Hi there, we’re Harisystems
"Unlock your potential and soar to new heights with our exclusive online courses! Ignite your passion, acquire valuable skills, and embrace limitless possibilities. Don't miss out on our limited-time sale - invest in yourself today and embark on a journey of personal and professional growth. Enroll now and shape your future with knowledge that lasts a lifetime!".
For corporate trainings, projects, and real world experience reach us. We believe that education should be accessible to all, regardless of geographical location or background.
1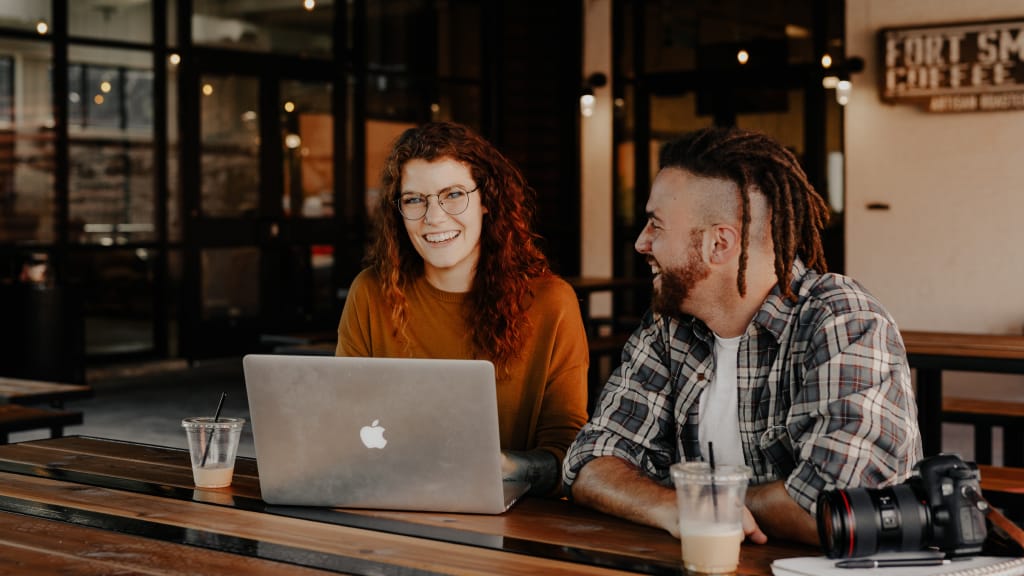
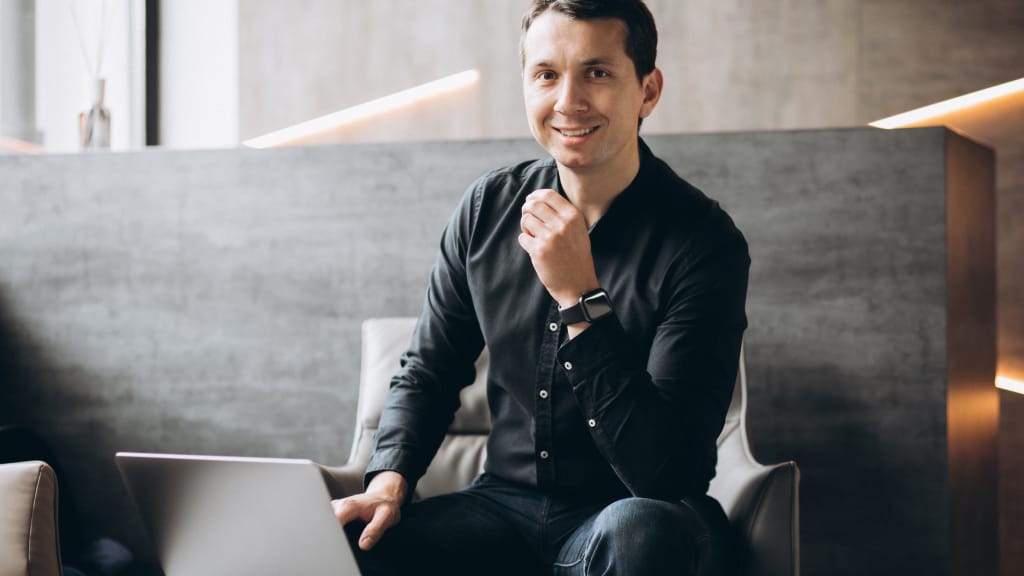
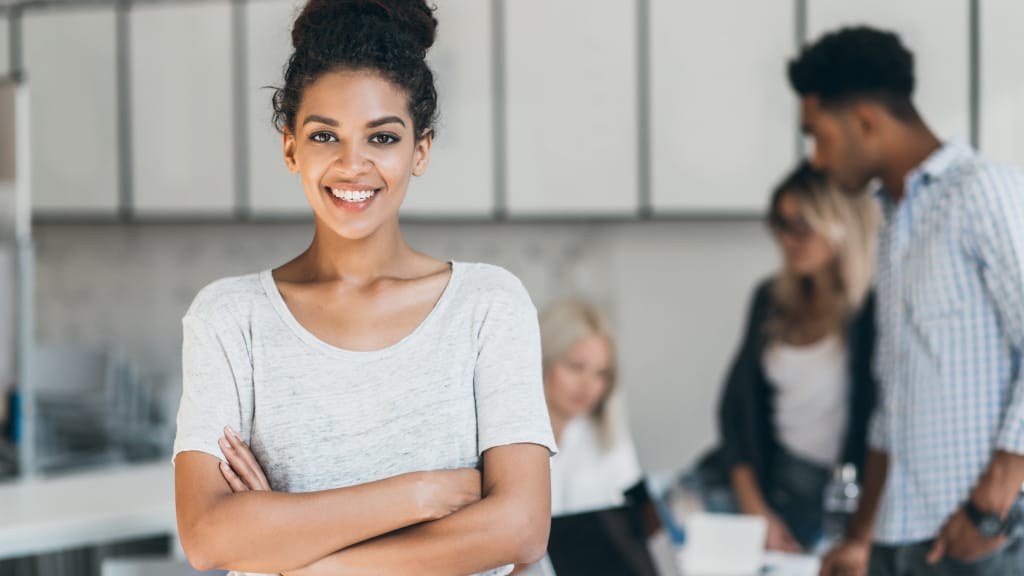
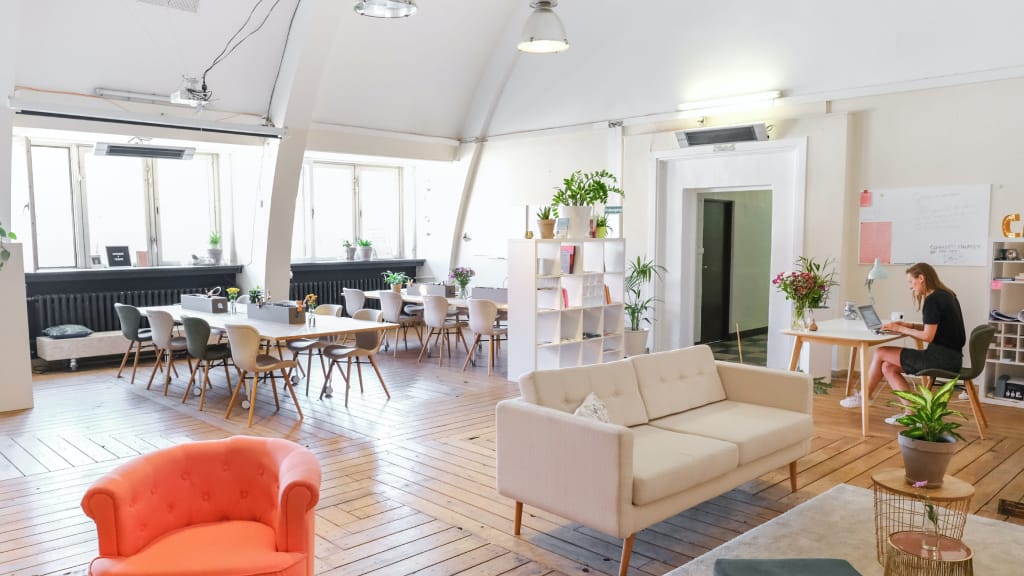
