Hi there, we’re Harisystems
"Unlock your potential and soar to new heights with our exclusive online courses! Ignite your passion, acquire valuable skills, and embrace limitless possibilities. Don't miss out on our limited-time sale - invest in yourself today and embark on a journey of personal and professional growth. Enroll now and shape your future with knowledge that lasts a lifetime!".
For corporate trainings, projects, and real world experience reach us. We believe that education should be accessible to all, regardless of geographical location or background.
1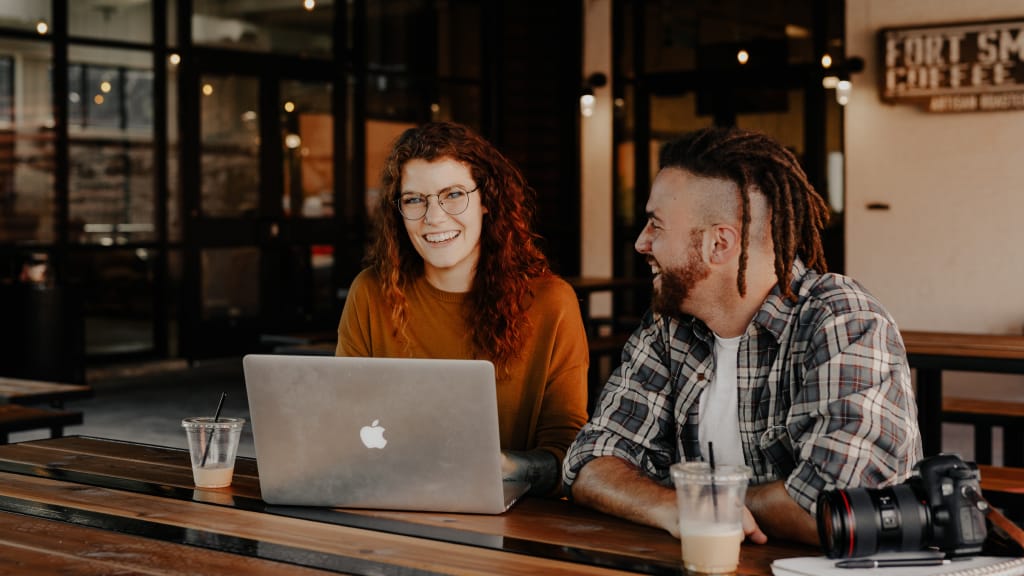
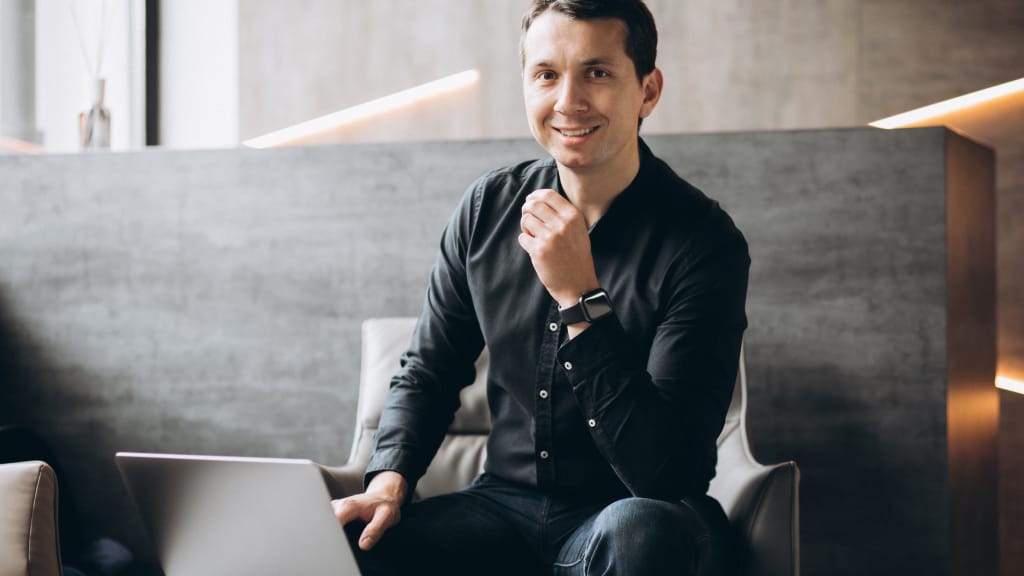
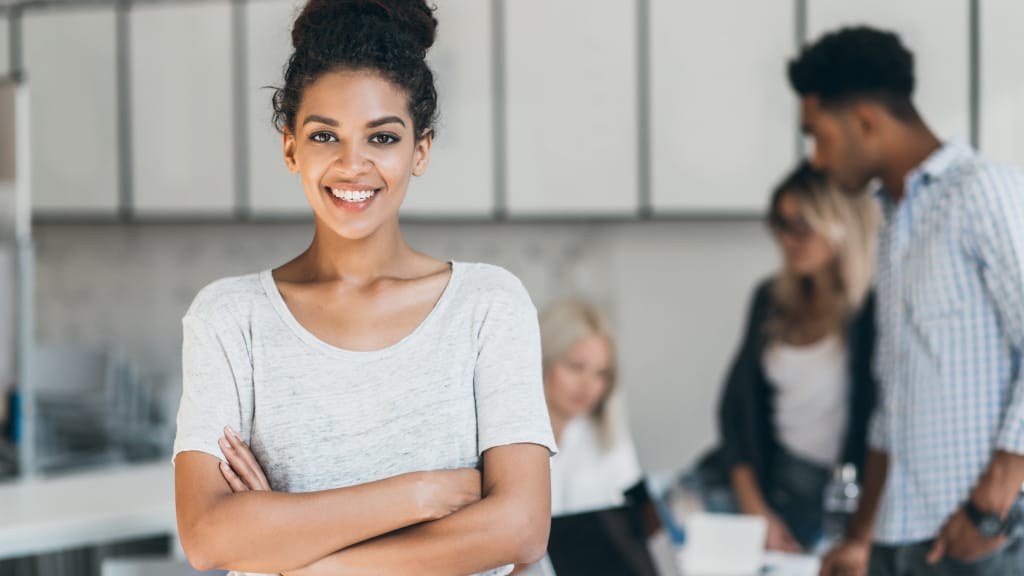
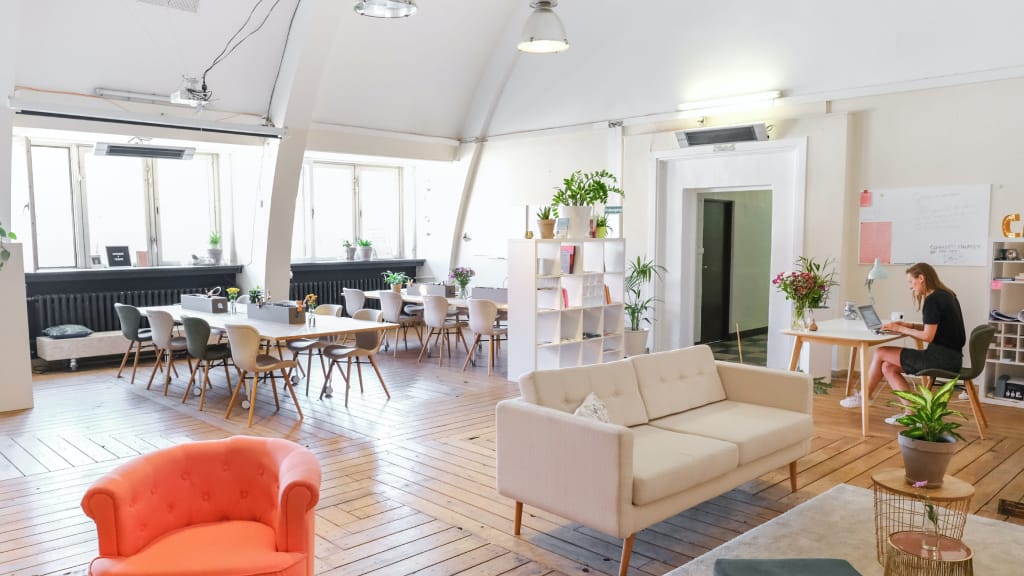
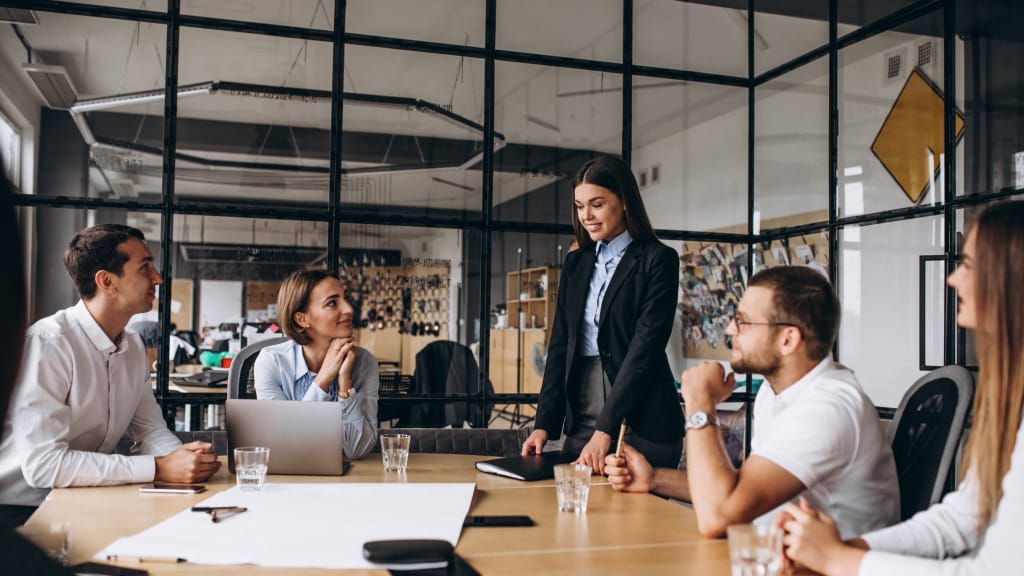
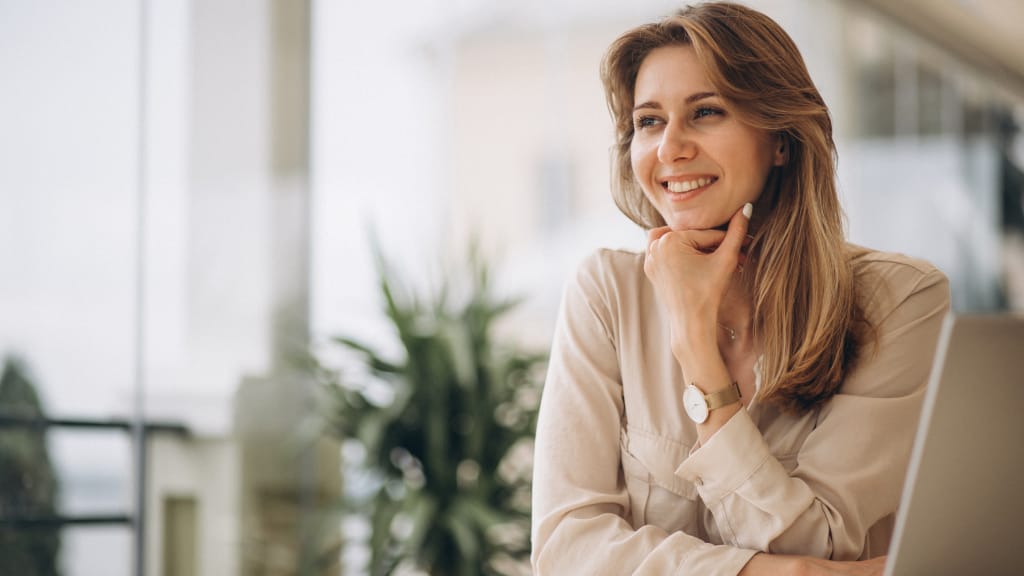
Python for Artificial Intelligence
Python has become one of the most popular programming languages for developing artificial intelligence (AI) applications. Its simplicity, readability, and extensive library ecosystem make it an ideal choice for AI tasks such as machine learning, deep learning, natural language processing, and more. In this article, we'll explore how Python can be used for AI and provide example code snippets to showcase its capabilities.
