Hi there, we’re Harisystems
"Unlock your potential and soar to new heights with our exclusive online courses! Ignite your passion, acquire valuable skills, and embrace limitless possibilities. Don't miss out on our limited-time sale - invest in yourself today and embark on a journey of personal and professional growth. Enroll now and shape your future with knowledge that lasts a lifetime!".
For corporate trainings, projects, and real world experience reach us. We believe that education should be accessible to all, regardless of geographical location or background.
1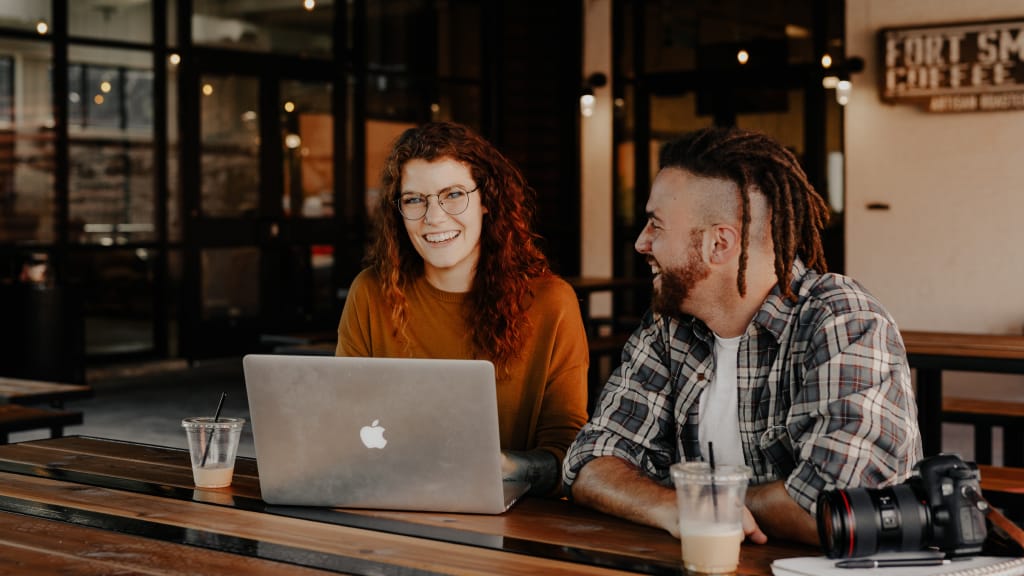
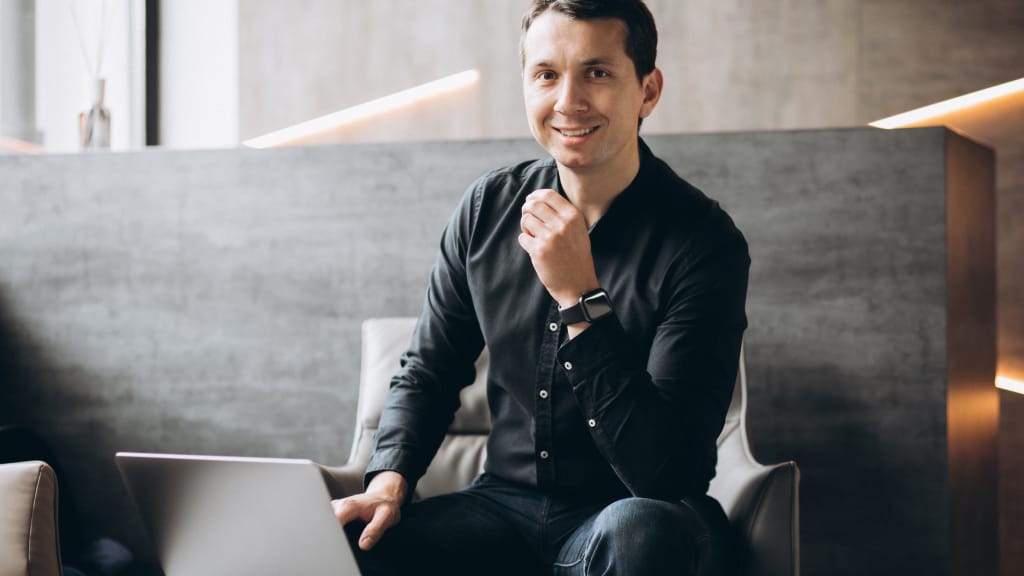
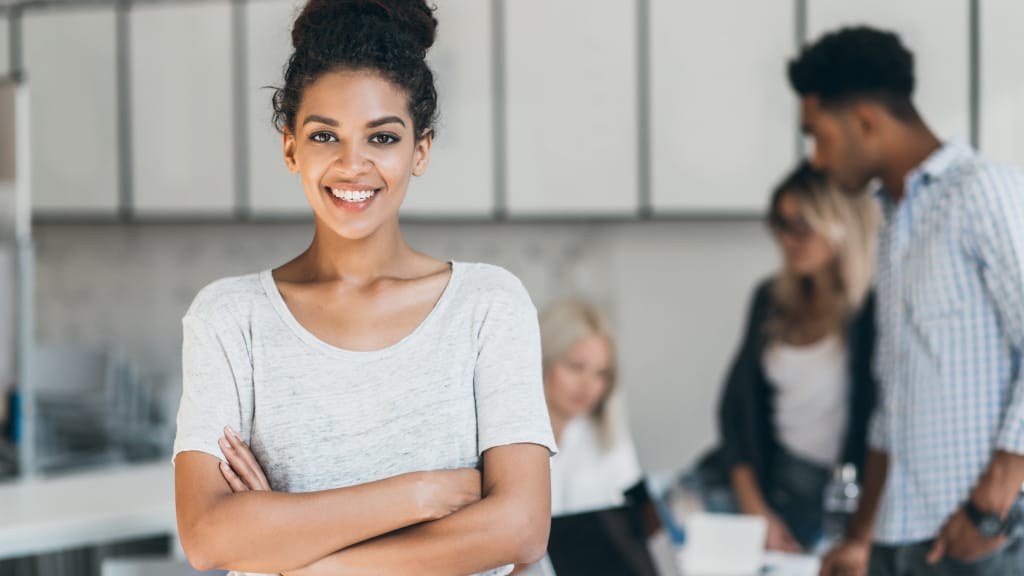
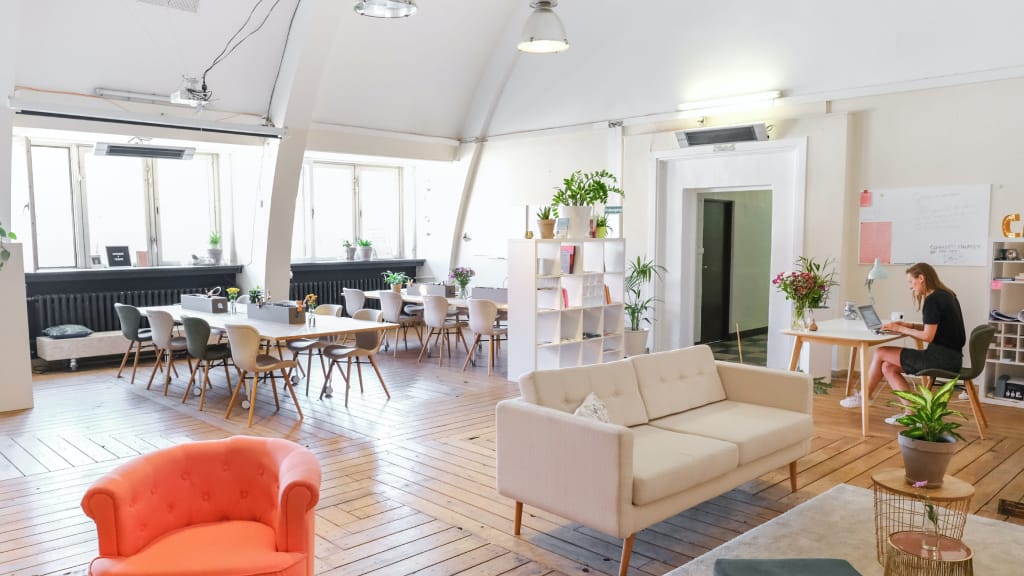
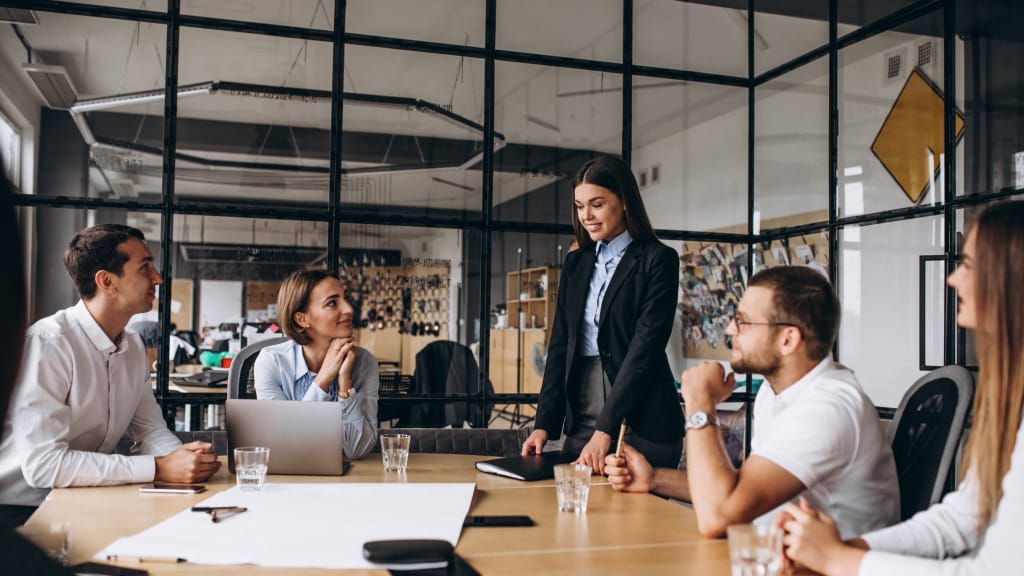
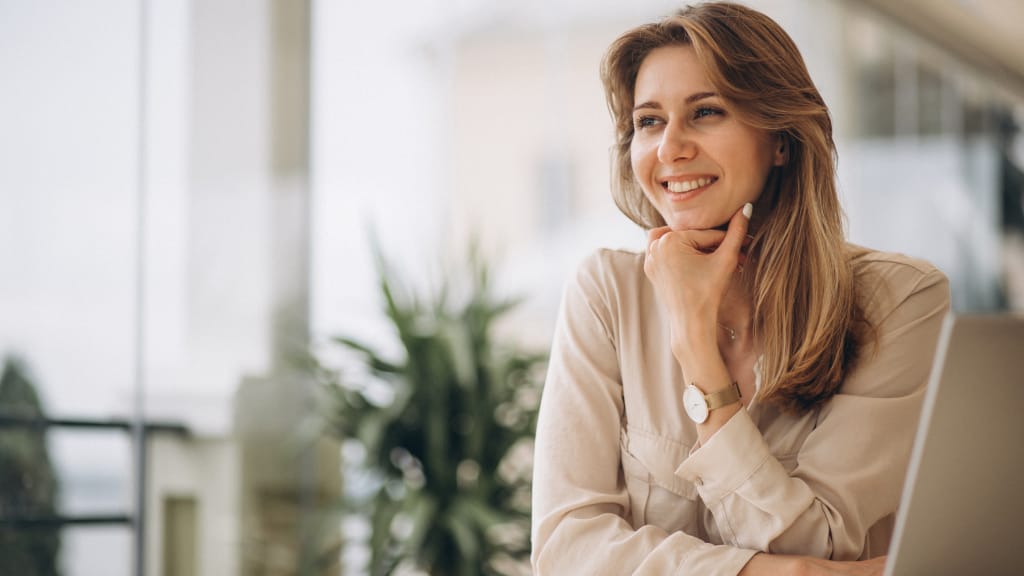
Java switch Statements: Multi-Way Branching
In Java, the switch
statement is used for multi-way branching. It allows you to select one of many code blocks to be executed based on the value of a variable or an expression. The switch
statement provides an alternative to multiple if-else
statements when you have a specific set of values to compare. In this article, we will explore the switch
statement in Java and provide examples to help you understand its usage.
