Hi there, we’re Harisystems
"Unlock your potential and soar to new heights with our exclusive online courses! Ignite your passion, acquire valuable skills, and embrace limitless possibilities. Don't miss out on our limited-time sale - invest in yourself today and embark on a journey of personal and professional growth. Enroll now and shape your future with knowledge that lasts a lifetime!".
For corporate trainings, projects, and real world experience reach us. We believe that education should be accessible to all, regardless of geographical location or background.
1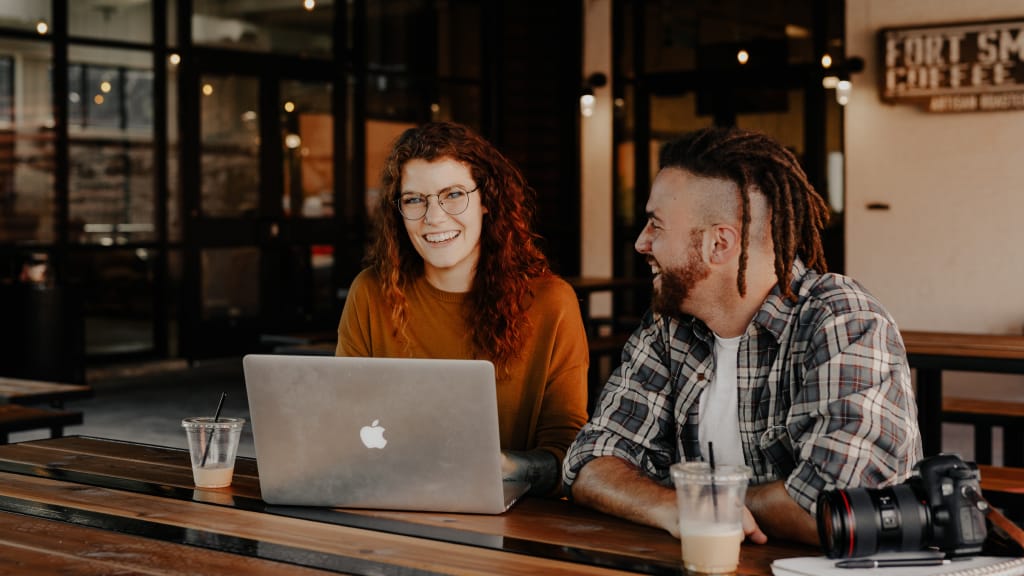
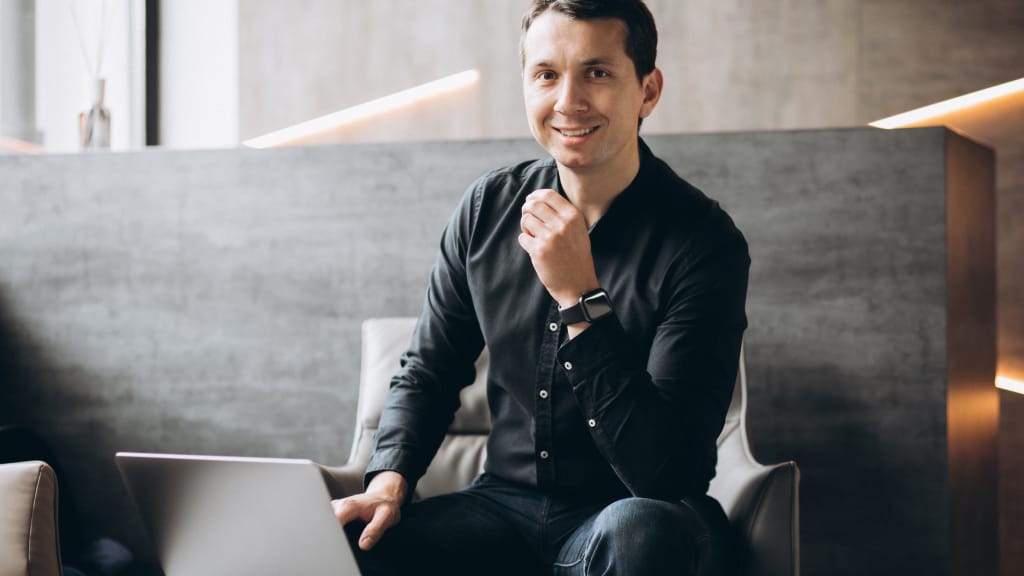
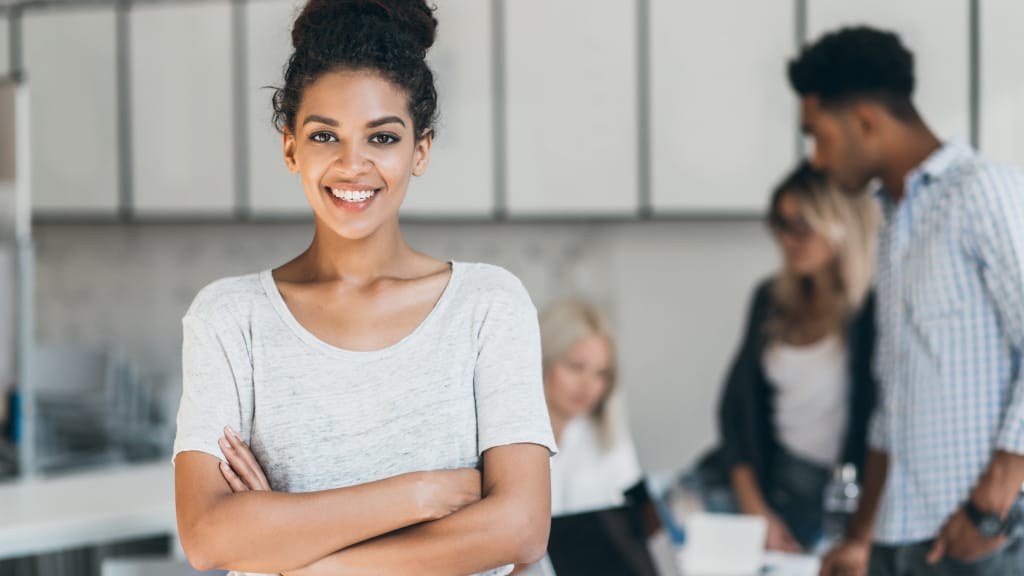
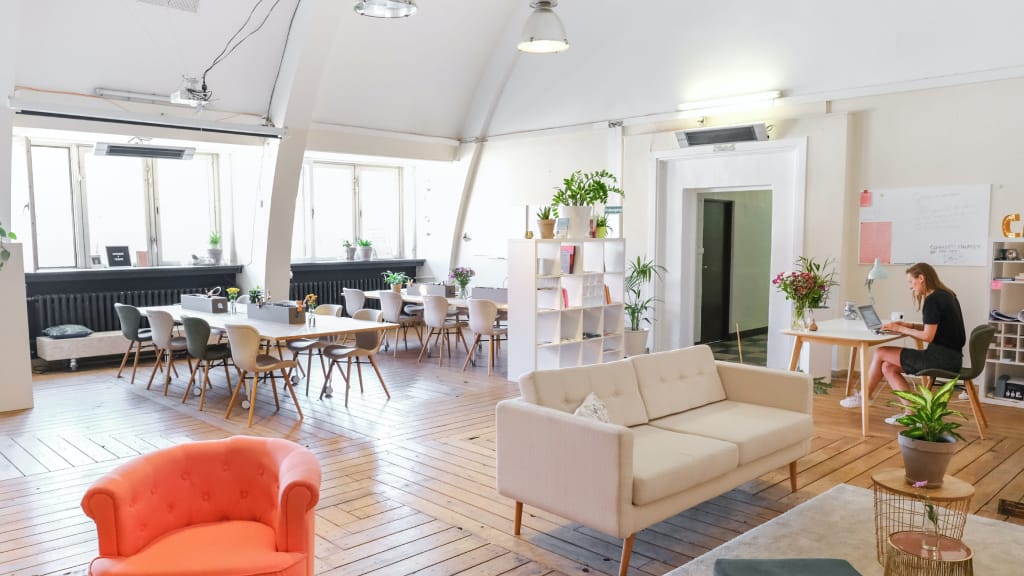
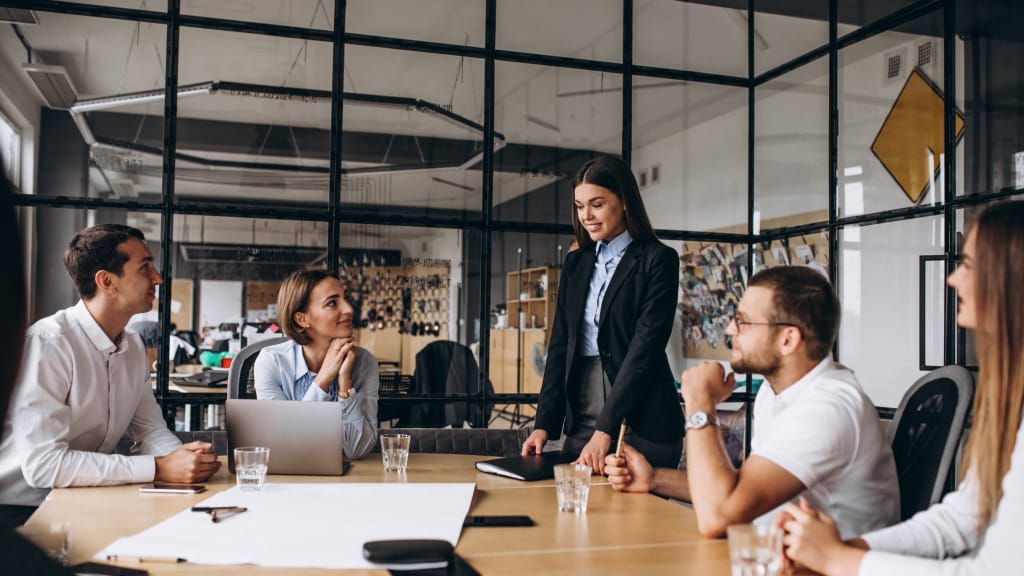
