Hi there, we’re Harisystems
"Unlock your potential and soar to new heights with our exclusive online courses! Ignite your passion, acquire valuable skills, and embrace limitless possibilities. Don't miss out on our limited-time sale - invest in yourself today and embark on a journey of personal and professional growth. Enroll now and shape your future with knowledge that lasts a lifetime!".
For corporate trainings, projects, and real world experience reach us. We believe that education should be accessible to all, regardless of geographical location or background.
1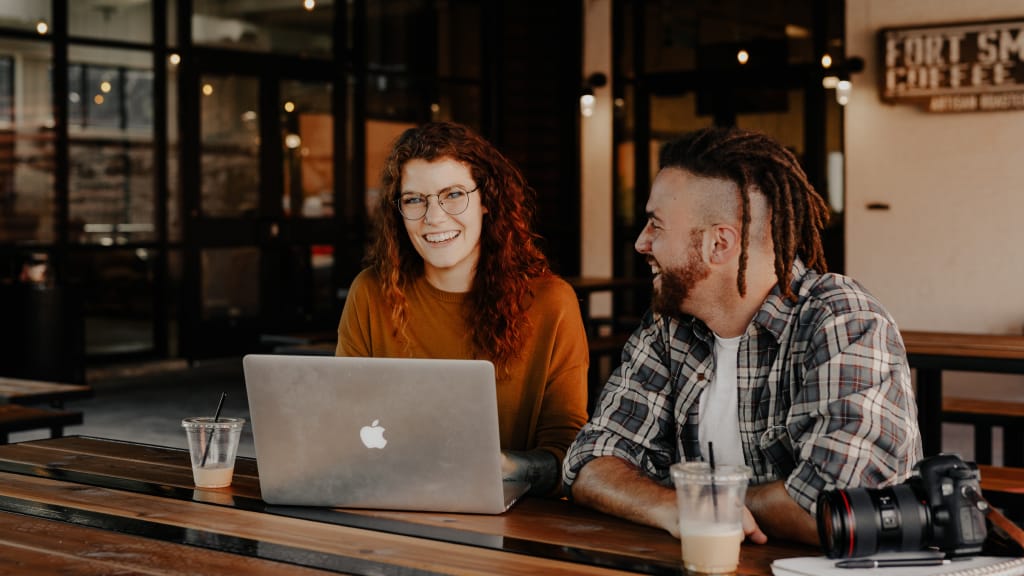
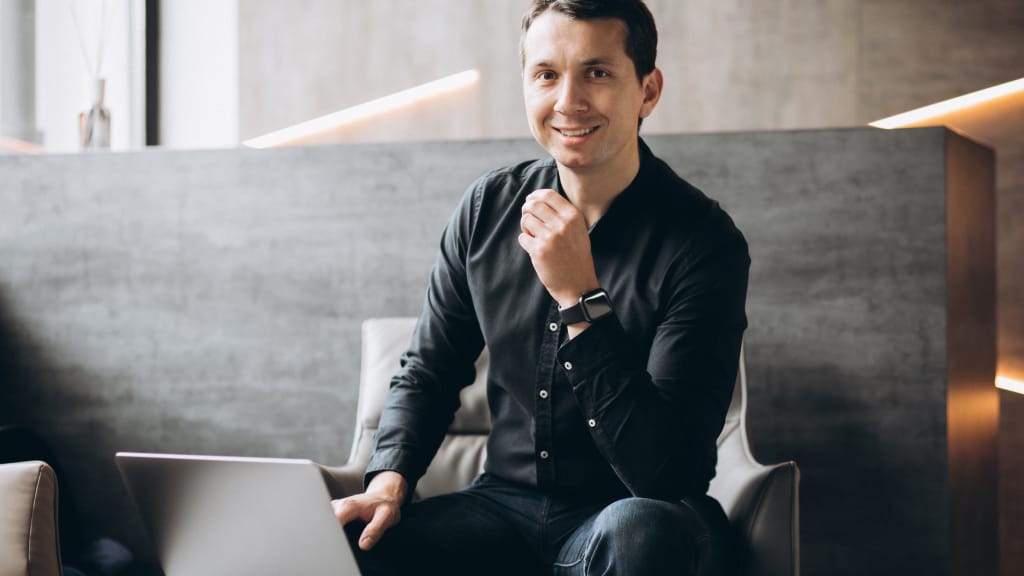
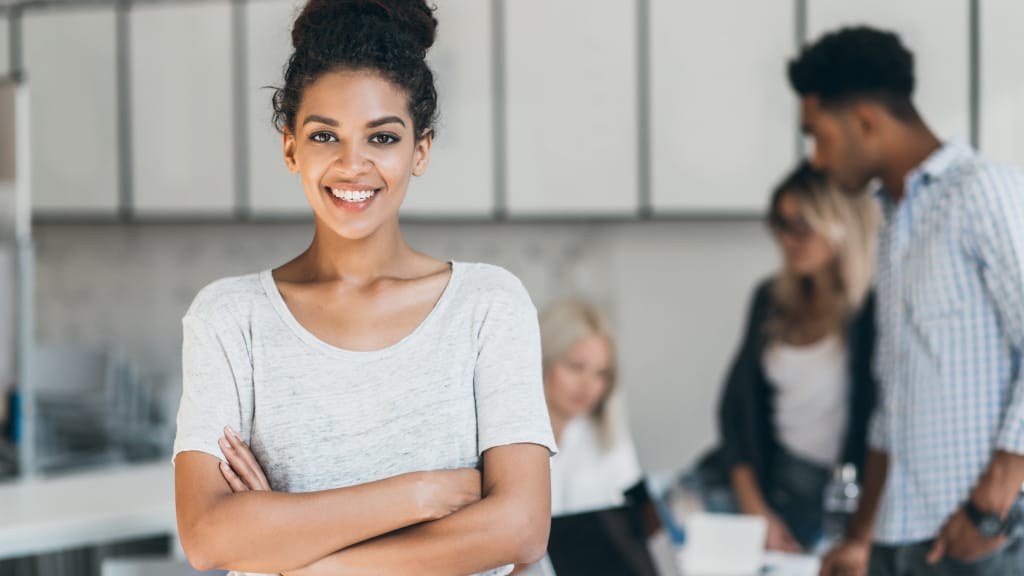
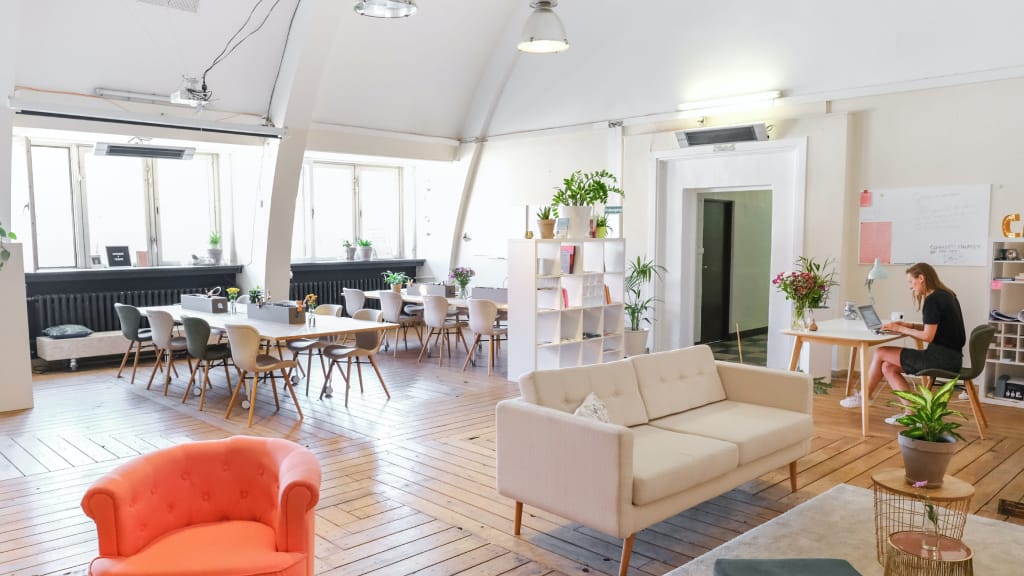
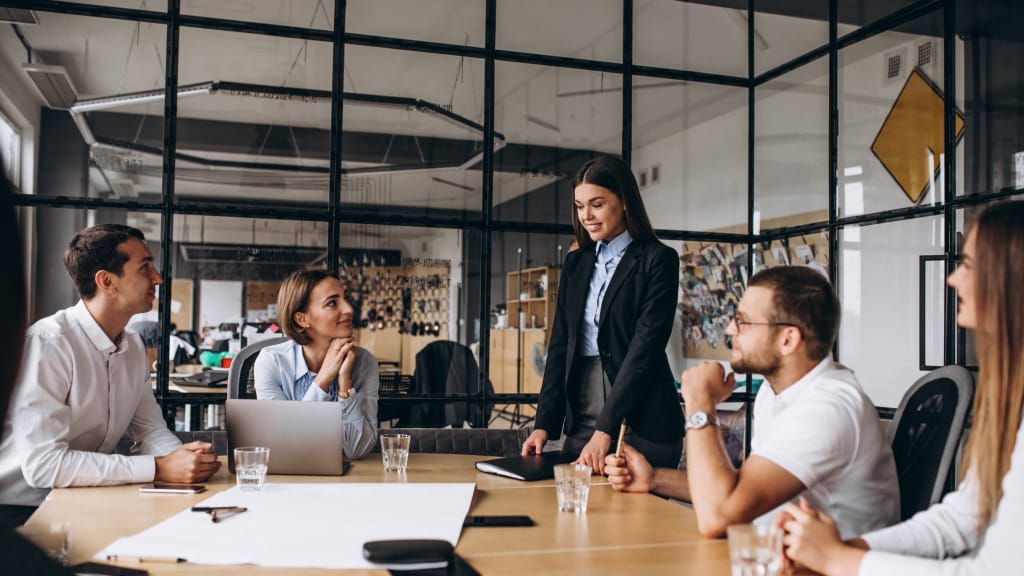
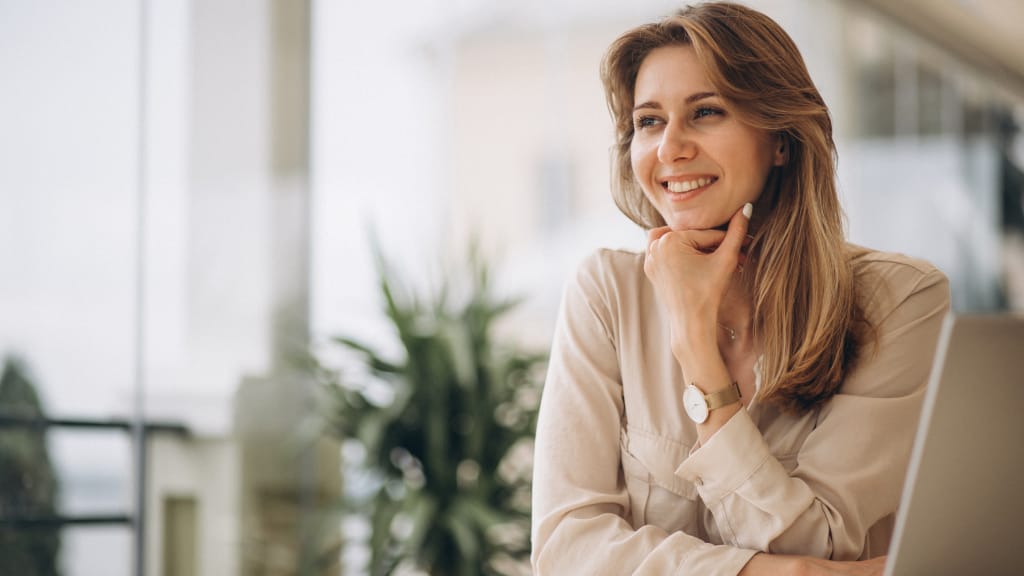
Java Object-Oriented Programming: Classes and Objects
In Java, classes are the foundation of object-oriented programming (OOP). A class represents a blueprint or template for creating objects, which are instances of that class. Classes provide a structure to define attributes (data) and behaviors (methods) that objects of that class can possess. In this article, we will explore classes and objects in Java and provide examples to help you understand their usage.