The switch Statement Syntax
The syntax of the switch
statement in Java is as follows:
switch (expression) {
case value1:
// code to execute if expression matches value1
break;
case value2:
// code to execute if expression matches value2
break;
// additional cases
default:
// code to execute if expression doesn't match any case
}
The expression
is evaluated and compared to the values specified in each case
. If a match is found, the corresponding code block is executed. The break
statement is used to exit the switch
block after executing the corresponding code. The default
case is optional and is executed if no match is found.
Example: Checking Day of the Week
Let's consider an example where we want to check the day of the week based on a given number. We can use a switch
statement to perform this check:
int day = 3;
String dayName;
switch (day) {
case 1:
dayName = "Monday";
break;
case 2:
dayName = "Tuesday";
break;
case 3:
dayName = "Wednesday";
break;
case 4:
dayName = "Thursday";
break;
case 5:
dayName = "Friday";
break;
case 6:
dayName = "Saturday";
break;
case 7:
dayName = "Sunday";
break;
default:
dayName = "Invalid day";
break;
}
System.out.println("The day is: " + dayName);
In the above code, if the day
is 3, the program assigns the value "Wednesday"
to the dayName
variable and prints "The day is: Wednesday"
.
Using Enumerations in switch Statements
The switch
statement can also be used with enumerations. Enumerations define a set of named constants, which can be used as cases in a switch
statement. Here's an example:
enum Direction {
NORTH,
SOUTH,
EAST,
WEST
}
Direction direction = Direction.WEST;
switch (direction) {
case NORTH:
System.out.println("Moving north");
break;
case SOUTH:
System.out.println("Moving south");
break;
case EAST:
System.out.println("Moving east");
break;
case WEST:
System.out.println("Moving west");
break;
}
In the above code, the switch
statement compares the direction
with each case of the Direction
enumeration and executes the corresponding code block.
Conclusion
The switch
statement in Java provides a concise way to perform multi-way branching based on a variable or an expression. In this article, we explored the syntax of the switch
statement and provided examples to demonstrate its usage. We also discussed the use of enumerations in switch
statements, which provides type safety and clarity. By utilizing the switch
statement effectively, you can simplify your code and improve readability when dealing with multiple cases. Continuously practice using switch
statements and explore more advanced topics, such as nested switch
statements and fall-through cases, to enhance your control flow capabilities in Java programming.
4.5L
Learners
20+
Instructors
50+
Courses
6.0L
Course enrollments
Future Trending Courses
When selecting, a course, Here are a few areas that are expected to be in demand in the future:.
Future Learning for all
If you’re passionate and ready to dive in, we’d love to join 1:1 classes for you. We’re committed to support our learners and professionals their development and well-being.
View Courses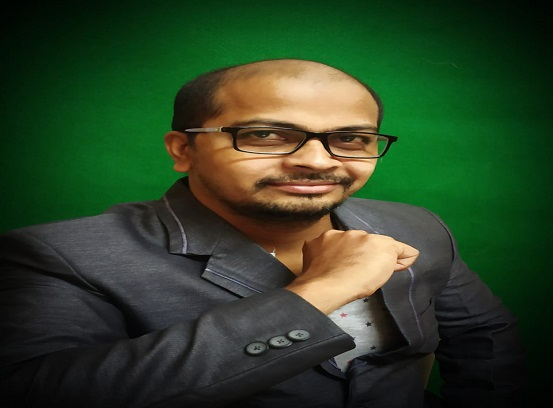
Most Popular Course topics
These are the most popular course topics among Software Courses for learners