Hi there, we’re Harisystems
"Unlock your potential and soar to new heights with our exclusive online courses! Ignite your passion, acquire valuable skills, and embrace limitless possibilities. Don't miss out on our limited-time sale - invest in yourself today and embark on a journey of personal and professional growth. Enroll now and shape your future with knowledge that lasts a lifetime!".
For corporate trainings, projects, and real world experience reach us. We believe that education should be accessible to all, regardless of geographical location or background.
1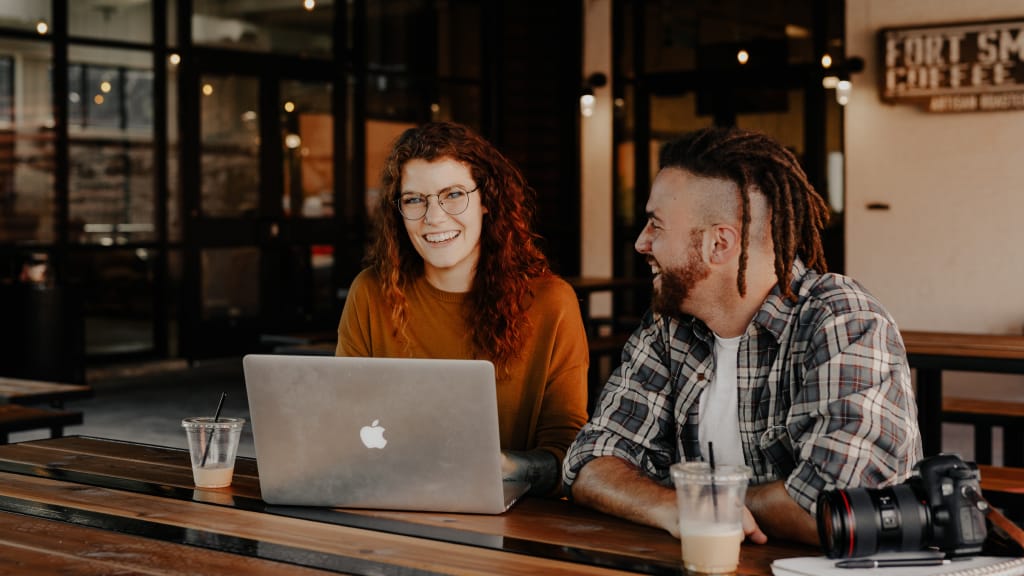
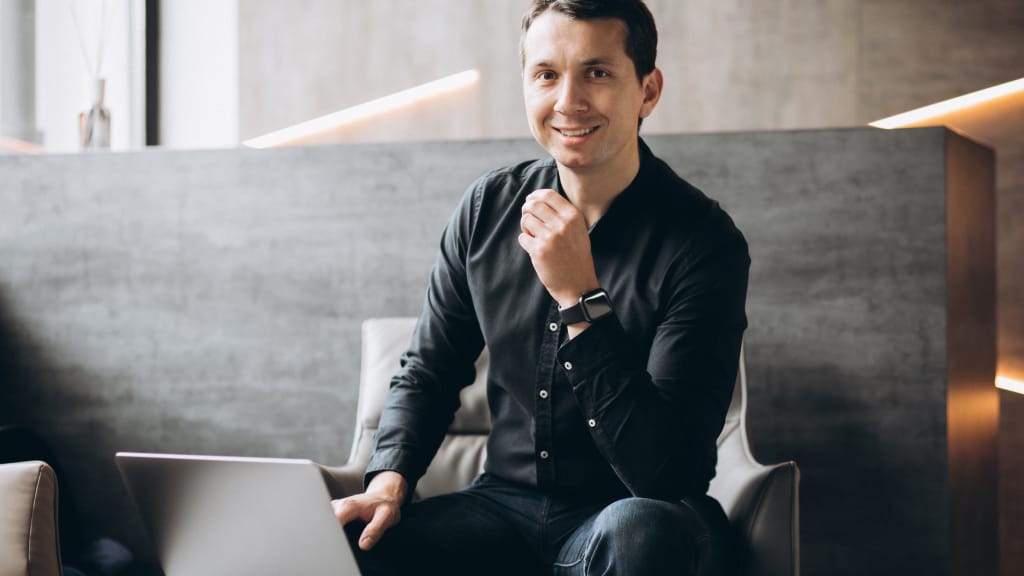
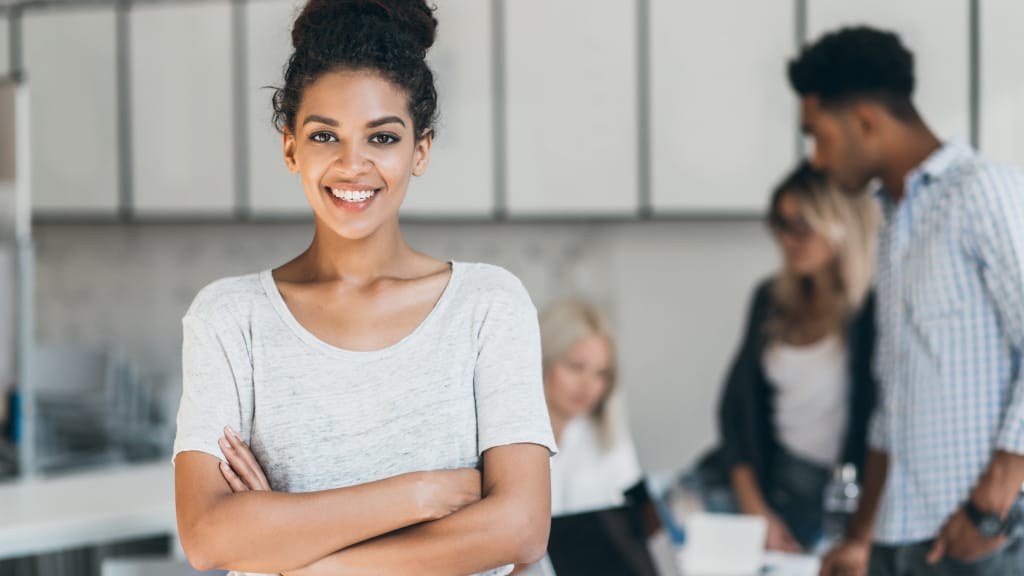
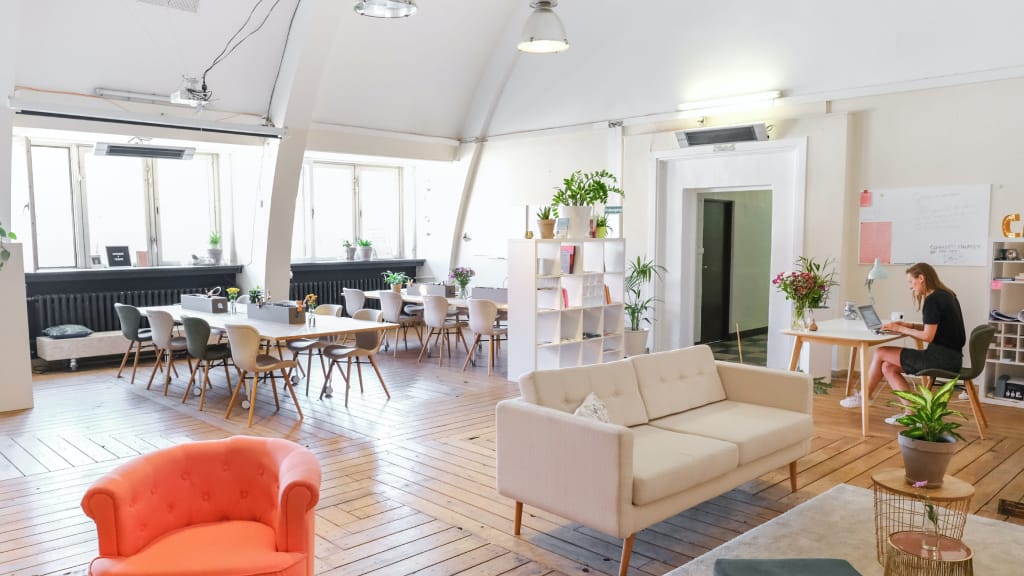
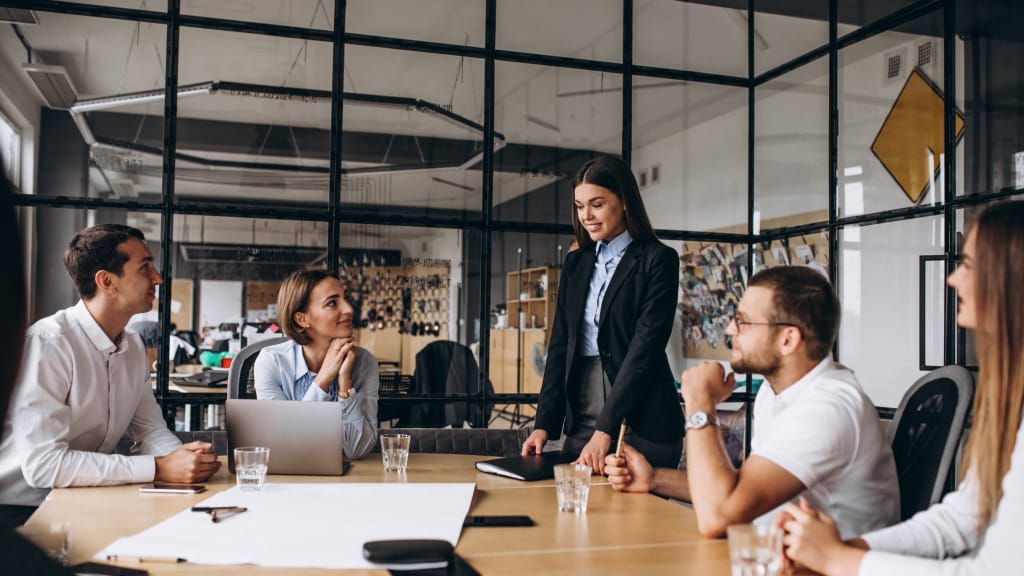
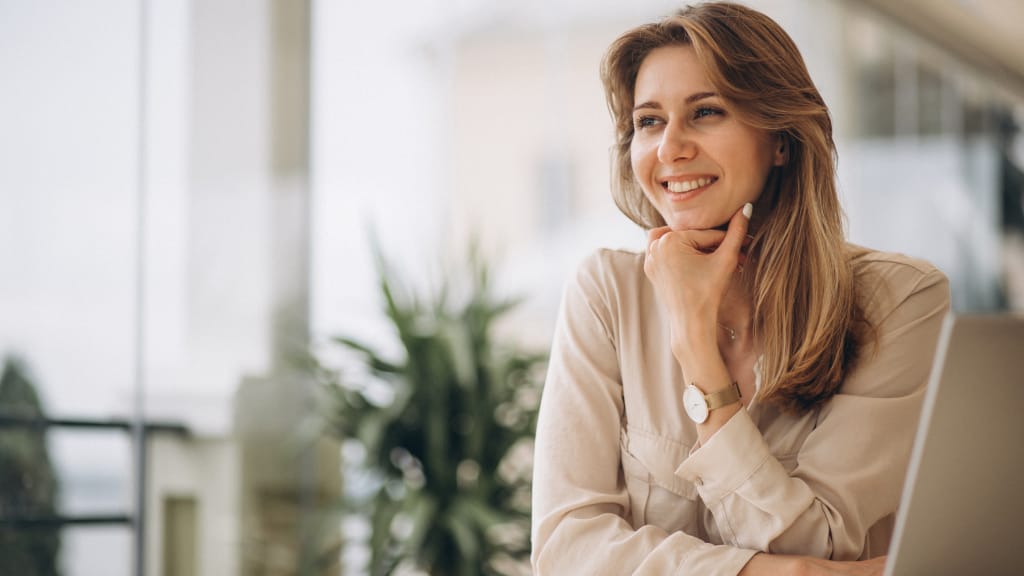
Java Inheritance: Creating Hierarchies of Classes
Inheritance is a fundamental concept in object-oriented programming (OOP) that allows you to create hierarchies of classes. In Java, inheritance enables a class to inherit properties and behaviors from another class, forming a parent-child relationship. In this article, we will explore inheritance in Java and provide examples to help you understand its usage.
