Hi there, we’re Harisystems
"Unlock your potential and soar to new heights with our exclusive online courses! Ignite your passion, acquire valuable skills, and embrace limitless possibilities. Don't miss out on our limited-time sale - invest in yourself today and embark on a journey of personal and professional growth. Enroll now and shape your future with knowledge that lasts a lifetime!".
For corporate trainings, projects, and real world experience reach us. We believe that education should be accessible to all, regardless of geographical location or background.
1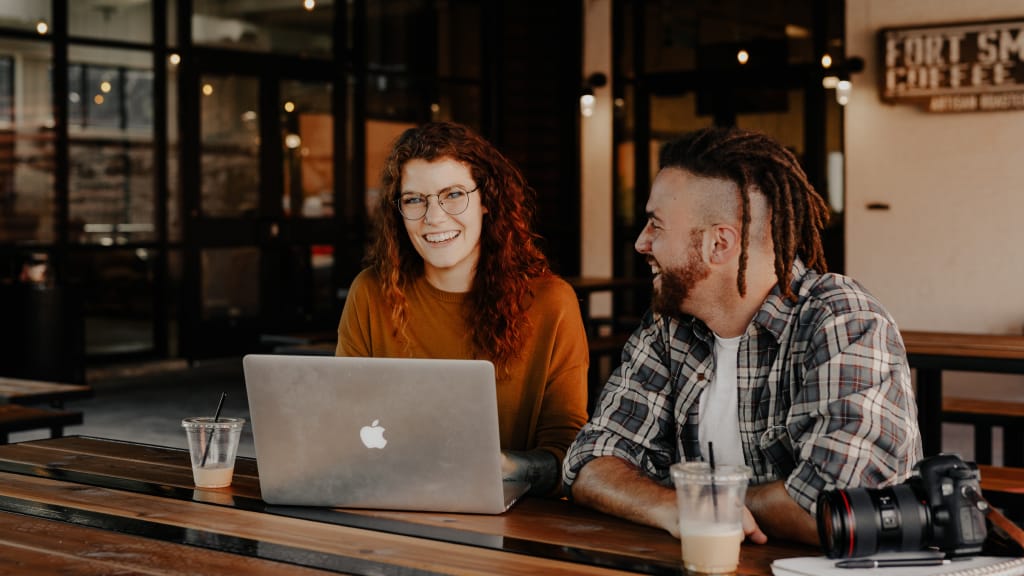
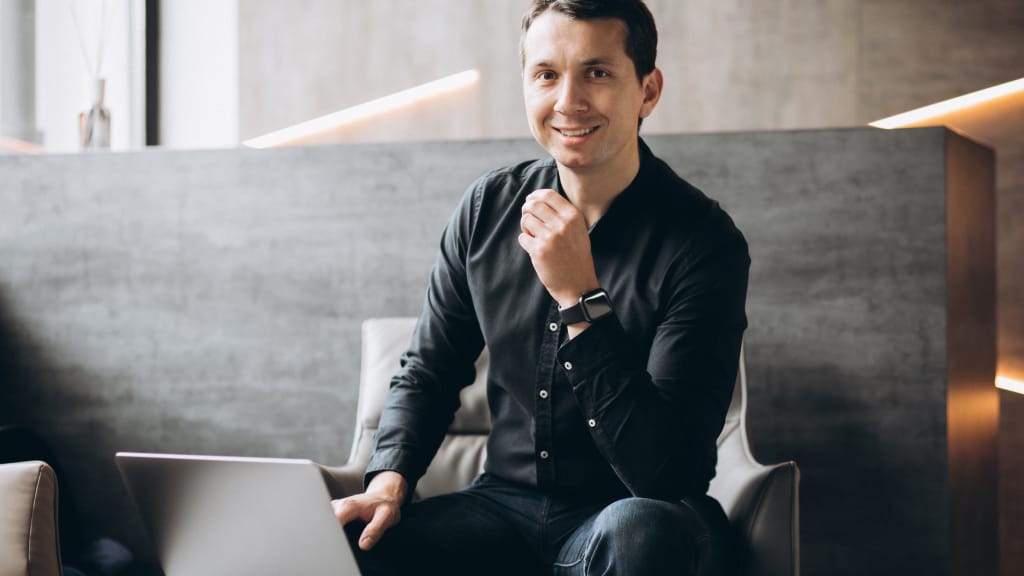
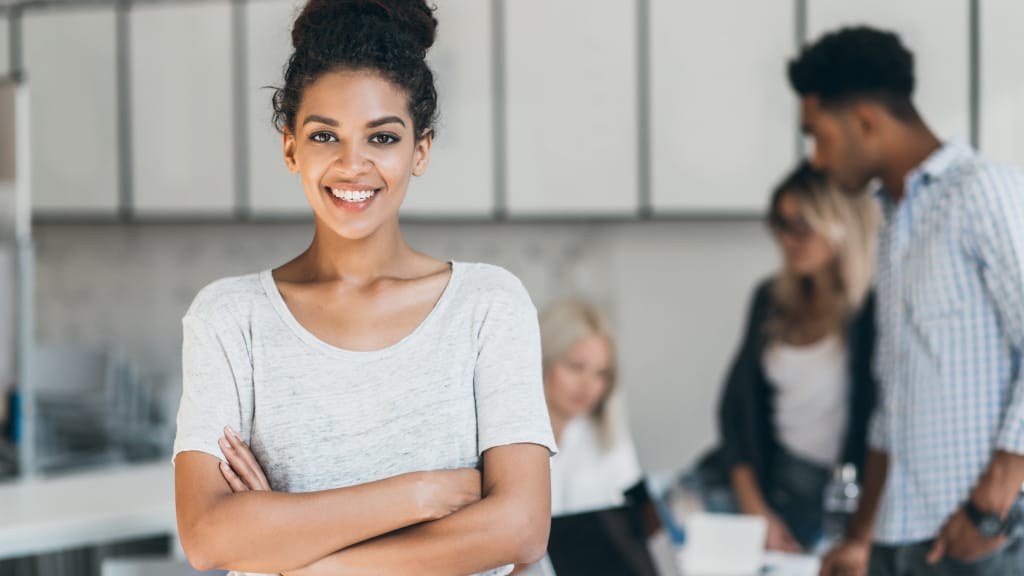
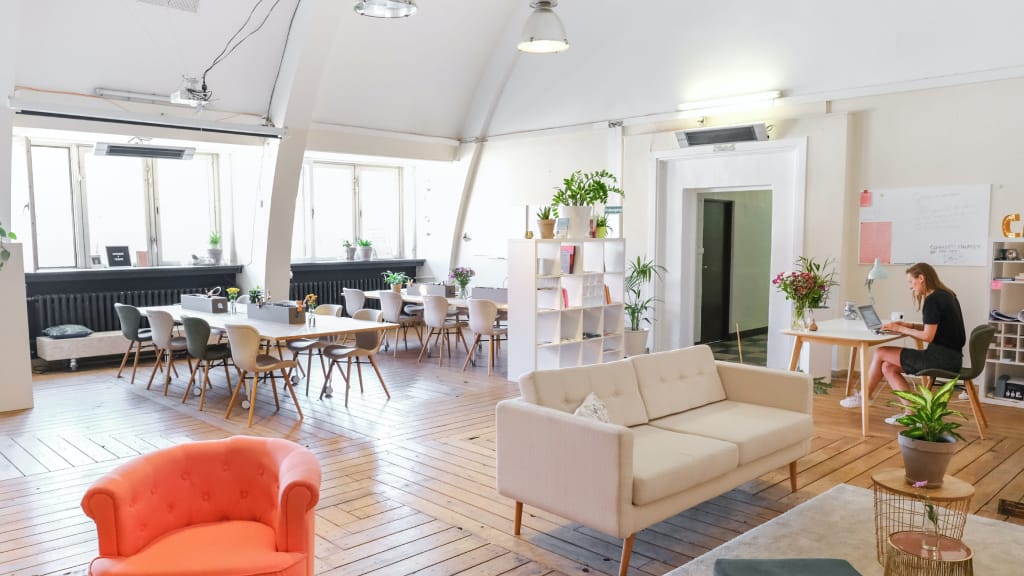
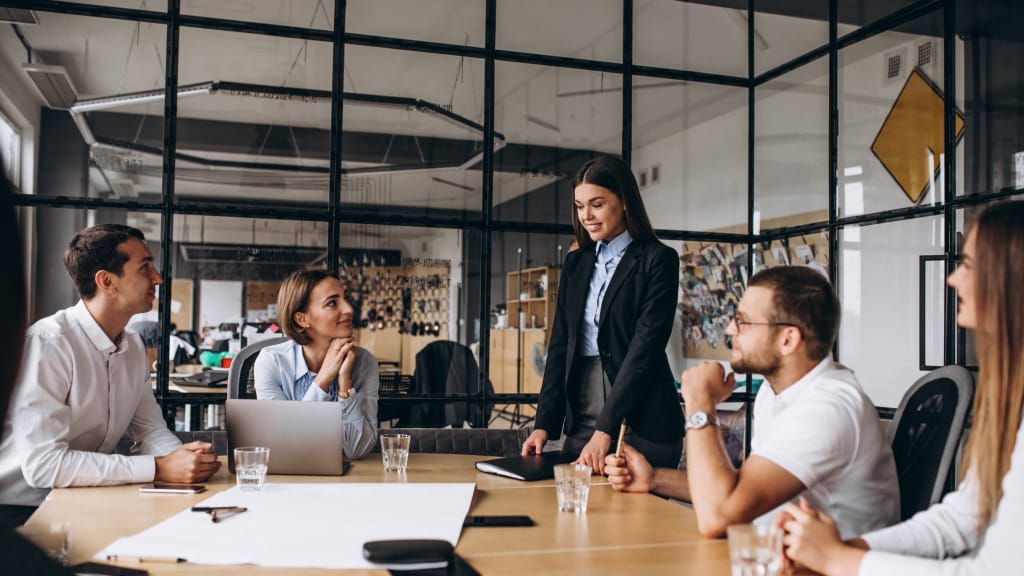
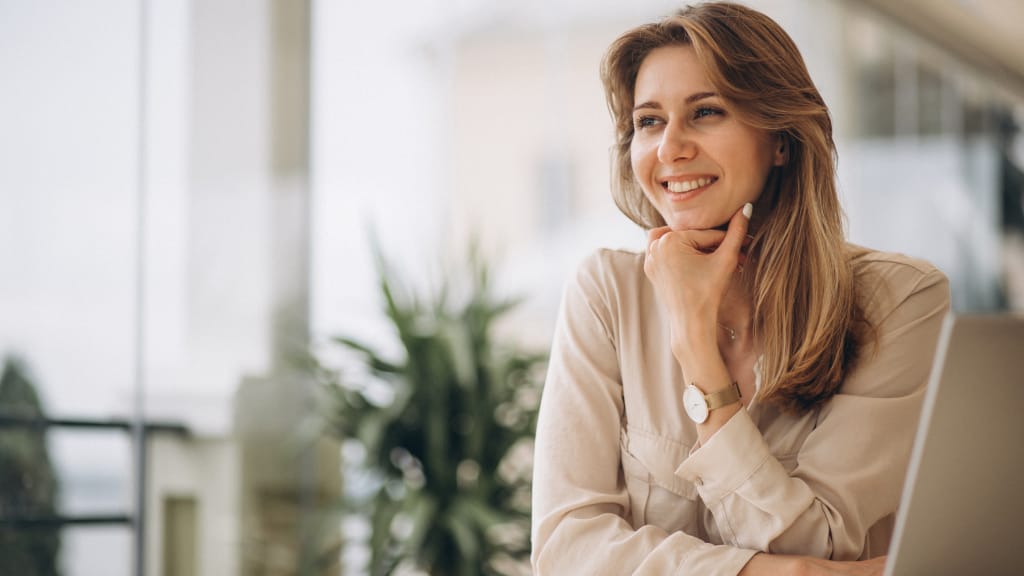
JavaScript Conditional Statements: Explained with Examples
Conditional statements are an essential part of JavaScript that allows you to control the flow of your code based on certain conditions. They enable you to make decisions and execute specific blocks of code accordingly. In this article, we will explore the different types of conditional statements in JavaScript and provide examples of their usage.
