Hi there, we’re Harisystems
"Unlock your potential and soar to new heights with our exclusive online courses! Ignite your passion, acquire valuable skills, and embrace limitless possibilities. Don't miss out on our limited-time sale - invest in yourself today and embark on a journey of personal and professional growth. Enroll now and shape your future with knowledge that lasts a lifetime!".
For corporate trainings, projects, and real world experience reach us. We believe that education should be accessible to all, regardless of geographical location or background.
1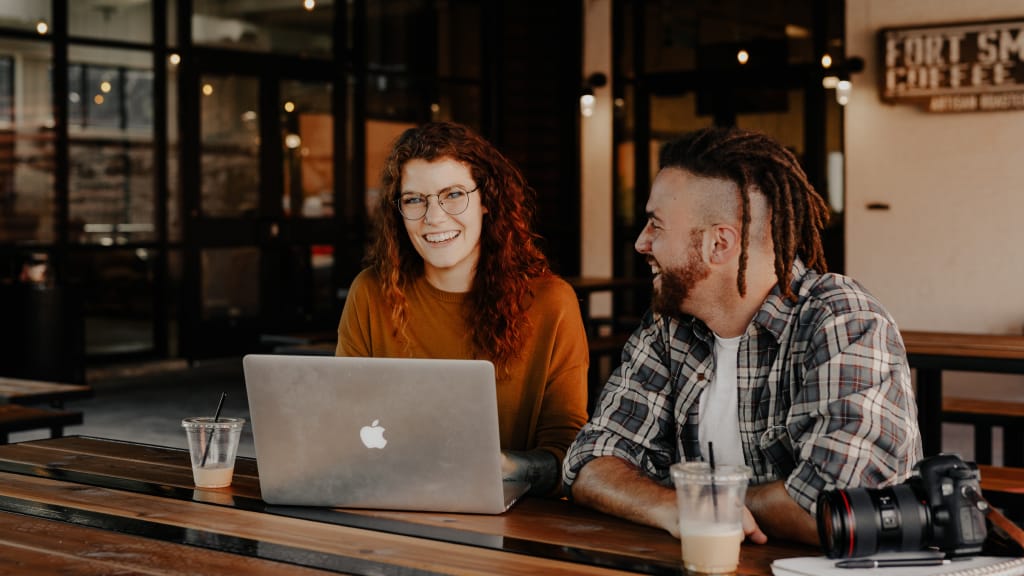
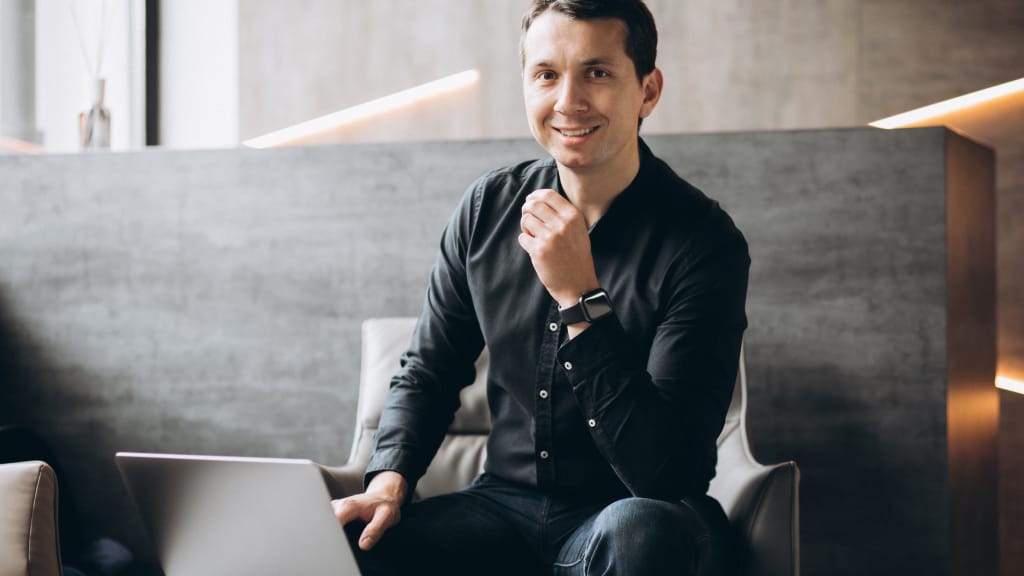
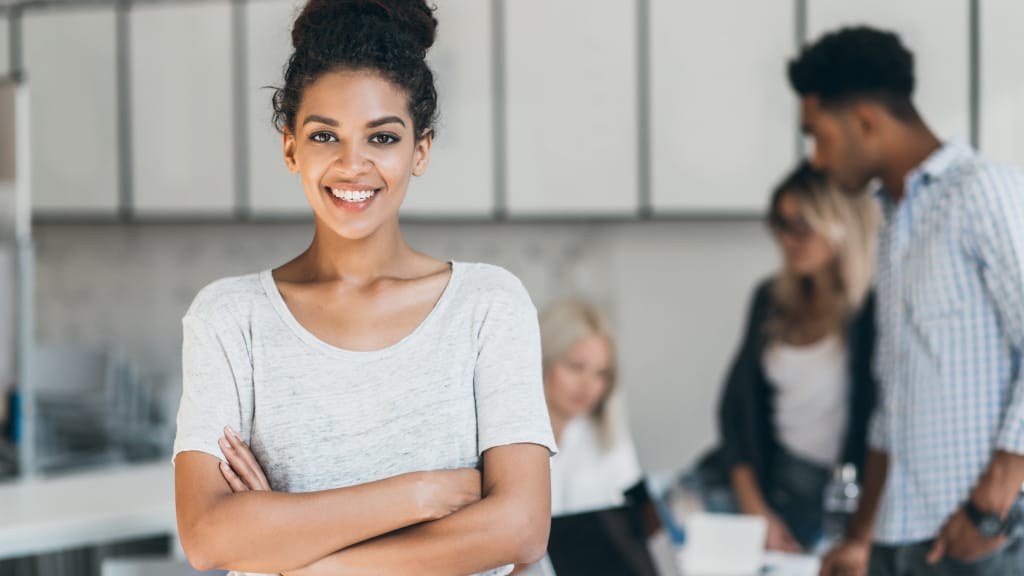
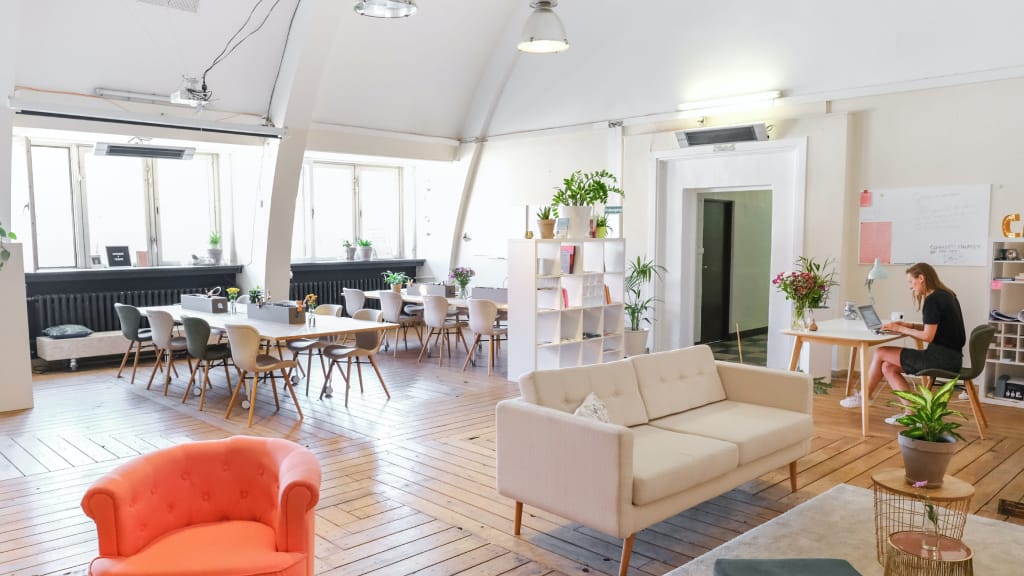
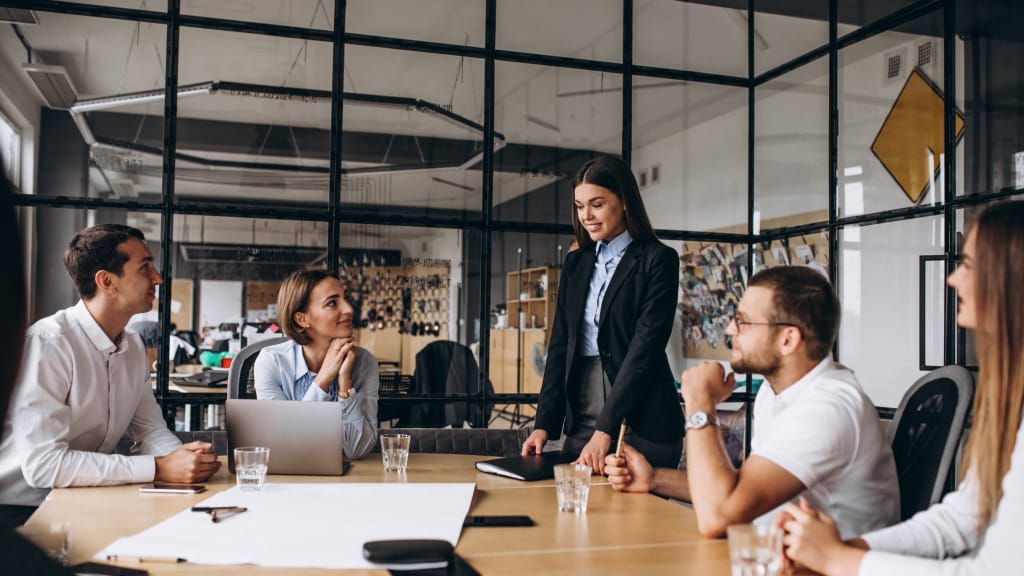
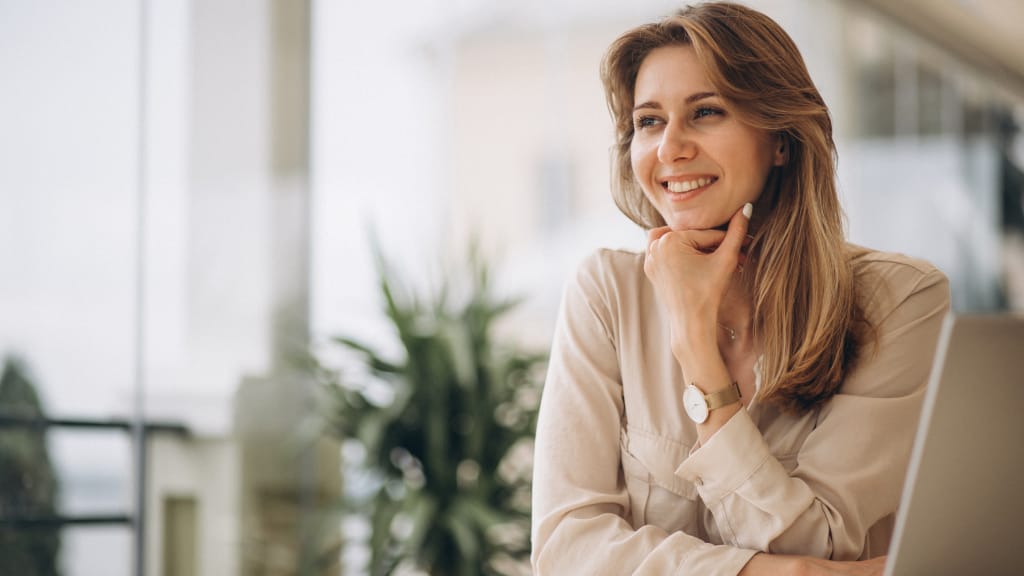
Java Object-Oriented Programming: Building Modular and Reusable Code
Java is an object-oriented programming (OOP) language that emphasizes the concept of objects, encapsulation, inheritance, and polymorphism. OOP allows you to structure your code into modular and reusable components, making it easier to understand, maintain, and extend. In this article, we will explore the fundamentals of object-oriented programming in Java and provide examples to help you understand its usage.