Defining a Parent Class
In Java, a parent class, also known as a base class or superclass, is the class from which other classes inherit properties and behaviors. To define a parent class, you can create a regular class with attributes and methods. Here's an example:
public class Animal {
// Attributes
protected String name;
protected int age;
// Constructor
public Animal(String name, int age) {
this.name = name;
this.age = age;
}
// Method
public void eat() {
System.out.println(name + " is eating.");
}
}
In the above code, we define a parent class named Animal
with two attributes: name
and age
. We provide a constructor to initialize these attributes, and a method named eat
to represent the common behavior of animals. The protected
access modifier allows subclasses to access these attributes.
Inheriting from a Parent Class
To create a child class that inherits from a parent class, you use the extends
keyword followed by the name of the parent class. Here's an example:
public class Dog extends Animal {
// Additional attributes
private String breed;
// Constructor
public Dog(String name, int age, String breed) {
super(name, age);
this.breed = breed;
}
// Additional method
public void bark() {
System.out.println(name + " is barking.");
}
}
In the above code, we define a child class named Dog
that extends the Animal
class. The Dog
class inherits the attributes name
and age
from the Animal
class. It also adds an additional attribute breed
. We provide a constructor that calls the parent class constructor using the super
keyword and initializes the breed
attribute. The Dog
class also adds a method bark
to represent the unique behavior of dogs.
Creating Objects and Accessing Inherited Members
To create objects of the child class and access both the inherited members and the additional members, you can do the following:
Dog dog = new Dog("Buddy", 3, "Labrador");
dog.eat(); // Inherited from Animal class
dog.bark(); // Defined in Dog class
System.out.println(dog.name); // Inherited from Animal class
System.out.println(dog.breed); // Unique to Dog class
In the above code, we create an instance of the Dog
class called dog
and provide values for its attributes using the constructor. We can access the inherited method eat
and call the additional method bark
. We can also access the inherited attribute name
and the additional attribute breed
.
Benefits of Inheritance
Inheritance offers several benefits in Java programming:
- Code reusability: Inheritance allows you to reuse the attributes and methods defined in a parent class, reducing code duplication and promoting code organization.
- Polymorphism: Inheritance enables you to treat objects of a child class as objects of the parent class, facilitating polymorphic behavior and code flexibility.
- Hierarchy and specialization: Inheritance allows you to create hierarchies of classes, organizing related classes based on their similarities and differences. Child classes can specialize and add unique attributes and behaviors to the parent class.
Conclusion
Inheritance is a powerful concept in Java that allows you to create hierarchies of classes and inherit properties and behaviors from a parent class. In this article, we explored how to define a parent class and create a child class that inherits from it. We discussed the benefits of inheritance, such as code reusability, polymorphism, and hierarchy. By effectively utilizing inheritance, you can create more organized, maintainable, and extensible code in Java. Continuously practice working with inheritance and explore more advanced topics, such as method overriding and abstract classes, to further enhance your object-oriented programming skills.
4.5L
Learners
20+
Instructors
50+
Courses
6.0L
Course enrollments
Future Trending Courses
When selecting, a course, Here are a few areas that are expected to be in demand in the future:.
Future Learning for all
If you’re passionate and ready to dive in, we’d love to join 1:1 classes for you. We’re committed to support our learners and professionals their development and well-being.
View Courses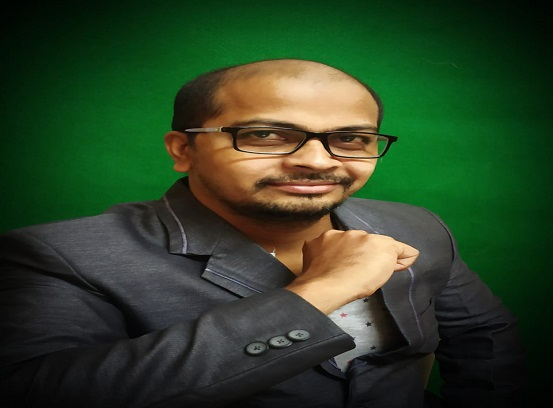
Most Popular Course topics
These are the most popular course topics among Software Courses for learners