Hi there, we’re Harisystems
"Unlock your potential and soar to new heights with our exclusive online courses! Ignite your passion, acquire valuable skills, and embrace limitless possibilities. Don't miss out on our limited-time sale - invest in yourself today and embark on a journey of personal and professional growth. Enroll now and shape your future with knowledge that lasts a lifetime!".
For corporate trainings, projects, and real world experience reach us. We believe that education should be accessible to all, regardless of geographical location or background.
1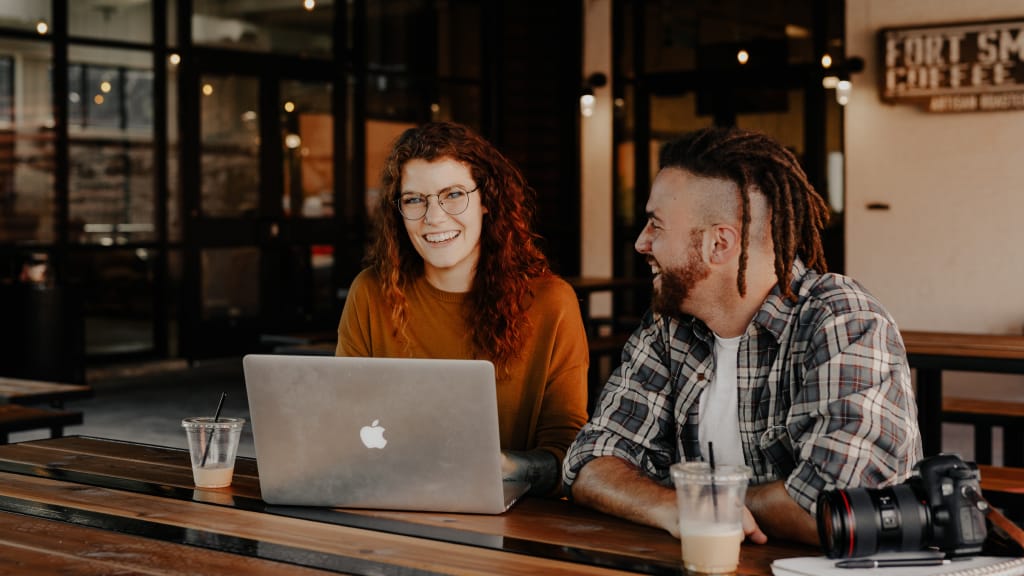
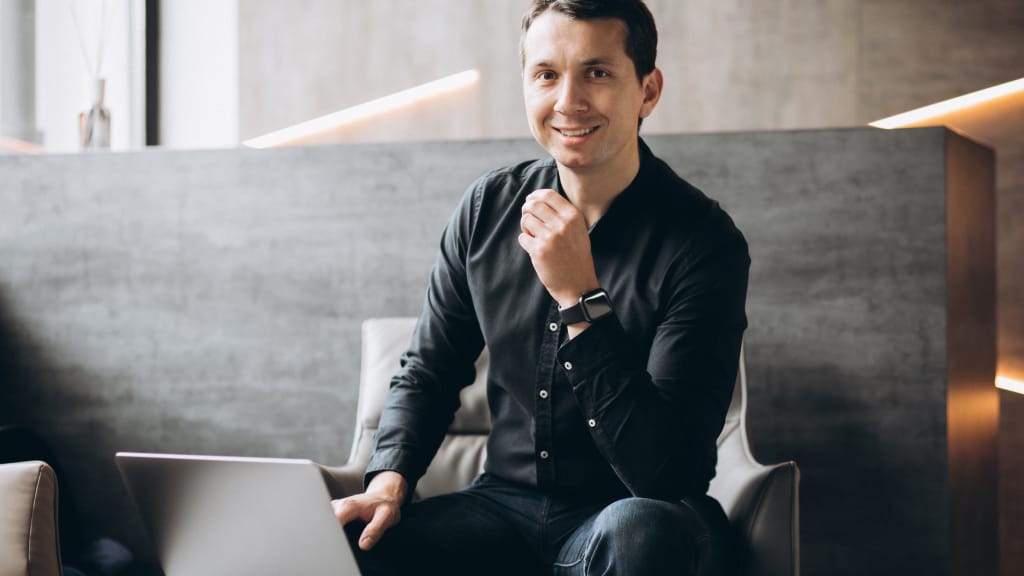
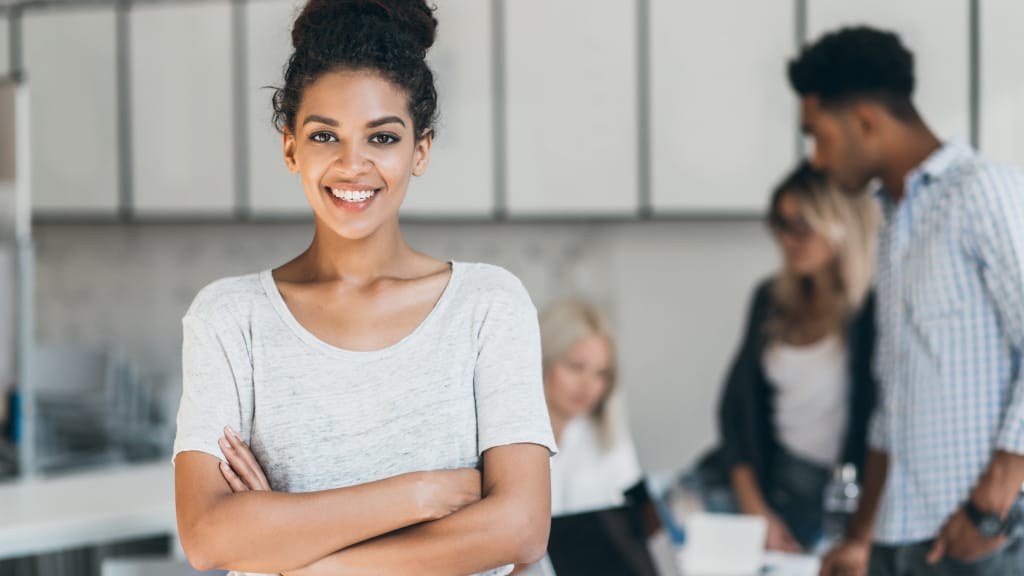
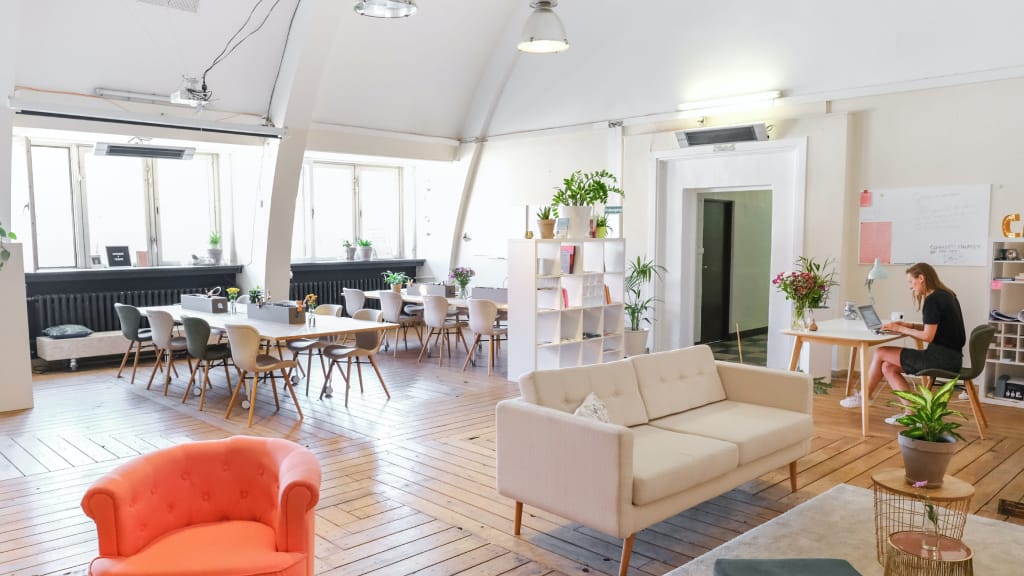
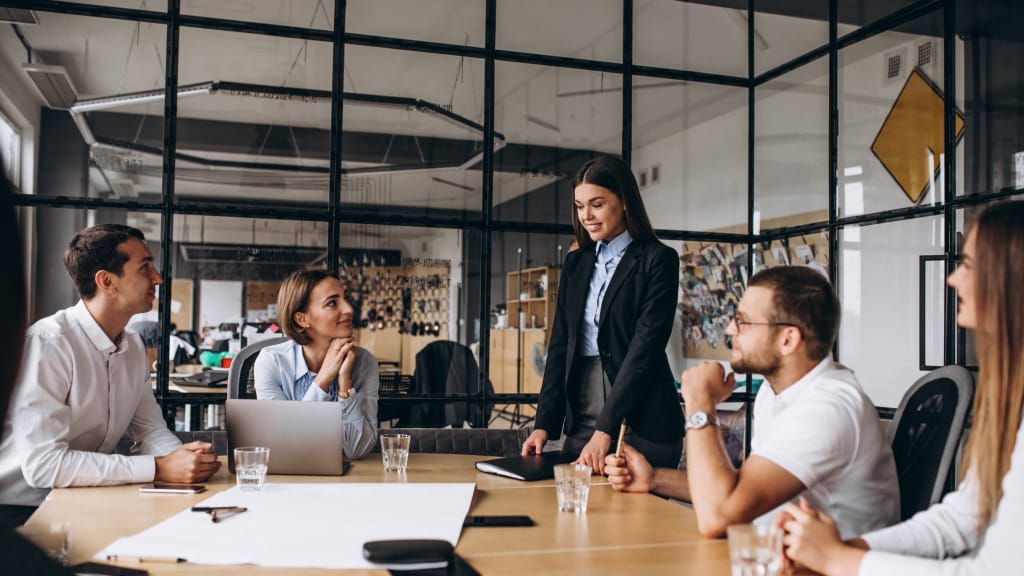
