Hi there, we’re Harisystems
"Unlock your potential and soar to new heights with our exclusive online courses! Ignite your passion, acquire valuable skills, and embrace limitless possibilities. Don't miss out on our limited-time sale - invest in yourself today and embark on a journey of personal and professional growth. Enroll now and shape your future with knowledge that lasts a lifetime!".
For corporate trainings, projects, and real world experience reach us. We believe that education should be accessible to all, regardless of geographical location or background.
1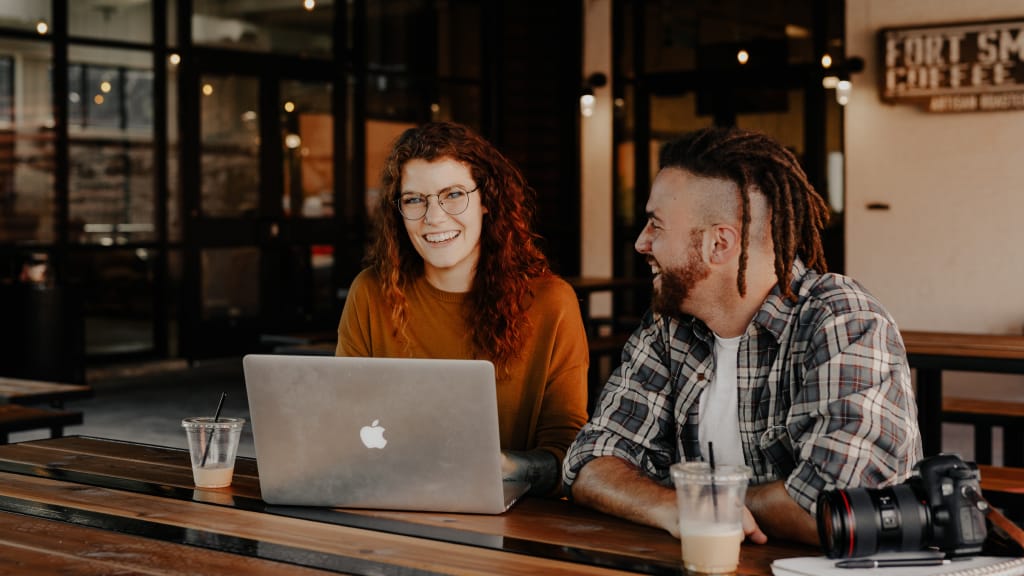
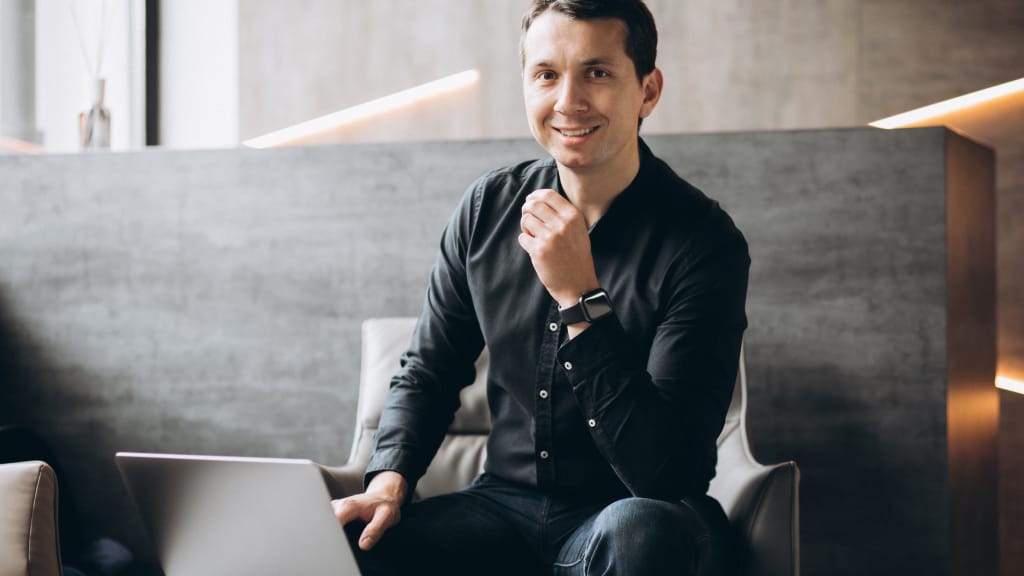
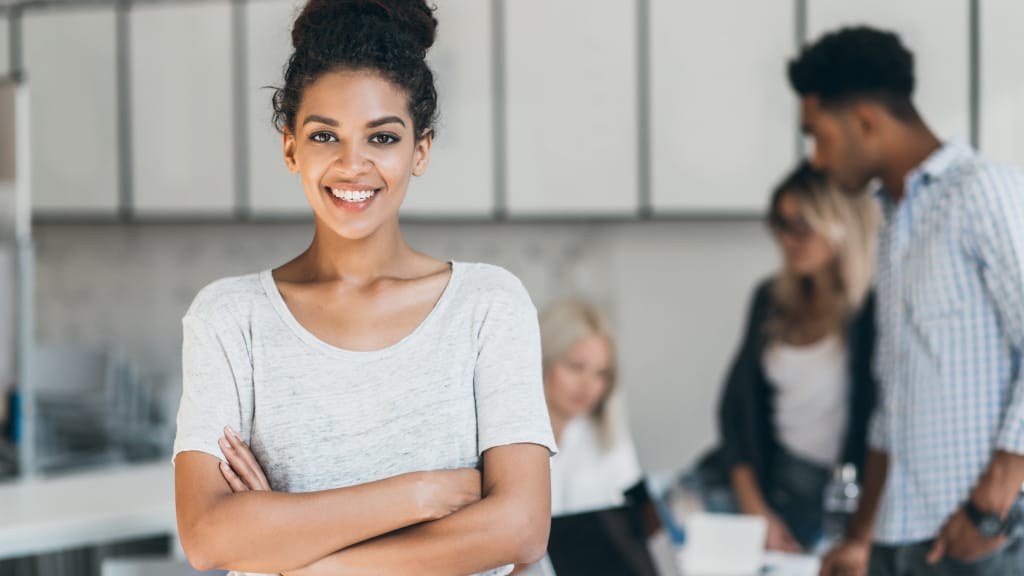
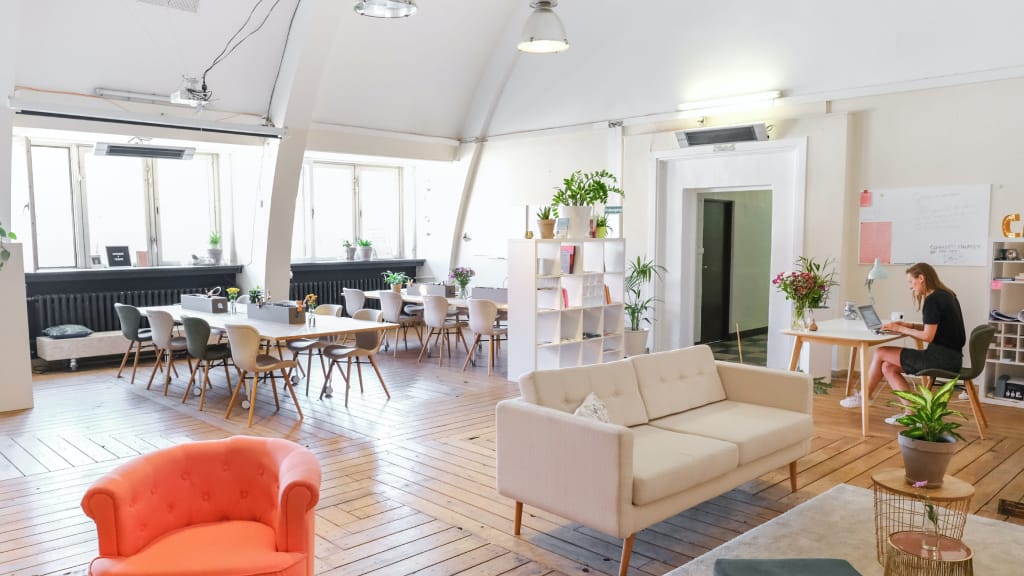
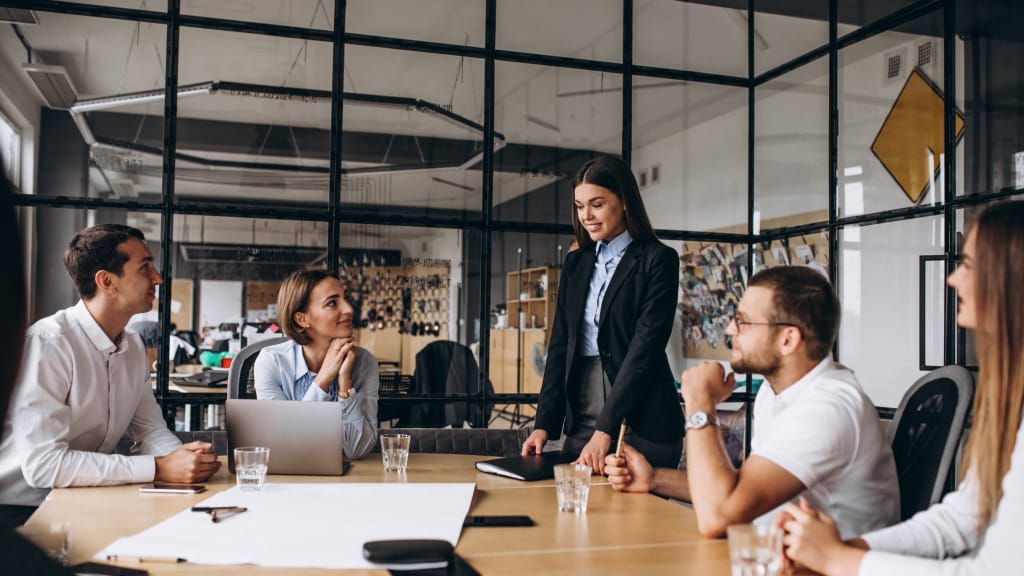
