Hi there, we’re Harisystems
"Unlock your potential and soar to new heights with our exclusive online courses! Ignite your passion, acquire valuable skills, and embrace limitless possibilities. Don't miss out on our limited-time sale - invest in yourself today and embark on a journey of personal and professional growth. Enroll now and shape your future with knowledge that lasts a lifetime!".
For corporate trainings, projects, and real world experience reach us. We believe that education should be accessible to all, regardless of geographical location or background.
1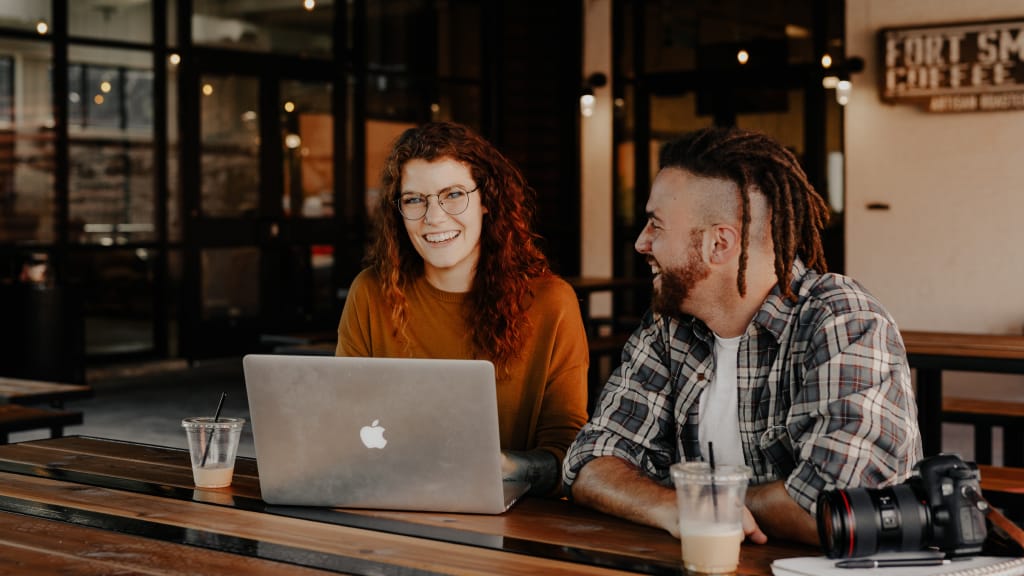
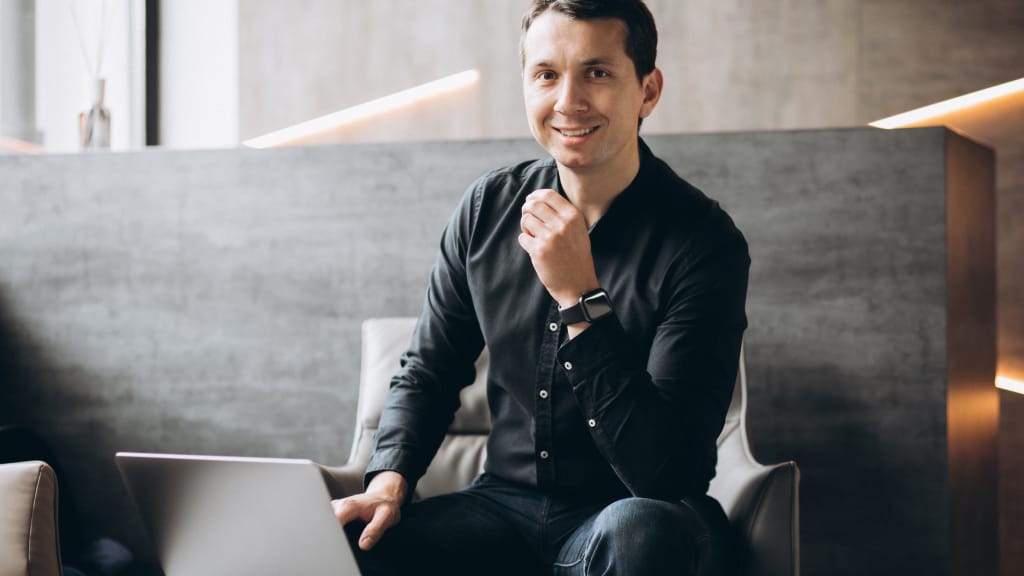
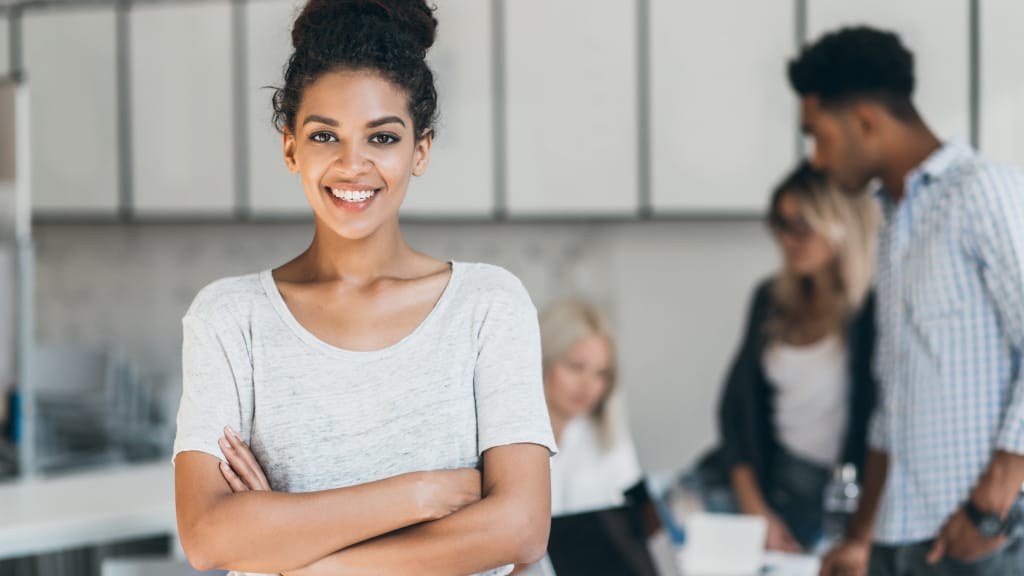
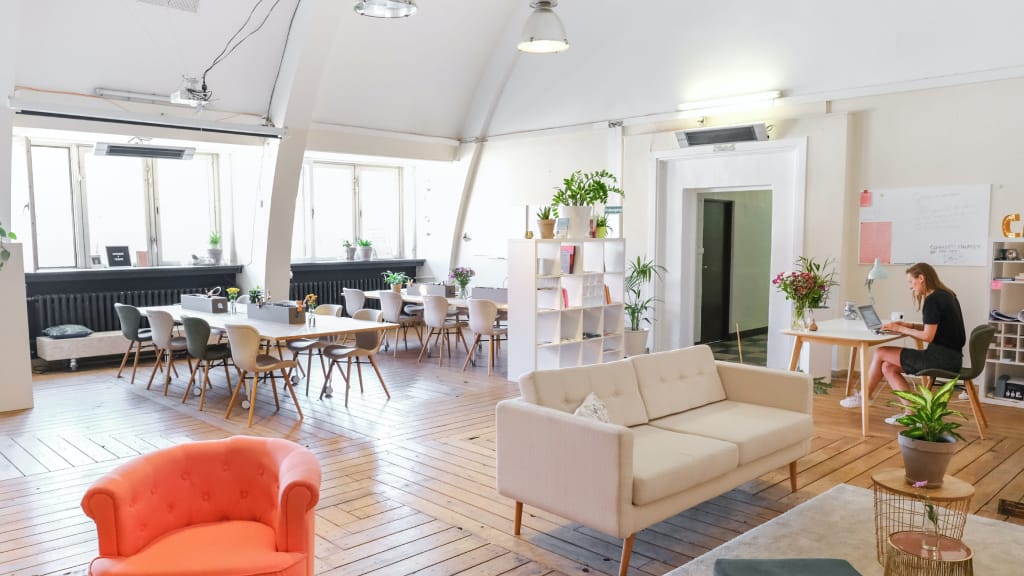
