Hi there, we’re Harisystems
"Unlock your potential and soar to new heights with our exclusive online courses! Ignite your passion, acquire valuable skills, and embrace limitless possibilities. Don't miss out on our limited-time sale - invest in yourself today and embark on a journey of personal and professional growth. Enroll now and shape your future with knowledge that lasts a lifetime!".
For corporate trainings, projects, and real world experience reach us. We believe that education should be accessible to all, regardless of geographical location or background.
1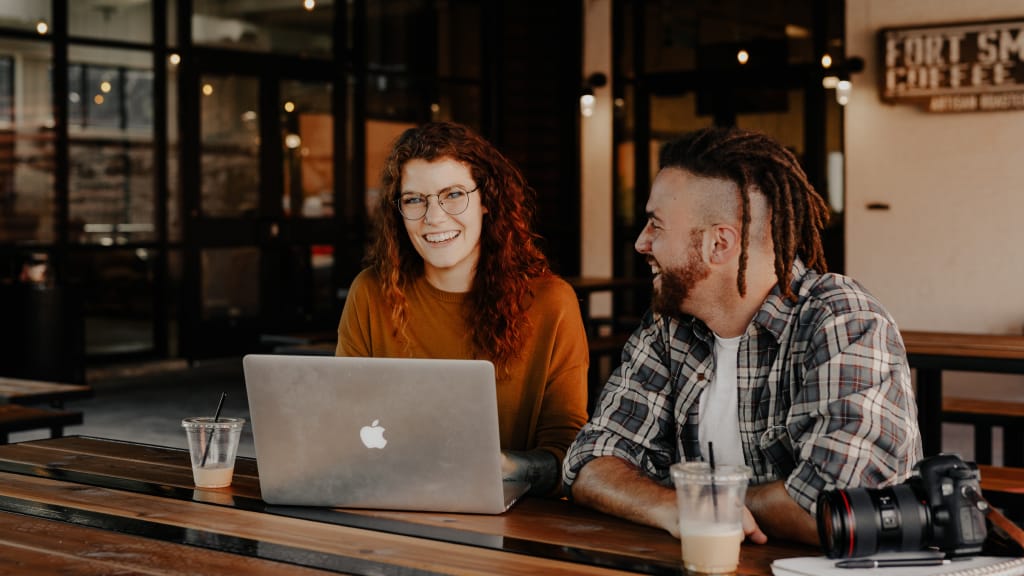
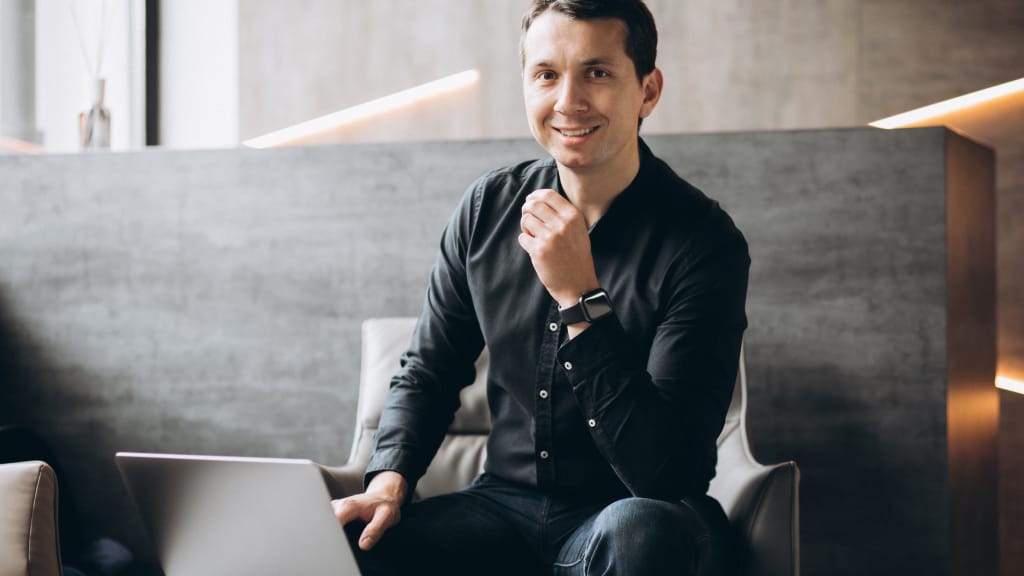
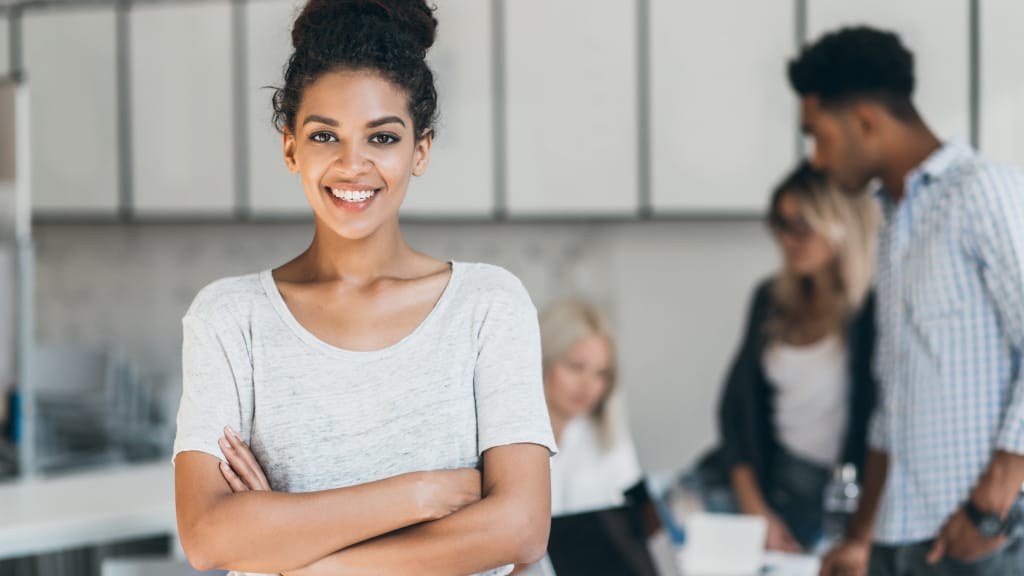
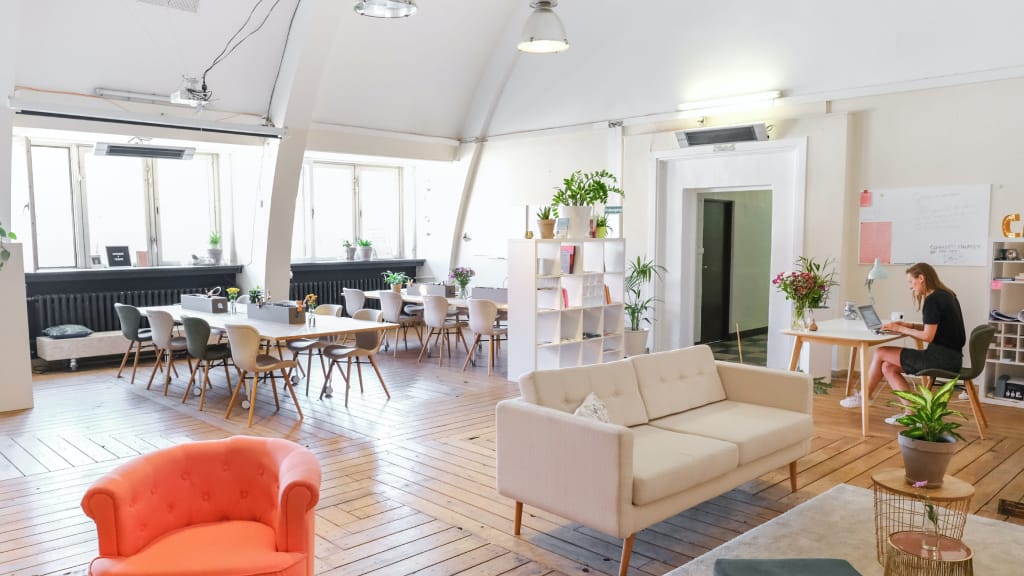
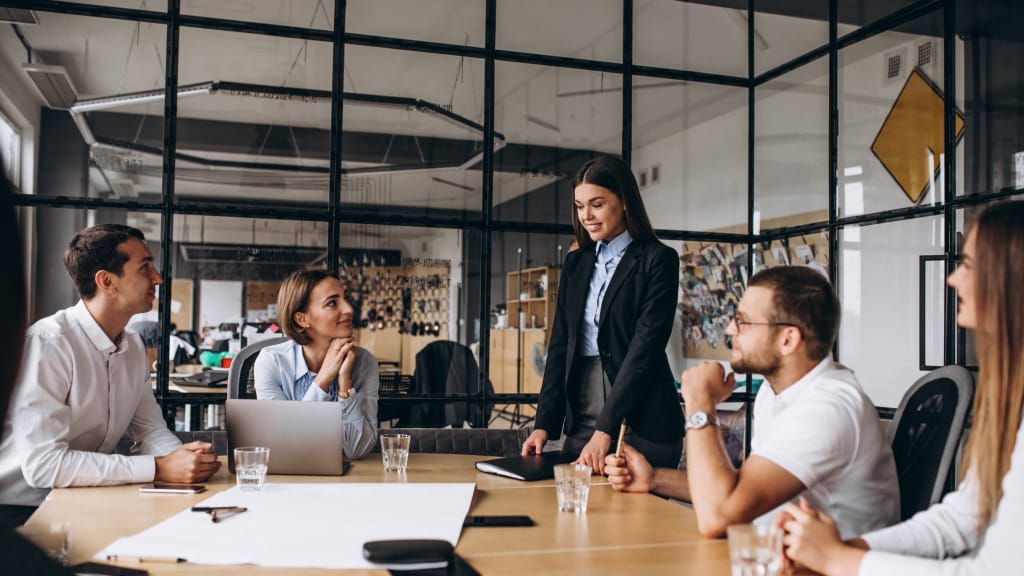
