Hi there, we’re Harisystems
"Unlock your potential and soar to new heights with our exclusive online courses! Ignite your passion, acquire valuable skills, and embrace limitless possibilities. Don't miss out on our limited-time sale - invest in yourself today and embark on a journey of personal and professional growth. Enroll now and shape your future with knowledge that lasts a lifetime!".
For corporate trainings, projects, and real world experience reach us. We believe that education should be accessible to all, regardless of geographical location or background.
1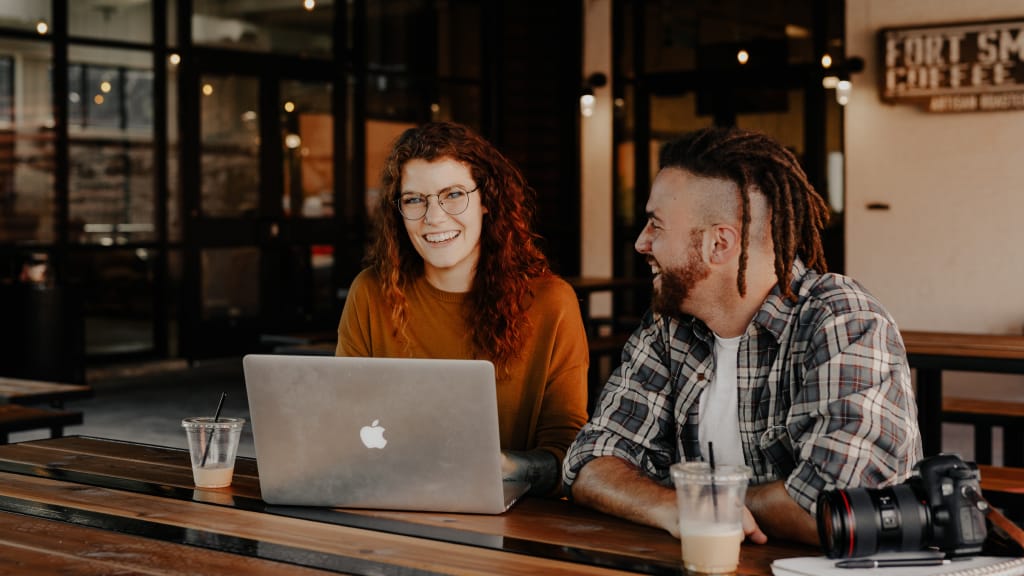
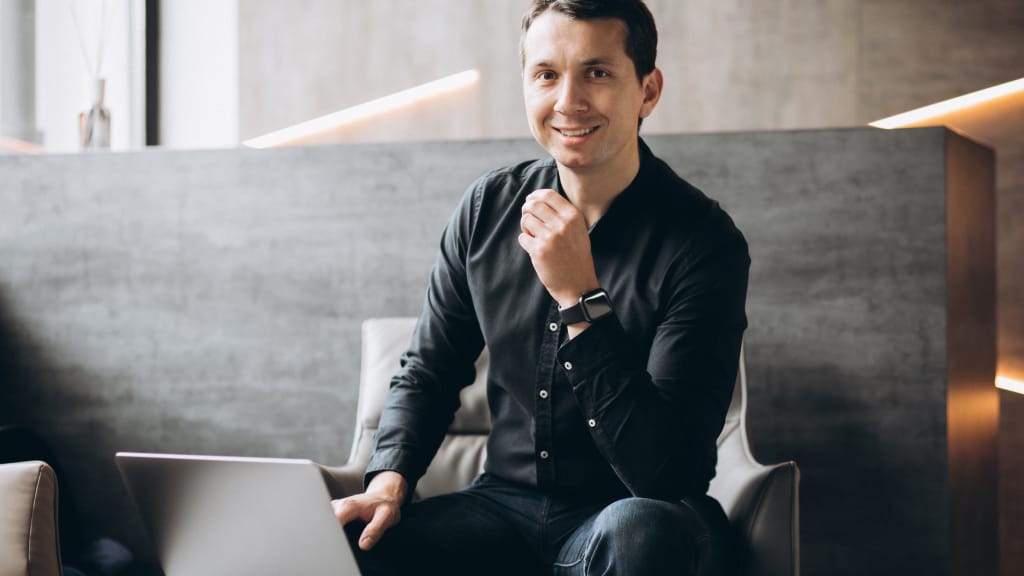
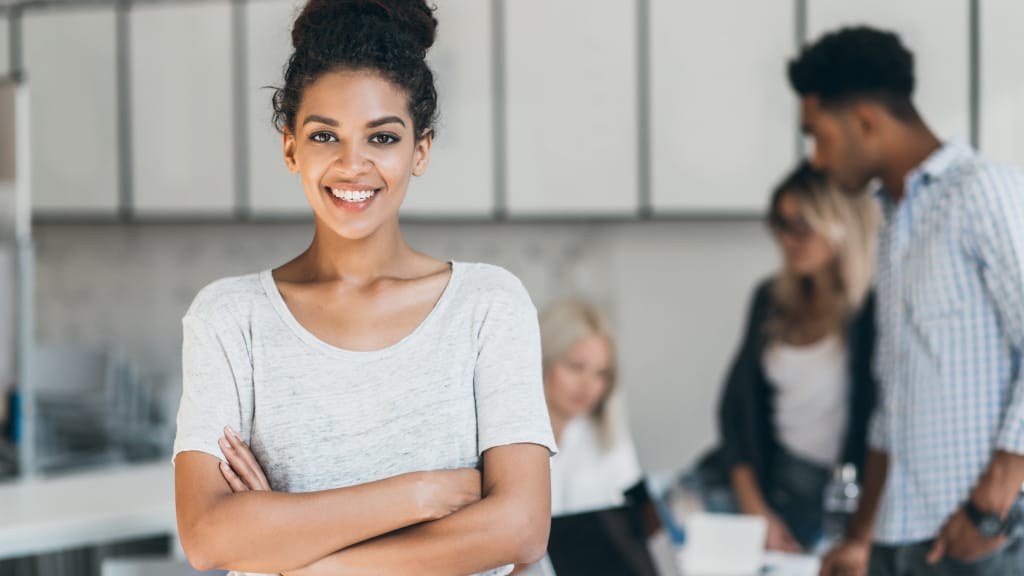
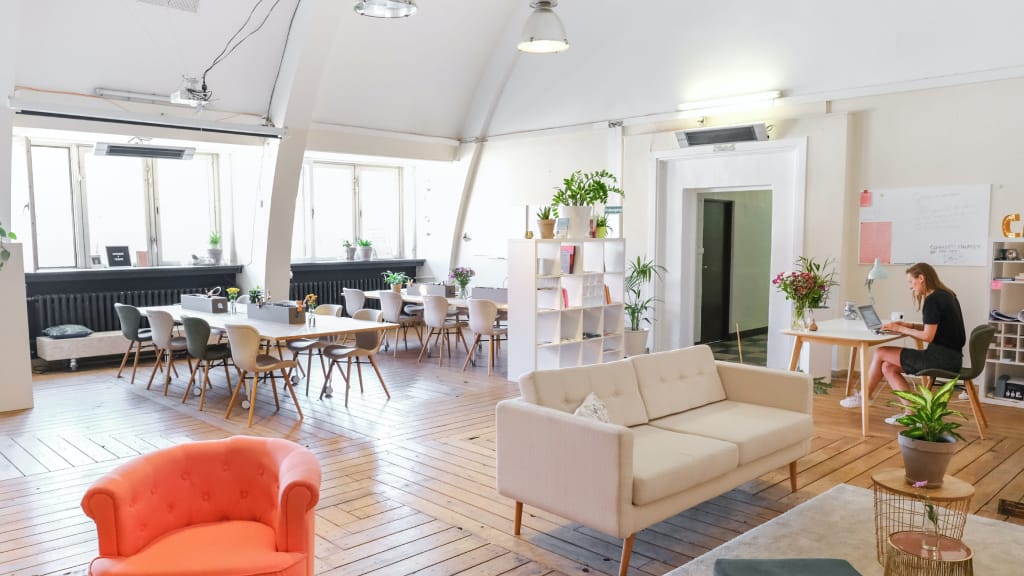
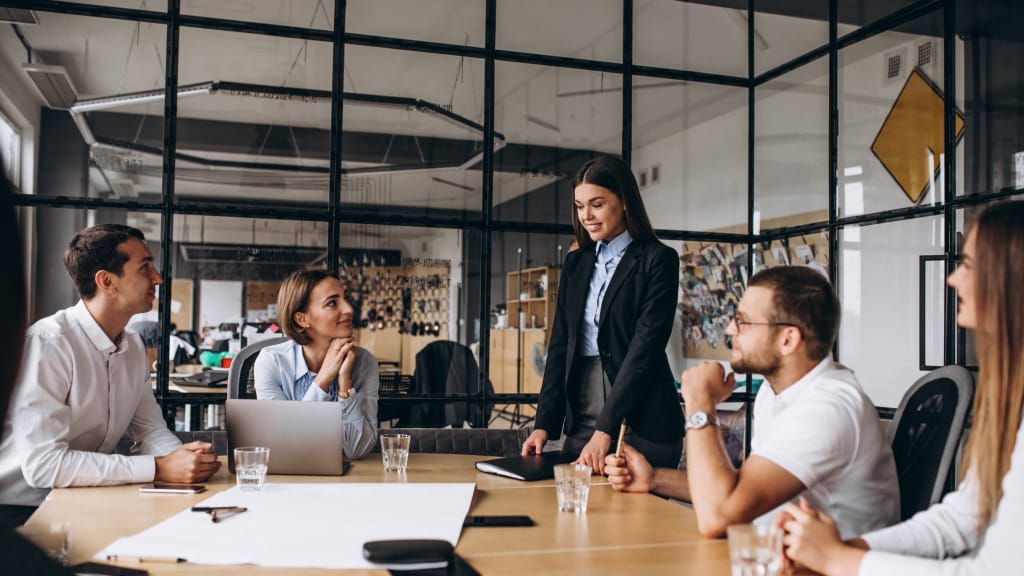
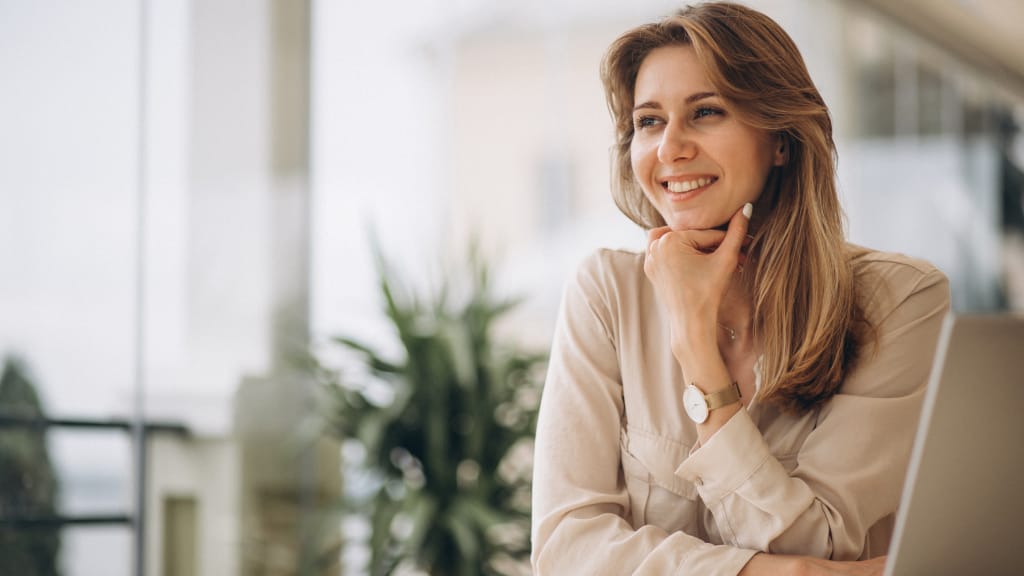
Java Object-Oriented Programming: Class Constructors
In Java, class constructors are special methods that are used to create and initialize objects of a class. Constructors are called automatically when an object is created and provide a way to set the initial state of the object. In this article, we will explore class constructors in Java and provide examples to help you understand their usage.