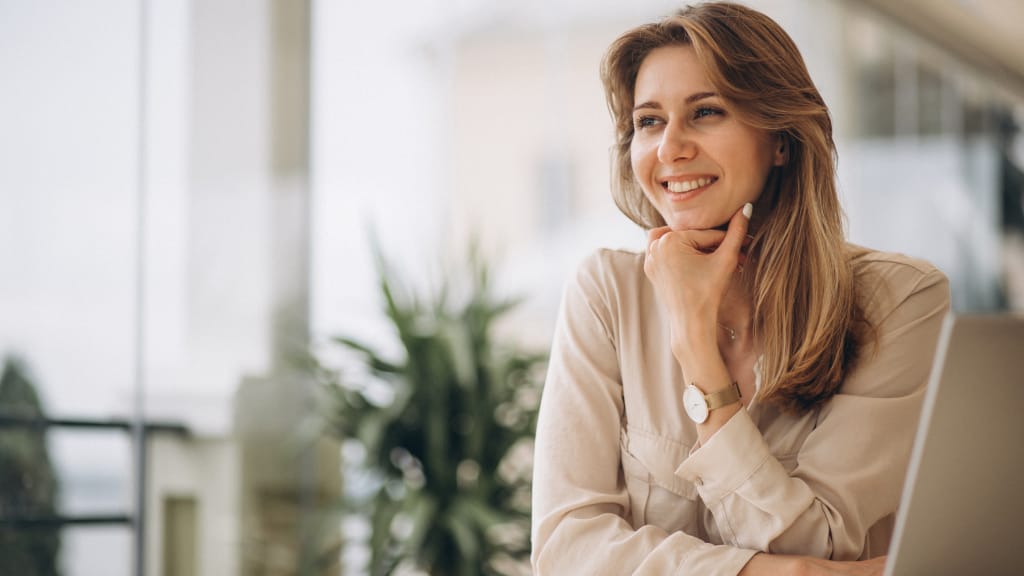
Java Introduction: Building Powerful Applications
Java is a widely-used programming language known for its versatility and robustness. It is widely adopted for building a variety of applications, including desktop, web, mobile, and enterprise applications. In this article, we will explore the basics of Java programming and provide examples of its implementation.
