Hi there, we’re Harisystems
"Unlock your potential and soar to new heights with our exclusive online courses! Ignite your passion, acquire valuable skills, and embrace limitless possibilities. Don't miss out on our limited-time sale - invest in yourself today and embark on a journey of personal and professional growth. Enroll now and shape your future with knowledge that lasts a lifetime!".
For corporate trainings, projects, and real world experience reach us. We believe that education should be accessible to all, regardless of geographical location or background.
1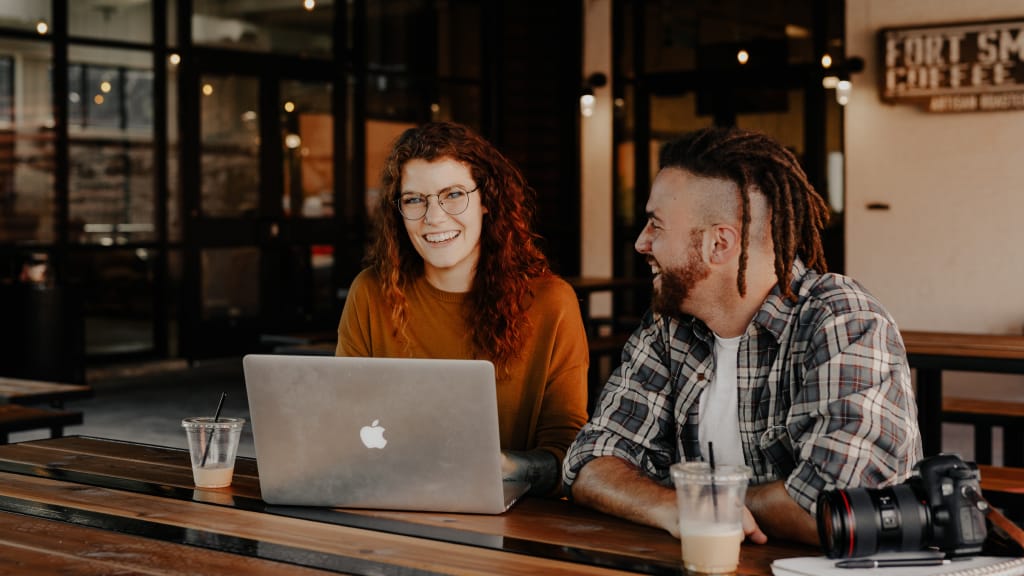
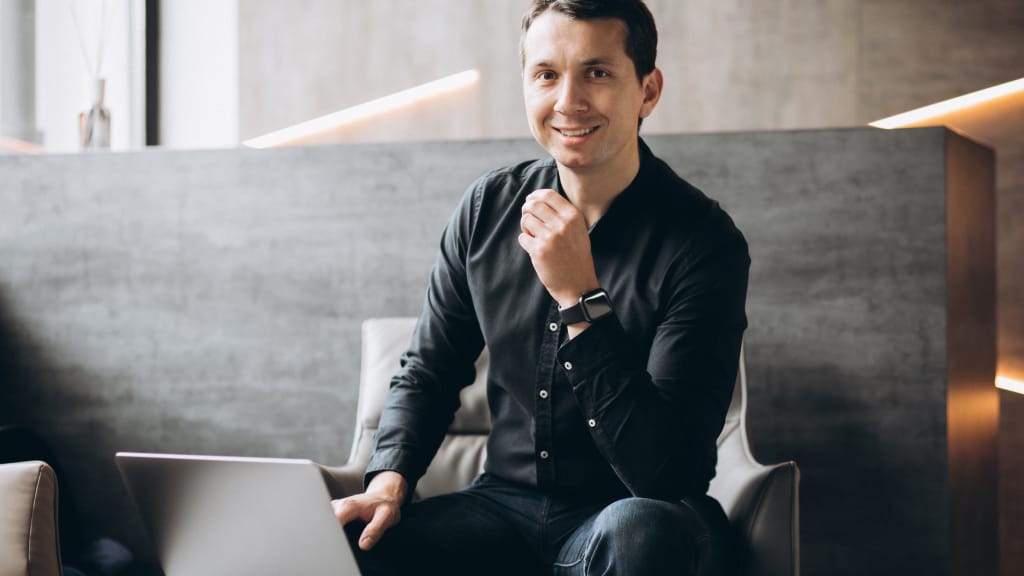
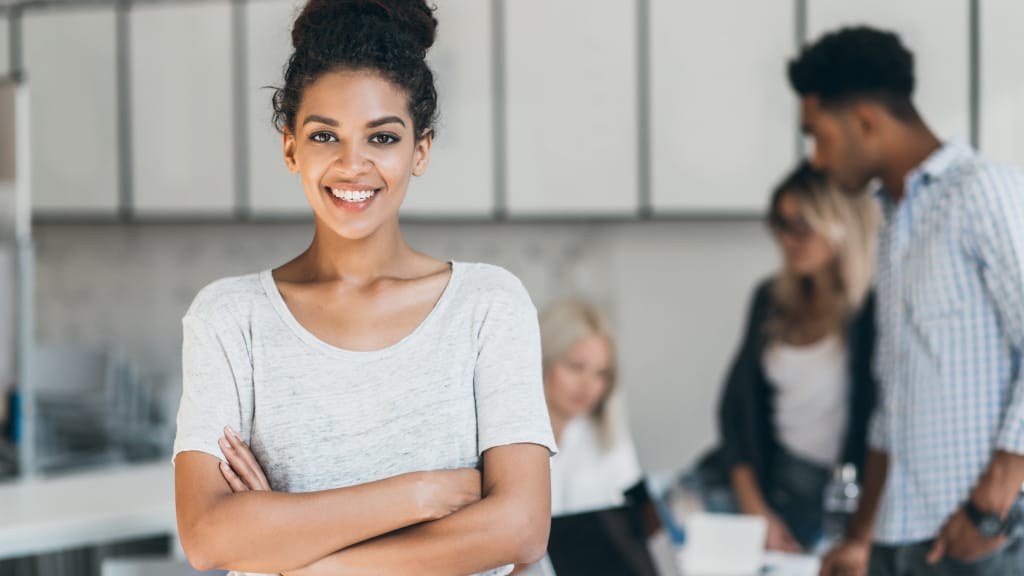
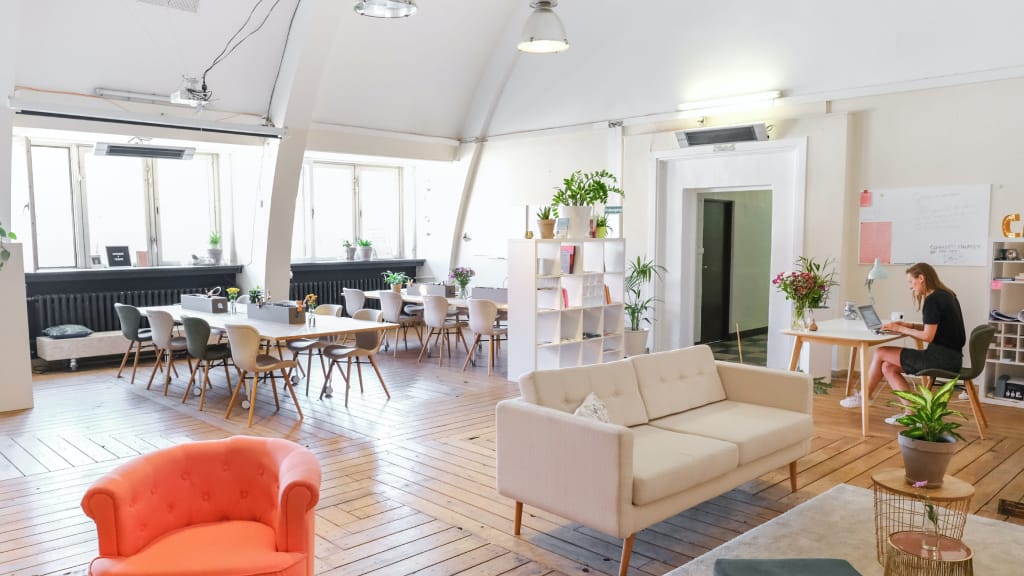