Defining Class Constructors
In Java, a constructor has the same name as the class and does not have a return type, not even void
. Here's an example:
public class MyClass {
// Attributes
// Default Constructor
public MyClass() {
// Constructor code
}
// Parameterized Constructor
public MyClass(String param1, int param2) {
// Constructor code
}
}
In the above code, we define a class named MyClass
with two constructors: a default constructor and a parameterized constructor. The default constructor takes no arguments, while the parameterized constructor takes two arguments of type String
and int
. The constructor code inside the curly braces is where you can initialize the attributes of the object or perform any other necessary setup.
Creating Objects and Using Constructors
To create objects from a class, you use the new
keyword followed by the class name and parentheses. Here's an example:
MyClass obj1 = new MyClass();
MyClass obj2 = new MyClass("Parameter", 123);
In the above code, we create two objects of the MyClass
class: obj1
and obj2
. The first object uses the default constructor, while the second object uses the parameterized constructor. The arguments passed to the constructor match the parameter types defined in the constructor.
Constructor Overloading
Similar to methods, constructors can be overloaded, which means you can define multiple constructors with different parameter lists. This allows you to create objects using different combinations of arguments. Here's an example:
public class MyClass {
// Attributes
// Default Constructor
public MyClass() {
// Constructor code
}
// Parameterized Constructor 1
public MyClass(String param1) {
// Constructor code
}
// Parameterized Constructor 2
public MyClass(int param2) {
// Constructor code
}
}
In the above code, we define three constructors in the MyClass
class: a default constructor, a parameterized constructor that takes a String
argument, and a parameterized constructor that takes an int
argument. Each constructor can have its own initialization logic or perform specific tasks based on the provided arguments.
Conclusion
Class constructors in Java are special methods used to create and initialize objects. In this article, we explored how to define and use class constructors, including the default constructor and parameterized constructors. We also discussed constructor overloading, which allows you to define multiple constructors with different parameter lists. By effectively utilizing constructors, you can ensure that objects are properly initialized and set their initial state. Continuously practice working with constructors and explore more advanced topics, such as constructor chaining and the use of constructors in inheritance, to further enhance your object-oriented programming skills in Java.
4.5L
Learners
20+
Instructors
50+
Courses
6.0L
Course enrollments
Future Trending Courses
When selecting, a course, Here are a few areas that are expected to be in demand in the future:.
Future Learning for all
If you’re passionate and ready to dive in, we’d love to join 1:1 classes for you. We’re committed to support our learners and professionals their development and well-being.
View Courses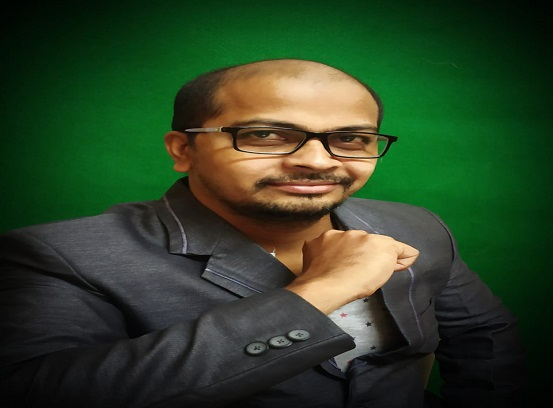
Most Popular Course topics
These are the most popular course topics among Software Courses for learners