Hi there, we’re Harisystems
"Unlock your potential and soar to new heights with our exclusive online courses! Ignite your passion, acquire valuable skills, and embrace limitless possibilities. Don't miss out on our limited-time sale - invest in yourself today and embark on a journey of personal and professional growth. Enroll now and shape your future with knowledge that lasts a lifetime!".
For corporate trainings, projects, and real world experience reach us. We believe that education should be accessible to all, regardless of geographical location or background.
1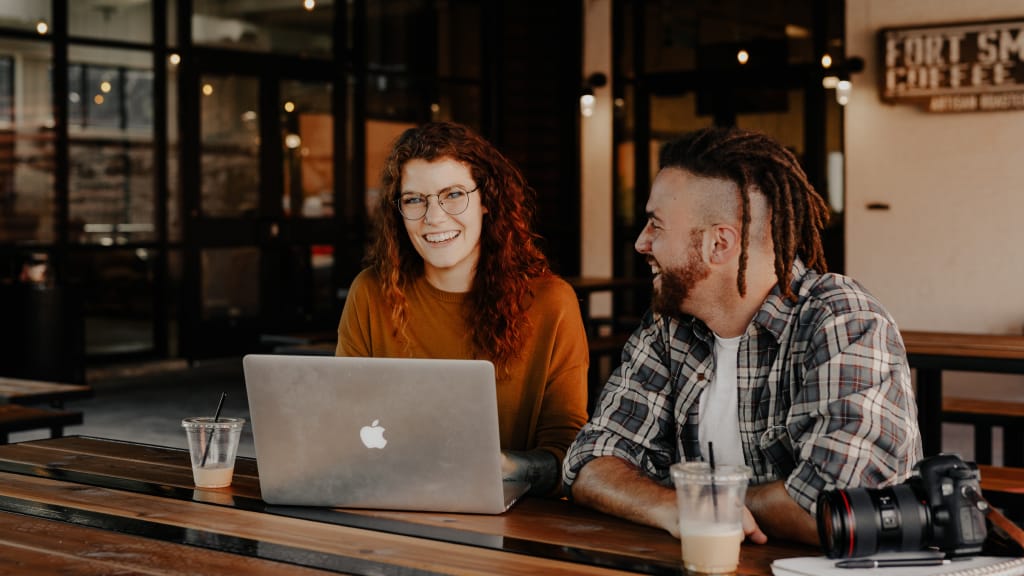
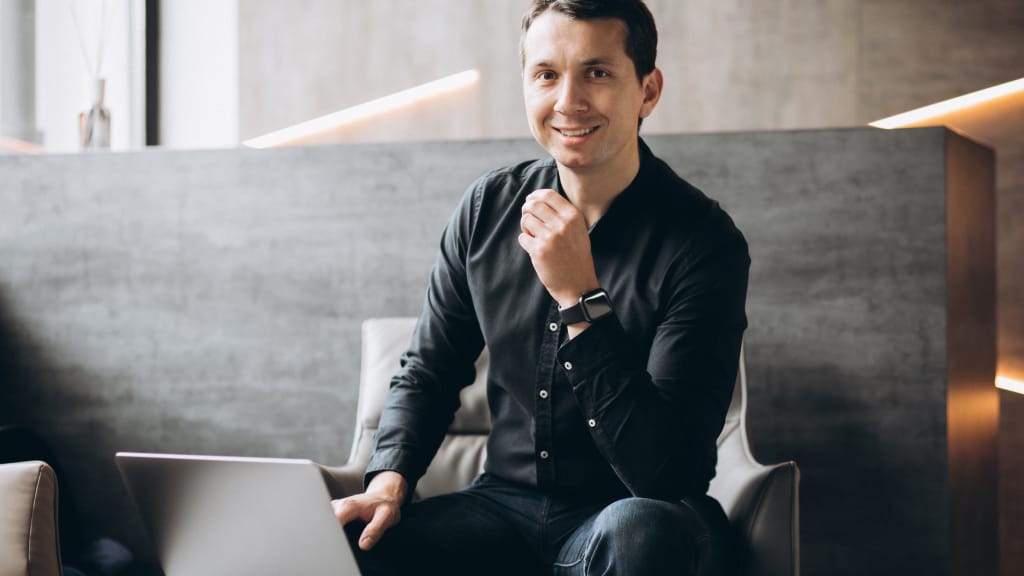
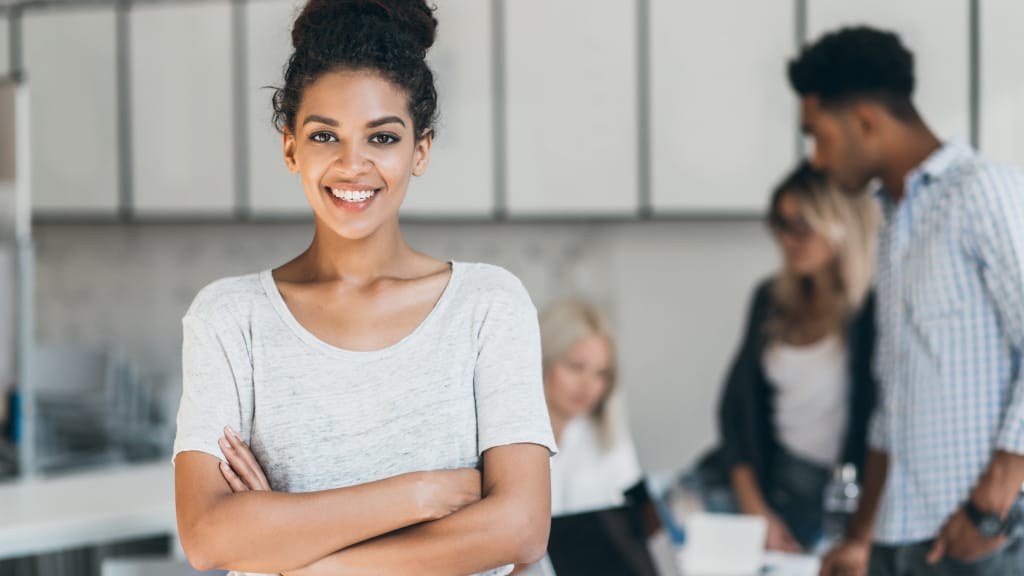
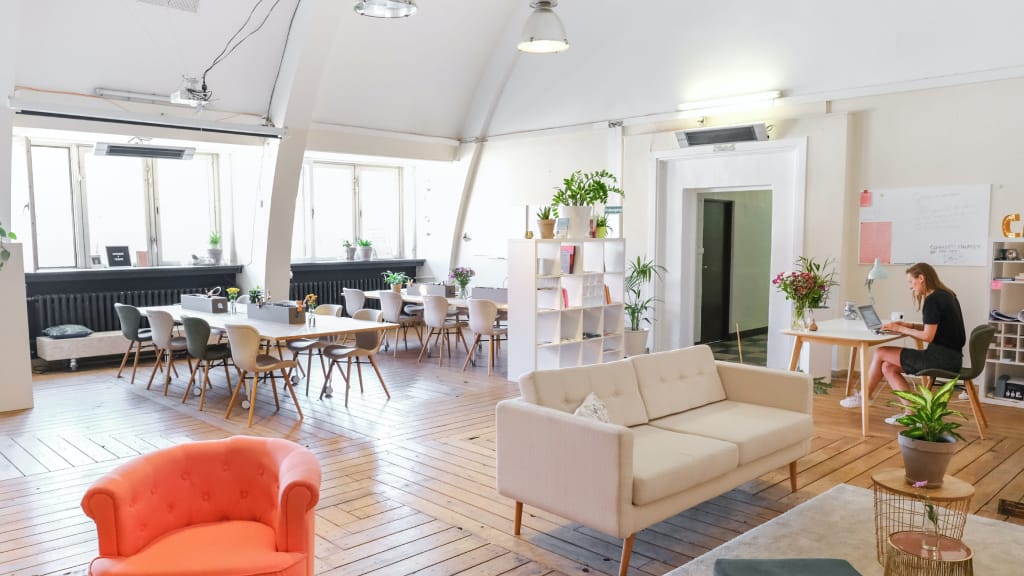
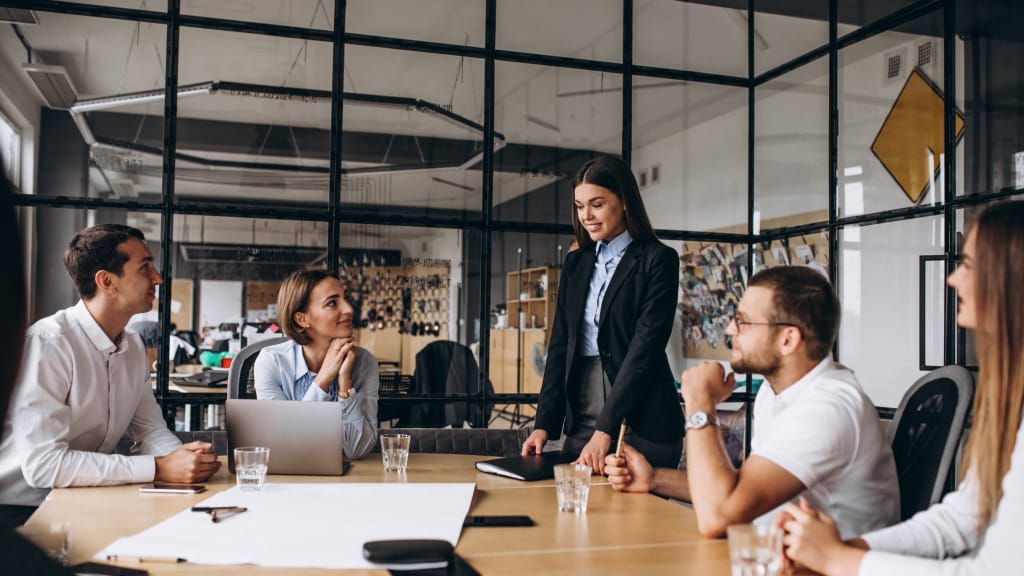
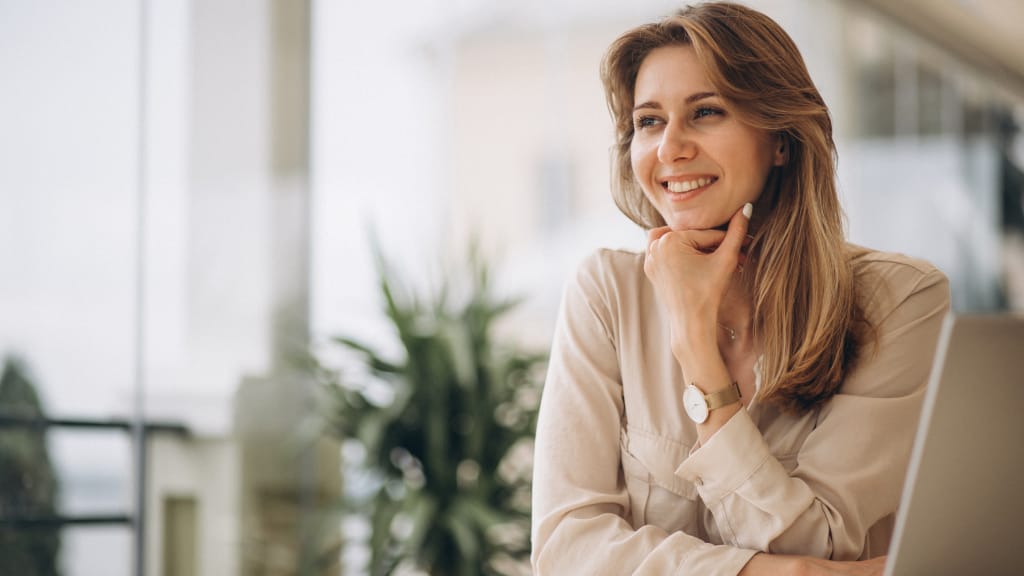
Web Development: Building Dynamic Web Applications with AngularJS
AngularJS is a popular JavaScript framework that simplifies web development by providing a structured approach to building dynamic and responsive web applications. It enhances HTML with additional features and allows developers to create interactive user interfaces and handle data binding effortlessly. In this article, we will explore the basics of using AngularJS and provide examples of its implementation in web development projects.
