Hi there, we’re Harisystems
"Unlock your potential and soar to new heights with our exclusive online courses! Ignite your passion, acquire valuable skills, and embrace limitless possibilities. Don't miss out on our limited-time sale - invest in yourself today and embark on a journey of personal and professional growth. Enroll now and shape your future with knowledge that lasts a lifetime!".
For corporate trainings, projects, and real world experience reach us. We believe that education should be accessible to all, regardless of geographical location or background.
1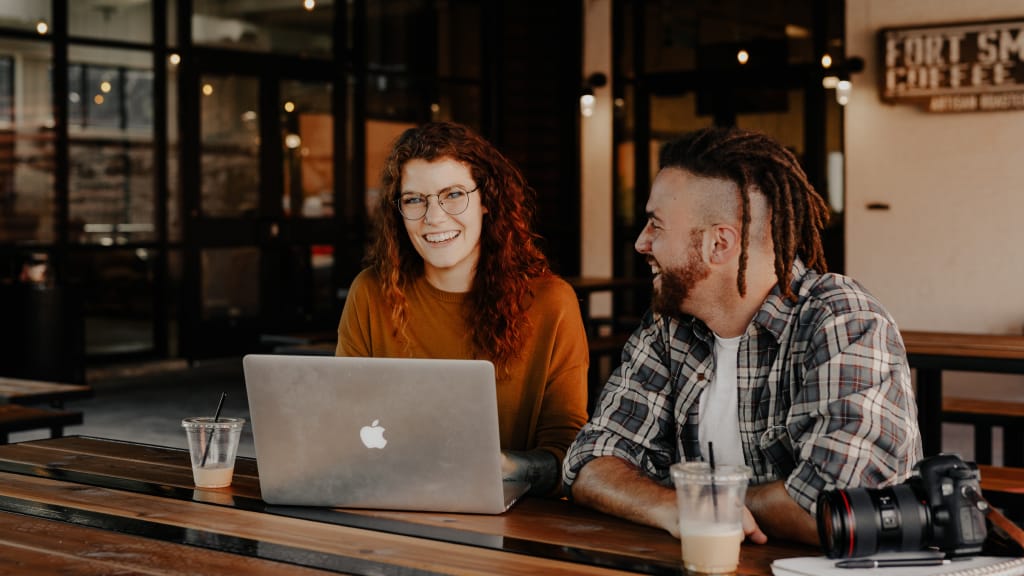
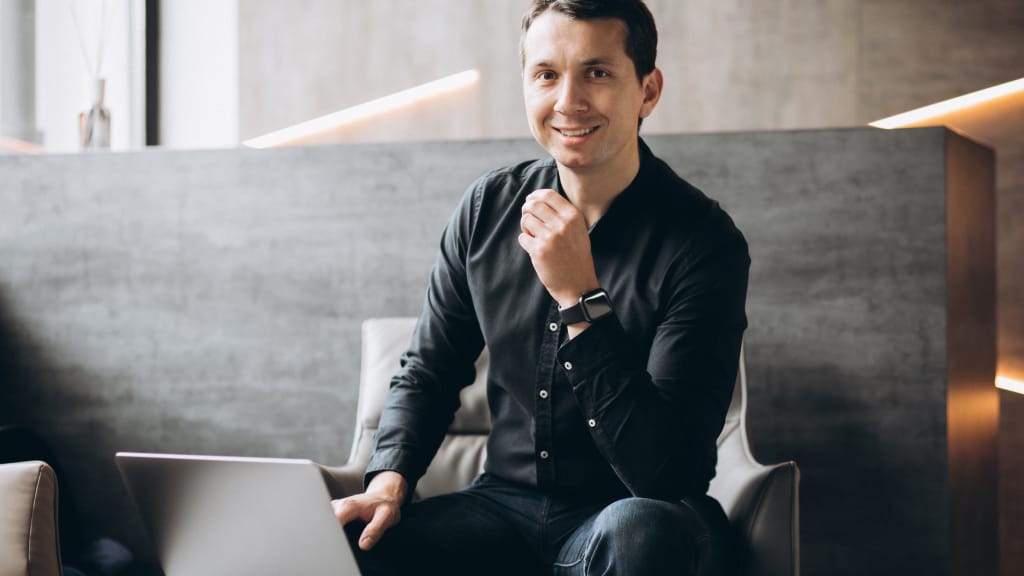
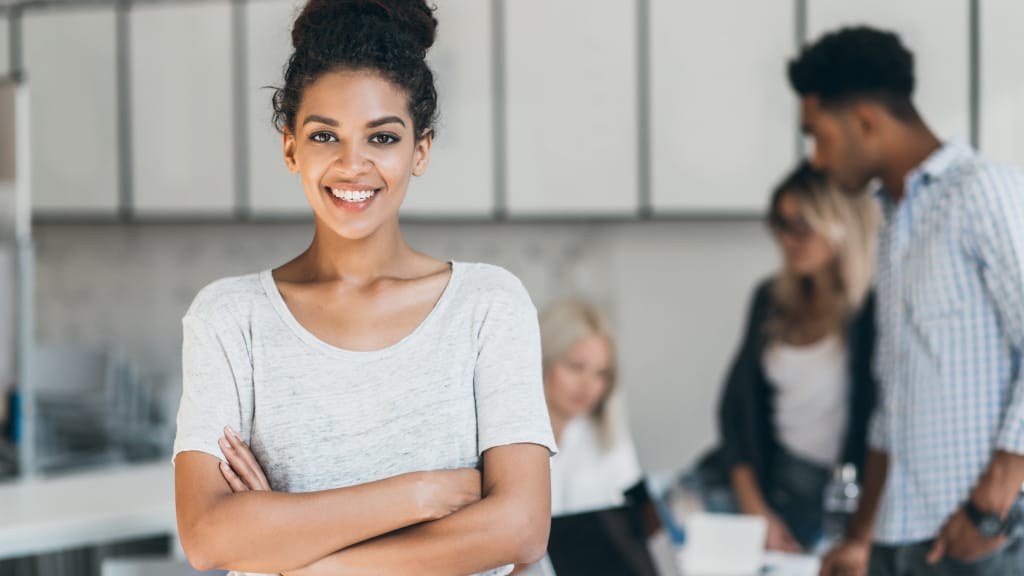
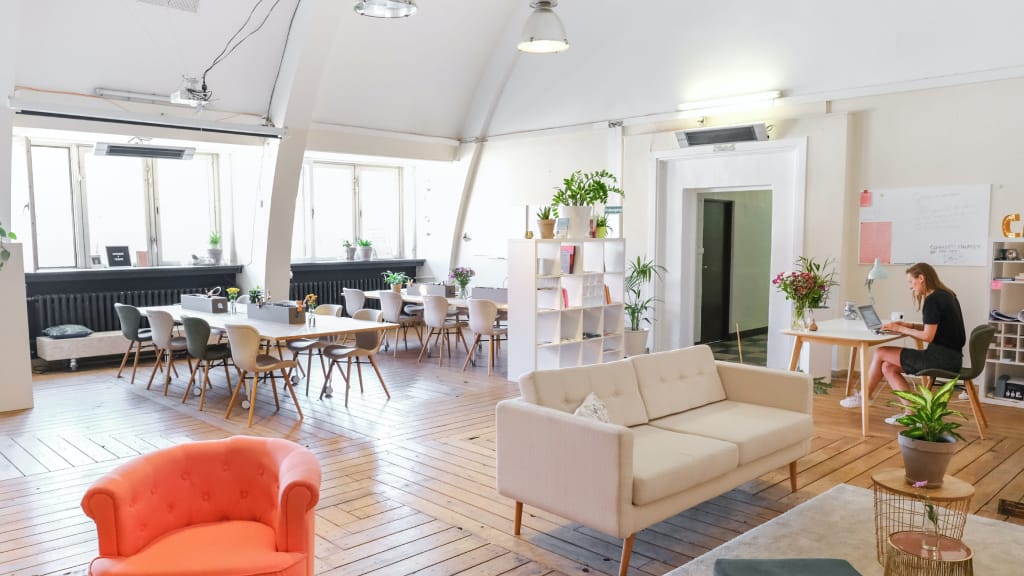
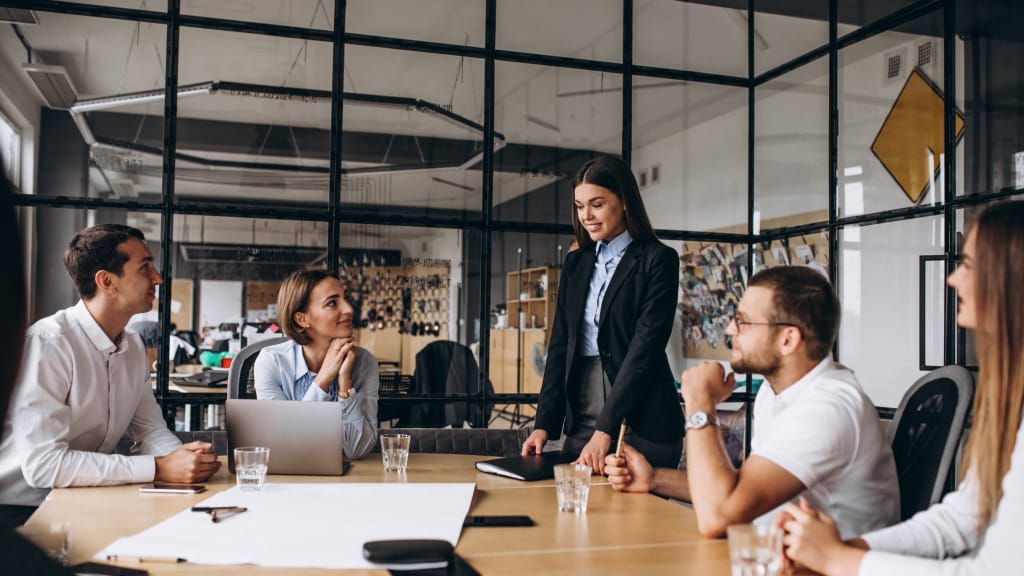
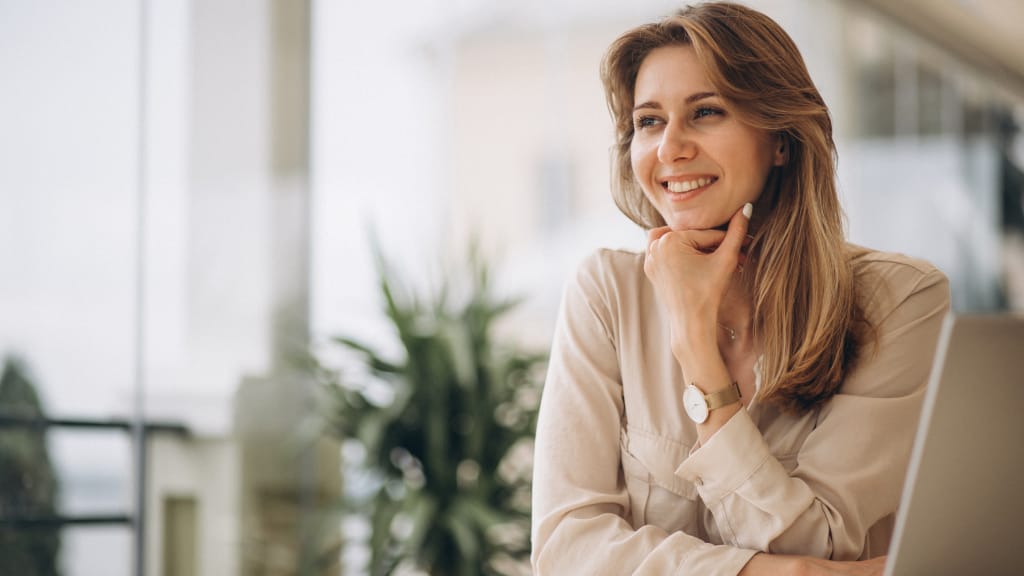
Web Development: Exploring SQL in Web Applications
SQL (Structured Query Language) is a powerful programming language used for managing and manipulating data in relational databases. In web development, SQL plays a crucial role in handling data storage, retrieval, and manipulation in web applications. In this article, we will explore the basics of using SQL in web development and provide examples of its implementation.
