Hi there, we’re Harisystems
"Unlock your potential and soar to new heights with our exclusive online courses! Ignite your passion, acquire valuable skills, and embrace limitless possibilities. Don't miss out on our limited-time sale - invest in yourself today and embark on a journey of personal and professional growth. Enroll now and shape your future with knowledge that lasts a lifetime!".
For corporate trainings, projects, and real world experience reach us. We believe that education should be accessible to all, regardless of geographical location or background.
1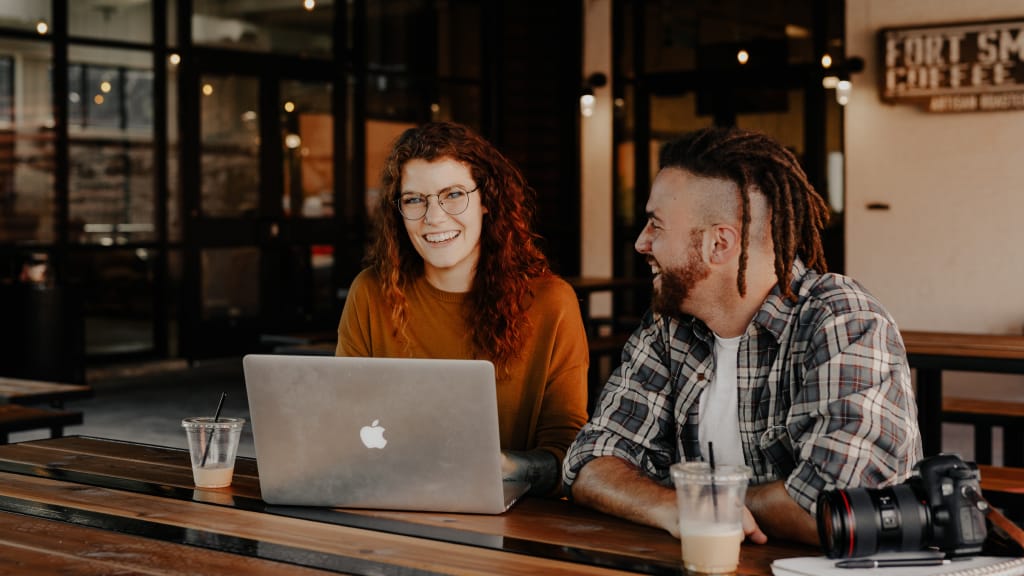
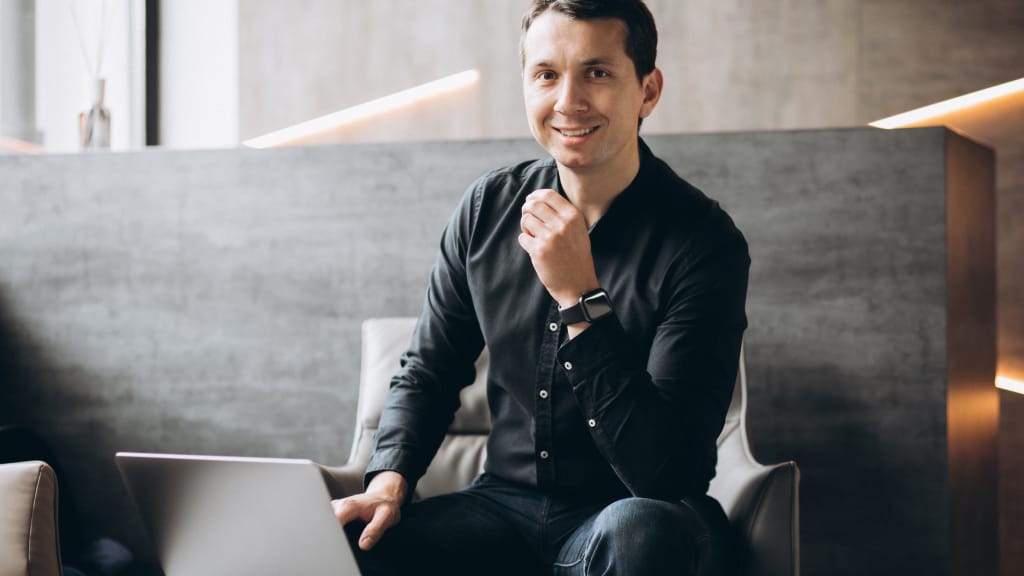
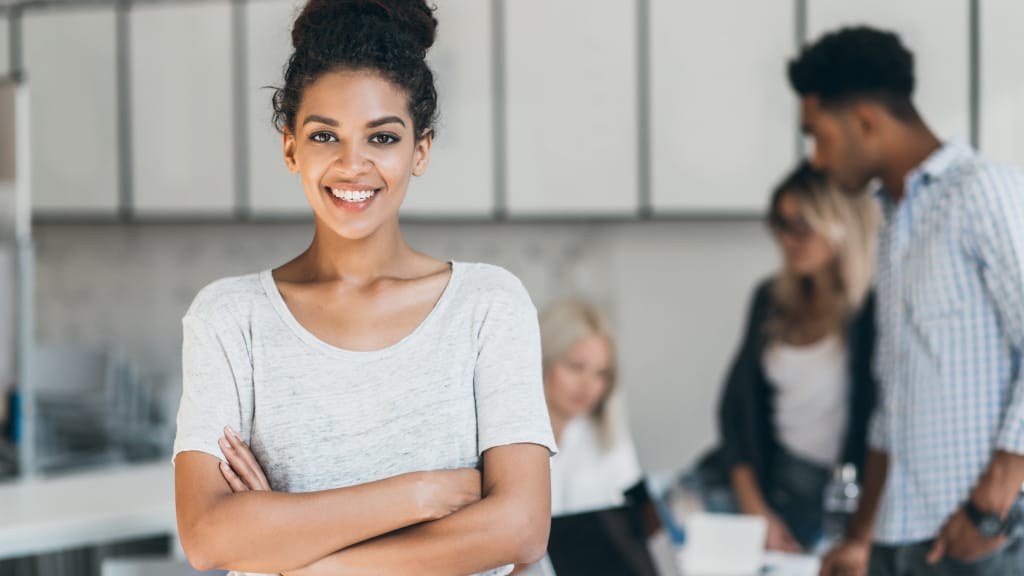
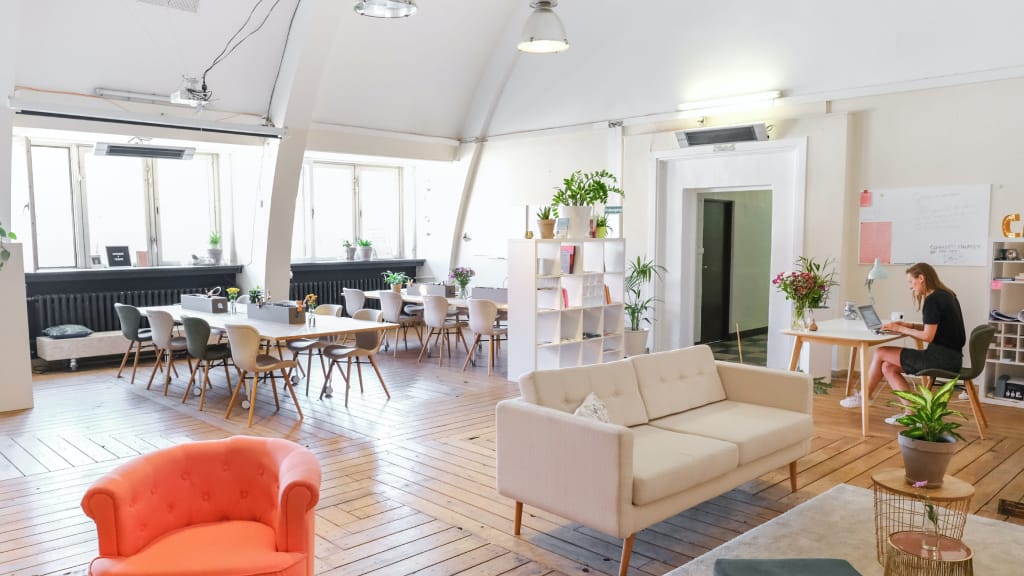
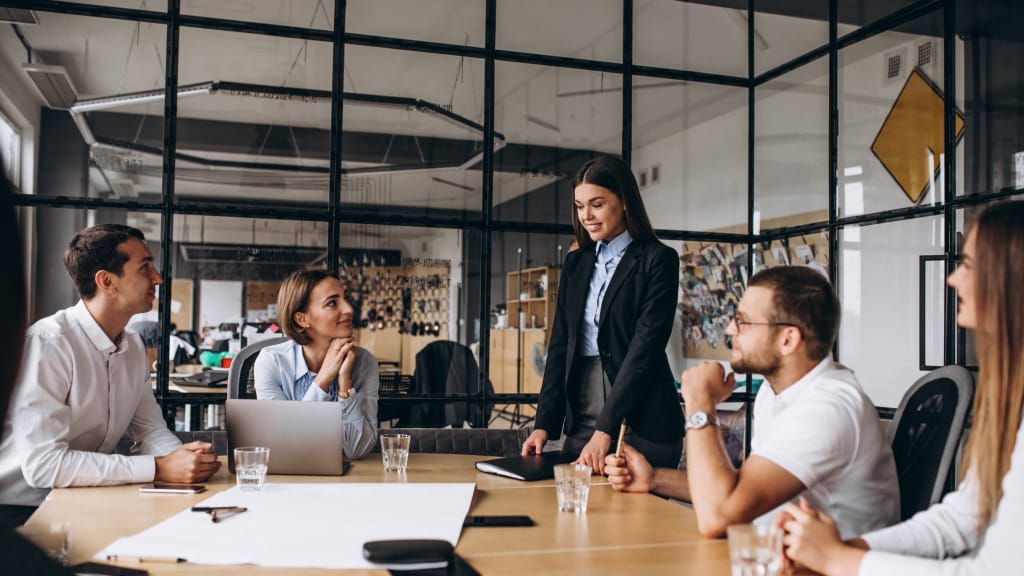
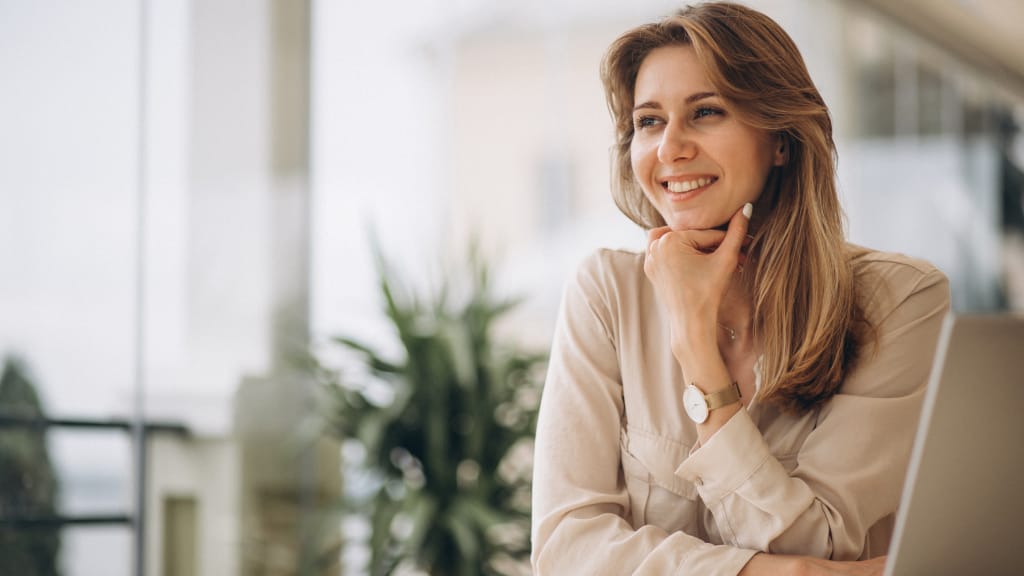
Java While Loop: Repeating Code Blocks
In Java, the while
loop is used to repeat a code block as long as a given condition is true. It allows you to execute a set of statements repeatedly until the condition becomes false. The while
loop is useful when you want to perform an action multiple times without knowing the exact number of iterations in advance. In this article, we will explore the while
loop in Java and provide examples to help you understand its usage.
