Hi there, we’re Harisystems
"Unlock your potential and soar to new heights with our exclusive online courses! Ignite your passion, acquire valuable skills, and embrace limitless possibilities. Don't miss out on our limited-time sale - invest in yourself today and embark on a journey of personal and professional growth. Enroll now and shape your future with knowledge that lasts a lifetime!".
For corporate trainings, projects, and real world experience reach us. We believe that education should be accessible to all, regardless of geographical location or background.
1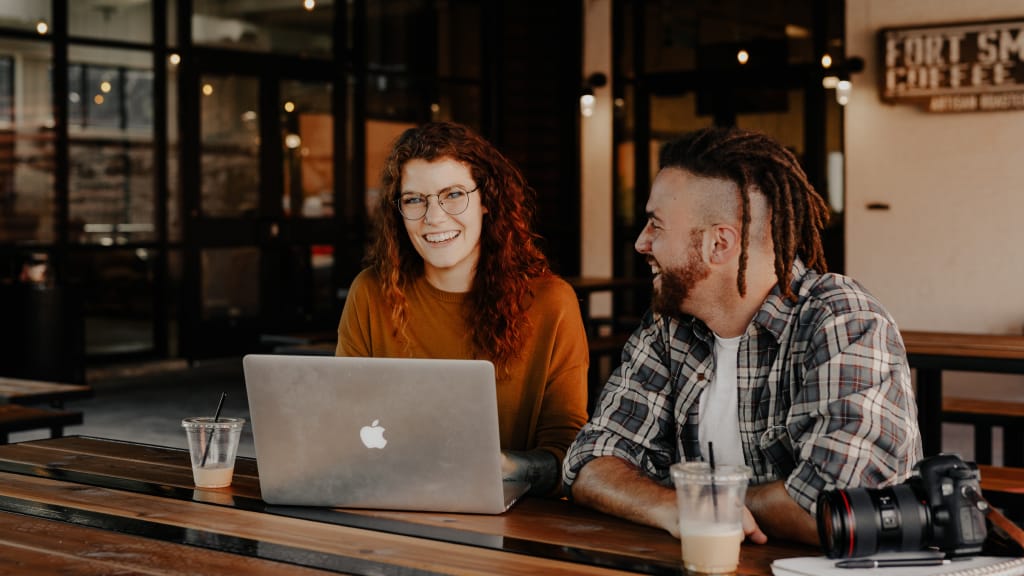
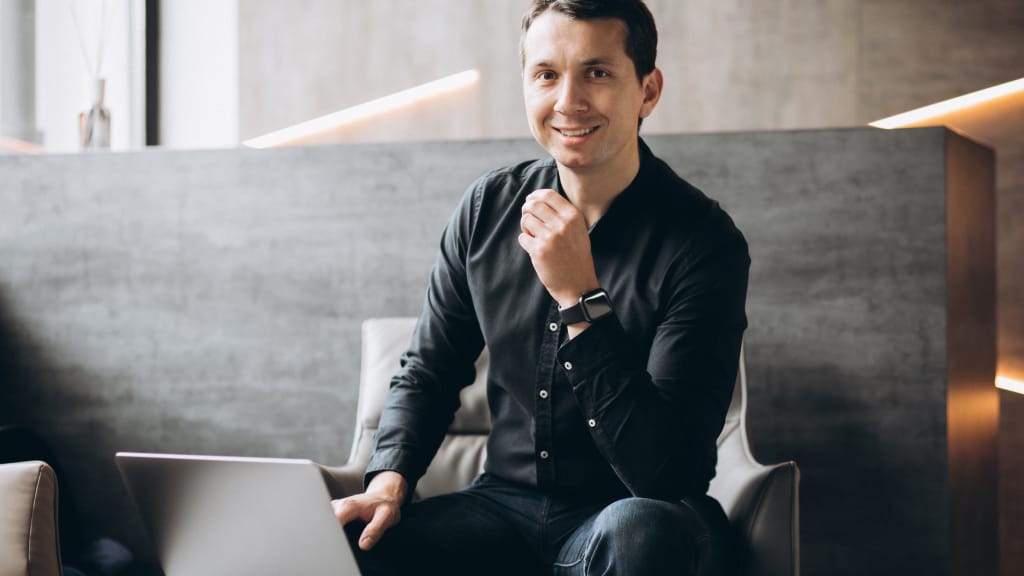
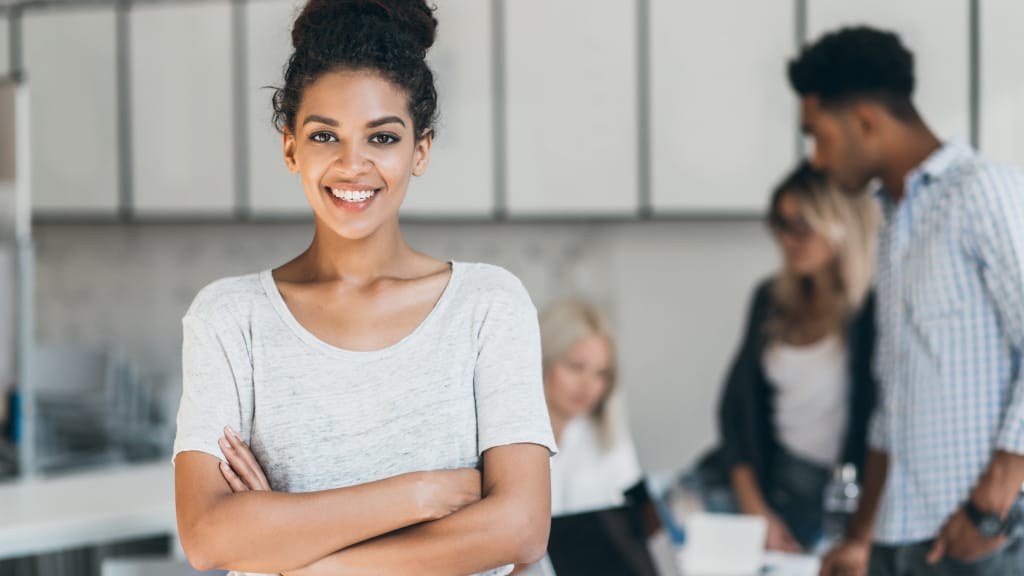
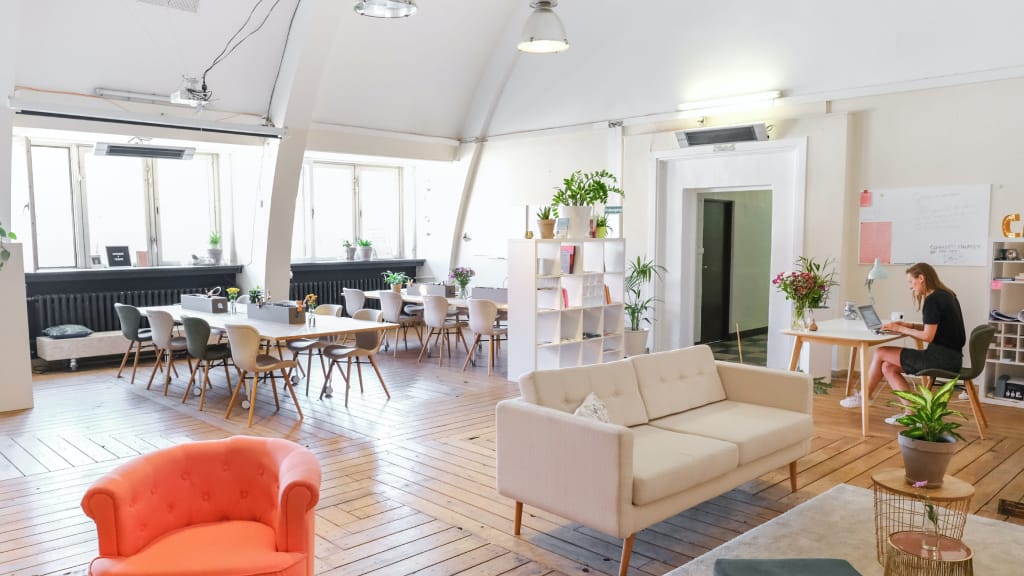
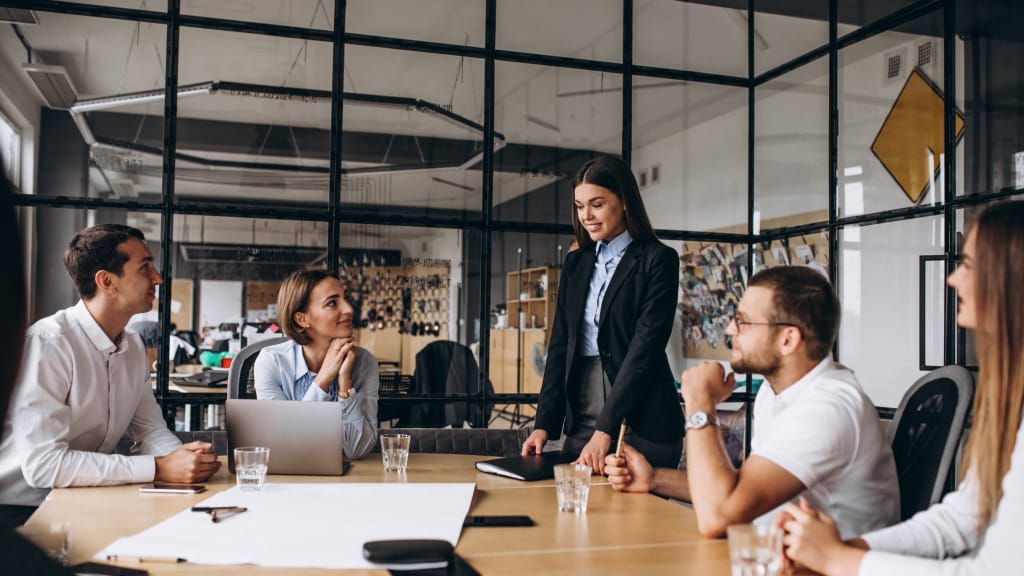