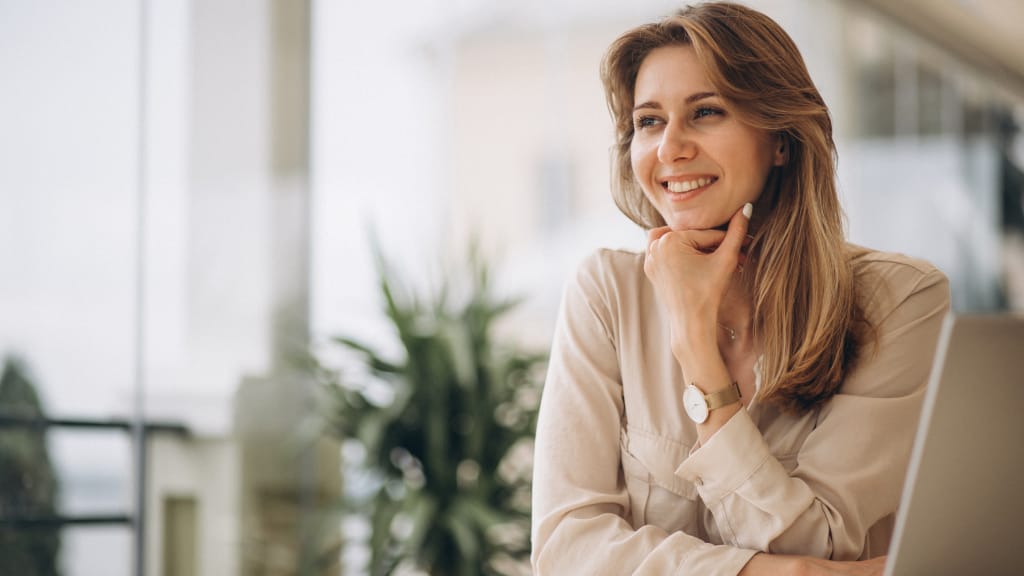
Django Insert Data: Saving Data to the Database
Introduction
In Django, inserting data into the database is a fundamental task when building web applications. Django provides a powerful database API that allows you to easily save data to your database tables. In this guide, we will explore how to insert data into the database using Django with examples.