if Statement
The if
statement is the most basic type of conditional statement. It allows you to execute a block of code only if a specified condition is true. Here's an example:
let x = 5;
if (x > 0) {
console.log("x is positive");
}
In this example, the code inside the if
block will only execute if the condition x > 0
is true. If the condition is false, the block will be skipped.
if-else Statement
The if-else
statement allows you to execute one block of code if a condition is true, and another block of code if the condition is false. Here's an example:
let age = 20;
if (age >= 18) {
console.log("You are an adult");
} else {
console.log("You are not an adult");
}
In this example, if the condition age >= 18
is true, the first block of code will execute and display "You are an adult". Otherwise, if the condition is false, the second block of code will execute and display "You are not an adult".
if-else if-else Statement
The if-else if-else
statement allows you to test multiple conditions and execute different blocks of code based on the conditions' results. Here's an example:
let day = "Wednesday";
if (day === "Monday") {
console.log("Today is Monday");
} else if (day === "Tuesday") {
console.log("Today is Tuesday");
} else if (day === "Wednesday") {
console.log("Today is Wednesday");
} else {
console.log("Today is not Monday, Tuesday, or Wednesday");
}
In this example, the code will check each condition one by one. If the condition matches, the corresponding block of code will execute. If none of the conditions match, the last else
block will execute as a fallback option.
switch Statement
The switch
statement allows you to select one of many code blocks to execute based on a specified value. It provides a concise way to handle multiple conditions. Here's an example:
let color = "blue";
switch (color) {
case "red":
console.log("The color is red");
break;
case "blue":
console.log("The color is blue");
break;
case "green":
console
.log("The color is green");
break;
default:
console.log("The color is unknown");
break;
}
In this example, the code will check the value of color
and execute the corresponding block of code. If none of the cases match, the default
block will execute.
Conclusion
Conditional statements are powerful tools in JavaScript that allow you to control the flow of your code based on specific conditions. In this article, we explored the if
, if-else
, if-else if-else
, and switch
statements. By mastering these conditional statements, you'll have the ability to create dynamic and flexible code that can handle different scenarios and make decisions based on various conditions.
4.5L
Learners
20+
Instructors
50+
Courses
6.0L
Course enrollments
Future Trending Courses
When selecting, a course, Here are a few areas that are expected to be in demand in the future:.
Future Learning for all
If you’re passionate and ready to dive in, we’d love to join 1:1 classes for you. We’re committed to support our learners and professionals their development and well-being.
View Courses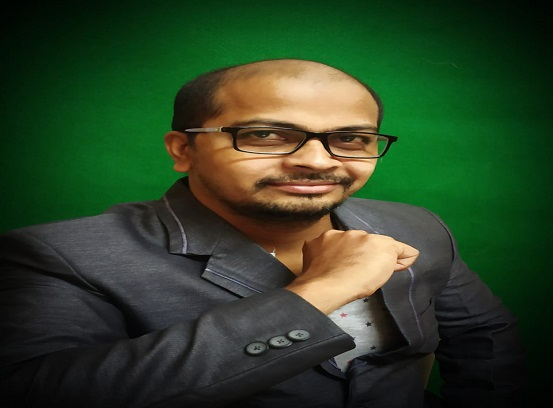
Most Popular Course topics
These are the most popular course topics among Software Courses for learners