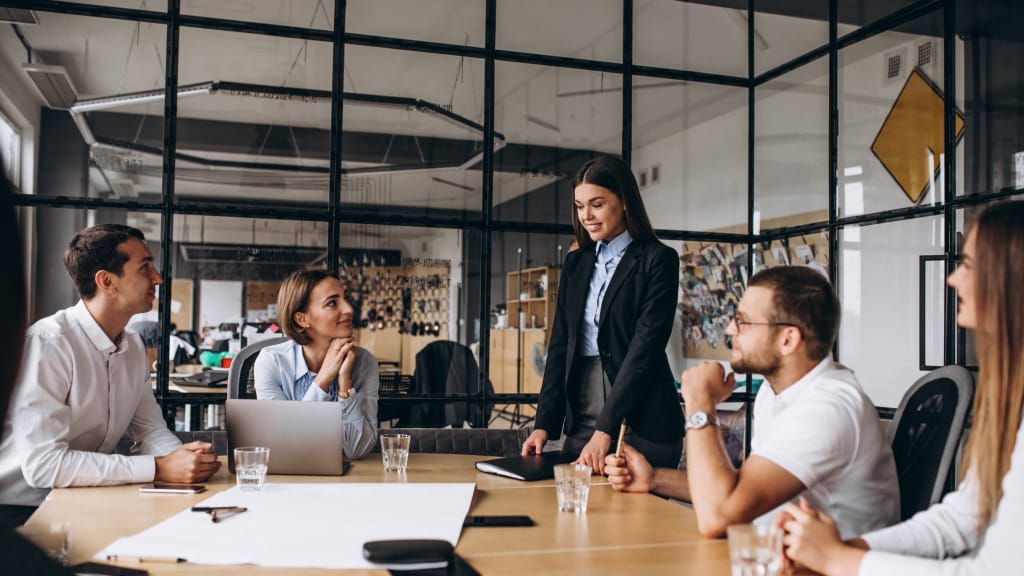
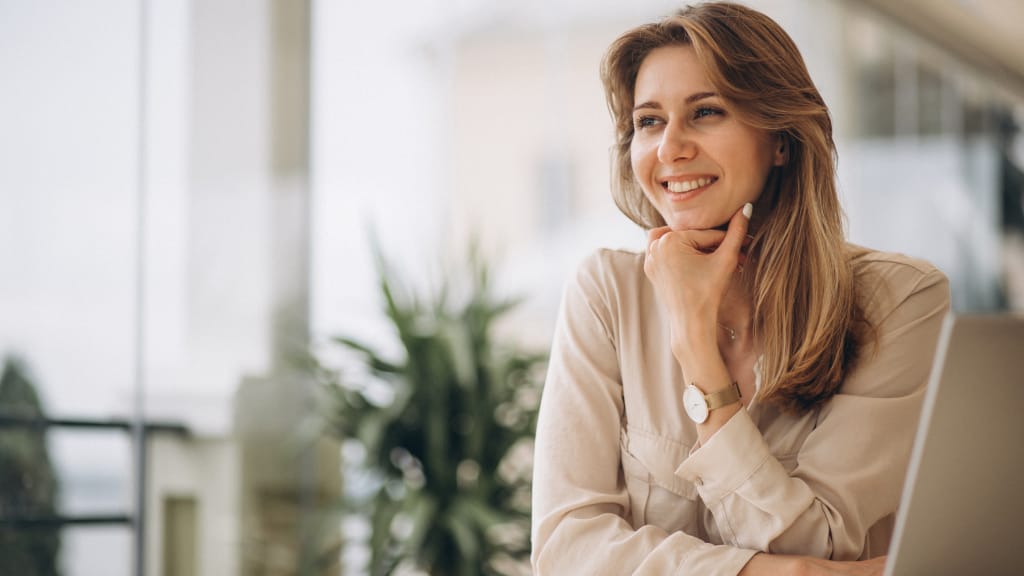
Exploring Advanced Features of JavaScript ES6
JavaScript ES6 (ECMAScript 2015) introduced a plethora of new features and improvements that significantly enhance the capabilities and readability of JavaScript code. In this article, we will explore some advanced features of ES6 and provide examples of their usage.