The while Loop Syntax
The syntax of the while
loop in Java is as follows:
while (condition) {
// code to be executed
}
The condition
is a boolean expression that determines whether the loop should continue or terminate. If the condition
is true, the code block within the while
loop is executed. After each iteration, the condition
is evaluated again. If the condition
becomes false, the loop terminates, and the program continues executing the code following the loop.
Example: Counting Numbers
Let's consider an example where we want to count from 1 to 5 using a while
loop:
int count = 1;
while (count <= 5) {
System.out.println(count);
count++;
}
In the above code, the while
loop continues as long as the count
is less than or equal to 5. The program prints the value of count
and increments it by 1 after each iteration. The output of the above code will be:
1
2
3
4
5
Example: User Input Validation
The while
loop is often used for input validation, where you repeatedly ask the user for input until a valid value is provided. Here's an example:
import java.util.Scanner;
Scanner scanner = new Scanner(System.in);
int age = -1;
while (age < 0) {
System.out.print("Enter your age: ");
age = scanner.nextInt();
if (age < 0) {
System.out.println("Invalid age. Please enter a positive value.");
}
}
System.out.println("Your age is: " + age);
In the above code, the program asks the user to enter their age. If the entered age is less than 0, it displays an error message and asks for input again. The loop continues until the user enters a valid, non-negative age. The program then displays the entered age.
Conclusion
The while
loop in Java provides a way to repeat a code block based on a condition. In this article, we explored the syntax of the while
loop and provided examples to demonstrate its usage. We saw how to count numbers and perform input validation using a while
loop. By utilizing the while
loop effectively, you can create powerful iterative algorithms and control the flow of your program based on dynamic conditions. Continuously practice using while
loops and explore more advanced topics, such as do-while
loops and nested loops, to enhance your control flow capabilities in Java programming.
4.5L
Learners
20+
Instructors
50+
Courses
6.0L
Course enrollments
Future Trending Courses
When selecting, a course, Here are a few areas that are expected to be in demand in the future:.
Future Learning for all
If you’re passionate and ready to dive in, we’d love to join 1:1 classes for you. We’re committed to support our learners and professionals their development and well-being.
View Courses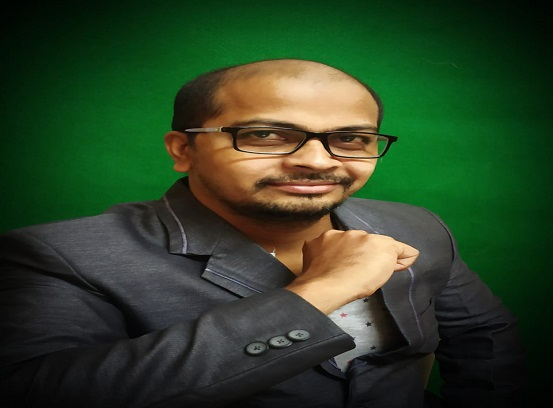
Most Popular Course topics
These are the most popular course topics among Software Courses for learners