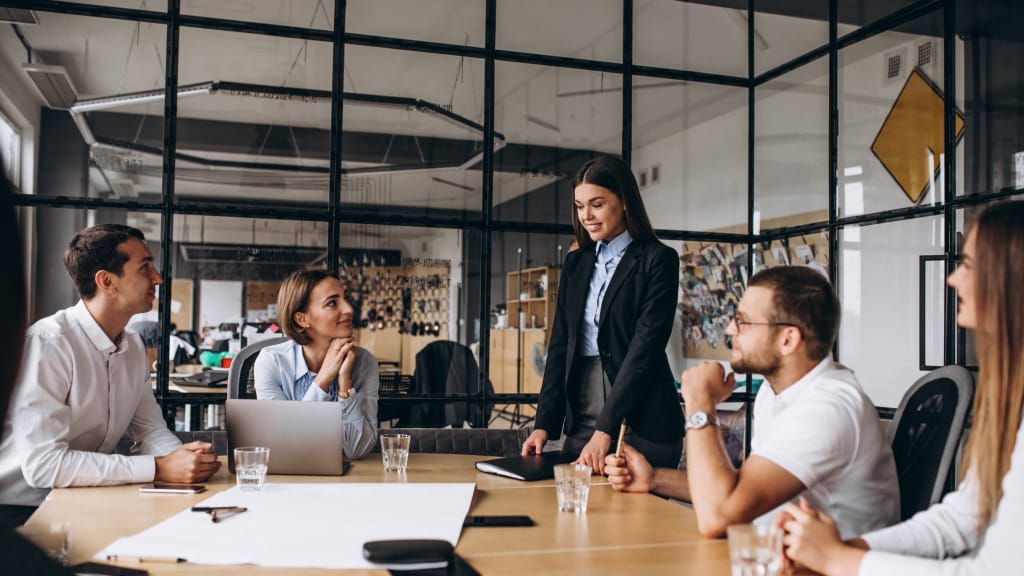
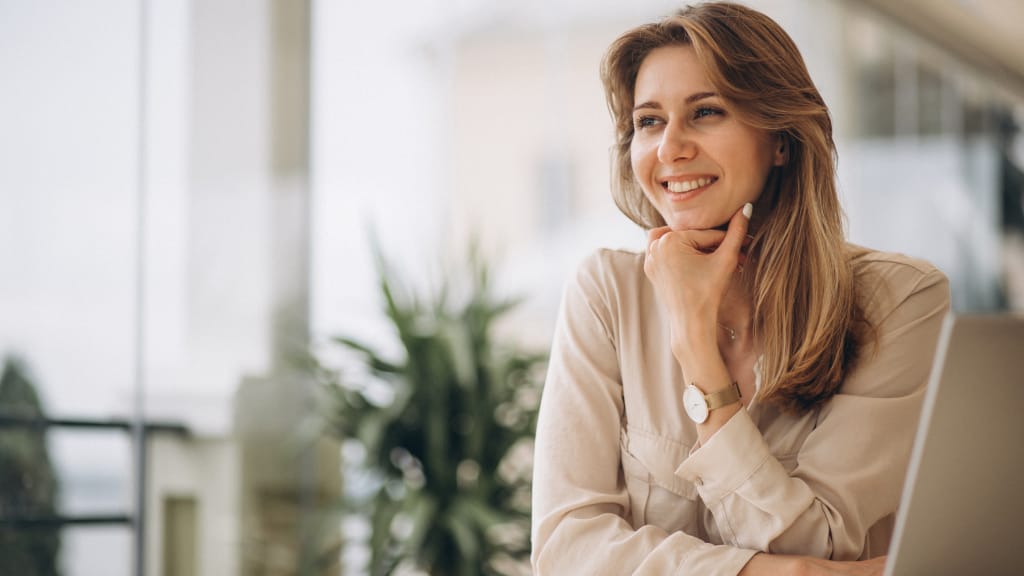
JavaScript Variables: Understanding and Usage with Examples
Variables are fundamental to programming as they allow us to store and manipulate data. In JavaScript, variables play a crucial role in storing values of different data types. Let's explore JavaScript variables and see how they are used with examples.
