Hi there, we’re Harisystems
"Unlock your potential and soar to new heights with our exclusive online courses! Ignite your passion, acquire valuable skills, and embrace limitless possibilities. Don't miss out on our limited-time sale - invest in yourself today and embark on a journey of personal and professional growth. Enroll now and shape your future with knowledge that lasts a lifetime!".
For corporate trainings, projects, and real world experience reach us. We believe that education should be accessible to all, regardless of geographical location or background.
1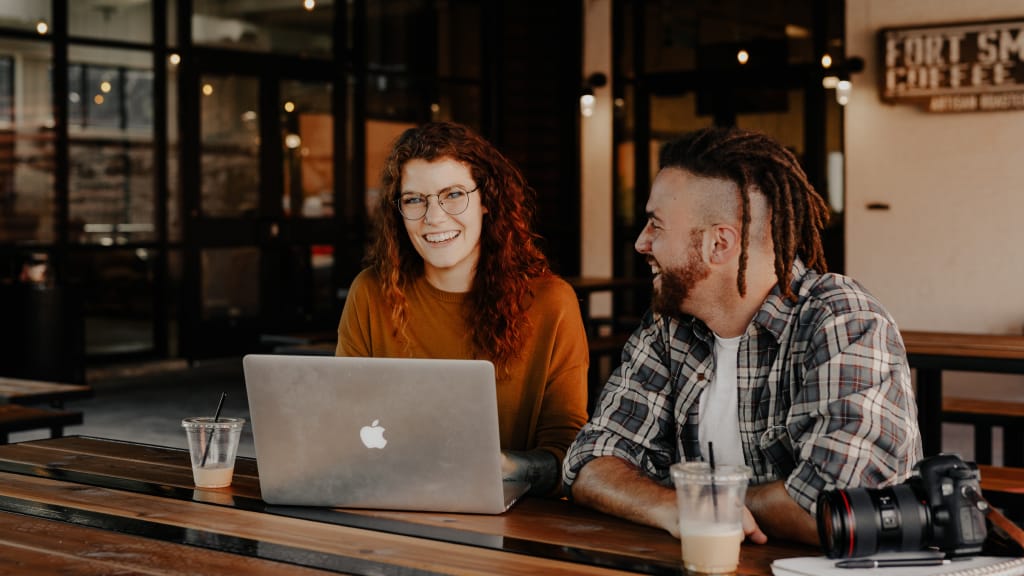
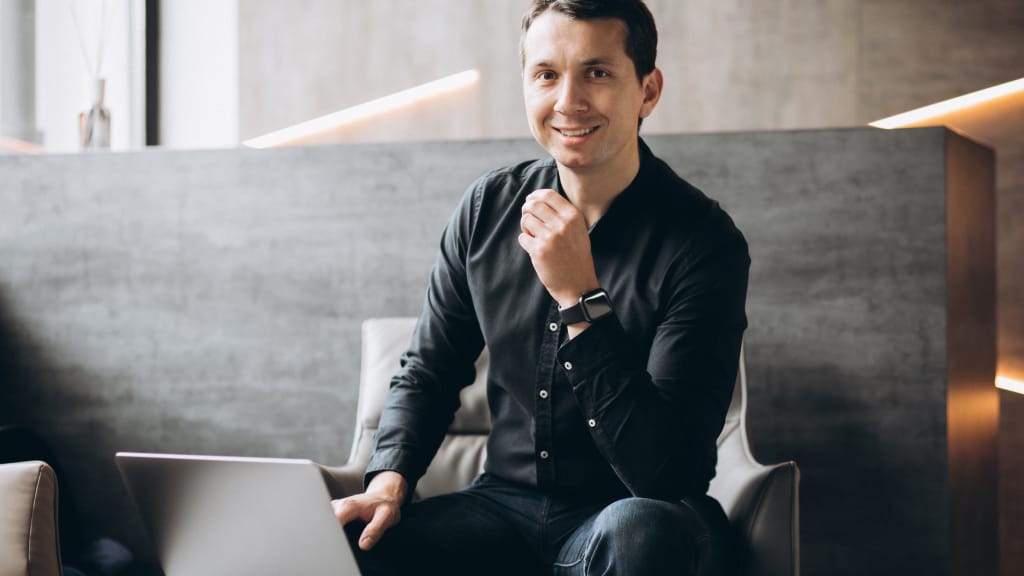
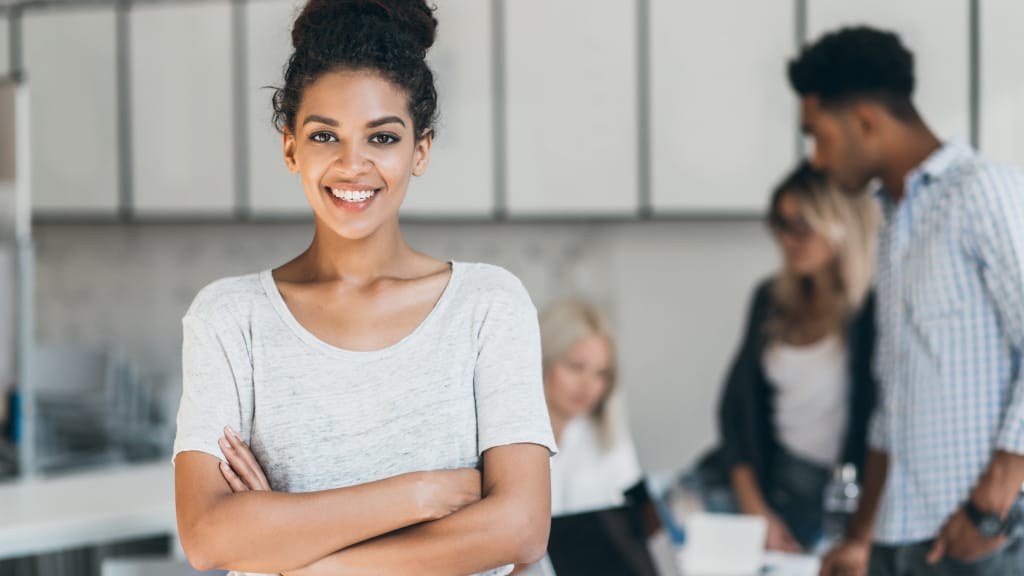
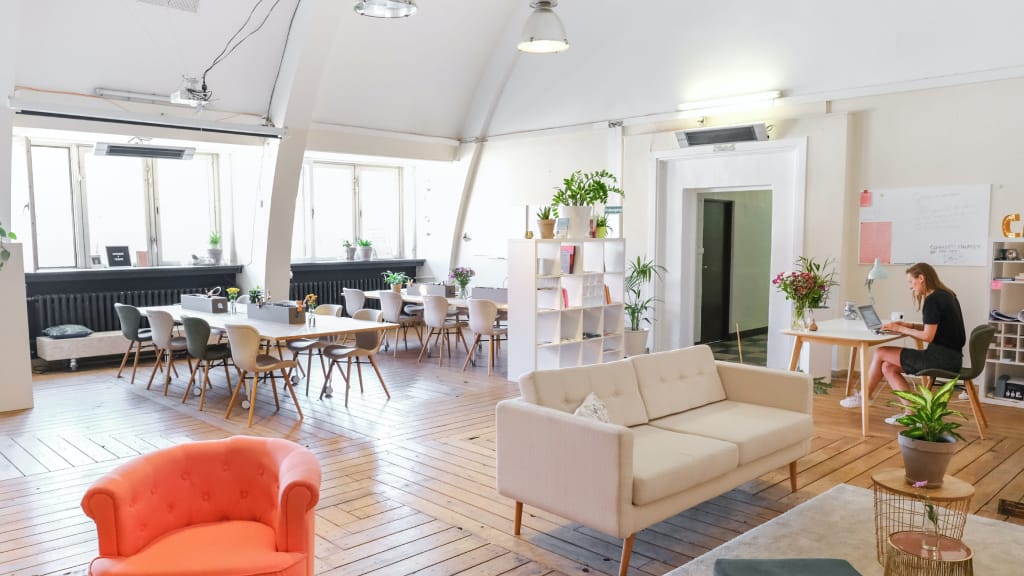
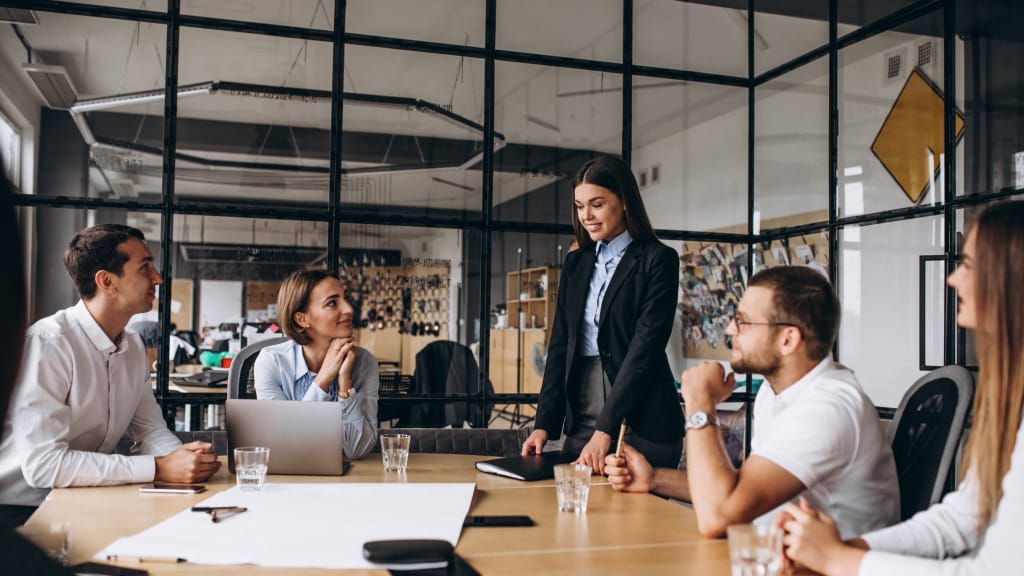
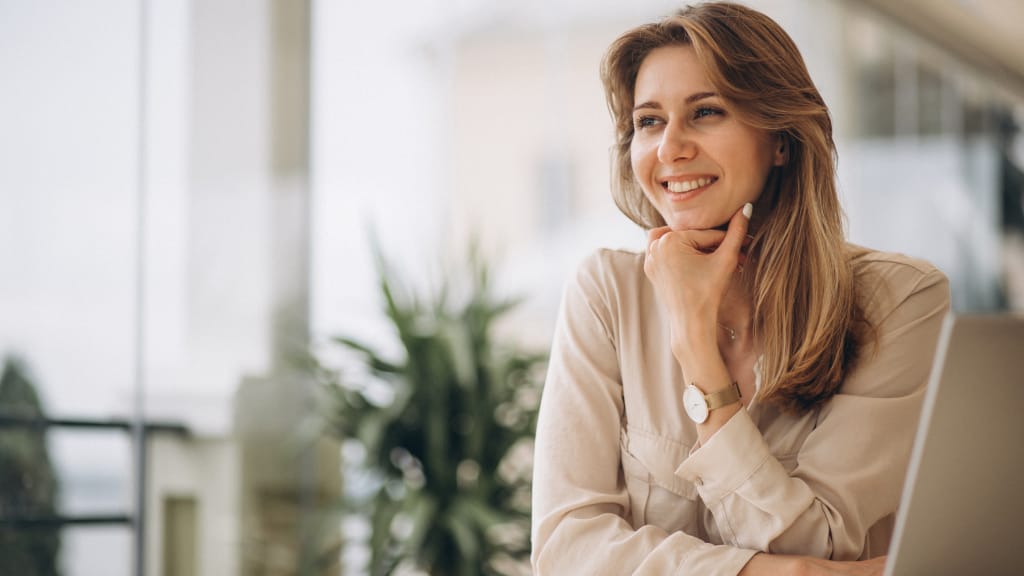
Exploring Modern JavaScript: Enhancing Web Development with ES6+
JavaScript has evolved significantly in recent years, introducing new features and enhancements that make web development more powerful and efficient. The introduction of ECMAScript 6 (ES6) and subsequent versions has transformed the way JavaScript code is written and organized. In this article, we will explore some of the modern JavaScript features and provide examples to showcase their capabilities.
