Hi there, we’re Harisystems
"Unlock your potential and soar to new heights with our exclusive online courses! Ignite your passion, acquire valuable skills, and embrace limitless possibilities. Don't miss out on our limited-time sale - invest in yourself today and embark on a journey of personal and professional growth. Enroll now and shape your future with knowledge that lasts a lifetime!".
For corporate trainings, projects, and real world experience reach us. We believe that education should be accessible to all, regardless of geographical location or background.
1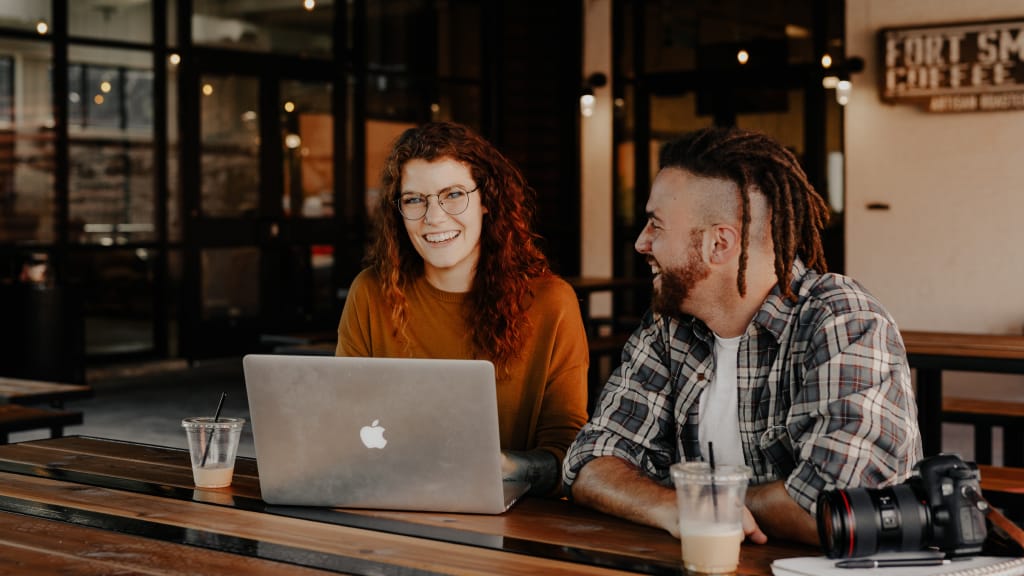
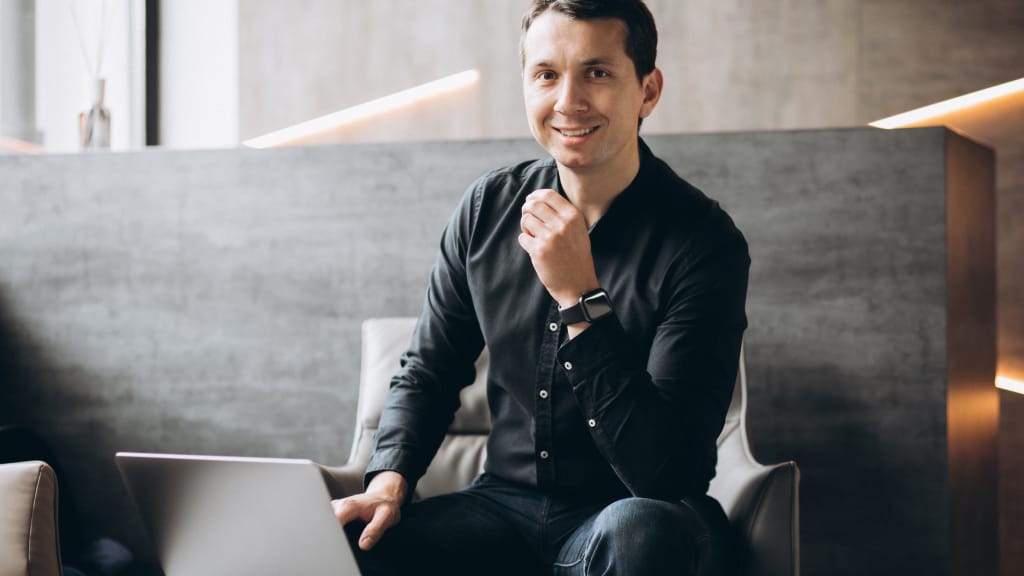
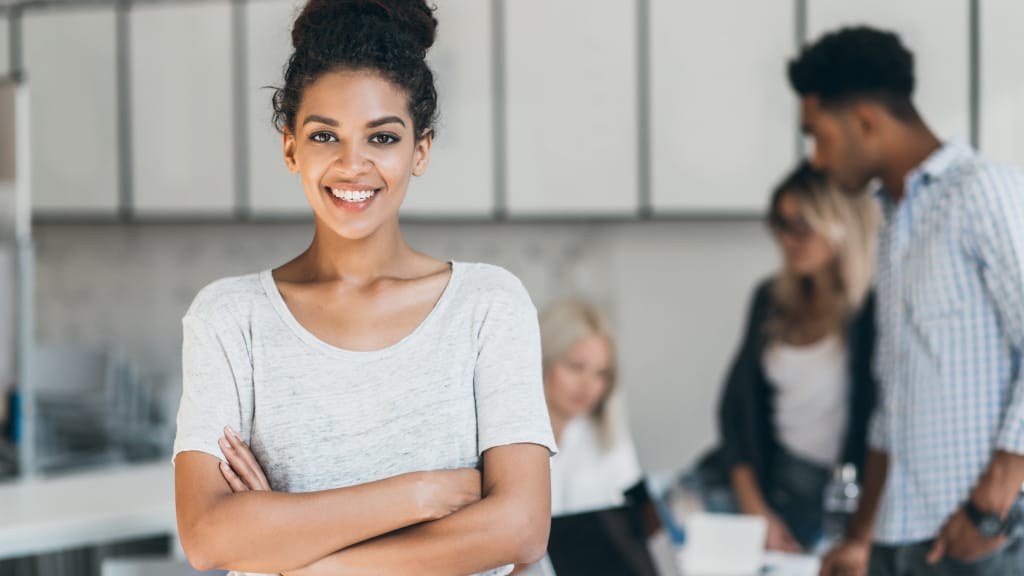
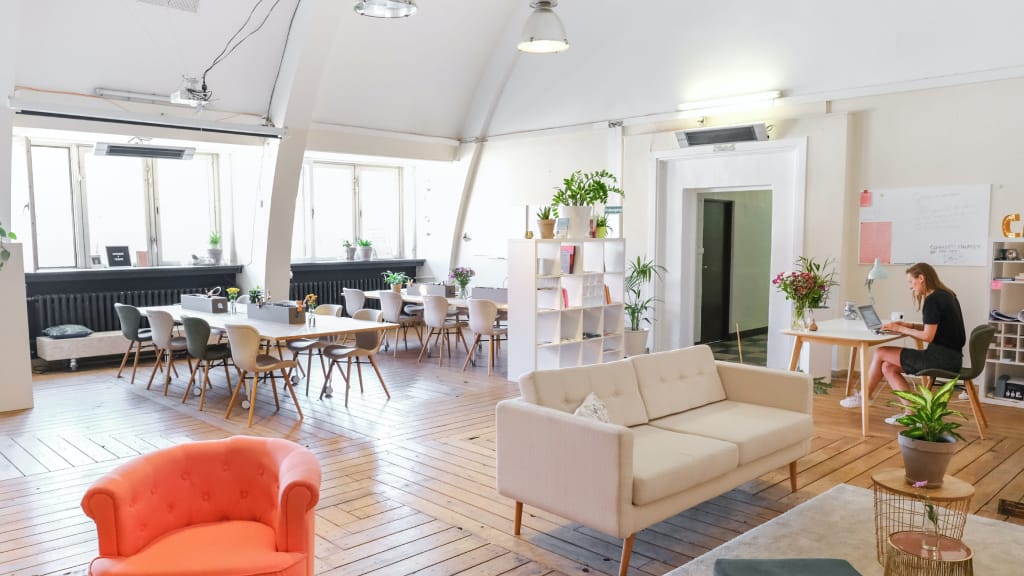
