Hi there, we’re Harisystems
"Unlock your potential and soar to new heights with our exclusive online courses! Ignite your passion, acquire valuable skills, and embrace limitless possibilities. Don't miss out on our limited-time sale - invest in yourself today and embark on a journey of personal and professional growth. Enroll now and shape your future with knowledge that lasts a lifetime!".
For corporate trainings, projects, and real world experience reach us. We believe that education should be accessible to all, regardless of geographical location or background.
1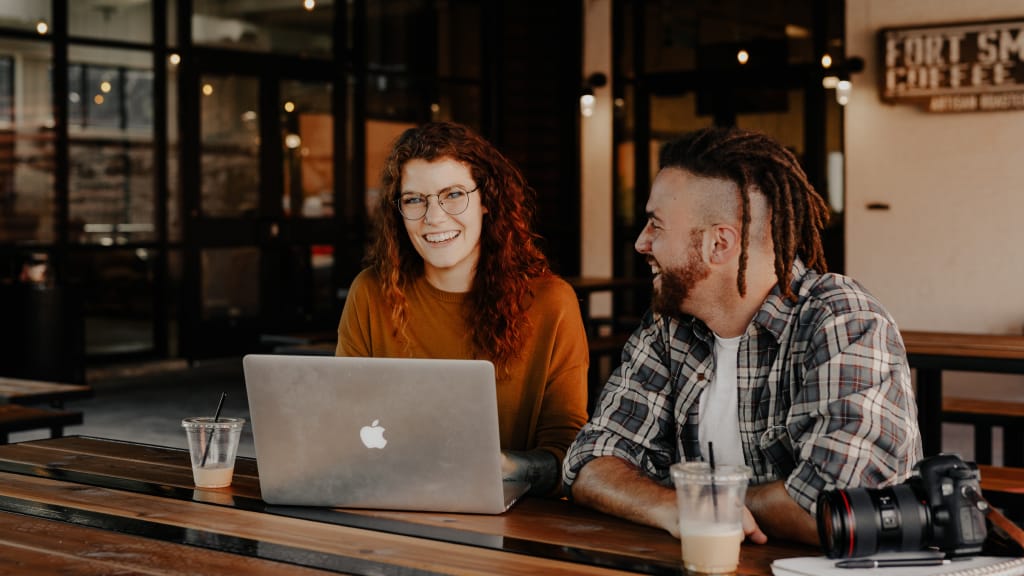
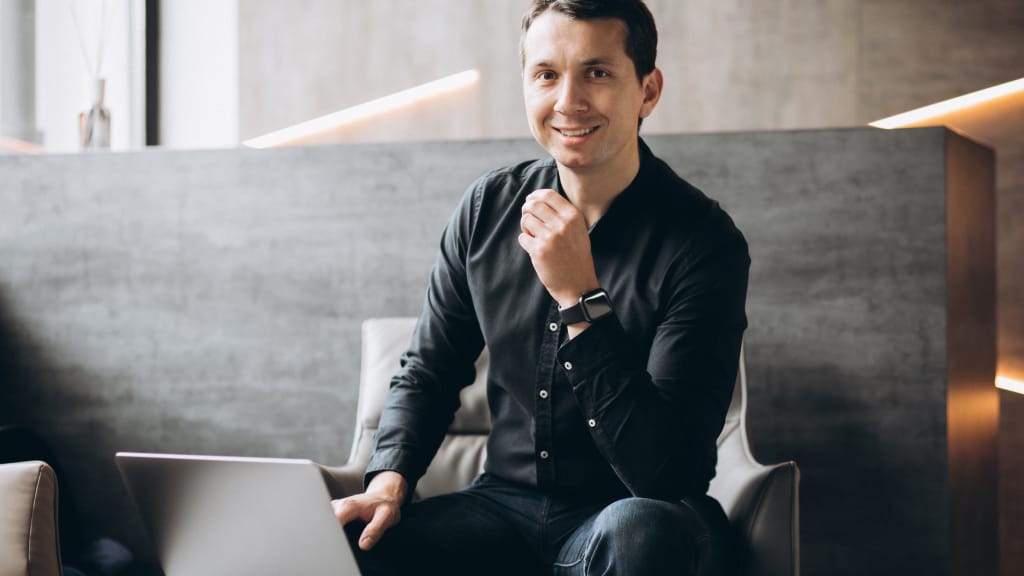
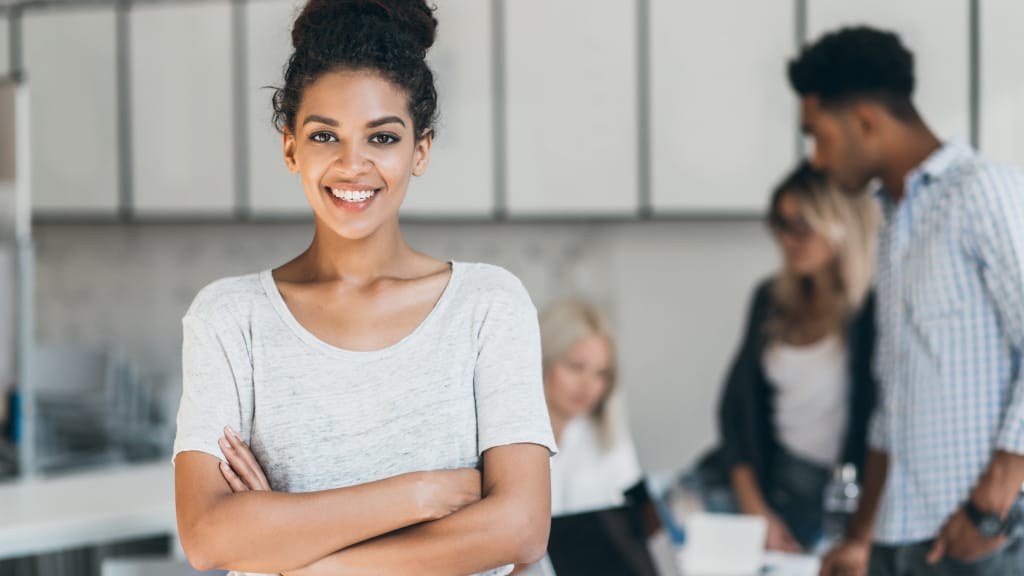
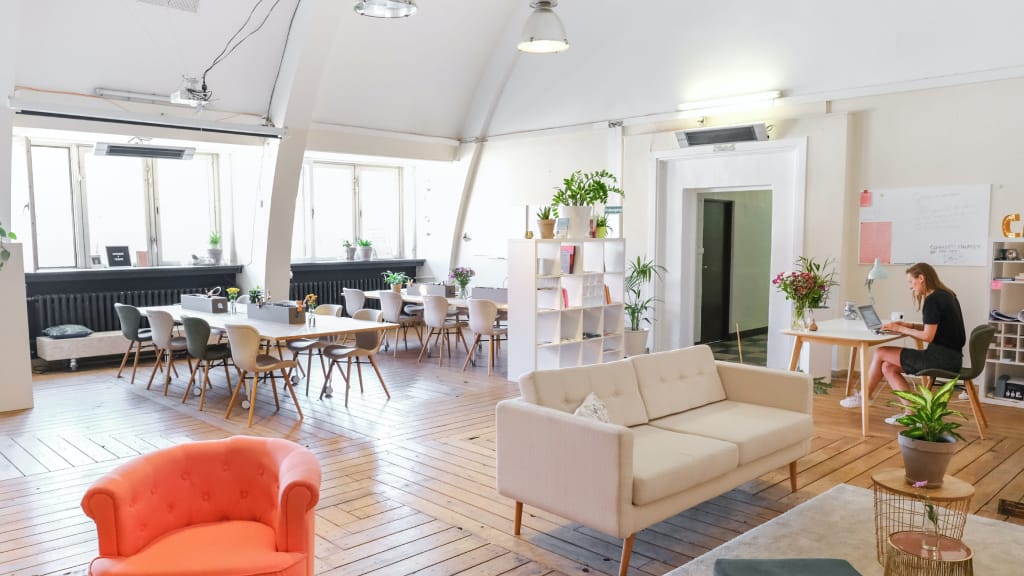
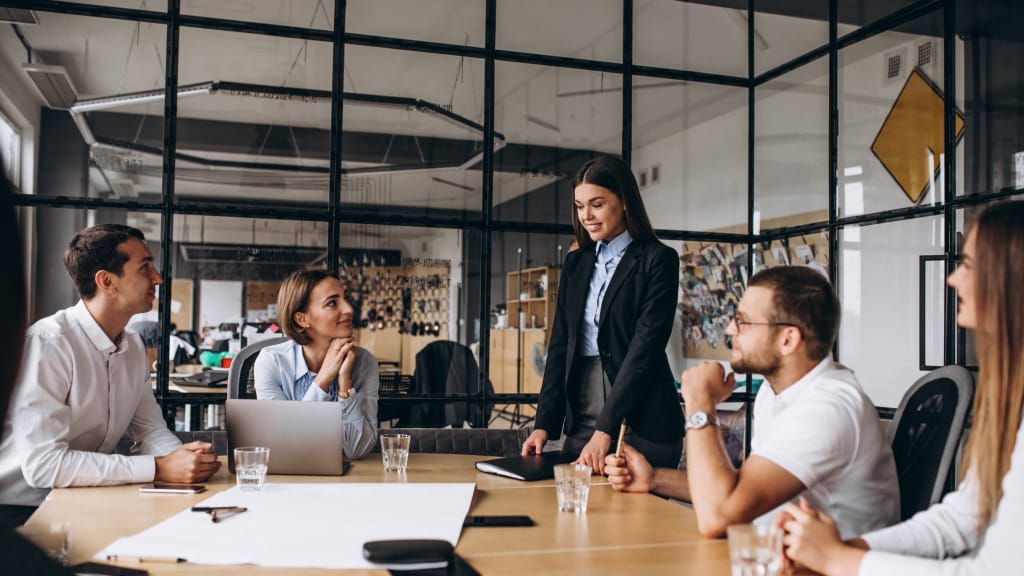
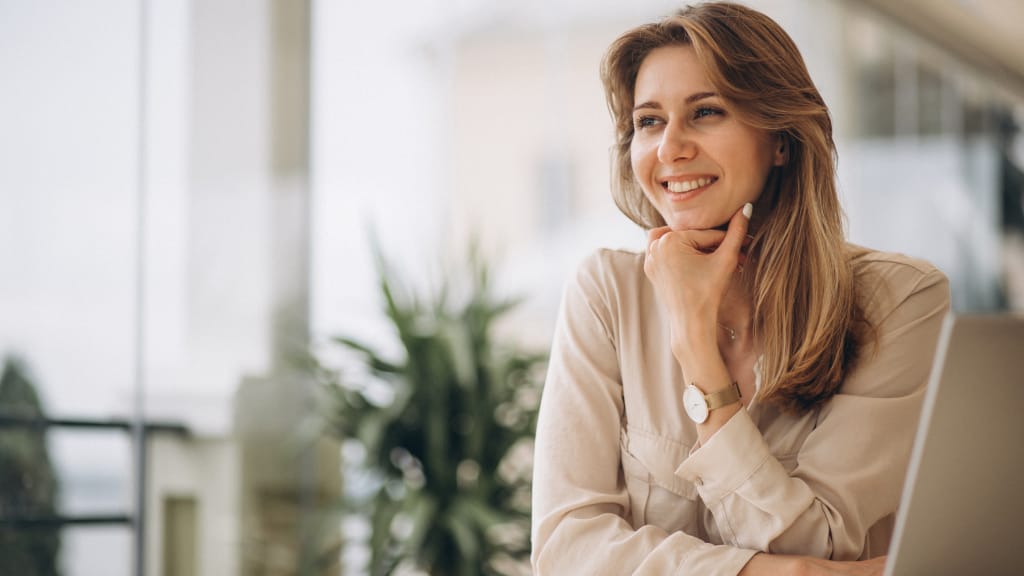
Java Recursion: Solving Problems by Calling Yourself
In Java, recursion is a powerful technique that allows a method to call itself to solve a problem. Recursion provides an elegant and efficient way to solve complex problems by breaking them down into smaller, manageable subproblems. In this article, we will explore recursion in Java and provide examples to help you understand its usage.
The Concept of Recursion
Recursion is a programming technique where a method calls itself to solve a smaller instance of the same problem. It involves two key elements: the base case and the recursive case. The base case is the terminating condition that indicates when the recursion should stop. The recursive case is the part where the method calls itself with a smaller or simpler input to make progress towards the base case.
