Hi there, we’re Harisystems
"Unlock your potential and soar to new heights with our exclusive online courses! Ignite your passion, acquire valuable skills, and embrace limitless possibilities. Don't miss out on our limited-time sale - invest in yourself today and embark on a journey of personal and professional growth. Enroll now and shape your future with knowledge that lasts a lifetime!".
For corporate trainings, projects, and real world experience reach us. We believe that education should be accessible to all, regardless of geographical location or background.
1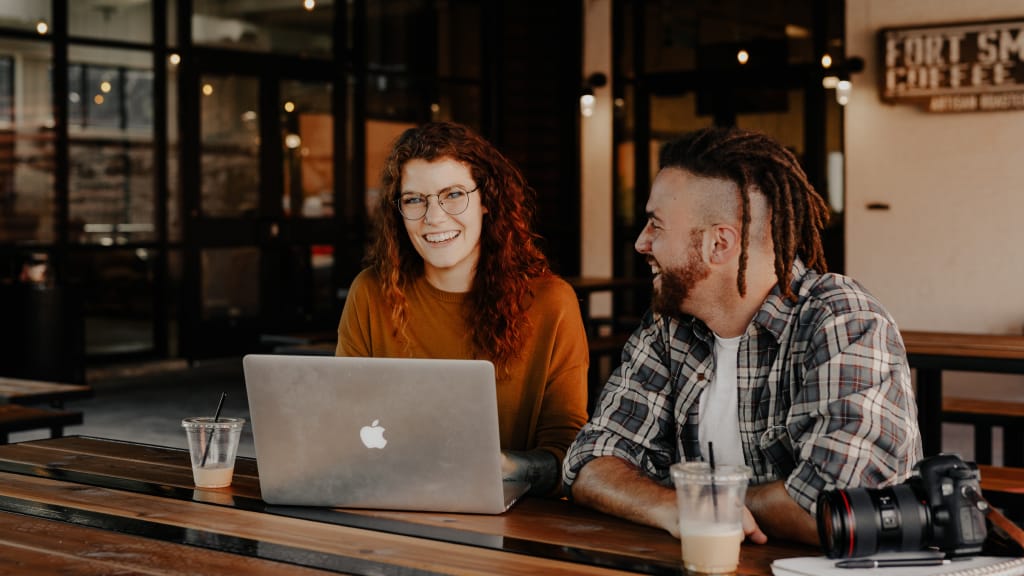
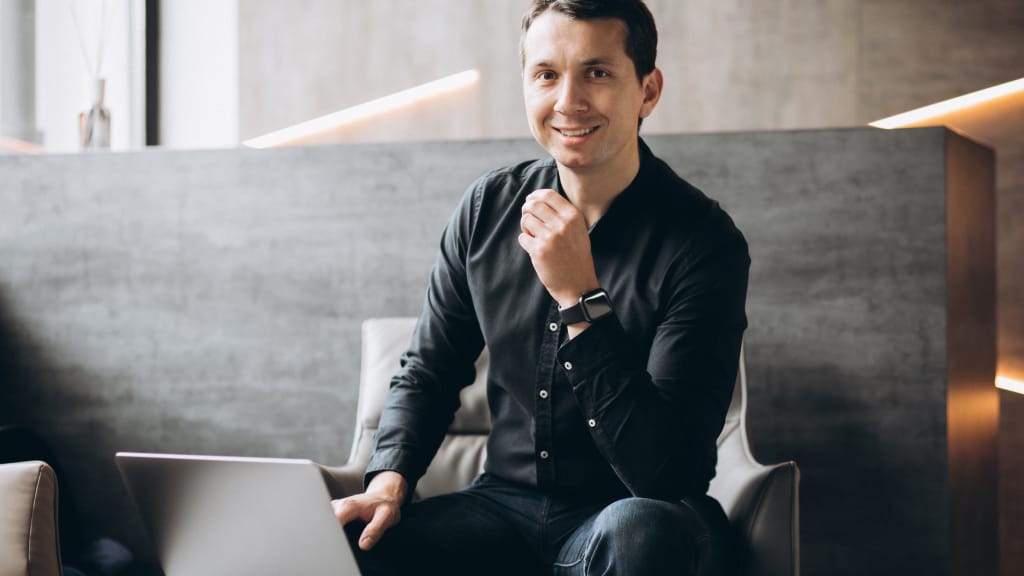
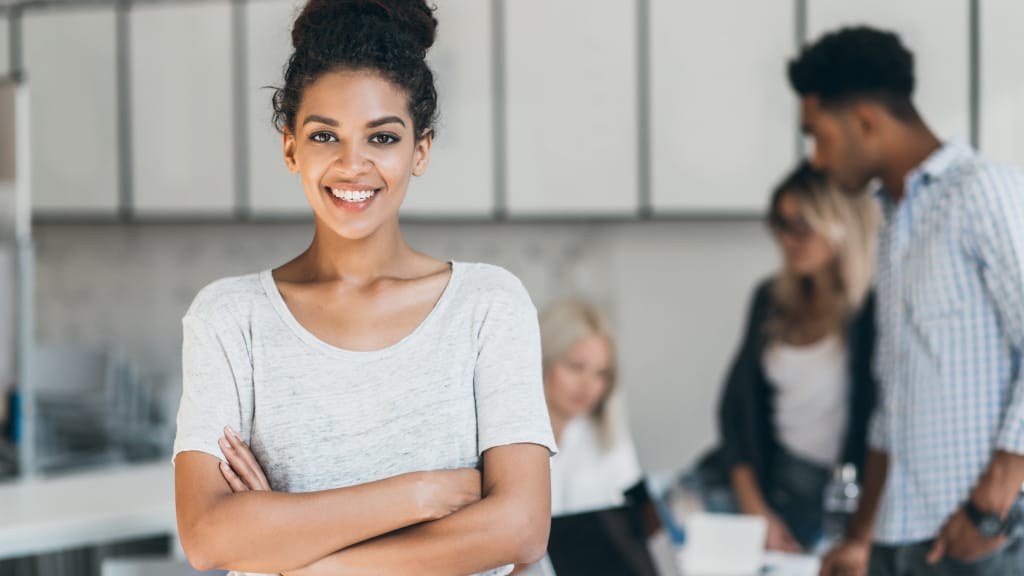
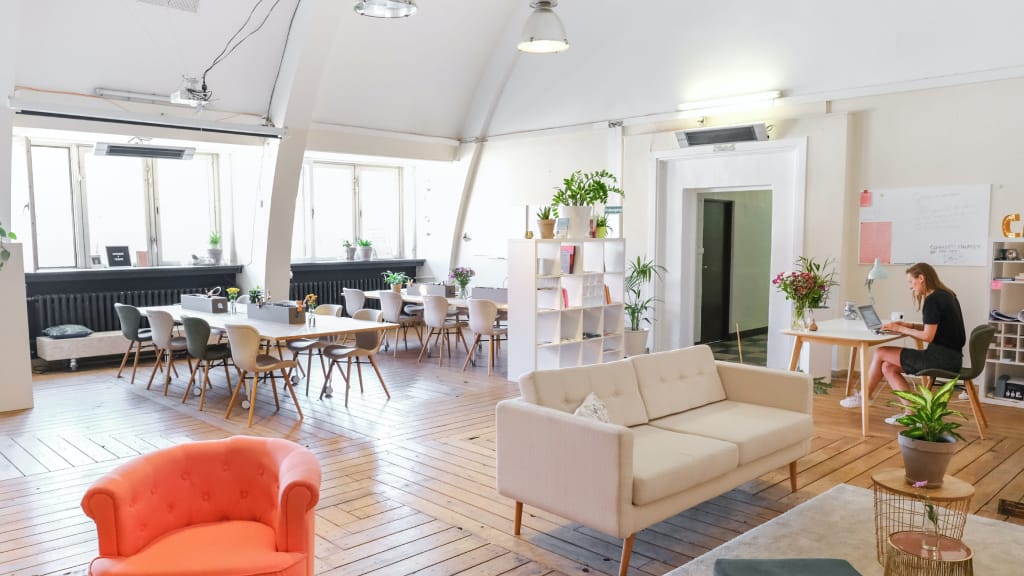
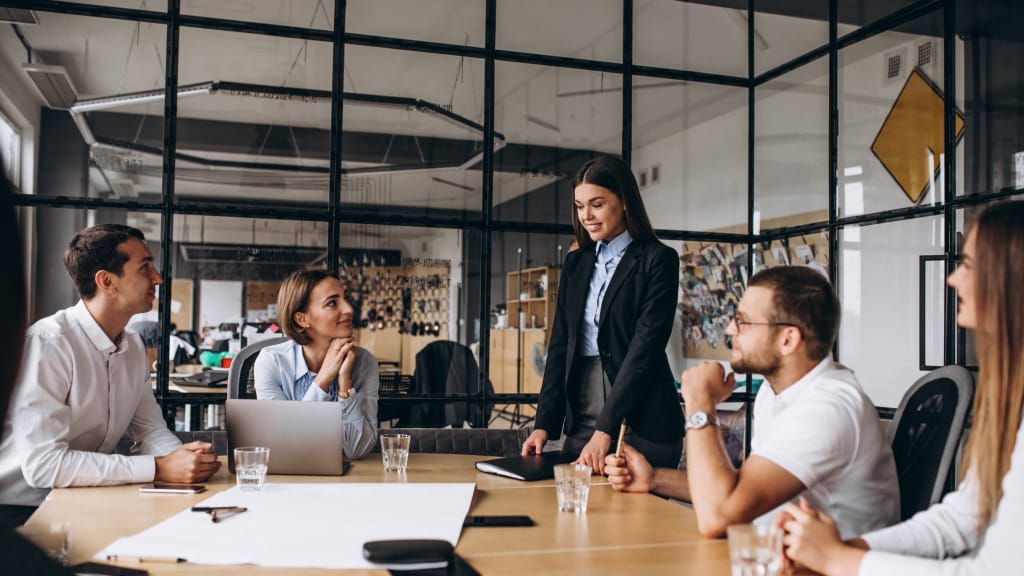
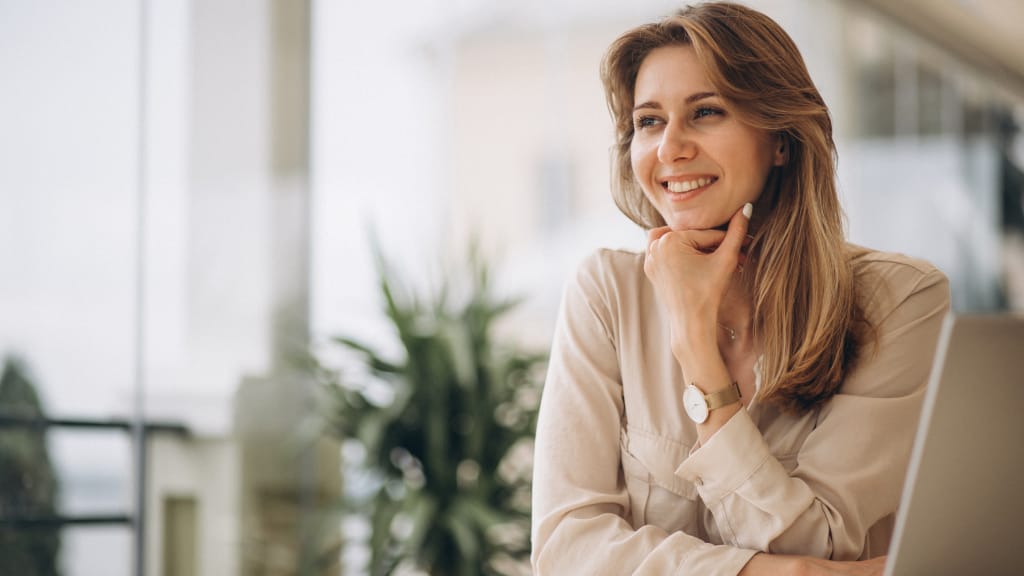
Python for Machine Learning
Python has become the go-to programming language for machine learning tasks. Its simplicity, extensive libraries, and powerful frameworks make it an ideal choice for building and deploying machine learning models. In this article, we'll explore how Python can be used for machine learning and provide example code snippets to showcase its capabilities.