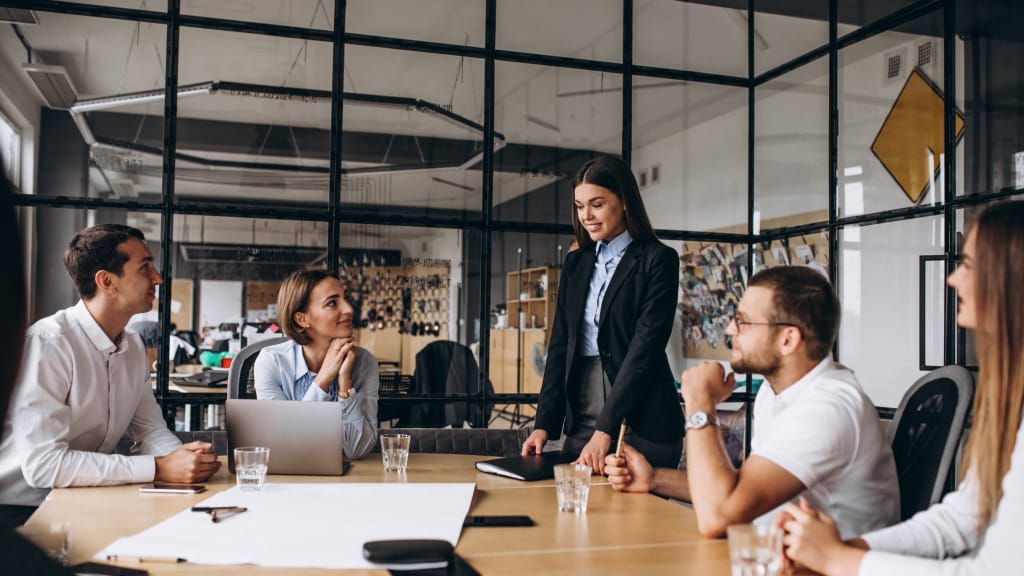
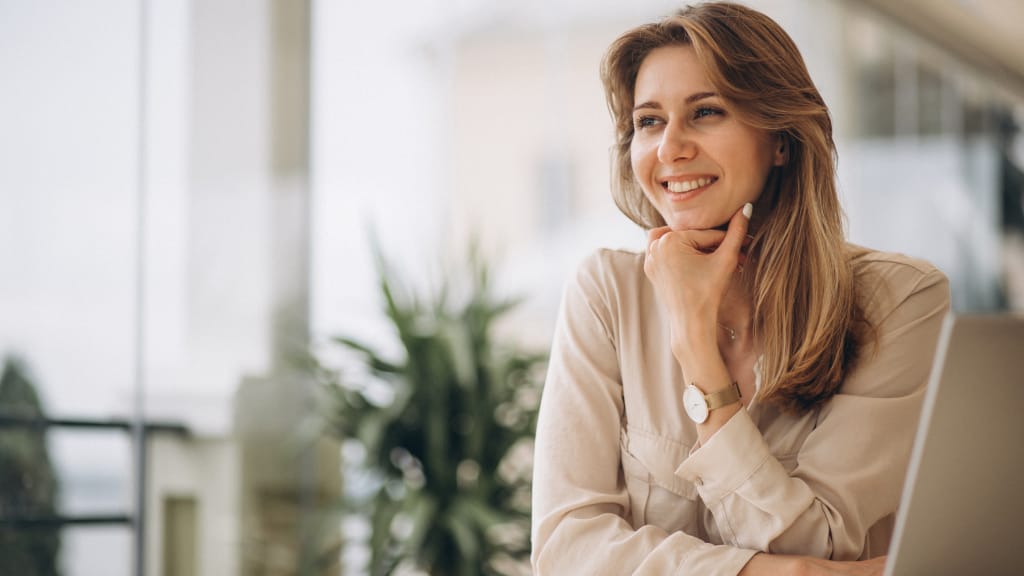
C++ Methods
In C++, methods are member functions of a class that define the behavior of objects. Methods allow you to perform actions, manipulate data, and interact with other objects. In this article, we will explore the usage of methods in C++ with examples.