What is SQL?
SQL is a standard programming language for managing relational databases. It enables developers to define, manipulate, and query structured data. SQL is used to create and manage database tables, insert and retrieve data, update records, and perform various other database operations.
Using SQL in Web Applications
Web applications often require interaction with a database to store and retrieve data. SQL is used to communicate with the database and perform operations such as data insertion, retrieval, and updates. Here's an example of using SQL in a web application:
<!DOCTYPE html>
<html>
<head>
<title>Web Application with SQL Example</title>
</head>
<body>
<h1>Users</h1>
<ul>
<?php
$conn = new mysqli('localhost', 'username', 'password', 'database_name');
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
$sql = "SELECT * FROM users";
$result = $conn->query($sql);
if ($result->num_rows > 0) {
while ($row = $result->fetch_assoc()) {
echo "<li>" . $row["username"] . "</li>";
}
} else {
echo "No users found.";
}
$conn->close();
?>
</ul>
</body>
</html>
The example above demonstrates a simple PHP script that connects to a database, executes an SQL query to retrieve user data, and displays it in a list format on a web page.
SQL Query Examples
SQL queries are used to interact with the database and perform various operations. Here are some common SQL query examples:
- SELECT: Retrieve data from a table.
- INSERT: Insert new records into a table.
- UPDATE: Update existing records in a table.
- DELETE: Remove records from a table.
Here's an example of using SQL queries:
SELECT * FROM users;
INSERT INTO users (username, email) VALUES ('john', 'john@example.com');
UPDATE users SET email = 'newemail@example.com' WHERE id = 1;
DELETE FROM users WHERE id = 1;
Conclusion
SQL is a fundamental language in web development for managing relational databases. It allows developers to interact with databases, perform various data operations, and retrieve or modify data in web applications. Understanding SQL and its usage in web development is essential for building robust and data-driven web applications. Continuously learning and exploring SQL techniques and best practices will enable developers to efficiently manage data and create powerful web applications.
4.5L
Learners
20+
Instructors
50+
Courses
6.0L
Course enrollments
Future Trending Courses
When selecting, a course, Here are a few areas that are expected to be in demand in the future:.
Future Learning for all
If you’re passionate and ready to dive in, we’d love to join 1:1 classes for you. We’re committed to support our learners and professionals their development and well-being.
View Courses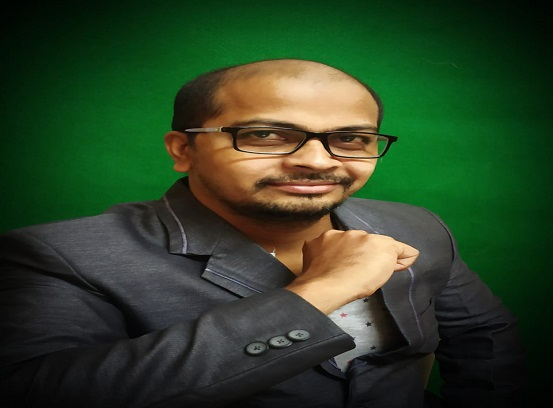
Most Popular Course topics
These are the most popular course topics among Software Courses for learners