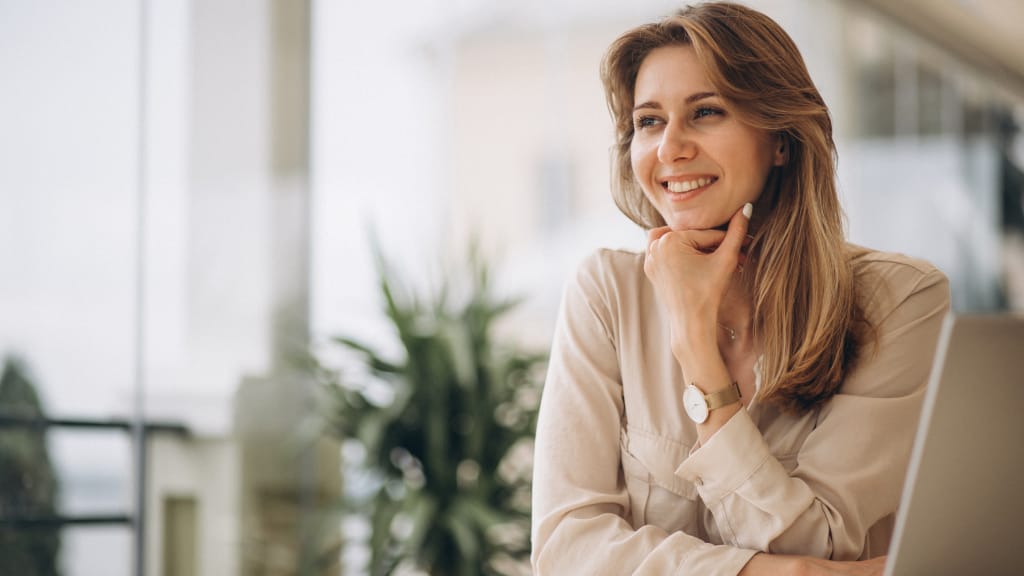
Python Project Source Code Examples
Python is a versatile programming language that allows you to develop a wide range of projects, from small scripts to complex applications. In this article, we'll showcase a few Python project source code examples to inspire you and demonstrate the possibilities of what you can build using Python.
