Hi there, we’re Harisystems
"Unlock your potential and soar to new heights with our exclusive online courses! Ignite your passion, acquire valuable skills, and embrace limitless possibilities. Don't miss out on our limited-time sale - invest in yourself today and embark on a journey of personal and professional growth. Enroll now and shape your future with knowledge that lasts a lifetime!".
For corporate trainings, projects, and real world experience reach us. We believe that education should be accessible to all, regardless of geographical location or background.
1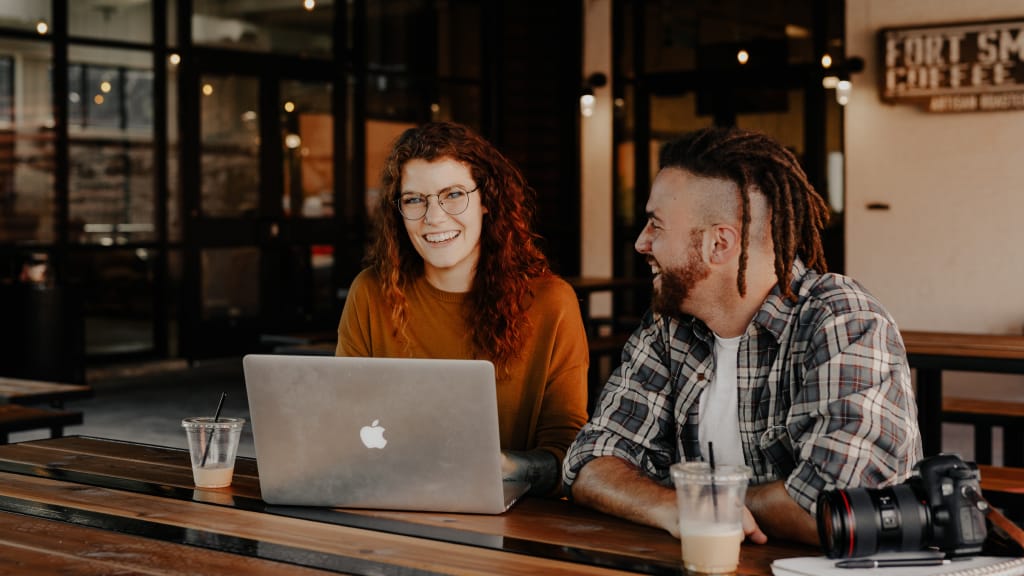
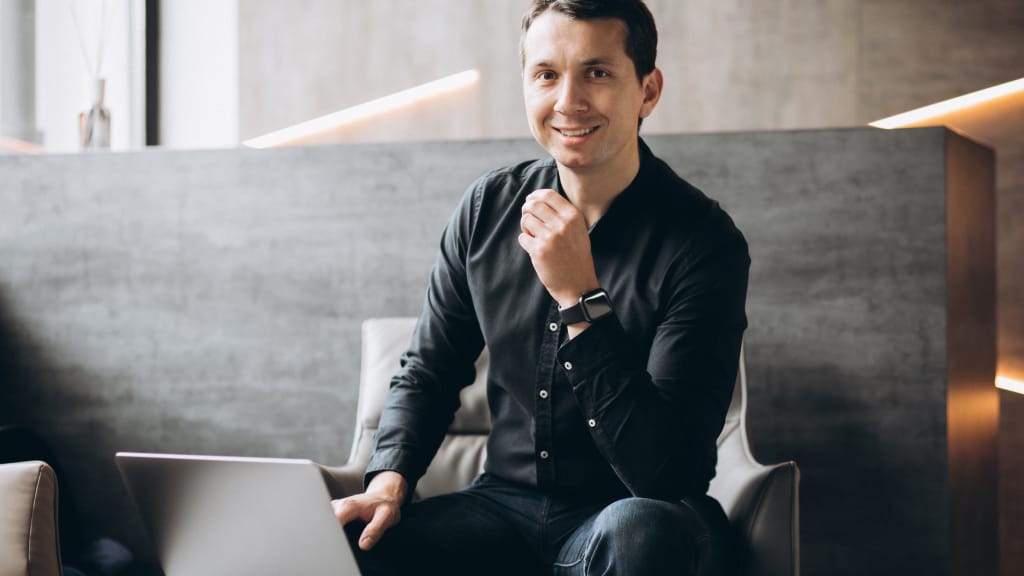
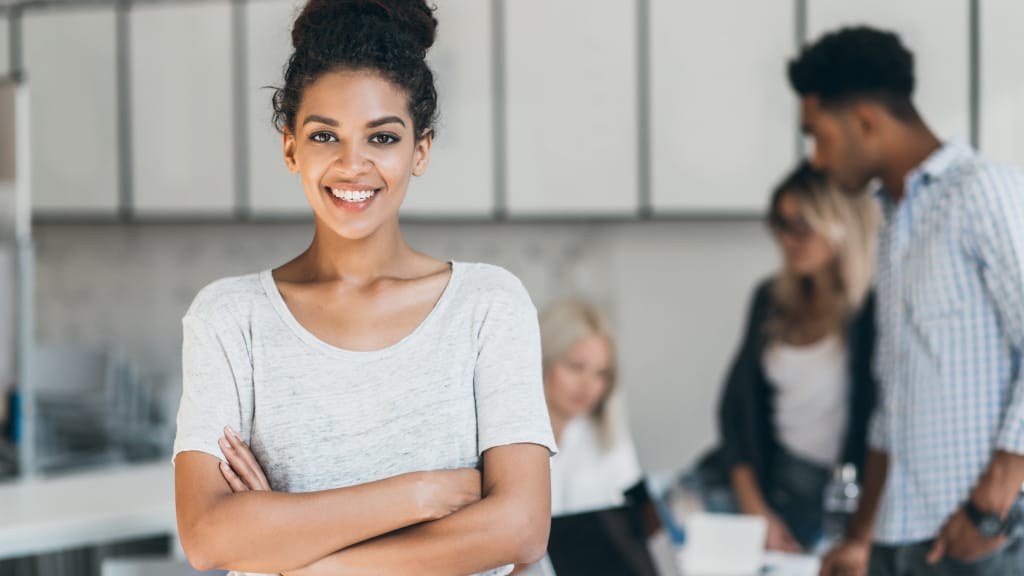
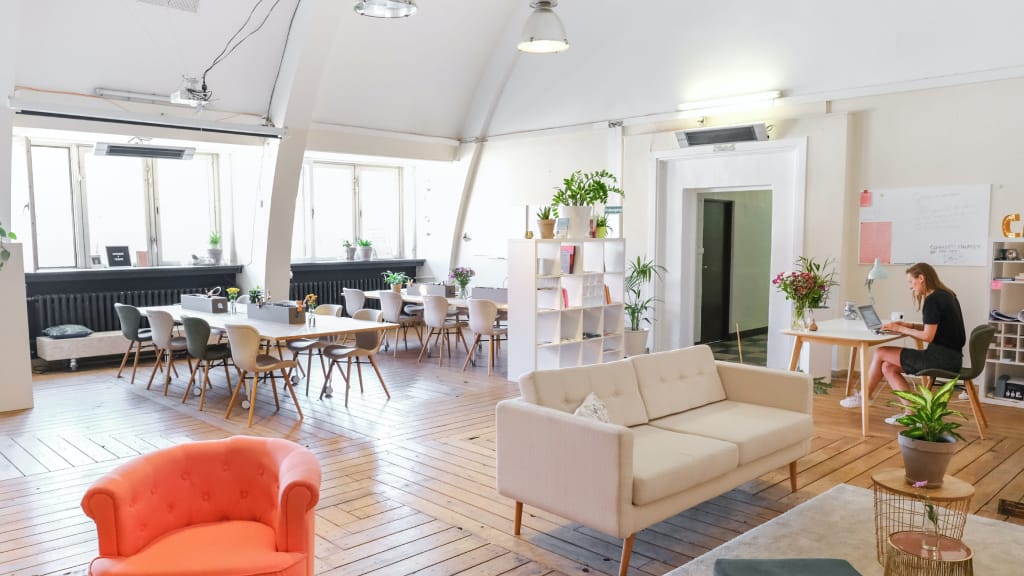
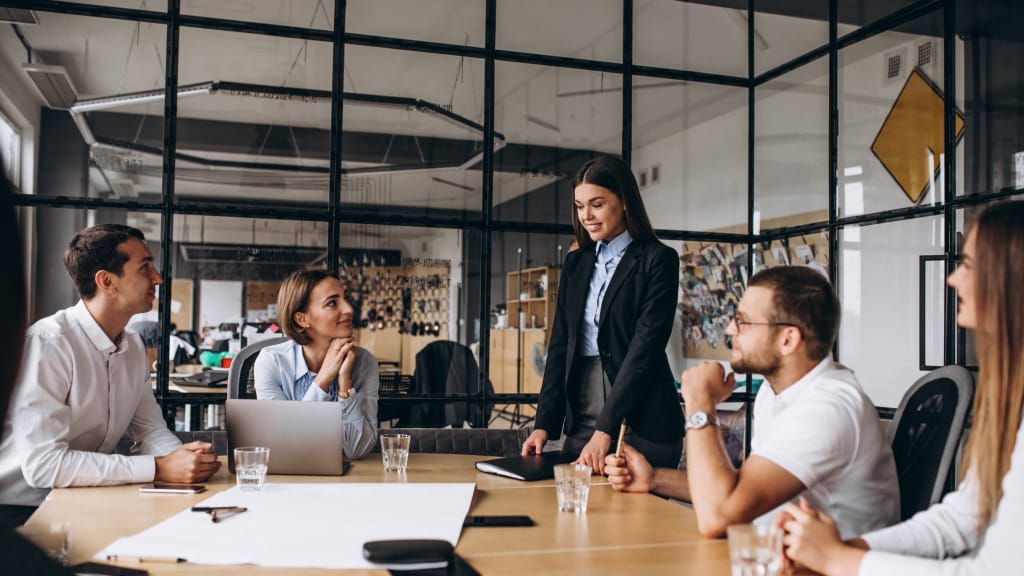
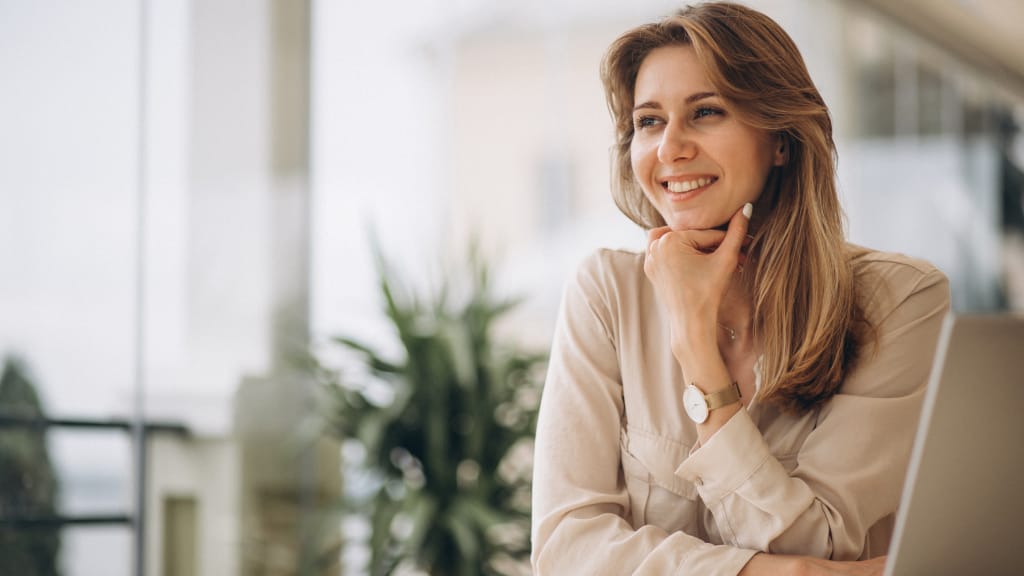
Web Development: Asynchronous JavaScript with AJAX
Asynchronous JavaScript and XML (AJAX) is a powerful technique that allows web developers to send and receive data from a server without reloading the entire web page. AJAX enables dynamic and interactive web experiences by fetching and updating data in the background. In this article, we will explore the basics of AJAX and provide examples of its implementation in web development.