1. Arrow Functions
Arrow functions provide a concise syntax for writing anonymous functions. They have a more compact syntax and automatically bind the this
value to the enclosing context. Here's an example:
const numbers = [1, 2, 3, 4, 5];
const doubledNumbers = numbers.map((num) => num * 2);
console.log(doubledNumbers); // Output: [2, 4, 6, 8, 10]
In this example, we use an arrow function as the argument to the map
method to double each element in the numbers
array. The result is a new array doubledNumbers
containing the doubled values.
2. Destructuring Assignment
Destructuring assignment allows you to extract values from arrays or objects and assign them to variables in a concise way. Here's an example with an array:
const fruits = ['apple', 'banana', 'orange'];
const [firstFruit, secondFruit] = fruits;
console.log(firstFruit); // Output: 'apple'
console.log(secondFruit); // Output: 'banana'
In this example, we use destructuring assignment to assign the first element of the fruits
array to firstFruit
and the second element to secondFruit
. This provides a convenient way to access individual elements of an array.
3. Template Literals
Template literals are an enhanced way to work with strings in JavaScript. They allow for multiline strings and provide a convenient syntax for embedding variables and expressions. Here's an example:
const name = 'John';
const age = 30;
const greeting = `My name is ${name} and I am ${age} years old.`;
console.log(greeting); // Output: 'My name is John and I am 30 years old.'
In this example, we use template literals to create a string that includes the values of the name
and age
variables. The variables are enclosed in curly braces preceded by a dollar sign, making it easy to insert dynamic values into the string.
4. Spread Operator
The spread operator allows you to expand an iterable object, such as an array or string, into individual elements. It is useful for combining arrays or creating copies of existing arrays. Here's an example:
const numbers = [1, 2, 3];
const moreNumbers = [4, 5, 6];
const combinedNumbers
= [...numbers, ...moreNumbers];
console.log(combinedNumbers); // Output: [1, 2, 3, 4, 5, 6]
In this example, we use the spread operator to combine the elements of the numbers
and moreNumbers
arrays into a new array combinedNumbers
. This provides a concise way to merge arrays without modifying the original arrays.
5. Classes
ES6 introduced class syntax, which provides a more familiar and convenient way to define objects and inheritance in JavaScript. Here's an example:
class Person {
constructor(name) {
this.name = name;
}
sayHello() {
console.log(`Hello, my name is ${this.name}.`);
}
}
const person = new Person('John');
person.sayHello(); // Output: 'Hello, my name is John.'
In this example, we define a Person
class with a constructor and a sayHello
method. We create an instance of the class using the new
keyword and call the sayHello
method on the instance. This provides a more object-oriented approach to creating and working with objects in JavaScript.
Conclusion
JavaScript ES6 introduced several advanced features that enhance the capabilities and readability of JavaScript code. In this article, we explored examples of arrow functions, destructuring assignment, template literals, spread operator, and classes. By utilizing these advanced features, you can write more concise, expressive, and efficient JavaScript code.
4.5L
Learners
20+
Instructors
50+
Courses
6.0L
Course enrollments
Future Trending Courses
When selecting, a course, Here are a few areas that are expected to be in demand in the future:.
Future Learning for all
If you’re passionate and ready to dive in, we’d love to join 1:1 classes for you. We’re committed to support our learners and professionals their development and well-being.
View Courses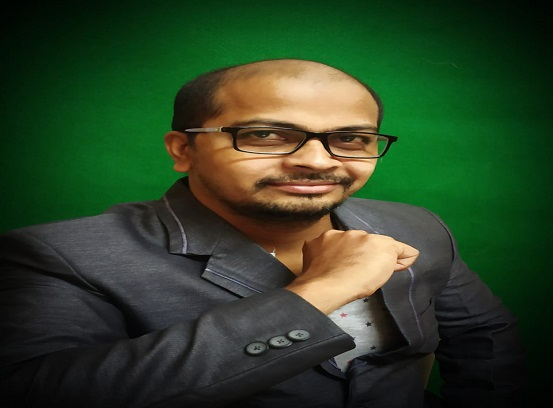
Most Popular Course topics
These are the most popular course topics among Software Courses for learners