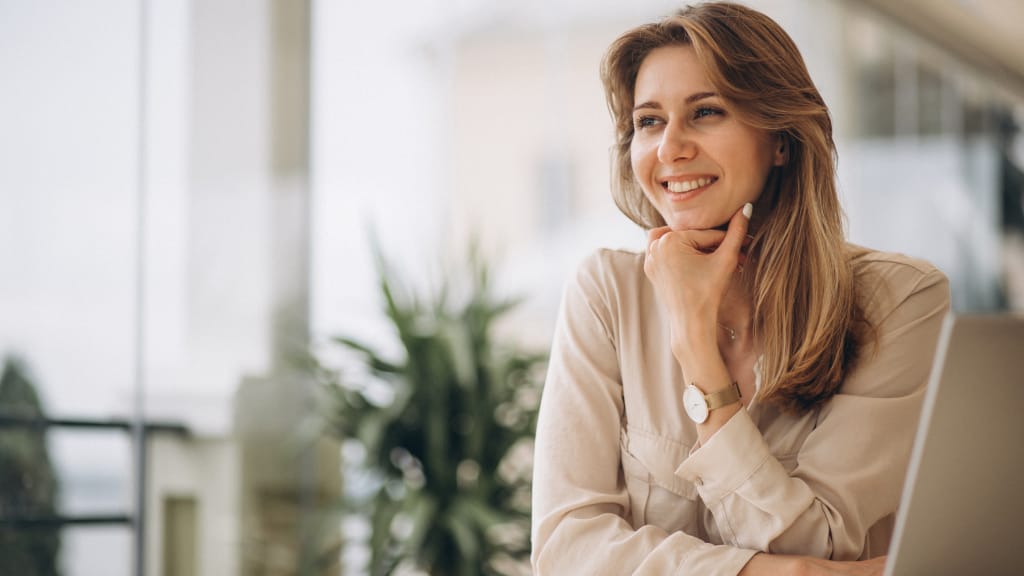
Django Templates: Building Dynamic Web Pages
Introduction
In Django, templates play a crucial role in separating the presentation logic from the business logic of your web application. Templates allow you to create dynamic web pages by combining HTML with Django's template language. In this guide, we will explore Django templates with examples.
