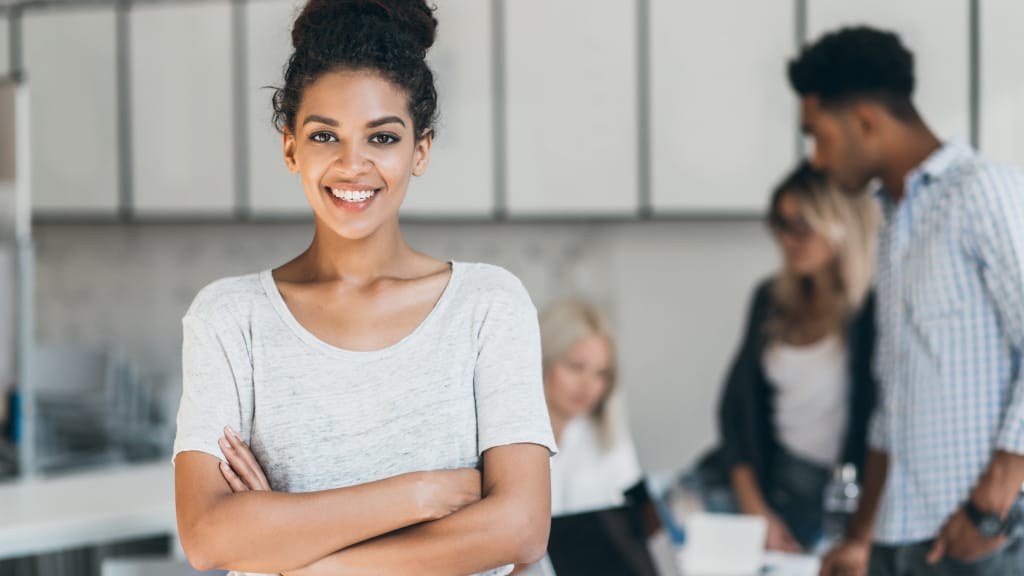
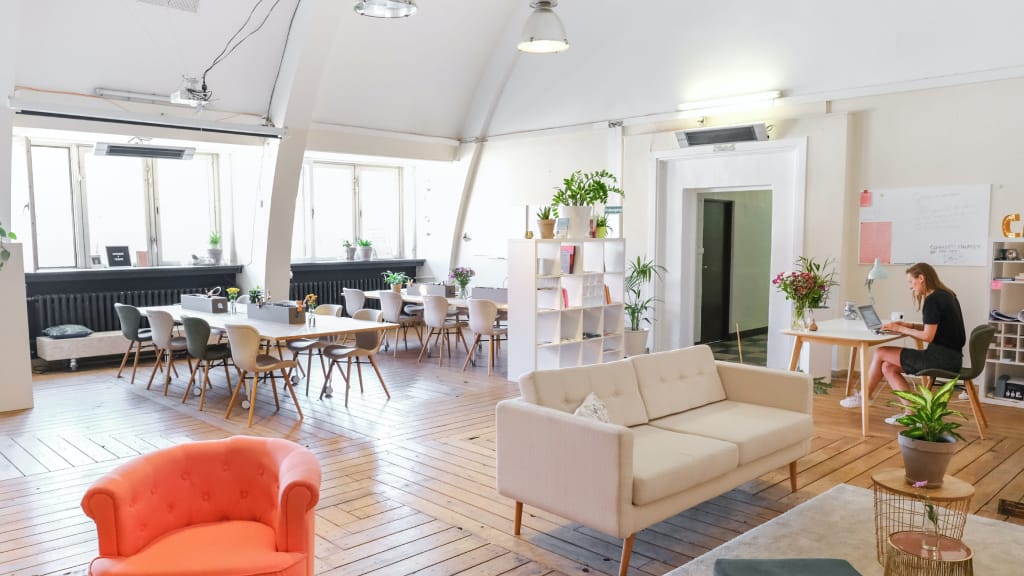
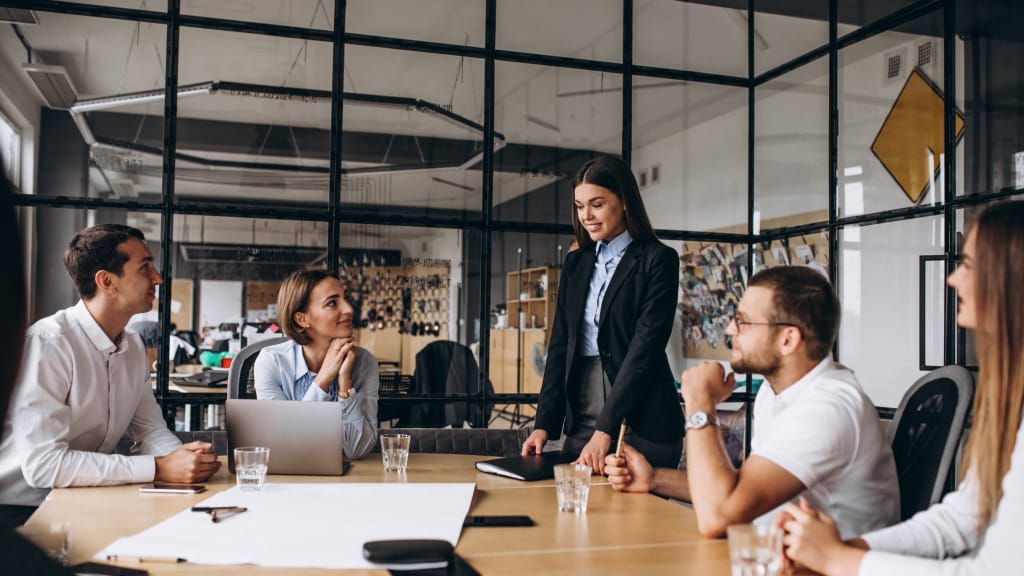
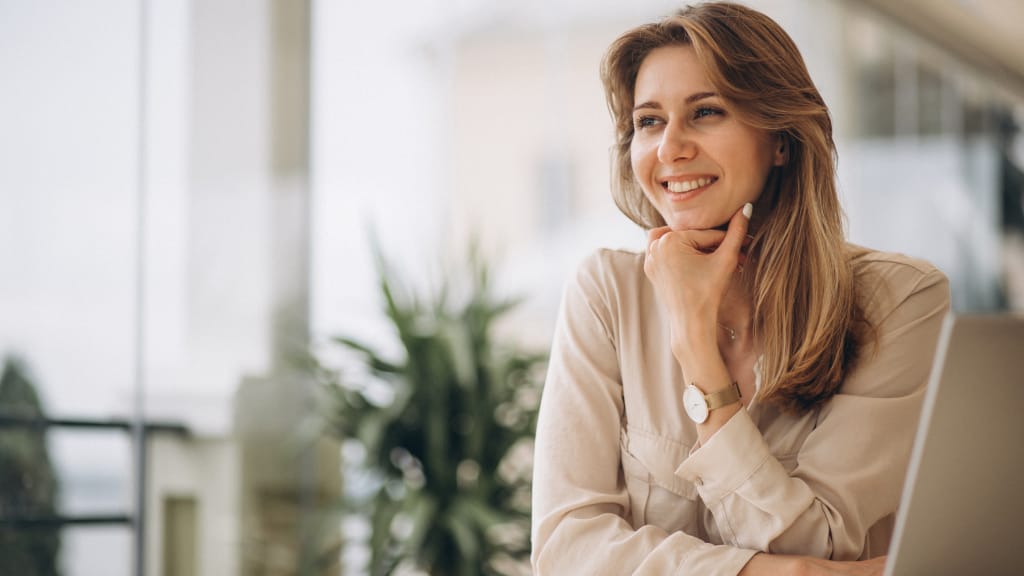
Java if-else Statements: Controlling Program Flow
In Java, the if-else
statement is used to control the flow of a program based on certain conditions. It allows you to execute different blocks of code depending on whether a condition is true or false. In this article, we will explore the if-else
statement in Java and provide examples to help you understand its usage.
The if-else Statement Syntax
The syntax of the if-else
statement in Java is as follows:
if (condition) {
// code to execute if the condition is true
} else {
// code to execute if the condition is false
}
The condition
is a boolean expression that evaluates to either true
or false
. If the condition
is true, the code block within the if
statement is executed. Otherwise, the code block within the else
statement is executed.
Example: Checking a Number
Let's consider an example where we want to check if a number is positive or negative. We can use an if-else
statement to perform this check:
int number = 10;
if (number > 0) {
System.out.println("The number is positive.");
} else {
System.out.println("The number is negative.");
}
In the above code, if the number
is greater than zero, the message "The number is positive."
is printed. Otherwise, the message "The number is negative."
is printed.
Nested if-else Statements
You can also nest if-else
statements within each other to handle more complex conditions. Here's an example:
int score = 85;
