Hi there, we’re Harisystems
"Unlock your potential and soar to new heights with our exclusive online courses! Ignite your passion, acquire valuable skills, and embrace limitless possibilities. Don't miss out on our limited-time sale - invest in yourself today and embark on a journey of personal and professional growth. Enroll now and shape your future with knowledge that lasts a lifetime!".
For corporate trainings, projects, and real world experience reach us. We believe that education should be accessible to all, regardless of geographical location or background.
1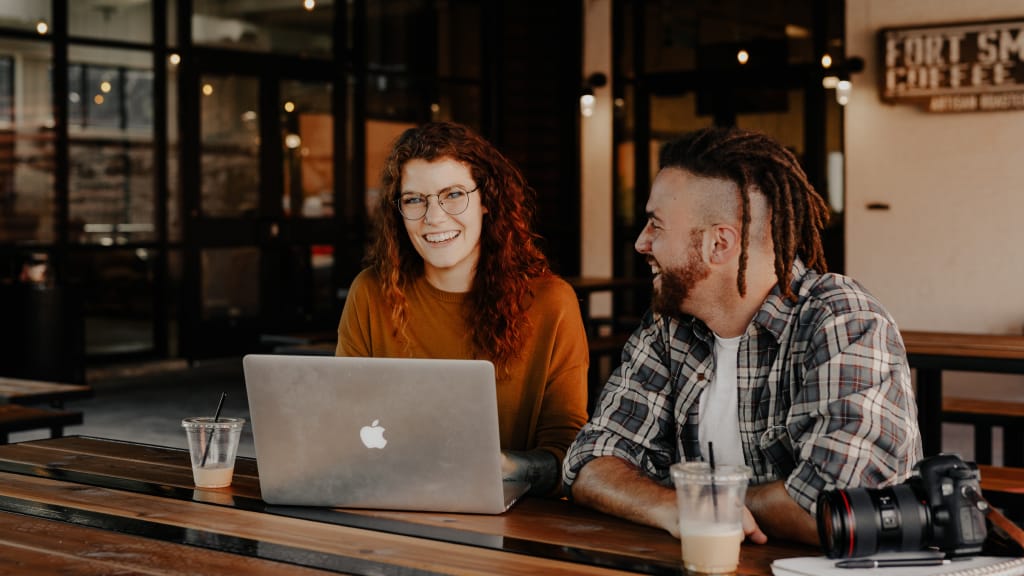
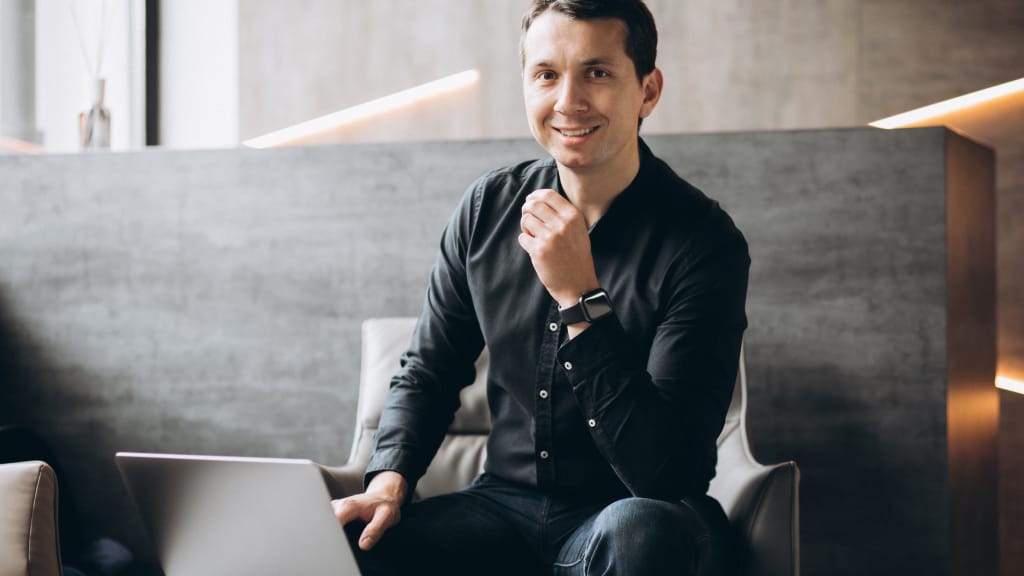
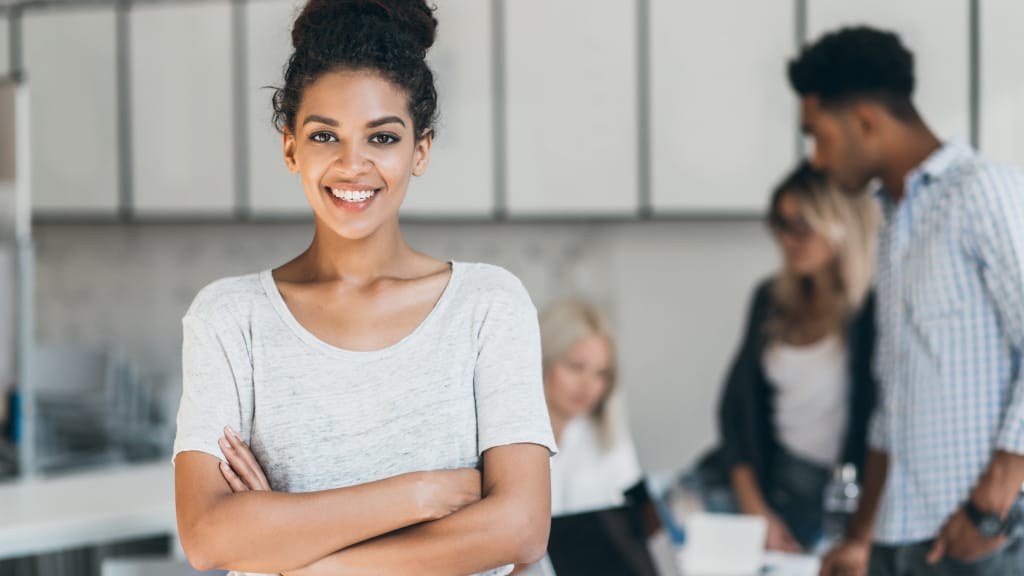
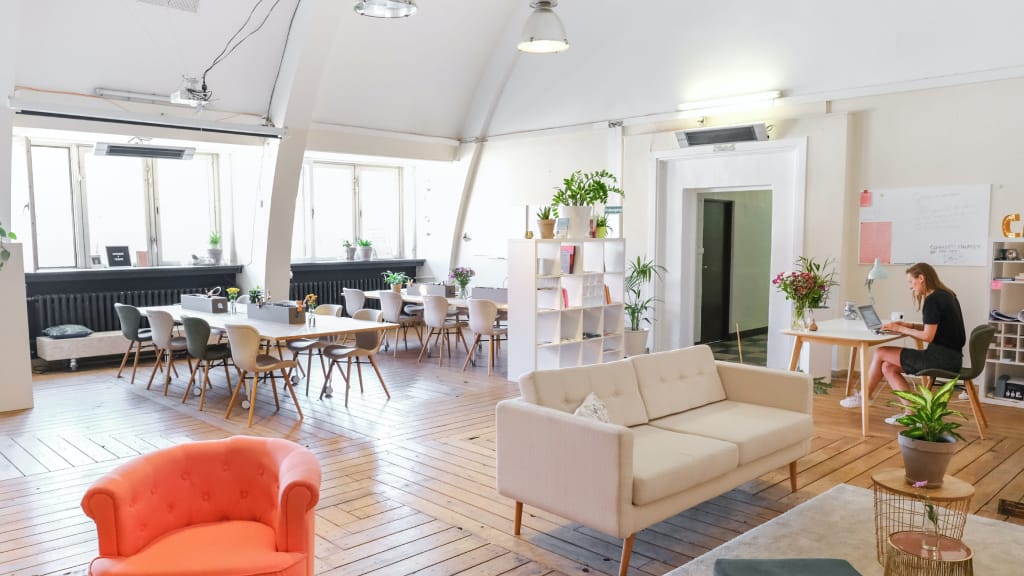
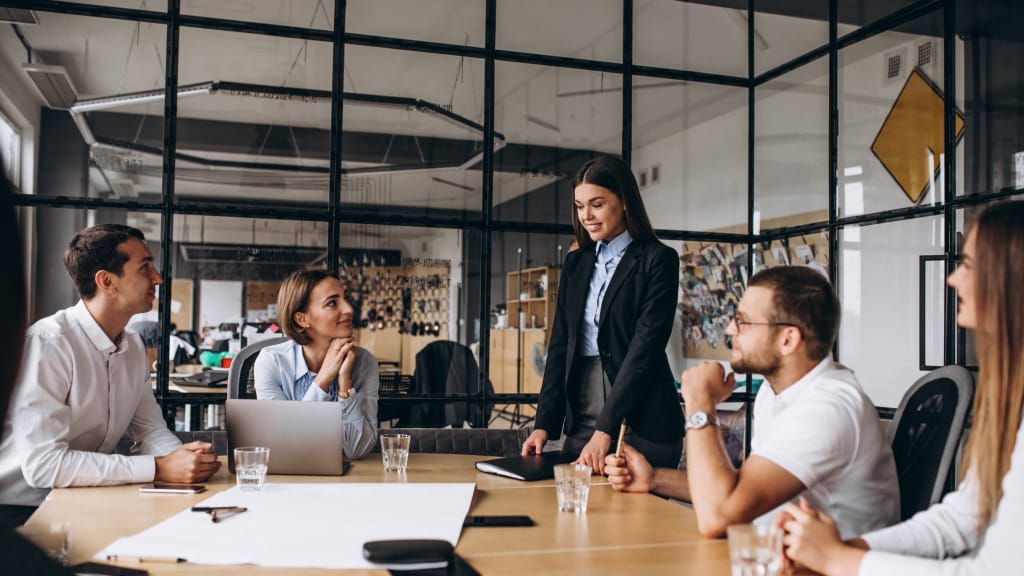
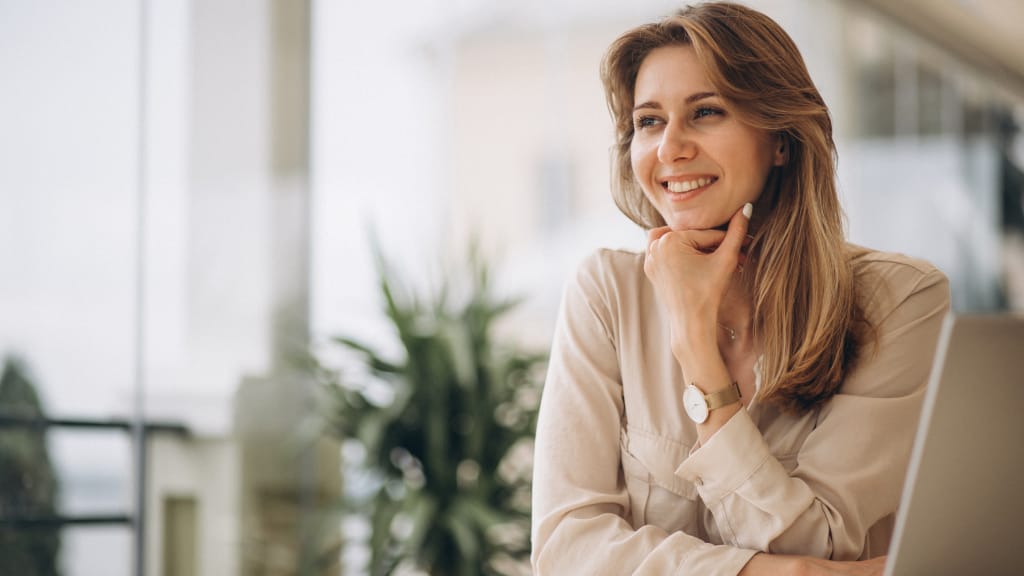
JavaScript for Data Science: Analyzing Data with Code
When it comes to data science, JavaScript may not be the first language that comes to mind. However, JavaScript's versatility and ubiquity make it a valuable tool for data analysis and visualization. In this article, we will explore how JavaScript can be used for data science tasks and provide examples to showcase its capabilities.