Step 1: Creating a Django Project
Before we dive into templates, make sure you have a Django project set up. If you haven't created a Django project yet, you can refer to the previous guide on creating a Django project.
Step 2: Understanding Django Templates
Django templates are HTML files that include special template tags and variables. These tags and variables allow you to inject dynamic content into your HTML, making your web pages flexible and interactive.
Step 3: Creating a Template
To create a template, navigate to your app's directory and create a new directory called "templates". Inside the "templates" directory, create a new HTML file, for example, "mytemplate.html". Here's an example of a simple template:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>My Template</title>
</head>
<body>
<h1>Hello, {{ name }}!</h1>
</body>
</html>
In this example, we've created a template that includes a heading tag with a dynamic value, represented by the {{ name }}
template variable.
Step 4: Rendering a Template
To render a template, you need to define a view function that retrieves data and passes it to the template for rendering. Here's an example:
from django.shortcuts import render
def my_view(request):
name = 'John'
return render(request, 'myapp/mytemplate.html', {'name': name})
In this example, we define a view function called my_view
. Inside the function, we define a variable named name
with the value 'John'. We then use the render
function to render the mytemplate.html
template and pass the name
variable as a context variable.
Step 5: Context Variables
Context variables allow you to pass dynamic data from your views to your templates. In the previous example, we passed the name
variable as a context variable. You can include multiple variables in the context dictionary, providing the necessary data for rendering your template.
Step 6: Template Tags
Django provides various template tags that allow you to perform logic and control flow in your templates. For example, you can use the {% if %}
tag to conditionally display content, or the {% for %}
tag to loop over a collection of items. Here's an example:
<ul>
{% for item in items %}
<li>{{ item }}</li>
{% endfor %}
</ul>
In this example, we use the {% for %}
tag to iterate over a list of items and display each item in an HTML list.
Step 7: Template Inheritance
Django templates support inheritance, allowing you to define a base template and extend it in child templates. This feature helps you reuse common HTML structure and promote code organization. Here's an example:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>My Website</title>
</head>
<body>
<header>
<h1>My Website</h1>
</header>
<main>
{% block content %}
{% endblock %}
</main>
<footer>
<p>Copyright © 2023</p>
</footer>
</body>
</html>
{% extends 'base.html' %}
{% block content %}
<h2>Welcome to my website!</h2>
<p>This is the child template.</p>
{% endblock %}
In this example, we define a base template called base.html
that includes a header, main content area, and footer. The {% block %}
tags define the content area that can be overridden in child templates. The child.html
template extends the base.html
template and overrides the {% block content %}
block to include specific content.
Conclusion
Django templates provide a powerful mechanism for creating dynamic web pages. By following this guide, you have learned how to create templates, render them with context variables, use template tags for logic and control flow, and leverage template inheritance for code reuse. With Django templates, you can build flexible and interactive web applications with ease.
4.5L
Learners
20+
Instructors
50+
Courses
6.0L
Course enrollments
Future Trending Courses
When selecting, a course, Here are a few areas that are expected to be in demand in the future:.
Future Learning for all
If you’re passionate and ready to dive in, we’d love to join 1:1 classes for you. We’re committed to support our learners and professionals their development and well-being.
View Courses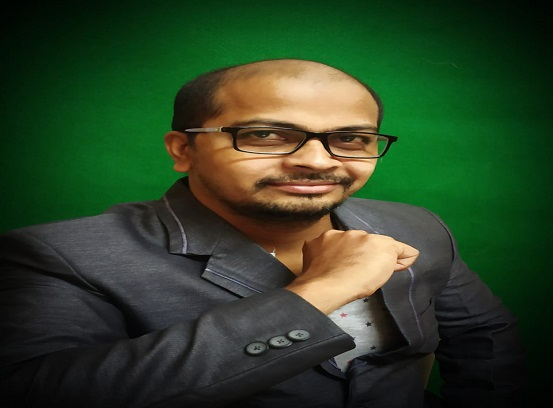
Most Popular Course topics
These are the most popular course topics among Software Courses for learners