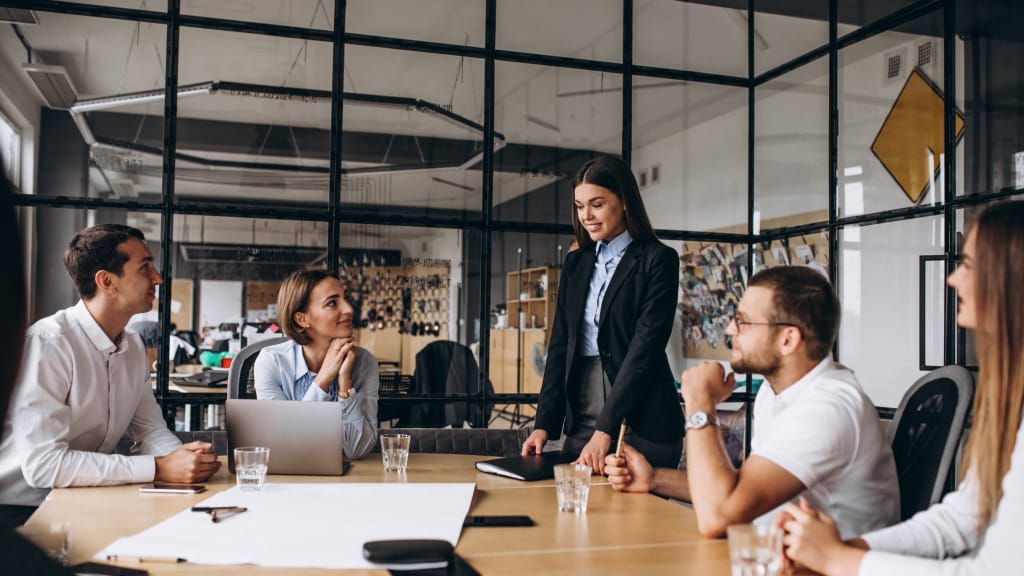
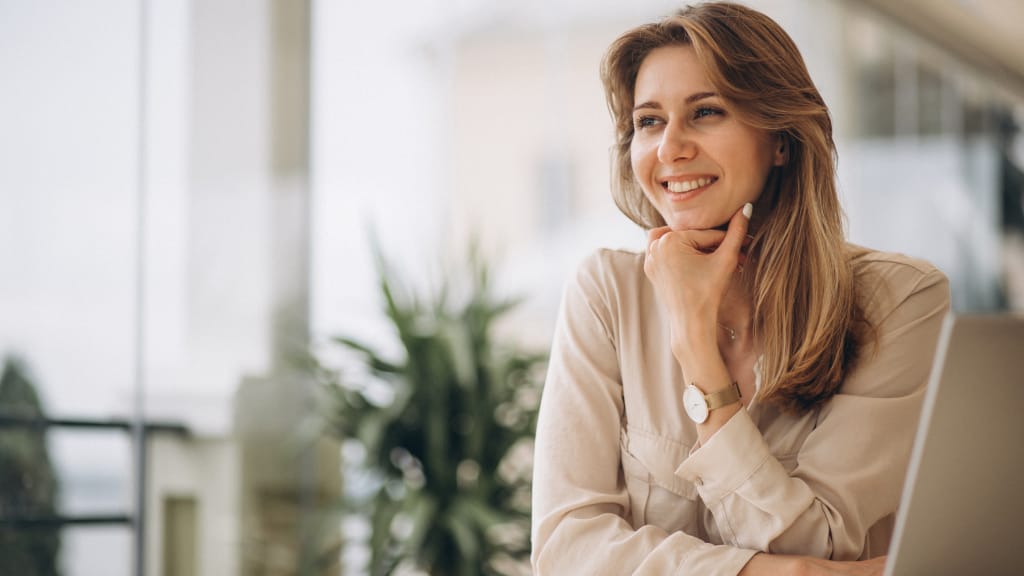
Creating a Django App: A Step-by-Step Guide
Introduction
Django is a powerful web framework that follows the Model-View-Controller (MVC) architectural pattern. In this guide, we will walk you through the process of creating a Django app, which represents a specific functionality within a Django project.