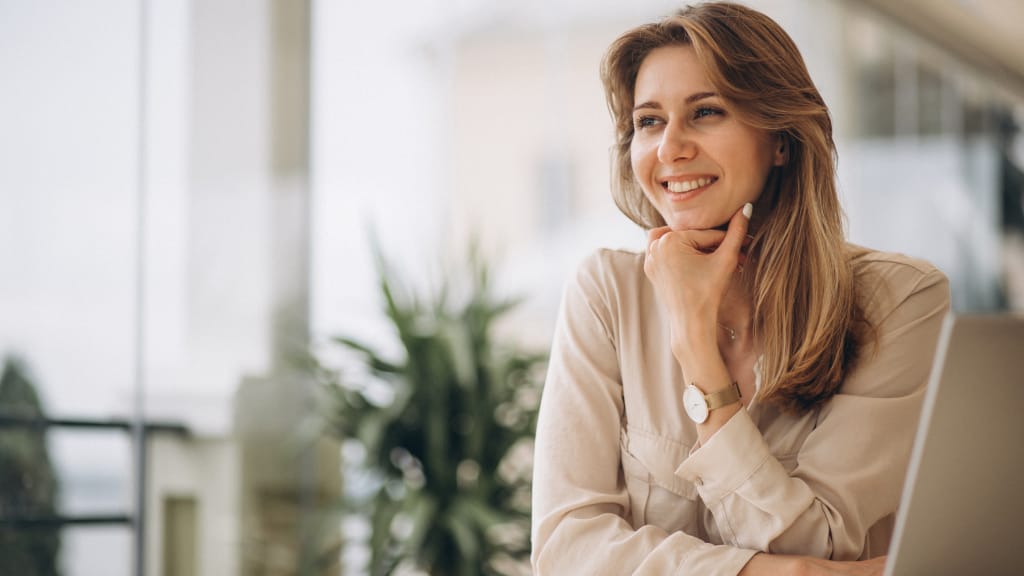
Java Data Types: Understanding Variable Types
In Java, data types define the type of data that can be stored in a variable. Each data type has its own set of values and operations that can be performed on it. In this article, we will explore the common data types in Java and provide examples to help you understand their usage.
