Hi there, we’re Harisystems
"Unlock your potential and soar to new heights with our exclusive online courses! Ignite your passion, acquire valuable skills, and embrace limitless possibilities. Don't miss out on our limited-time sale - invest in yourself today and embark on a journey of personal and professional growth. Enroll now and shape your future with knowledge that lasts a lifetime!".
For corporate trainings, projects, and real world experience reach us. We believe that education should be accessible to all, regardless of geographical location or background.
1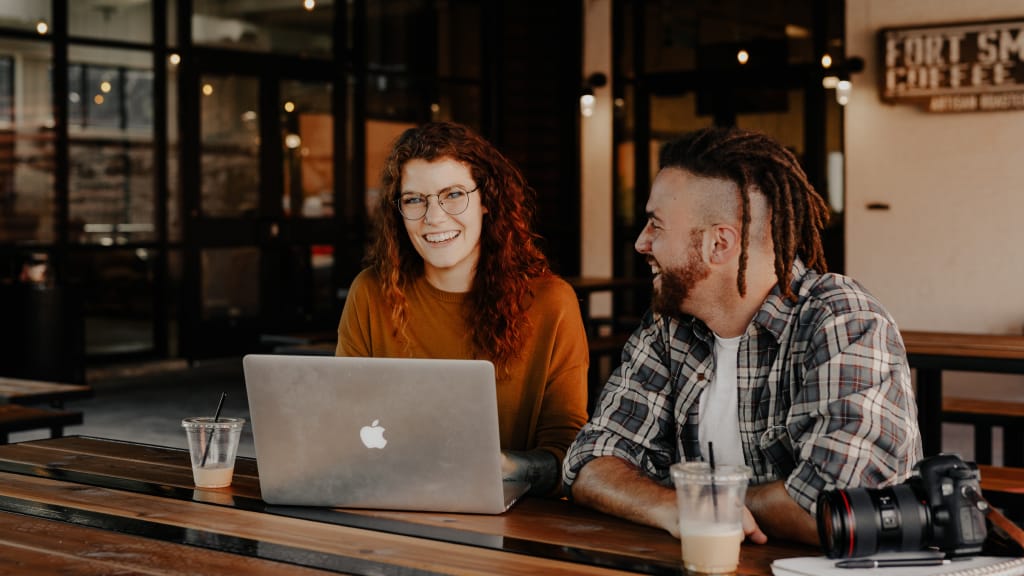
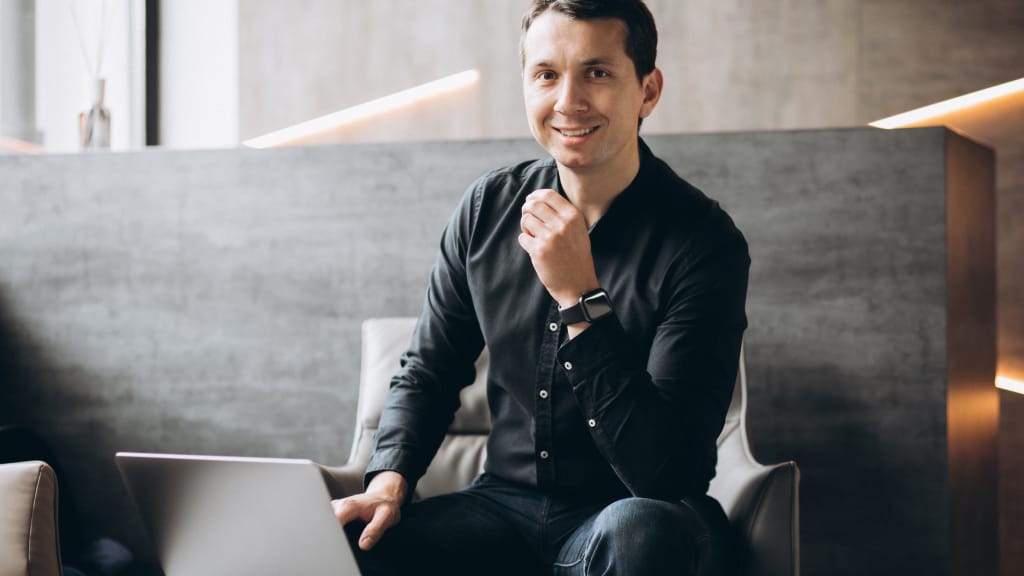
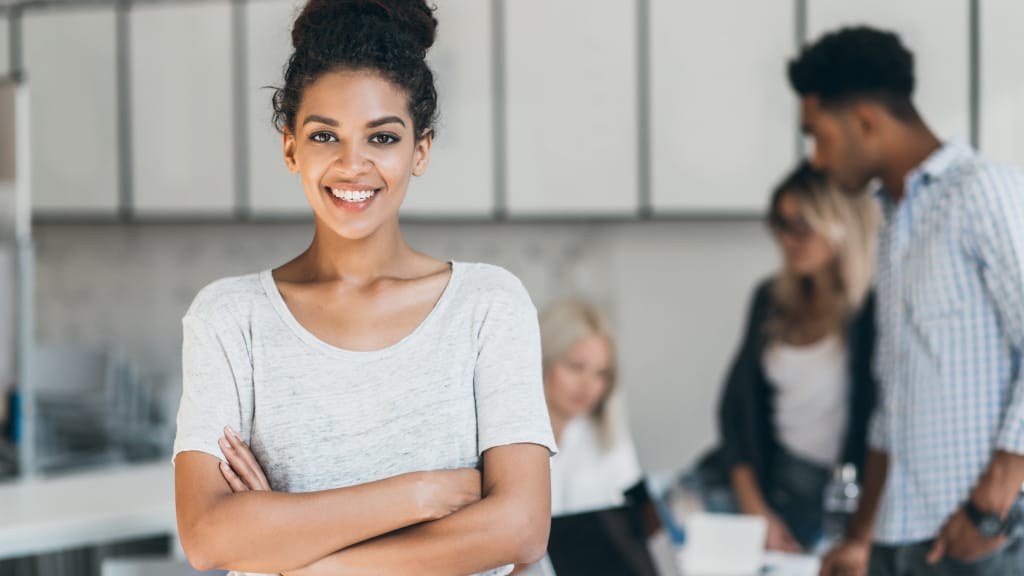
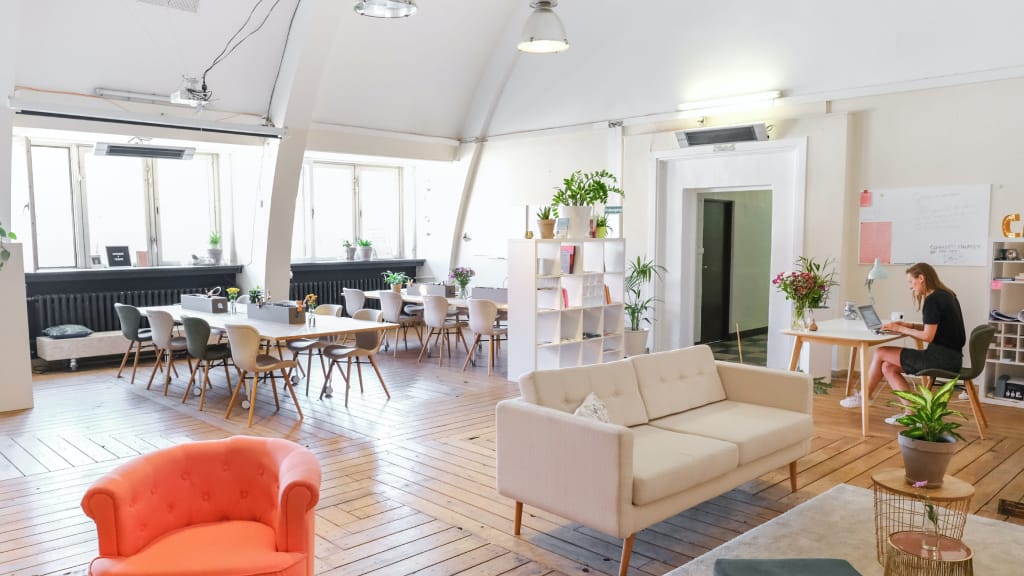
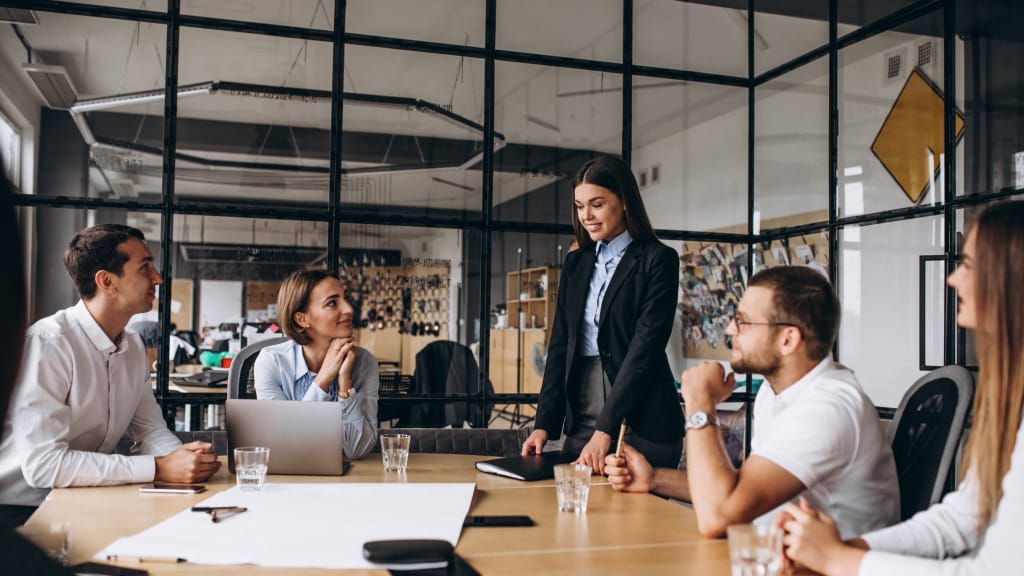