if (score >= 90) {
System.out.println("Excellent!");
} else if (score >= 80) {
System.out.println("Good job!");
} else if (score >= 70) {
System.out.println("Keep it up!");
} else {
System.out.println("You can do better.");
}
In the above code, the program checks the score
and prints different messages based on the score range. If the score is 85, the message "Good job!"
is printed.
Conclusion
The if-else
statement is a fundamental construct in Java that allows you to control the flow of a program based on conditions. In this article, we explored the syntax of the if-else
statement and provided examples to demonstrate its usage. We also discussed the concept of nested if-else
statements for handling complex conditions. By using if-else
statements effectively, you can make your programs more flexible and responsive to different scenarios. Continuously practice using if-else
statements and explore more advanced topics, such as switch statements and ternary operators, to enhance your control flow capabilities in Java programming.
4.5L
Learners
20+
Instructors
50+
Courses
6.0L
Course enrollments
Future Trending Courses
When selecting, a course, Here are a few areas that are expected to be in demand in the future:.
Future Learning for all
If you’re passionate and ready to dive in, we’d love to join 1:1 classes for you. We’re committed to support our learners and professionals their development and well-being.
View Courses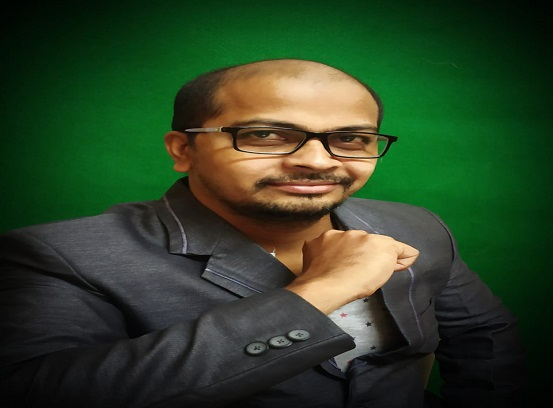
Most Popular Course topics
These are the most popular course topics among Software Courses for learners