1. Creating Methods
To create a method in C++, you define a member function within a class. Here's an example:
#include <iostream>
#include <string>
class Person {
private:
std::string name;
int age;
public:
void displayInfo() {
std::cout << "Name: " << name << std::endl;
std::cout << "Age: " << age << std::endl;
}
void setName(const std::string& _name) {
name = _name;
}
void setAge(int _age) {
age = _age;
}
};
int main() {
Person person;
person.setName("John Doe");
person.setAge(25);
person.displayInfo();
return 0;
}
In the above code, we define a class Person
with private member variables name
and age
. We create three methods: displayInfo()
, setName()
, and setAge()
. The displayInfo()
method outputs the person's name and age. The setName()
and setAge()
methods set the values of the corresponding member variables. In the main()
function, we create an object of the Person
class, set its name and age using the methods, and then display the information using the displayInfo()
method.
2. Passing Arguments to Methods
Methods can also accept arguments that allow you to pass data to them. Here's an example:
#include <iostream>
class Calculator {
public:
int add(int a, int b) {
return a + b;
}
int subtract(int a, int b) {
return a - b;
}
};
int main() {
Calculator calculator;
int sum = calculator.add(5, 3);
int difference = calculator.subtract(10, 7);
std::cout << "Sum: " << sum << std::endl;
std::cout << "Difference: " << difference << std::endl;
return 0;
}
In the above code, we define a class Calculator
with two methods: add()
and subtract()
. Both methods accept two integer arguments and return the respective calculations. In the main()
function, we create an object of the Calculator
class and call the methods, passing the appropriate arguments. The results are then displayed on the console.
3. Returning Values from Methods
Methods can also return values, allowing you to retrieve computed results. Here's an example:
#include <iostream>
class Square {
public:
int calculateArea(int side) {
return side * side;
}
};
int main() {
Square square;
int area = square.calculateArea(5);
std::cout << "Area: " << area << std::endl;
return 0;
}
In the above code, we define a class Square
with a method calculateArea()
that accepts the length of a side and returns the area of the square. In the main()
function, we create an object of the Square
class and call the calculateArea()
method, passing the side length. The computed area is then displayed on the console.
Methods in C++ provide a way to encapsulate behavior within objects. They allow you to perform actions, manipulate data, and interact with other objects. Utilize methods to design classes and define the behaviors of your objects in a structured and modular manner.
4.5L
Learners
20+
Instructors
50+
Courses
6.0L
Course enrollments
Future Trending Courses
When selecting, a course, Here are a few areas that are expected to be in demand in the future:.
Future Learning for all
If you’re passionate and ready to dive in, we’d love to join 1:1 classes for you. We’re committed to support our learners and professionals their development and well-being.
View Courses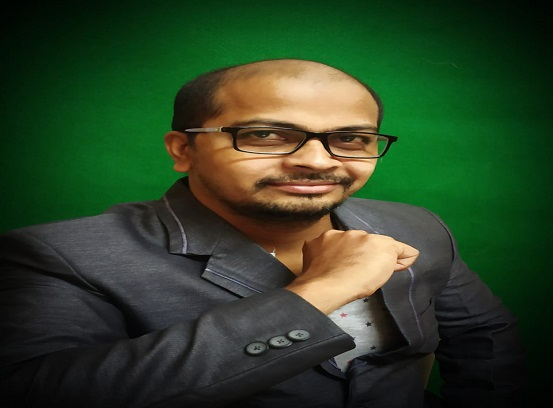
Most Popular Course topics
These are the most popular course topics among Software Courses for learners