Recursion follows a divide-and-conquer approach, where a complex problem is divided into smaller subproblems until they reach the base case, which is usually a simple and trivial case. By solving the subproblems and combining their results, the original problem is eventually solved.
Example: Factorial Calculation
Let's consider an example of calculating the factorial of a number using recursion. The factorial of a non-negative integer n
is the product of all positive integers less than or equal to n
. Here's a recursive implementation of the factorial calculation:
public int factorial(int n) {
if (n == 0 || n == 1) {
return 1; // Base case: factorial of 0 or 1 is 1
} else {
return n * factorial(n - 1); // Recursive case: multiply n with factorial of (n - 1)
}
}
In the above code, the factorial
method takes an integer n
as input. If n
is 0 or 1, the method returns 1, which is the base case. Otherwise, the method calls itself with n - 1
as the input and multiplies the result by n
, which is the recursive case.
To calculate the factorial of a number, you can call the factorial
method as follows:
int result = factorial(5);
System.out.println("Factorial of 5: " + result);
The output of the above code will be:
Factorial of 5: 120
The factorial of 5 is calculated by multiplying 5 with the factorial of 4, which is further calculated by multiplying 4 with the factorial of 3, and so on, until the base case is reached.
Example: Fibonacci Sequence
Another classic example of recursion is computing the Fibonacci sequence, where each number in the sequence is the sum of the two preceding numbers. Here's a recursive implementation of the Fibonacci sequence:
public int fibonacci(int n) {
if (n == 0) {
return 0; // Base case: Fibonacci of 0 is 0
} else if (n == 1) {
return 1; // Base case: Fibonacci of 1 is 1
} else {
return fibonacci(n - 1) + fibonacci(n - 2); // Recursive case: sum of the previous two Fibonacci numbers
}
}
In the above code, the fibonacci
method takes an integer n
as input. If n
is 0 or 1, the method returns 0 or 1, respectively, which are the base cases. Otherwise, the method calls itself recursively with n - 1
and n - 2
as inputs and returns the sum of the two results, which is the recursive case.
To compute a Fibonacci number, you can call the fibonacci
method as follows:
int result = fibonacci(6);
System.out.println("Fibonacci of 6: " + result);
The output of the above code will be:
Fibonacci of 6: 8
The Fibonacci sequence up to the 6th number is 0, 1, 1, 2, 3, 5, 8. The recursive calls calculate the sum of the previous two Fibonacci numbers until the base cases are reached.
Conclusion
Recursion is a powerful technique in Java that allows a method to call itself to solve a problem. In this article, we explored the concept of recursion, including the base case and the recursive case. We provided examples of calculating the factorial and computing the Fibonacci sequence using recursion. By understanding and effectively utilizing recursion, you can solve complex problems in a more concise and elegant manner. However, it's important to ensure that recursive methods have appropriate base cases to prevent infinite recursion. Continuously practice using recursion and explore more advanced topics, such as tail recursion and memoization, to further enhance your problem-solving capabilities in Java programming.
4.5L
Learners
20+
Instructors
50+
Courses
6.0L
Course enrollments
Future Trending Courses
When selecting, a course, Here are a few areas that are expected to be in demand in the future:.
Future Learning for all
If you’re passionate and ready to dive in, we’d love to join 1:1 classes for you. We’re committed to support our learners and professionals their development and well-being.
View Courses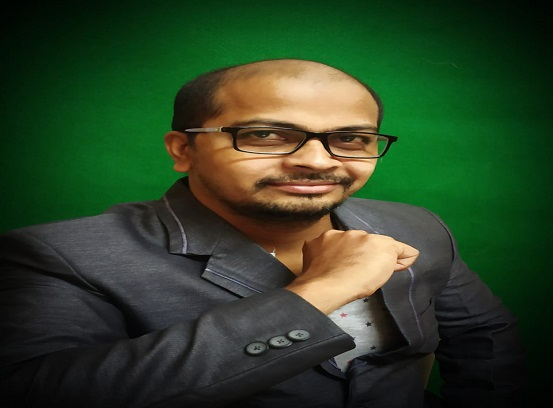
Most Popular Course topics
These are the most popular course topics among Software Courses for learners