Hi there, we’re Harisystems
"Unlock your potential and soar to new heights with our exclusive online courses! Ignite your passion, acquire valuable skills, and embrace limitless possibilities. Don't miss out on our limited-time sale - invest in yourself today and embark on a journey of personal and professional growth. Enroll now and shape your future with knowledge that lasts a lifetime!".
For corporate trainings, projects, and real world experience reach us. We believe that education should be accessible to all, regardless of geographical location or background.
1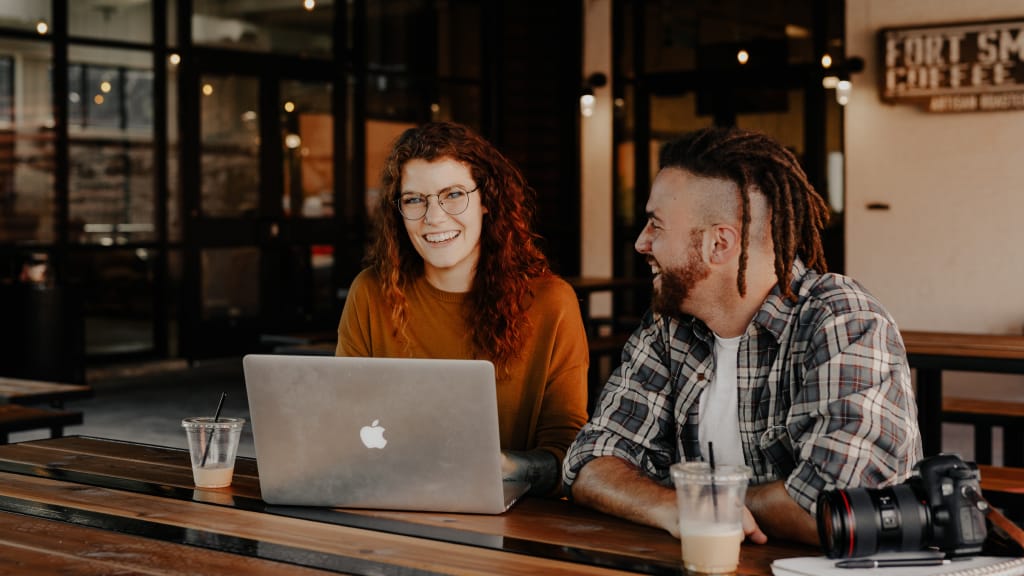
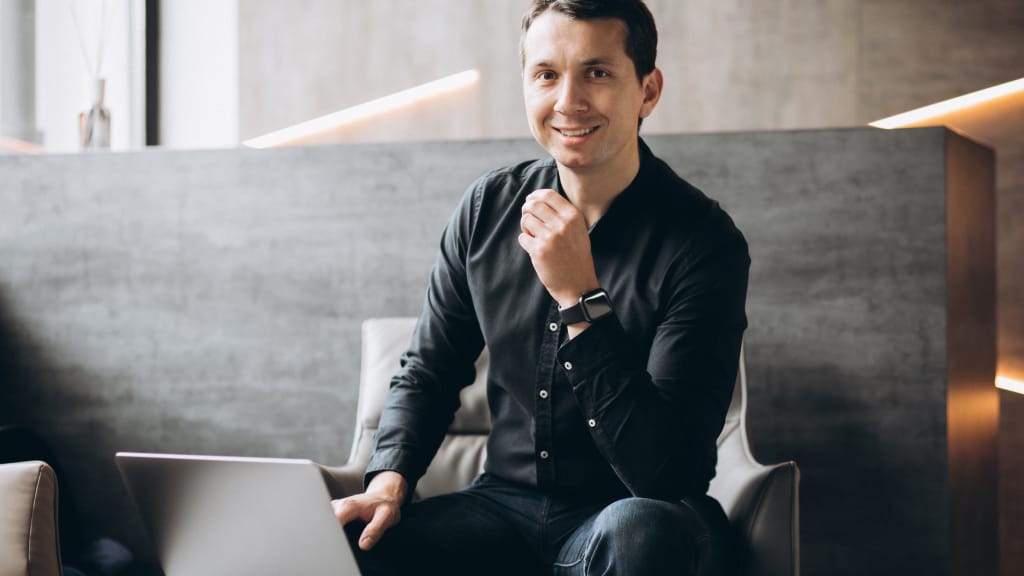
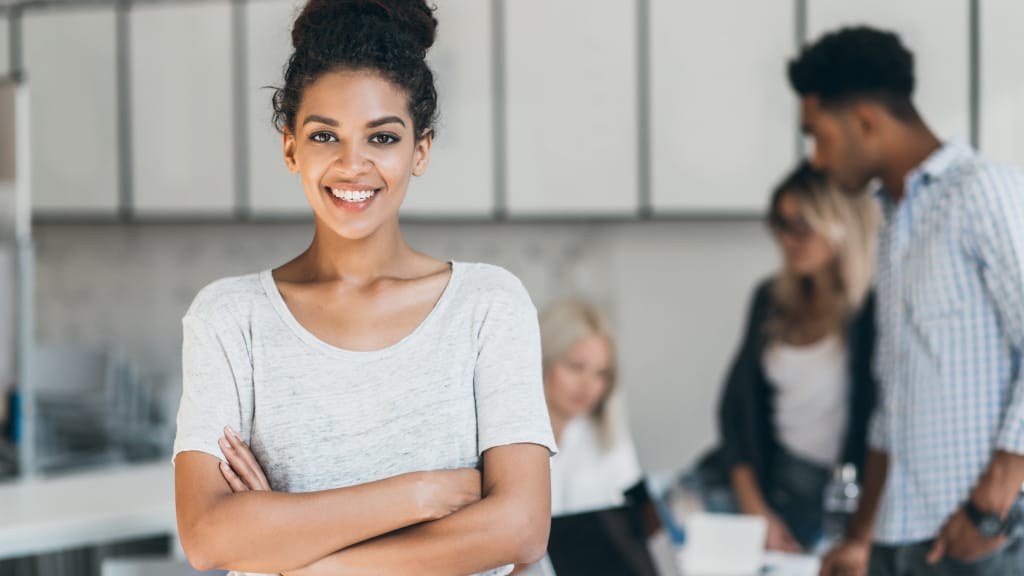
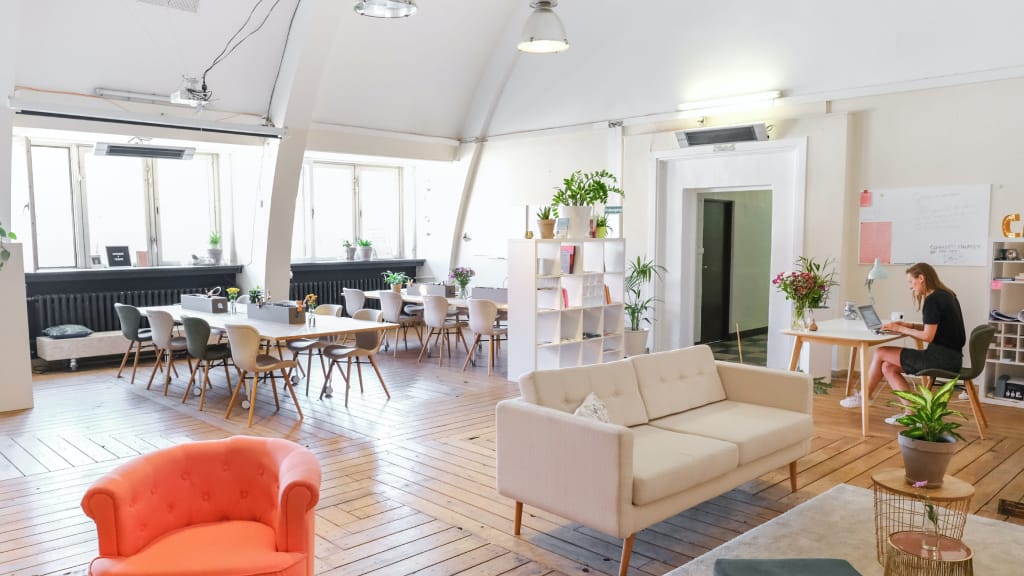
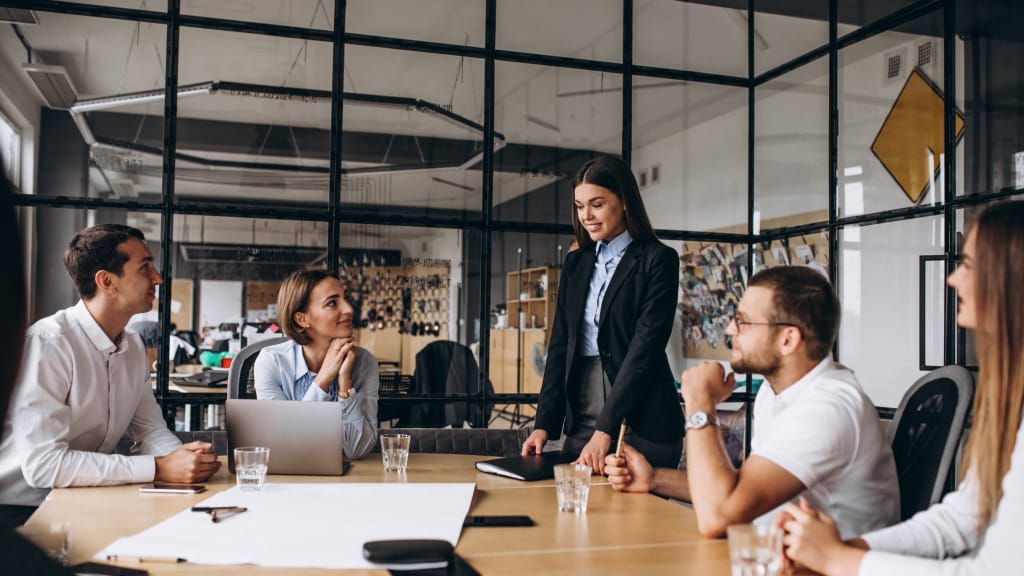