Primitive Data Types
Java has eight primitive data types:
- byte: Used to store small integer values. Range: -128 to 127.
- short: Used to store small integer values. Range: -32,768 to 32,767.
- int: Used to store integer values. Range: -2,147,483,648 to 2,147,483,647.
- long: Used to store large integer values. Range: -9,223,372,036,854,775,808 to 9,223,372,036,854,775,807.
- float: Used to store floating-point values. Example: 3.14f.
- double: Used to store floating-point values with higher precision. Example: 3.14159.
- boolean: Used to store true/false values.
- char: Used to store single characters. Example: 'A', '$'.
Here's an example that demonstrates the declaration and initialization of variables with primitive data types:
int age = 25;
double salary = 50000.5;
boolean isEmployed = true;
char grade = 'A';
Reference Data Types
In addition to primitive data types, Java also has reference data types. These data types refer to objects in memory. Examples of reference data types include:
- String: Used to store sequences of characters. Example: "Hello, World!".
- Array: Used to store a collection of elements of the same type.
- Class: Used to define custom data types.
Here's an example that demonstrates the declaration and initialization of variables with reference data types:
String name = "John Smith";
int[] numbers = {1, 2, 3, 4, 5};
Person person = new Person("Alice", 30);
In the above example, name
is a variable of type String
that stores a sequence of characters, numbers
is an array of type int
that stores a collection of integers, and person
is a variable of type Person
that refers to an instance of the Person
class.
Casting
Java also allows you to perform type casting, which is the process of converting a value from one data type to another. There are two types of casting:
- Widening Casting (Implicit): It occurs automatically when converting a smaller type to a larger type. Example: converting an
int
to adouble
. - Narrowing Casting (Explicit): It requires explicit type casting and can lead to loss of data. Example: converting a
double
to anint
.
Here's an example that demonstrates type casting:
int x = 10;
double y = x; // Widening casting
double z = 12.34;
int w = (int) z; // Narrowing casting
Conclusion
Understanding data types is crucial for effective Java programming. In this article, we explored the primitive and reference data types in Java, including examples of their usage. We also discussed type casting and the concepts of widening and narrowing casting. By mastering data types, you can ensure proper data storage and manipulation in your Java programs. Continuously practice using different data types and explore more advanced topics, such as arrays and custom classes, to strengthen your Java programming skills.
4.5L
Learners
20+
Instructors
50+
Courses
6.0L
Course enrollments
Future Trending Courses
When selecting, a course, Here are a few areas that are expected to be in demand in the future:.
Future Learning for all
If you’re passionate and ready to dive in, we’d love to join 1:1 classes for you. We’re committed to support our learners and professionals their development and well-being.
View Courses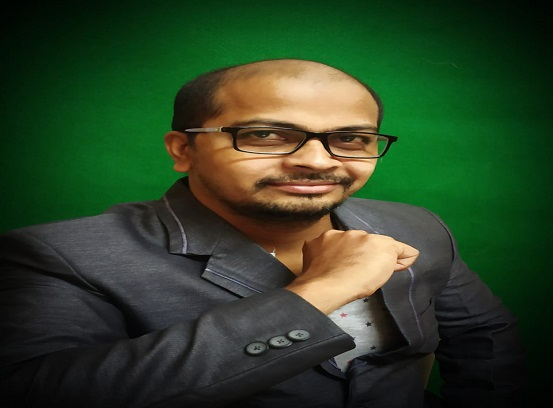
Most Popular Course topics
These are the most popular course topics among Software Courses for learners