Example 1: Linear Regression with scikit-learn
scikit-learn is a popular Python library for machine learning. Here's an example of using scikit-learn to build a simple linear regression model:
import numpy as np
from sklearn.linear_model import LinearRegression
# Create sample input data
X = np.array([[1], [2], [3], [4], [5]])
y = np.array([2, 4, 6, 8, 10])
# Create a linear regression model
model = LinearRegression()
# Train the model
model.fit(X, y)
# Make predictions
X_test = np.array([[6], [7], [8]])
predictions = model.predict(X_test)
# Print the predictions
print(predictions)
In this code, we import numpy for array manipulation and the LinearRegression class from scikit-learn. We create sample input data X and corresponding output data y. We then create a LinearRegression model, train it using the fit method, and make predictions on new input data X_test using the predict method. Finally, we print the predictions.
Example 2: Image Classification with TensorFlow
TensorFlow is a popular Python library for deep learning. Here's an example of using TensorFlow to build an image classification model:
import tensorflow as tf
from tensorflow.keras.datasets import mnist
# Load the MNIST dataset
(X_train, y_train), (X_test, y_test) = mnist.load_data()
# Preprocess the data
X_train = X_train / 255.0
X_test = X_test / 255.0
# Create a deep learning model
model = tf.keras.models.Sequential([
tf.keras.layers.Flatten(input_shape=(28, 28)),
tf.keras.layers.Dense(128, activation='relu'),
tf.keras.layers.Dense(10, activation='softmax')
])
# Compile the model
model.compile(optimizer='adam',
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
# Train the model
model.fit(X_train, y_train, epochs=5)
# Evaluate the model
loss, accuracy = model.evaluate(X_test, y_test)
print("Loss:", loss)
print("Accuracy:", accuracy)
In this code, we import TensorFlow and load the MNIST dataset, which consists of handwritten digit images. We preprocess the data by dividing the pixel values by 255 to scale them between 0 and 1. We then create a deep learning model using the Sequential API, which includes a flatten layer, a dense layer with ReLU activation, and an output layer with softmax activation. We compile the model with the Adam optimizer and sparse categorical cross-entropy loss. We train the model on the training data and evaluate its performance on the test data, printing the loss and accuracy metrics.
Example 3: Clustering with scikit-learn
scikit-learn provides various algorithms for clustering tasks. Here's an example of using scikit-learn to perform K-means clustering:
import numpy as np
from sklearn.cluster import KMeans
# Create sample data
X = np.array([[1, 2], [1, 4], [1, 0], [4, 2], [4, 4], [4, 0]])
# Create a K-means clustering model
model = KMeans(n_clusters=2)
# Perform clustering
model.fit(X)
# Get the cluster labels
labels = model.labels_
# Print the cluster labels
print(labels)
In this code, we import numpy and the KMeans class from scikit-learn. We create sample data X consisting of 2-dimensional points. We create a KMeans model with 2 clusters and fit it to the data using the fit method. We then retrieve the cluster labels using the labels_ attribute and print them.
Conclusion
Python provides a powerful and versatile environment for machine learning tasks. The examples presented in this article demonstrate Python's capabilities in linear regression, deep learning for image classification, and clustering. Whether you're just starting with machine learning or already have experience, Python's rich ecosystem of libraries and frameworks, such as scikit-learn and TensorFlow, will enable you to build and deploy sophisticated machine learning models. Feel free to explore these examples further and leverage Python for your machine learning projects!
4.5L
Learners
20+
Instructors
50+
Courses
6.0L
Course enrollments
Future Trending Courses
When selecting, a course, Here are a few areas that are expected to be in demand in the future:.
Future Learning for all
If you’re passionate and ready to dive in, we’d love to join 1:1 classes for you. We’re committed to support our learners and professionals their development and well-being.
View Courses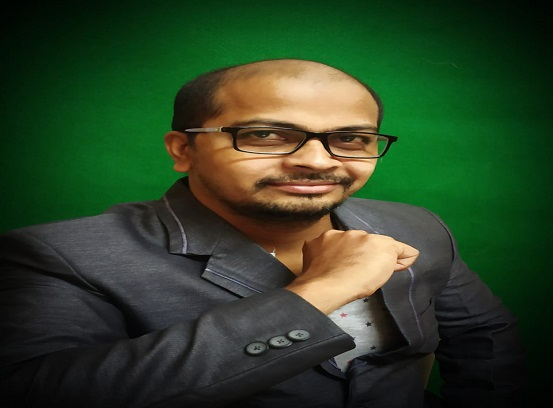
Most Popular Course topics
These are the most popular course topics among Software Courses for learners