Step 1: Creating a Django Project
Before we dive into inserting data, make sure you have a Django project set up. If you haven't created a Django project yet, you can refer to the previous guide on creating a Django project.
Step 2: Defining a Model
To insert data into the database, you need to have a corresponding model defined. Let's assume we have a model called Product
that represents a product entity. Here's an example:
from django.db import models
class Product(models.Model):
name = models.CharField(max_length=200)
price = models.DecimalField(max_digits=8, decimal_places=2)
description = models.TextField()
In this example, we define a Product
model with three fields: name
, price
, and description
. The CharField
represents a string field, the DecimalField
represents a decimal number, and the TextField
represents a large text field.
Step 3: Saving Data
To save data to the database, you create an instance of the model, set the field values, and call the save()
method. Here's an example:
product = Product()
product.name = 'Example Product'
product.price = 9.99
product.description = 'This is an example product description.'
product.save()
In this example, we create a new instance of the Product
model, set the field values, and save it to the database using the save()
method.
Step 4: Using the Constructor
You can also use the constructor of the model to initialize and save data in one step. Here's an example:
product = Product(name='Example Product', price=9.99, description='This is an example product description.')
product.save()
In this example, we use the constructor of the Product
model to create an instance and save it to the database in a single line.
Step 5: Bulk Insert
If you have multiple records to insert, you can use the bulk_create()
method to optimize the database insertion. Here's an example:
products = [
Product(name='Product 1', price=9.99, description='Description 1'),
Product(name='Product 2', price=19.99, description='Description 2'),
Product(name='Product 3', price=29.99, description='Description 3')
]
Product.objects.bulk_create(products)
In this example, we create a list of Product
instances and pass it to the bulk_create()
method of the Product.objects
manager to efficiently insert multiple records into the database.
Step 6: Validating Data
Before saving data to the database, Django automatically validates the data based on the model's field definitions. If the data does not meet the field constraints, such as maximum length or data type, Django raises a ValidationError
. It's important to handle and handle these validation errors appropriately in your code.
Conclusion
Inserting data into the database is a fundamental task in web development. By following this guide, you have learned how to define a model, save data using the save()
method or constructor, perform bulk inserts using bulk_create()
, and handle data validation in Django. With these techniques, you can efficiently insert and manage data in your Django web applications.
4.5L
Learners
20+
Instructors
50+
Courses
6.0L
Course enrollments
Future Trending Courses
When selecting, a course, Here are a few areas that are expected to be in demand in the future:.
Future Learning for all
If you’re passionate and ready to dive in, we’d love to join 1:1 classes for you. We’re committed to support our learners and professionals their development and well-being.
View Courses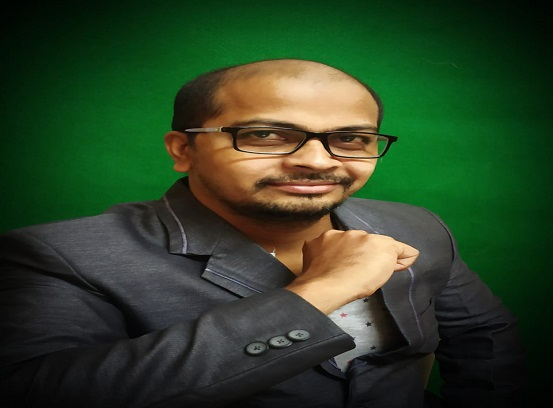
Most Popular Course topics
These are the most popular course topics among Software Courses for learners