Example 1: Machine Learning with Scikit-learn
Scikit-learn is a widely-used Python library for machine learning. Here's an example of using Scikit-learn to build a simple classification model:
from sklearn.datasets import load_iris
from sklearn.model_selection import train_test_split
from sklearn.neighbors import KNeighborsClassifier
# Load the Iris dataset
iris = load_iris()
X, y = iris.data, iris.target
# Split the dataset into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Create a K-Nearest Neighbors classifier
knn = KNeighborsClassifier(n_neighbors=3)
# Train the classifier
knn.fit(X_train, y_train)
# Make predictions on the test set
predictions = knn.predict(X_test)
# Evaluate the accuracy of the model
accuracy = sum(predictions == y_test) / len(y_test)
print("Accuracy:", accuracy)
In this code, we import the necessary modules from Scikit-learn. We load the famous Iris dataset, split it into training and testing sets, and create a K-Nearest Neighbors classifier. We then train the classifier on the training data and make predictions on the test set. Finally, we evaluate the accuracy of the model by comparing the predicted labels with the actual labels.
Example 2: Deep Learning with TensorFlow
TensorFlow is a powerful Python library for deep learning. Here's an example of using TensorFlow to build a simple neural network:
import tensorflow as tf
# Define the model architecture
model = tf.keras.Sequential([
tf.keras.layers.Dense(64, activation='relu', input_shape=(784,)),
tf.keras.layers.Dense(64, activation='relu'),
tf.keras.layers.Dense(10, activation='softmax')
])
# Compile the model
model.compile(optimizer='adam',
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
# Load and preprocess the data
mnist = tf.keras.datasets.mnist
(x_train, y_train), (x_test, y_test) = mnist.load_data()
x_train, x_test = x_train / 255.0, x_test / 255.0
# Train the model
model.fit(x_train, y_train, epochs=5)
# Evaluate the model
loss, accuracy = model.evaluate(x_test, y_test)
print("Loss:", loss)
print("Accuracy:", accuracy)
In this code, we define a sequential model using TensorFlow's Keras API. The model consists of three dense layers: two hidden layers with ReLU activation and an output layer with softmax activation. We compile the model with the Adam optimizer and sparse categorical crossentropy loss. We then load and preprocess the MNIST dataset, train the model on the training data, and evaluate its performance on the test data by calculating the loss and accuracy.
Example 3: Natural Language Processing with NLTK
NLTK (Natural Language Toolkit) is a Python library for working with human language data. Here's an example of using NLTK to perform sentiment analysis on text:
import nltk
from nltk.sentiment import SentimentIntensityAnalyzer
# Initialize the sentiment analyzer
sia = SentimentIntensityAnalyzer()
# Analyze the sentiment of a text
text = "I love using Python for AI projects!"
sentiment = sia.polarity_scores(text)
# Print the sentiment scores
print("Positive:", sentiment['pos'])
print("Negative:", sentiment['neg'])
print("Neutral:", sentiment['neu'])
In this code, we import the necessary modules from NLTK. We initialize a SentimentIntensityAnalyzer object, which allows us to analyze the sentiment of a given text. We provide a sample text and use the polarity_scores method to calculate the sentiment scores, which include positive, negative, and neutral values. We then print these scores to get insights into the sentiment of the text.
Conclusion
Python's versatility and rich ecosystem of libraries make it an excellent choice for artificial intelligence projects. Whether you're working on machine learning, deep learning, natural language processing, or other AI tasks, Python provides the tools and resources you need. The examples provided in this article are just a glimpse of what you can achieve with Python in the field of artificial intelligence. Feel free to explore these examples further and dive deeper into the exciting world of AI with Python!
4.5L
Learners
20+
Instructors
50+
Courses
6.0L
Course enrollments
Future Trending Courses
When selecting, a course, Here are a few areas that are expected to be in demand in the future:.
Future Learning for all
If you’re passionate and ready to dive in, we’d love to join 1:1 classes for you. We’re committed to support our learners and professionals their development and well-being.
View Courses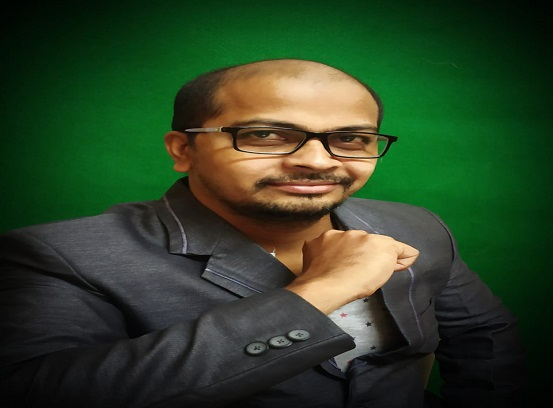
Most Popular Course topics
These are the most popular course topics among Software Courses for learners