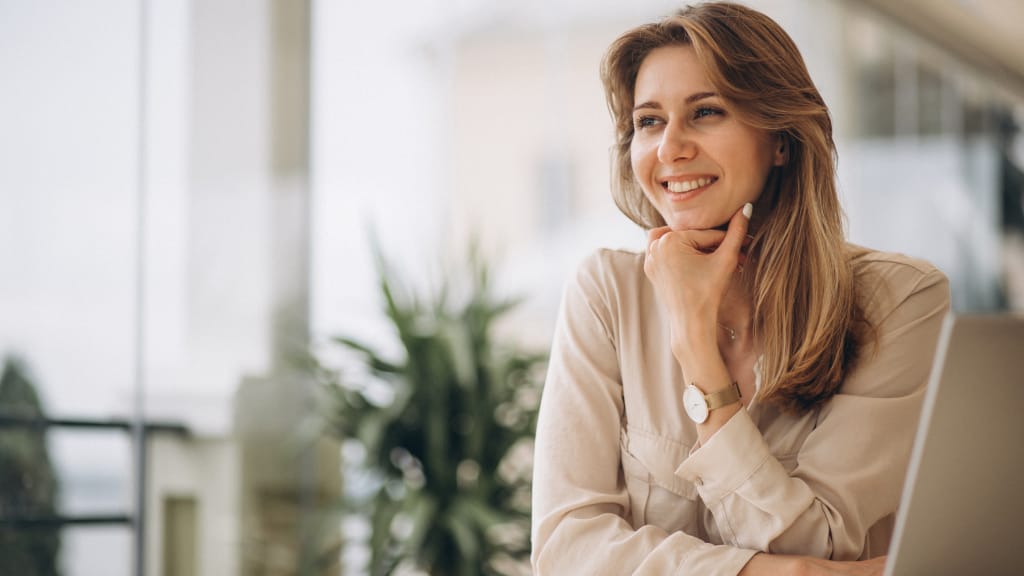
Java Operators: Performing Operations
In Java, operators are symbols that perform various operations on operands, such as numbers, variables, or expressions. They allow you to manipulate and combine values in your Java programs. In this article, we will explore the different types of operators in Java and provide examples to help you understand their usage.