What is AJAX?
AJAX combines JavaScript, XML (although other data formats like JSON are commonly used), and server-side technologies to enable asynchronous communication between the client and server. With AJAX, web applications can send requests to the server in the background, receive responses, and update the web page dynamically without interrupting the user's interaction.
The XMLHttpRequest Object
The XMLHttpRequest object is the core component of AJAX. It provides methods and properties to send HTTP requests and handle server responses. Here's an example of using the XMLHttpRequest object to fetch data from a server:
<script>
var xhttp = new XMLHttpRequest();
xhttp.onreadystatechange = function() {
if (this.readyState === 4 && this.status === 200) {
console.log(this.responseText);
}
};
xhttp.open("GET", "https://api.example.com/data", true);
xhttp.send();
</script>
Handling Asynchronous Requests
AJAX requests are asynchronous by default, meaning the JavaScript code continues to execute while waiting for a response from the server. To handle the response, you can use the onreadystatechange
event or the newer onload
and onerror
events. Here's an example:
<script>
var xhttp = new XMLHttpRequest();
xhttp.onload = function() {
if (this.status === 200) {
console.log(this.responseText);
}
};
xhttp.onerror = function() {
console.log("Request failed.");
};
xhttp.open("GET", "https://api.example.com/data", true);
xhttp.send();
</script>
Sending Data to the Server
AJAX can also send data to the server using HTTP methods like POST or PUT. Here's an example of sending data to a server using the POST
method:
<script>
var xhttp = new XMLHttpRequest();
xhttp.onreadystatechange = function() {
if (this.readyState === 4 && this.status === 200) {
console.log(this.responseText);
}
};
xhttp.open("POST", "https://api.example.com/data", true);
xhttp.setRequestHeader("Content-type", "application/json");
xhttp.send(JSON.stringify({ name: "John", age: 25 }));
</script>
Working with Fetch API
The Fetch API is a newer and more powerful alternative to the XMLHttpRequest object for making AJAX requests. Here's an example of fetching data using the Fetch API:
<script>
fetch("https://api.example.com/data")
.then(function(response) {
if (response.ok) {
return response.json();
} else {
throw new Error("Request failed.");
}
})
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error.message);
});
</script>
Conclusion
AJAX revolutionized web development by enabling dynamic and interactive web experiences without page reloads. With the XMLHttpRequest object or the Fetch API, web developers can send and receive data from the server asynchronously, making web applications more responsive and user-friendly. Understanding AJAX and its implementation in web development is crucial for building modern, data-driven web applications.
4.5L
Learners
20+
Instructors
50+
Courses
6.0L
Course enrollments
Future Trending Courses
When selecting, a course, Here are a few areas that are expected to be in demand in the future:.
Future Learning for all
If you’re passionate and ready to dive in, we’d love to join 1:1 classes for you. We’re committed to support our learners and professionals their development and well-being.
View Courses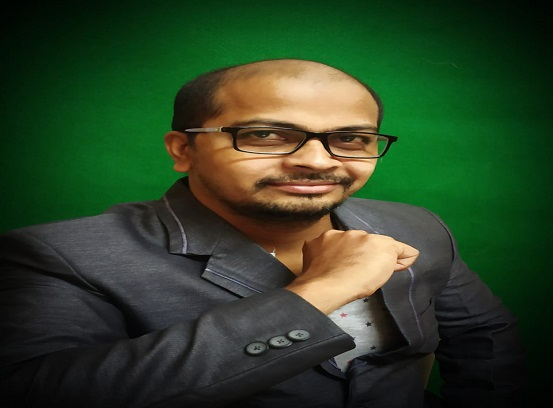
Most Popular Course topics
These are the most popular course topics among Software Courses for learners