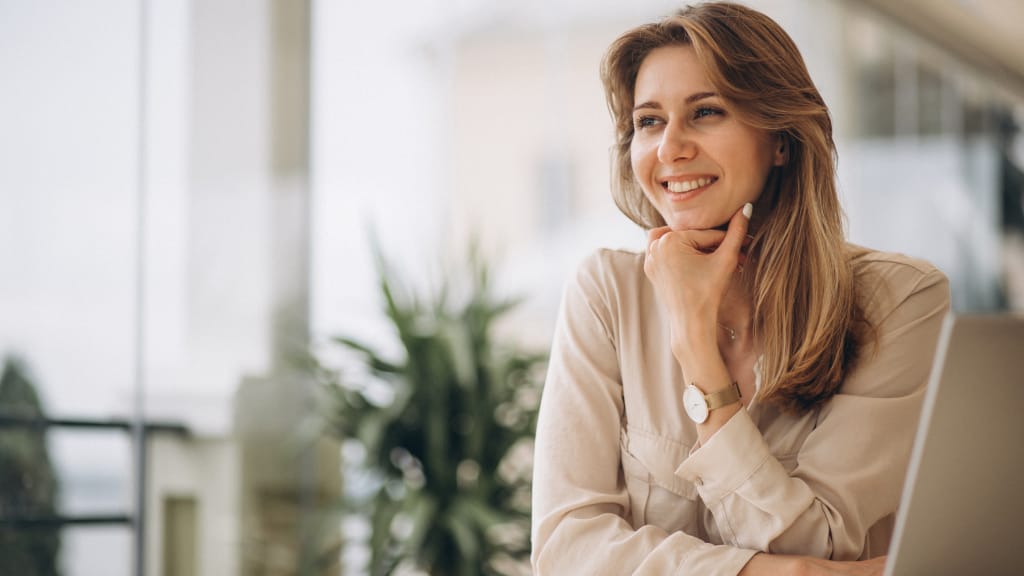
Java Type Casting: Converting Data Types
In Java, type casting is the process of converting a value from one data type to another. It allows you to manipulate data by changing its representation or size. In this article, we will explore the concept of type casting in Java and provide examples to help you understand its usage.