Example 1: Simple Todo List
Let's start with a simple project - a command-line todo list application:
class TodoList:
def __init__(self):
self.tasks = []
def add_task(self, task):
self.tasks.append(task)
def display_tasks(self):
print("Tasks:")
for task in self.tasks:
print(task)
# Create a new todo list
todo_list = TodoList()
# Add tasks to the list
todo_list.add_task("Buy groceries")
todo_list.add_task("Finish homework")
todo_list.add_task("Go for a run")
# Display the tasks
todo_list.display_tasks()
In this code, we define a "TodoList" class that has methods for adding tasks to the list and displaying them. We create an instance of the class, add tasks to the list, and then display the tasks on the console.
Example 2: Web Scraping with Beautiful Soup
Web scraping is a popular use case for Python. Here's an example of using the Beautiful Soup library to extract data from a webpage:
import requests
from bs4 import BeautifulSoup
# Make a request to the webpage
response = requests.get("https://example.com")
# Create a BeautifulSoup object
soup = BeautifulSoup(response.text, "html.parser")
# Extract data from the webpage
title = soup.title.text
paragraphs = soup.find_all("p")
# Print the extracted data
print("Title:", title)
print("Paragraphs:")
for p in paragraphs:
print(p.text)
In this code, we use the requests library to make an HTTP request to a webpage and retrieve its content. We then create a BeautifulSoup object and use its methods to extract specific elements, such as the page title and paragraphs. Finally, we print the extracted data on the console.
Example 3: Flask Web Application
Flask is a popular web framework for building web applications in Python. Here's an example of a simple Flask application:
from flask import Flask
app = Flask(__name__)
@app.route("/")
def hello():
return "Hello, world!"
if __name__ == "__main__":
app.run()
In this code, we import the Flask module and create a Flask application. We define a route ("/") that maps to the "hello" function. When a user visits the root URL, the function is executed, and it returns the "Hello, world!" message. We run the application using the "app.run()" method.
Conclusion
Python offers countless possibilities for building projects, whether it's a simple command-line application, a web scraping script, or a full-fledged web application. The examples provided in this article should give you a glimpse of the various project types you can create using Python. Feel free to explore these examples, modify them, and expand upon them to create your unique projects!
4.5L
Learners
20+
Instructors
50+
Courses
6.0L
Course enrollments
Future Trending Courses
When selecting, a course, Here are a few areas that are expected to be in demand in the future:.
Future Learning for all
If you’re passionate and ready to dive in, we’d love to join 1:1 classes for you. We’re committed to support our learners and professionals their development and well-being.
View Courses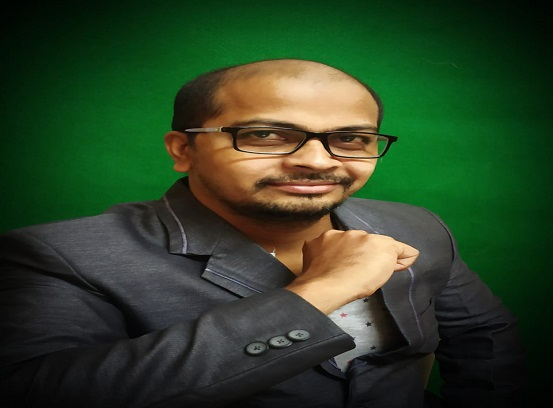
Most Popular Course topics
These are the most popular course topics among Software Courses for learners