Declaring Variables
In JavaScript, we can declare variables using the 'var', 'let', or 'const' keywords. Here's an example of declaring a variable using 'var':
var age = 25;
In this example, we declare a variable named 'age' and assign it the value of 25. The 'var' keyword is used for declaring variables that have function scope or global scope.
With the introduction of ES6, the 'let' and 'const' keywords were introduced. 'let' is used to declare variables with block scope, while 'const' is used to declare constants that cannot be reassigned. Here's an example using 'let':
let name = "John Doe";
In this case, we declare a variable named 'name' and assign it the value "John Doe". The variable 'name' is accessible within the block where it is declared.
Using Variables
Once we have declared a variable, we can use it in our JavaScript code. Variables can store various data types, such as numbers, strings, booleans, arrays, and objects. Here are a few examples:
var age = 25;
let name = "John Doe";
const PI = 3.14;
var isStudent = true;
var grades = [90, 85, 95];
var person = { firstName: "John", lastName: "Doe" };
In the examples above, 'age' stores a number, 'name' stores a string, 'PI' stores a constant value, 'isStudent' stores a boolean, 'grades' stores an array, and 'person' stores an object.
Modifying Variables
Variables in JavaScript can be modified by assigning new values to them. Let's see an example:
var counter = 0;
counter = counter + 1;
console.log(counter); // Output: 1
In this example, we initialize 'counter' with the value 0. Then we modify the 'counter' variable by adding 1 to its current value. The updated value of 'counter' is then printed to the console.
Variable Scope
JavaScript variables have different scopes based on how and where they are declared. Variables declared with 'var' have function scope or global scope, while variables declared with 'let' or 'const' have block scope.
function sayHello() { var message = "Hello!"; console.log(message); } sayHello(); // Output: Hello! console.log(message); // Error: message is not defined
pre>In this example, the 'message' variable is declared within the 'sayHello()' function. It is accessible only within that function. Trying to access it outside the function will result in an error.
Understanding variables and their scopes is essential for writing clean and maintainable JavaScript code.
4.5L
Learners
20+
Instructors
50+
Courses
6.0L
Course enrollments
Future Trending Courses
When selecting, a course, Here are a few areas that are expected to be in demand in the future:.
Future Learning for all
If you’re passionate and ready to dive in, we’d love to join 1:1 classes for you. We’re committed to support our learners and professionals their development and well-being.
View Courses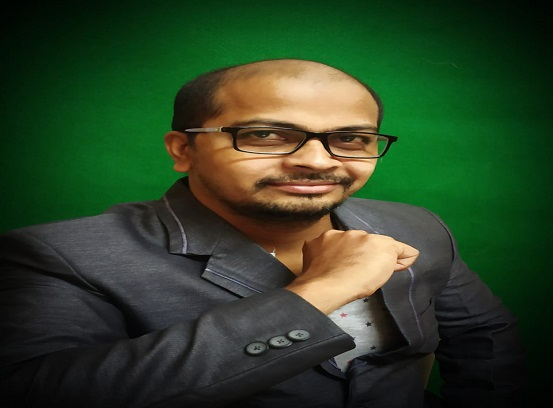
Most Popular Course topics
These are the most popular course topics among Software Courses for learners