The Basics of Object-Oriented Programming
Object-oriented programming is a programming paradigm that revolves around the concept of objects. An object represents a real-world entity or concept and encapsulates its data (attributes) and behavior (methods) into a single unit. Objects are instances of classes, which are blueprints or templates for creating objects.
Here are the key principles of object-oriented programming:
- Encapsulation: Encapsulation is the practice of bundling data and methods together within a class. It allows you to hide the internal implementation details of an object and expose only the necessary methods for interacting with it.
- Inheritance: Inheritance enables you to create new classes (derived classes or subclasses) based on existing classes (base classes or superclasses). The derived classes inherit the properties and behaviors of the base class, allowing code reuse and promoting the concept of "is-a" relationships.
- Polymorphism: Polymorphism allows objects of different types to be treated as objects of a common superclass. It enables you to write code that can work with objects of different classes, as long as they implement common methods or interfaces.
Example: Creating a Class
Let's consider an example of creating a class to represent a car in Java:
public class Car {
// Attributes
private String brand;
private String color;
private int year;
// Constructor
public Car(String brand, String color, int year) {
this.brand = brand;
this.color = color;
this.year = year;
}
// Methods
public void startEngine() {
System.out.println("Starting the engine of the " + brand + " car.");
}
public void drive() {
System.out.println("Driving the " + brand + " car.");
}
// Getters and setters
public String getBrand() {
return brand;
}
public void setBrand(String brand) {
this.brand = brand;
}
public String getColor() {
return color;
}
public void setColor(String color) {
this.color = color;
}
public int getYear() {
return year;
}
public void setYear(int year) {
this.year = year;
}
}
In the above code, we define a class named Car
with attributes (brand, color, and year), a constructor, and methods for starting the engine and driving. We also provide getters and setters for accessing and modifying the attributes.
Creating Objects and Using the Class
To create objects of the Car
class and use its methods and attributes, we can do the following:
Car myCar = new Car("Toyota", "Red", 2022);
myCar.startEngine();
myCar.drive();
System.out.println("Brand: " + myCar.getBrand());
System.out.println("Color: " + myCar.getColor());
System.out.println("Year: " + myCar.getYear());
In the above code, we create a new instance of the Car
class called myCar
and provide values for its attributes using the constructor. We then call the methods to start the engine and drive the car. Finally, we retrieve and print the values of the car's attributes using the getter methods.
Conclusion
Object-oriented programming is a powerful paradigm in Java that allows you to structure your code into modular and reusable components. In this article, we explored the basics of object-oriented programming, including encapsulation, inheritance, and polymorphism. We also provided an example of creating a class and using it to create objects and invoke methods. By understanding and applying object-oriented principles, you can design and develop more organized, maintainable, and extensible code. Continuously practice object-oriented programming and explore more advanced topics, such as abstraction, interfaces, and design patterns, to further enhance your Java programming skills.
4.5L
Learners
20+
Instructors
50+
Courses
6.0L
Course enrollments
Future Trending Courses
When selecting, a course, Here are a few areas that are expected to be in demand in the future:.
Future Learning for all
If you’re passionate and ready to dive in, we’d love to join 1:1 classes for you. We’re committed to support our learners and professionals their development and well-being.
View Courses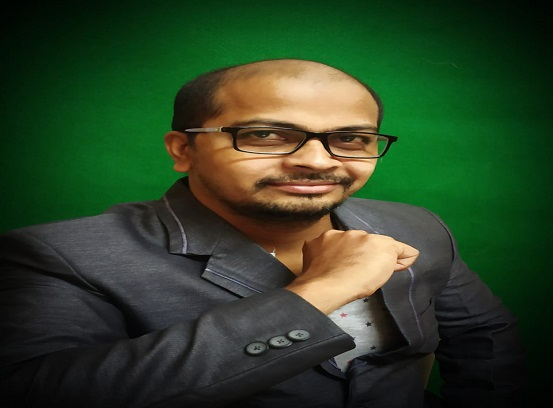
Most Popular Course topics
These are the most popular course topics among Software Courses for learners