1. Arrow Functions
Arrow functions provide a more concise syntax for writing functions. They have a shorter syntax compared to traditional function expressions and lexically bind the value of 'this'. Here's an example of an arrow function:
<script>
// Traditional Function
function greet(name) {
console.log('Hello, ' + name + '!');
}
greet('John'); // Output: Hello, John!
// Arrow Function
const greetArrow = (name) => {
console.log(`Hello, ${name}!`);
}
greetArrow('John'); // Output: Hello, John!
</script>
In this example, the 'greet' function and 'greetArrow' arrow function achieve the same functionality of greeting a person by name. Arrow functions provide a more concise and expressive way of defining functions.
2. Template Literals
Template literals, also known as template strings, allow for easy string interpolation and multiline strings. They are enclosed by backticks (`) instead of single or double quotes. Here's an example:
<script>
const name = 'John';
const age = 30;
// Traditional String Concatenation
const message = 'My name is ' + name + ' and I am ' + age + ' years old.';
console.log(message); // Output: My name is John and I am 30 years old.
// Template Literal
const messageTemplate = `My name is ${name} and I am ${age} years old.`;
console.log(messageTemplate); // Output: My name is John and I am 30 years old.
</script>
Template literals allow embedding expressions within the string using the '${}' syntax, making string interpolation more convenient and readable.
3. Destructuring Assignment
Destructuring assignment provides a concise way to extract values from arrays or objects and assign them to variables. It eliminates the need for manual assignment one by one. Here's an example:
<script>
// Destructuring an Array
const numbers = [1, 2, 3, 4, 5];
const [first, second, ...rest] = numbers;
console.log(first); // Output: 1
console.log(second); // Output: 2
console.log(rest); // Output: [3, 4, 5]
// Destructuring an Object
const person = {
name: 'John',
age: 30,
city: 'New York'
};
const { name, age, city } = person;
console.log(name); // Output: John
console.log(age); // Output: 30
console.log(city); // Output
: New York
</script>
Destructuring assignment simplifies the process of extracting values from arrays and objects, making the code more concise and readable.
4. Modules
Modules allow for better code organization and modularity by enabling the creation of separate files for different functionalities. Modules can export and import functions, objects, or values to be used in other modules. Here's an example:
<!-- File: greetings.js -->
<script type="module">
export function greet(name) {
console.log(`Hello, ${name}!`);
}
</script>
<!-- File: main.js -->
<script type="module">
import { greet } from './greetings.js';
greet('John'); // Output: Hello, John!
</script>
In this example, the 'greetings.js' module exports the 'greet' function, and the 'main.js' module imports and uses it. Modules enable better code organization, encapsulation, and reusability.
Conclusion
Modern JavaScript features like arrow functions, template literals, destructuring assignment, and modules have revolutionized the way developers write JavaScript code. These features enhance code readability, maintainability, and developer productivity. By leveraging these modern JavaScript features, developers can write more expressive and efficient code, ultimately leading to better web development experiences.
4.5L
Learners
20+
Instructors
50+
Courses
6.0L
Course enrollments
Future Trending Courses
When selecting, a course, Here are a few areas that are expected to be in demand in the future:.
Future Learning for all
If you’re passionate and ready to dive in, we’d love to join 1:1 classes for you. We’re committed to support our learners and professionals their development and well-being.
View Courses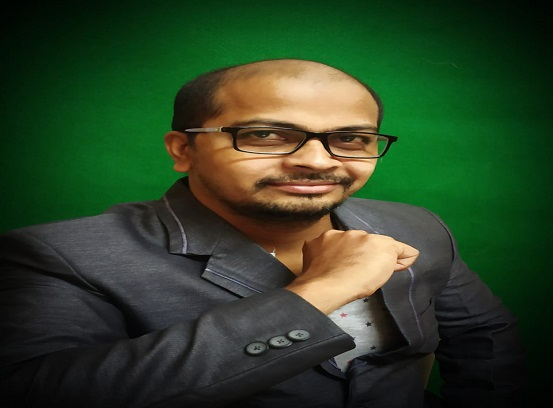
Most Popular Course topics
These are the most popular course topics among Software Courses for learners