Arithmetic Operators
Arithmetic operators perform basic mathematical operations. Here are the common arithmetic operators in Java:
- Addition (+): Adds two operands together. Example:
int sum = 5 + 3;
- Subtraction (-): Subtracts the second operand from the first operand. Example:
int difference = 10 - 5;
- Multiplication (*): Multiplies two operands. Example:
int product = 2 * 3;
- Division (/): Divides the first operand by the second operand. Example:
double quotient = 10 / 2;
- Modulus (%): Calculates the remainder of the division between the first operand and the second operand. Example:
int remainder = 10 % 3;
- Increment (++): Increases the value of the operand by 1. Example:
int count = 0; count++;
- Decrement (--): Decreases the value of the operand by 1. Example:
int count = 5; count--;
Assignment Operators
Assignment operators are used to assign values to variables. Here's an example:
int age = 25;
double salary = 50000.5;
In the above example, the =
operator assigns the value 25
to the variable age
and the value 50000.5
to the variable salary
.
Comparison Operators
Comparison operators compare two operands and return a boolean value indicating the result. Here are the common comparison operators in Java:
- Equal to (==): Checks if two operands are equal. Example:
boolean result = 5 == 5;
- Not equal to (!=): Checks if two operands are not equal. Example:
boolean result = 5 != 3;
- Greater than (>): Checks if the first operand is greater than the second operand. Example:
boolean result = 10 > 5;
- Less than (<): Checks if the first operand is less than the second operand. Example:
boolean result = 5 < 10;
- Greater than or equal to (>=): Checks if the first operand is greater than or equal to the second operand. Example:
boolean result = 5 >= 5;
- Less than or equal to (<=): Checks if the first operand is less than or equal to the second operand. Example:
boolean result = 5 <= 10;
Logical Operators
Logical operators perform logical operations on boolean values. Here are the common logical operators in Java:
- AND (&&): Returns
true
if both operands aretrue
. Example:boolean result = true && false;
- OR (||): Returns
true
if at least one of the operands istrue
. Example:boolean result = true || false;
- NOT (!): Negates the value of the operand. Example:
boolean result = !true;
Bitwise Operators
Bitwise operators perform operations on individual bits of integer values. Here are the common bitwise operators in Java:
- AND (&): Performs a bitwise AND operation. Example:
int result = 5 & 3;
- OR (|): Performs a bitwise OR operation. Example:
int result = 5 | 3;
- XOR (^): Performs a bitwise XOR (exclusive OR) operation. Example:
int result = 5 ^ 3;
- Shift Left (<<): Shifts the bits of the operand to the left. Example:
int result = 5 << 2;
- Shift Right (>>): Shifts the bits of the operand to the right. Example:
int result = 5 >> 2;
Ternary Operator
The ternary operator is a shorthand way of writing an if-else
statement. It takes three operands and returns a value based on a condition. Here's an example:
int age = 18;
String status = (age >= 18) ? "Adult" : "Minor";
In the above example, if age
is greater than or equal to 18
, the value of status
will be set to "Adult"
; otherwise, it will be set to "Minor"
.
Conclusion
Operators play a vital role in performing various operations in Java. In this article, we explored the different types of operators, including arithmetic, assignment, comparison, logical, bitwise, and the ternary operator. Understanding operators is essential for manipulating data and controlling the flow of your Java programs. Continuously practice using operators and explore more advanced topics, such as operator precedence and operator overloading, to enhance your Java programming skills.
4.5L
Learners
20+
Instructors
50+
Courses
6.0L
Course enrollments
Future Trending Courses
When selecting, a course, Here are a few areas that are expected to be in demand in the future:.
Future Learning for all
If you’re passionate and ready to dive in, we’d love to join 1:1 classes for you. We’re committed to support our learners and professionals their development and well-being.
View Courses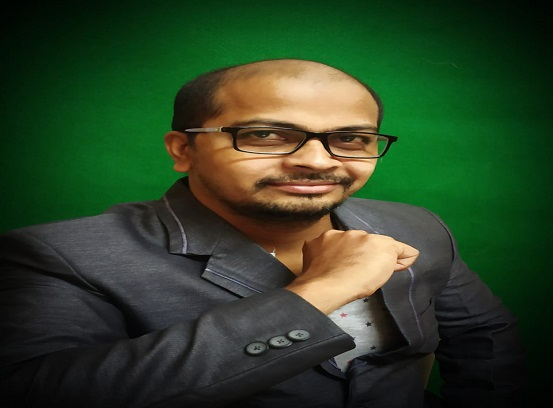
Most Popular Course topics
These are the most popular course topics among Software Courses for learners