Step 1: Create a Django Project
Before creating a Django app, you need to have a Django project set up. If you haven't created a Django project yet, you can refer to the previous guide on creating a Django project.
Step 2: Creating the App
To create a new Django app, navigate to your project directory in the terminal and run the following command:
python manage.py startapp myapp
This command will generate a new directory named "myapp" within your project directory. The "myapp" directory will contain the necessary files and folders for your app.
Step 3: Configuring the App
Once the app is created, you need to configure it in your Django project. Open the settings.py
file inside your project's main directory and locate the INSTALLED_APPS
list. Add the name of your app, "myapp", to the list of installed apps:
INSTALLED_APPS = [
...
'myapp',
...
]
Step 4: Defining Models
Models in Django represent the structure and behavior of your data. Inside the "myapp" directory, open the models.py
file and define your models. For example, let's create a simple model named "Person":
from django.db import models
class Person(models.Model):
name = models.CharField(max_length=100)
age = models.IntegerField()
In this example, we've defined a "Person" model with two fields: "name" and "age". Django provides various field types like CharField
, IntegerField
, DateField
, and many more to handle different data types.
Step 5: Applying Database Migrations
To create the necessary database tables for your models, run the following commands in your terminal:
python manage.py makemigrations
python manage.py migrate
The first command, makemigrations
, generates migration files based on the changes in your models. The second command, migrate
, applies those changes to the database.
Step 6: Creating Views and Templates
Views handle the logic of your application and interact with models and templates. Inside the "myapp" directory, create a new file called views.py
and define your views. For example, let's create a view that retrieves all "Person" objects from the database:
from django.shortcuts import render
from .models import Person
def person_list(request):
people = Person.objects.all()
return render(request, 'myapp/person_list.html', {'people': people})
In this example, we retrieve all "Person" objects from the database and pass them to the person_list.html
template.
Step 7: Creating Templates
Templates define the HTML structure of your views. Inside the "myapp" directory, create a new directory called "templates". Inside the "templates" directory, create a new HTML file named person_list.html
and add your HTML code. For example:
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Person List</title>
</head>
<body>
<h1>Person List</h1>
<ul>
{% for person in people %}
<li>Name: {{ person.name }}, Age: {{ person.age }}</li>
{% endfor %}
</ul>
</body>
</html>
Step 8: Mapping URLs
To map URLs to your views, open the urls.py
file inside your project's main directory and add the necessary URL patterns. For example:
from django.urls import path
from myapp.views import person_list
urlpatterns = [
path('persons/', person_list, name='person_list'),
]
Step 9: Testing the App
Finally, start the development server by running python manage.py runserver
in your terminal. Open your browser and navigate to http://localhost:8000/persons/
to see the list of people rendered from the database.
Conclusion
By following these steps, you have successfully created a Django app. Django's app-based architecture allows you to organize your project's functionality into separate apps, making your code more modular and maintainable. With your app in place, you can now expand and enhance it to build powerful web applications using Django's features.
4.5L
Learners
20+
Instructors
50+
Courses
6.0L
Course enrollments
Future Trending Courses
When selecting, a course, Here are a few areas that are expected to be in demand in the future:.
Future Learning for all
If you’re passionate and ready to dive in, we’d love to join 1:1 classes for you. We’re committed to support our learners and professionals their development and well-being.
View Courses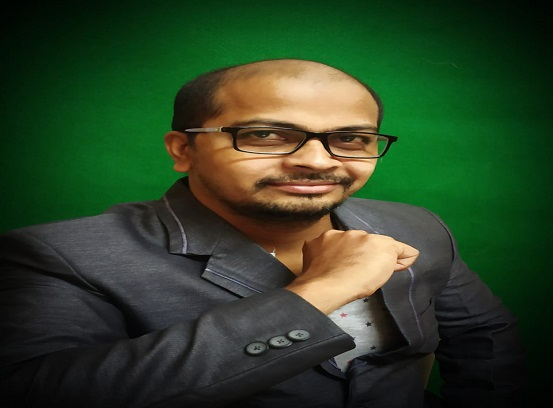
Most Popular Course topics
These are the most popular course topics among Software Courses for learners