Defining a Class
In Java, you can define a class using the following syntax:
public class ClassName {
// Attributes
// Methods
}
The public
keyword indicates that the class is accessible from other classes. The ClassName
is the name you give to your class. Within the class, you can define attributes and methods that represent the data and behaviors of objects created from that class.
Example: Creating a Class
Let's consider an example of creating a simple class to represent a person in Java:
public class Person {
// Attributes
private String name;
private int age;
// Constructor
public Person(String name, int age) {
this.name = name;
this.age = age;
}
// Method
public void sayHello() {
System.out.println("Hello, my name is " + name + " and I am " + age + " years old.");
}
}
In the above code, we define a class named Person
with two attributes: name
and age
. We provide a constructor to initialize the attributes, and a method named sayHello
to print a greeting message.
Creating Objects from a Class
To create objects from a class, you use the new
keyword followed by the class name and parentheses. Here's an example:
Person person1 = new Person("John Doe", 25);
Person person2 = new Person("Jane Smith", 30);
In the above code, we create two objects of the Person
class: person1
and person2
. We provide the necessary arguments to the constructor to initialize the attributes of each object.
Accessing Attributes and Invoking Methods
Once objects are created, you can access their attributes and invoke their methods using the dot notation. Here's an example:
System.out.println(person1.name);
System.out.println(person2.age);
person1.sayHello();
person2.sayHello();
In the above code, we access the name
attribute of person1
and the age
attribute of person2
. We also invoke the sayHello
method on both objects to print the greeting message.
Conclusion
Classes and objects are fundamental concepts in Java's object-oriented programming paradigm. In this article, we explored how to define a class, create objects from it, and access their attributes and invoke their methods. We provided an example of a Person
class to illustrate these concepts. By understanding and effectively utilizing classes and objects, you can structure your code into modular and reusable components, making your programs more organized, maintainable, and extensible. Continuously practice creating and working with classes and objects, and explore more advanced topics, such as class inheritance, interfaces, and access modifiers, to further enhance your object-oriented programming skills in Java.
4.5L
Learners
20+
Instructors
50+
Courses
6.0L
Course enrollments
Future Trending Courses
When selecting, a course, Here are a few areas that are expected to be in demand in the future:.
Future Learning for all
If you’re passionate and ready to dive in, we’d love to join 1:1 classes for you. We’re committed to support our learners and professionals their development and well-being.
View Courses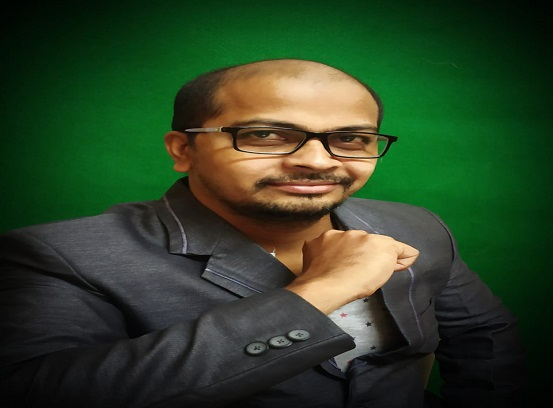
Most Popular Course topics
These are the most popular course topics among Software Courses for learners