1. Data Manipulation
JavaScript provides powerful built-in methods and libraries for data manipulation. One such library is Lodash, which offers a wide range of functions for working with arrays, objects, and collections. Here's an example of using Lodash to calculate the average of an array of numbers:
<script src="https://cdn.jsdelivr.net/npm/lodash@4.17.21/lodash.min.js"></script>
<script>
const numbers = [1, 2, 3, 4, 5];
const average = _.mean(numbers);
console.log(average); // Output: 3
</script>
In this example, we use the '_.mean' function from Lodash to calculate the average of the 'numbers' array. JavaScript libraries like Lodash provide a wide range of functions to perform various data manipulation tasks efficiently.
2. Data Visualization
JavaScript excels at data visualization with its powerful libraries and frameworks. One popular library for creating interactive and dynamic charts is Chart.js. Here's an example of using Chart.js to create a bar chart:
<canvas id="myChart" width="400" height="200"></canvas>
<script src="https://cdn.jsdelivr.net/npm/chart.js@2.9.4/dist/chart.min.js"></script>
<script>
const ctx = document.getElementById('myChart').getContext('2d');
const myChart = new Chart(ctx, {
type: 'bar',
data: {
labels: ['January', 'February', 'March', 'April', 'May'],
datasets: [{
label: 'Sales',
data: [12, 19, 8, 15, 22],
backgroundColor: 'rgba(54, 162, 235, 0.5)',
borderColor: 'rgba(54, 162, 235, 1)',
borderWidth: 1
}]
},
options: {
scales: {
y: {
beginAtZero: true
}
}
}
});
</script>
In this example, we create a bar chart using Chart.js. The chart displays sales data for different months. JavaScript libraries like Chart.js offer a wide range of customization options to create stunning visualizations to represent data effectively.
3. Machine Learning
While JavaScript may not be the primary language for machine learning, it has libraries that allow for basic machine learning tasks. One such library is Brain.js, which provides tools for implementing neural networks in JavaScript. Here's an example of using Brain.js to train a simple neural network for XOR logic:
<script src="https://unpkg.com/brain.js"></script>
<script>
const net = new brain.NeuralNetwork();
const trainingData = [
{ input: [0, 0], output: [0] },
{ input: [0, 1], output: [1] },
{ input: [1, 0], output: [1] },
{ input: [1, 1], output: [0] }
];
net.train(trainingData);
const output = net.run([1, 0]);
console.log(output); // Output: [0.987]
</script>
In this example, we use Brain.js to train a neural network with XOR logic. The trained network is then used to predict the output for a new input. JavaScript libraries like Brain.js provide a simplified way to experiment with basic machine learning concepts.
Conclusion
JavaScript may not be the traditional choice for data science, but its versatility and ecosystem make it a viable option for various data science tasks. With powerful libraries and frameworks available, JavaScript can handle data manipulation, visualization, and even basic machine learning. By harnessing the capabilities of JavaScript, data scientists can leverage their existing web development skills to perform data analysis and gain valuable insights.
4.5L
Learners
20+
Instructors
50+
Courses
6.0L
Course enrollments
Future Trending Courses
When selecting, a course, Here are a few areas that are expected to be in demand in the future:.
Future Learning for all
If you’re passionate and ready to dive in, we’d love to join 1:1 classes for you. We’re committed to support our learners and professionals their development and well-being.
View Courses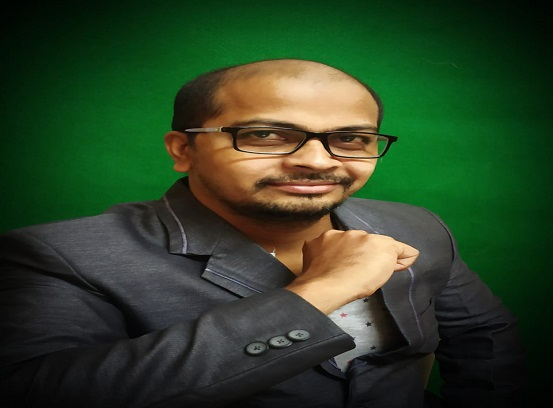
Most Popular Course topics
These are the most popular course topics among Software Courses for learners