What is AngularJS?
AngularJS is a client-side JavaScript framework developed by Google. It extends HTML by adding custom elements and attributes that enable dynamic and declarative programming. AngularJS follows the Model-View-Controller (MVC) architectural pattern, which helps in separating concerns and improving code organization.
Setting Up AngularJS
To start using AngularJS in your web development project, you need to include the AngularJS library in your HTML file. You can download AngularJS from the official AngularJS website or use a CDN (Content Delivery Network) to include it:
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.8.2/angular.min.js"></script>
Creating an AngularJS Application
To create an AngularJS application, you need to define an application module and attach it to an HTML element. Here's an example:
<div ng-app="myApp">
<!-- Your application code goes here -->
</div>
<script>
var app = angular.module('myApp', []);
</script>
Data Binding
Data binding is a powerful feature in AngularJS that allows you to bind data from the controller to the view and vice versa. Here's an example of data binding:
<div ng-app="myApp" ng-controller="myCtrl">
<p>{{ message }}</p>
<input type="text" ng-model="name">
<p>Hello, {{ name }}!</p>
</div>
<script>
var app = angular.module('myApp', []);
app.controller('myCtrl', function($scope) {
$scope.message = "Welcome to AngularJS";
});
</script>
Directives
AngularJS provides a set of built-in directives that extend HTML with additional functionality. Directives are special attributes that modify the behavior of HTML elements. Here's an example:
<div ng-app="myApp">
<p ng-show="showMessage">{{ message }}</p>
<button ng-click="showMessage = true">Show Message</button>
</div>
<script>
var app = angular.module('myApp', []);
</script>
Services
AngularJS services are reusable components that provide functionality across different parts of an application. They can be used to share data, perform HTTP requests, or implement custom logic. Here's an example:
<div ng-app="myApp" ng-controller="myCtrl">
<p>{{ message }}</p>
<button ng-click="updateMessage()">Update Message</button>
</div>
<script>
var app = angular.module('myApp', []);
app.controller('myCtrl', function($scope, myService) {
$scope.message = myService.getMessage();
$scope.updateMessage = function() {
$scope.message = myService.generateMessage();
};
});
app.service('myService', function() {
this.getMessage = function() {
return "Initial message";
};
this.generateMessage = function() {
return "Updated message";
};
});
</script>
Conclusion
AngularJS is a powerful JavaScript framework that simplifies web development by providing a structured approach to building dynamic web applications. Its features such as data binding, directives, and services enhance HTML and enable developers to create interactive user interfaces effortlessly. Understanding the basics of AngularJS and its implementation in web development is essential for building modern and feature-rich web applications. Explore the AngularJS documentation, experiment with its features, and leverage the framework to create engaging and dynamic web experiences.
4.5L
Learners
20+
Instructors
50+
Courses
6.0L
Course enrollments
Future Trending Courses
When selecting, a course, Here are a few areas that are expected to be in demand in the future:.
Future Learning for all
If you’re passionate and ready to dive in, we’d love to join 1:1 classes for you. We’re committed to support our learners and professionals their development and well-being.
View Courses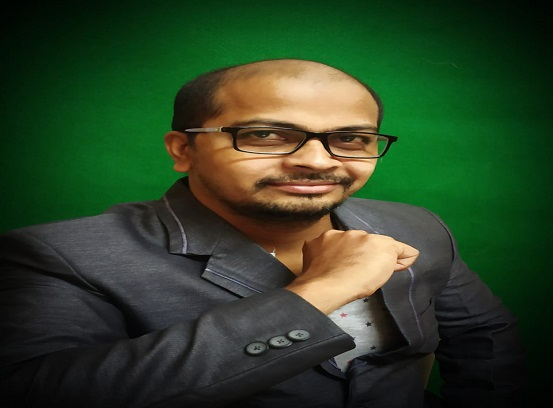
Most Popular Course topics
These are the most popular course topics among Software Courses for learners