Implicit Casting (Widening)
Implicit casting, also known as widening, occurs when you convert a smaller data type to a larger data type. Java performs implicit casting automatically as it doesn't result in any loss of data. Here's an example:
int num1 = 10;
double num2 = num1; // Implicit casting
System.out.println(num1); // Output: 10
System.out.println(num2); // Output: 10.0
In the above example, the value of num1
(an int
) is implicitly cast to num2
(a double
) since a double
can hold larger values than an int
.
Explicit Casting (Narrowing)
Explicit casting, also known as narrowing, occurs when you convert a larger data type to a smaller data type. Since this conversion may result in loss of data or precision, explicit casting requires you to specify the desired data type. Here's an example:
double num1 = 10.5;
int num2 = (int) num1; // Explicit casting
System.out.println(num1); // Output: 10.5
System.out.println(num2); // Output: 10
In the above example, the value of num1
(a double
) is explicitly cast to num2
(an int
) using parentheses and the target data type (int)
. The decimal part of num1
is truncated, resulting in the value of 10
for num2
.
Handling Incompatible Type Casting
Not all types of data conversions are valid. When casting between incompatible types, such as converting a String
to an int
, a ClassCastException
may occur at runtime. To avoid this, you can use appropriate methods or classes for converting between incompatible types, such as Integer.parseInt
for converting a String
to an int
. Here's an example:
String str = "10";
int num = Integer.parseInt(str); // Conversion using method
System.out.println(num); // Output: 10
In the above example, the parseInt
method is used to convert the String
"10"
to an int
value stored in the variable num
.
Conclusion
Type casting is a powerful feature in Java that allows you to convert values from one data type to another. In this article, we explored the concepts of implicit casting (widening) and explicit casting (narrowing), along with examples to illustrate their usage. We also discussed how to handle incompatible type casting by using appropriate methods or classes. By understanding type casting, you can effectively manipulate and transform data in your Java programs. Continuously practice using type casting and explore more advanced topics, such as type compatibility and type promotion, to enhance your Java programming skills.
4.5L
Learners
20+
Instructors
50+
Courses
6.0L
Course enrollments
Future Trending Courses
When selecting, a course, Here are a few areas that are expected to be in demand in the future:.
Future Learning for all
If you’re passionate and ready to dive in, we’d love to join 1:1 classes for you. We’re committed to support our learners and professionals their development and well-being.
View Courses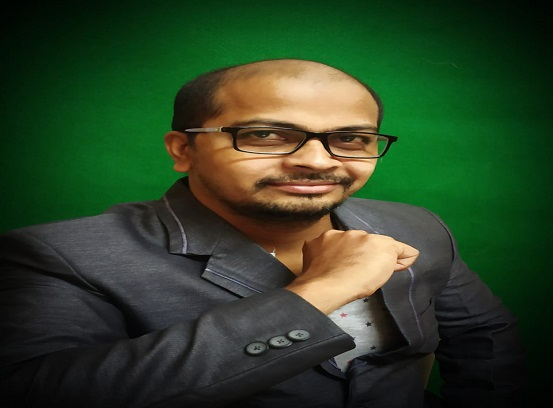
Most Popular Course topics
These are the most popular course topics among Software Courses for learners