What is Java?
Java is a general-purpose programming language that was developed by Sun Microsystems (now owned by Oracle) in the mid-1990s. It is an object-oriented language designed to be platform-independent, meaning that Java applications can run on any operating system that has a Java Virtual Machine (JVM) installed.
Getting Started with Java
To start programming in Java, you need to set up the Java Development Kit (JDK) on your computer. The JDK includes the Java compiler and other tools necessary for developing Java applications. You can download the JDK from the official Oracle website and follow the installation instructions for your operating system.
Hello World Example
A "Hello, World!" program is a traditional way to start learning a new programming language. Here's an example of a simple Hello World program in Java:
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
The above code defines a class named "HelloWorld" with a main method. The main method is the entry point of a Java program, and it prints "Hello, World!" to the console using the System.out.println method.
Variables and Data Types
Java is a statically-typed language, meaning that you need to declare the type of a variable before using it. Here's an example of declaring and initializing variables in Java:
int age = 25;
double salary = 50000.5;
String name = "John Smith";
boolean isEmployed = true;
Control Flow Statements
Java provides various control flow statements, such as if-else, for loops, while loops, and switch statements, to control the flow of execution in a program. Here's an example of an if-else statement:
int number = 10;
if (number % 2 == 0) {
System.out.println("The number is even.");
} else {
System.out.println("The number is odd.");
}
Object-Oriented Programming (OOP)
Java is an object-oriented programming language, which means that it supports concepts such as classes, objects, inheritance, and polymorphism. Here's an example of defining a class and creating objects in Java:
public class Car {
private String brand;
private String color;
public Car(String brand, String color) {
this.brand = brand;
this.color = color;
}
public void startEngine() {
System.out.println("Engine started.");
}
public static void main(String[] args) {
Car myCar = new Car("Toyota", "Red");
myCar.startEngine();
}
}
In the above example, we define a Car class with brand and color properties and a startEngine method. We then create an instance of the Car class and call the startEngine method.
Conclusion
Java is a versatile and powerful programming language widely used for developing a wide range of applications. It provides a robust and scalable platform for building desktop, web, mobile, and enterprise applications. Understanding the fundamentals of Java programming, including syntax, data types, control flow statements, and object-oriented concepts, is essential for becoming a proficient Java developer. Continuously exploring and learning new Java features and best practices will help you build powerful and efficient applications.
4.5L
Learners
20+
Instructors
50+
Courses
6.0L
Course enrollments
Future Trending Courses
When selecting, a course, Here are a few areas that are expected to be in demand in the future:.
Future Learning for all
If you’re passionate and ready to dive in, we’d love to join 1:1 classes for you. We’re committed to support our learners and professionals their development and well-being.
View Courses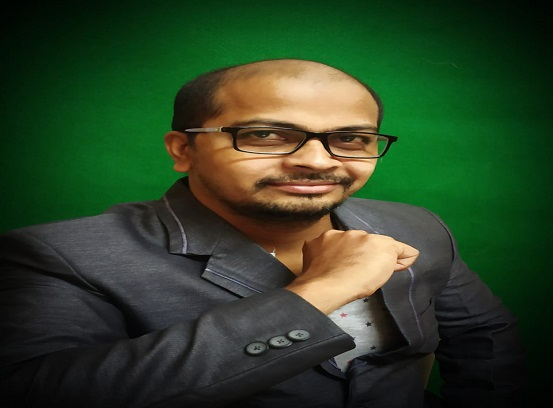
Most Popular Course topics
These are the most popular course topics among Software Courses for learners