Hi there, we’re Harisystems
"Unlock your potential and soar to new heights with our exclusive online courses! Ignite your passion, acquire valuable skills, and embrace limitless possibilities. Don't miss out on our limited-time sale - invest in yourself today and embark on a journey of personal and professional growth. Enroll now and shape your future with knowledge that lasts a lifetime!".
For corporate trainings, projects, and real world experience reach us. We believe that education should be accessible to all, regardless of geographical location or background.
1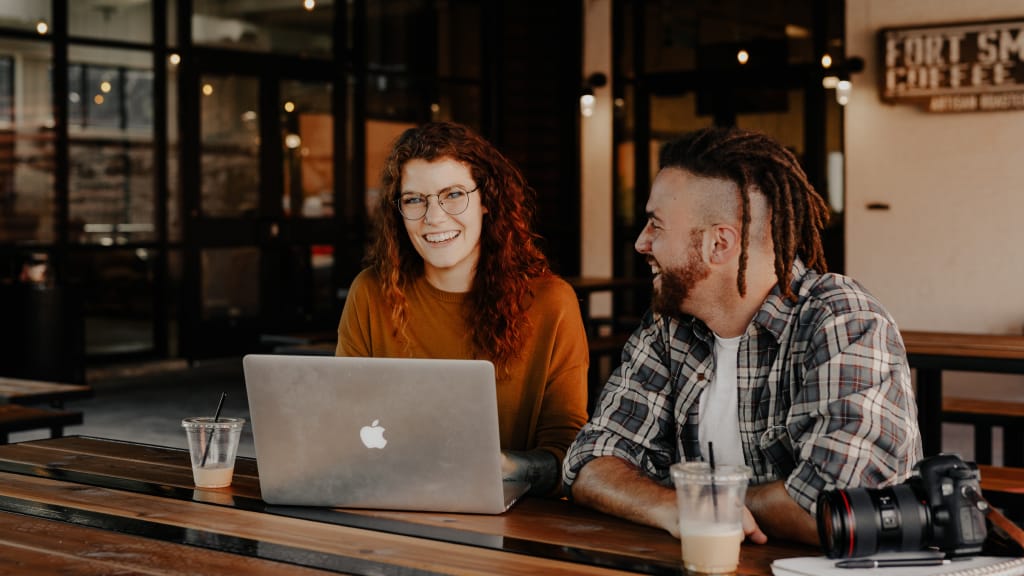
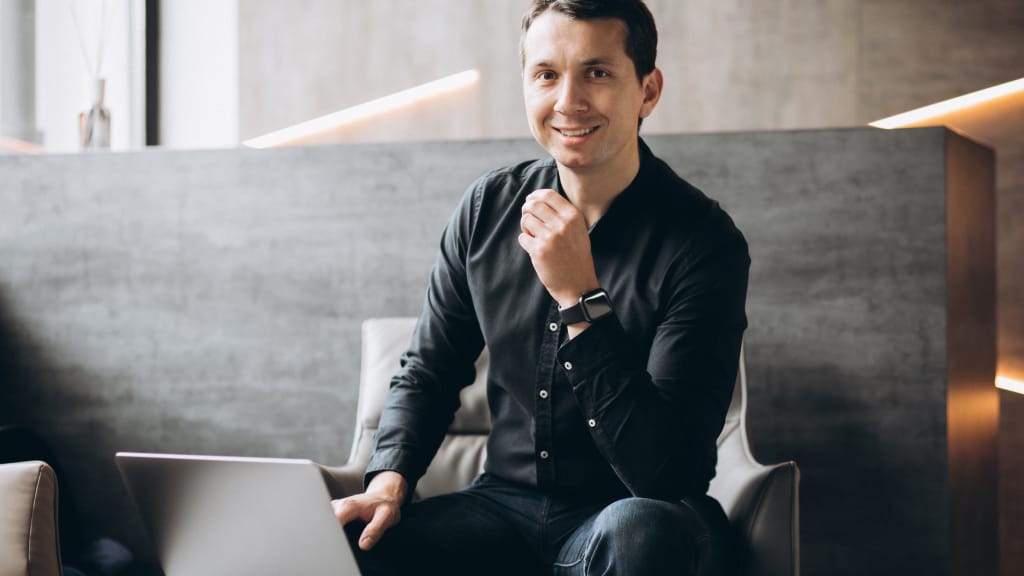
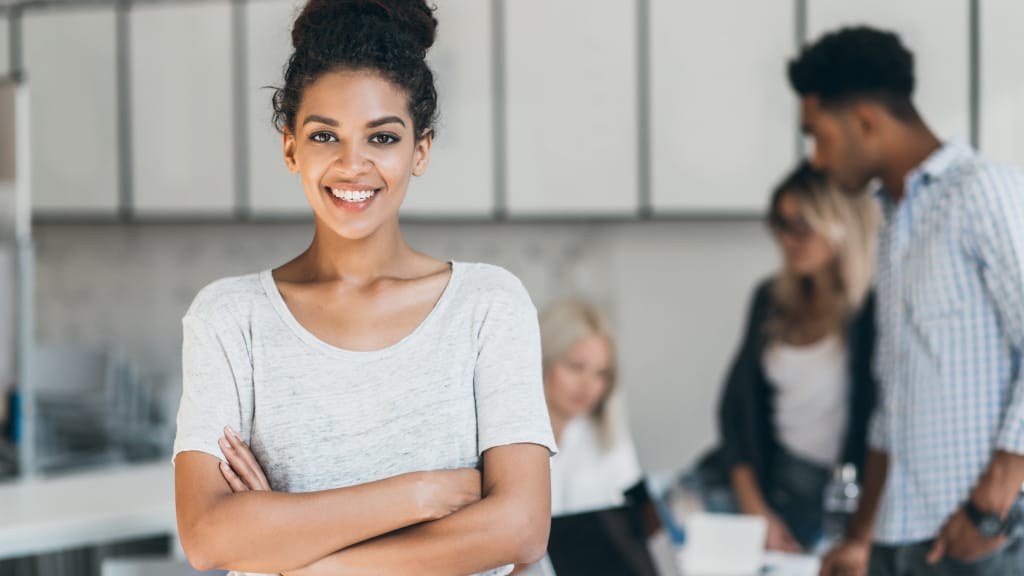
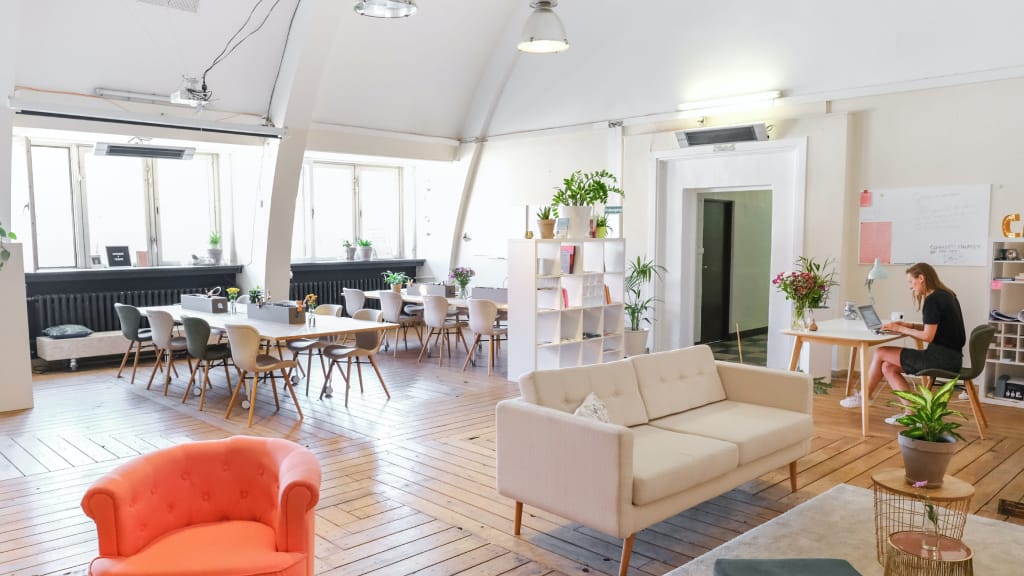
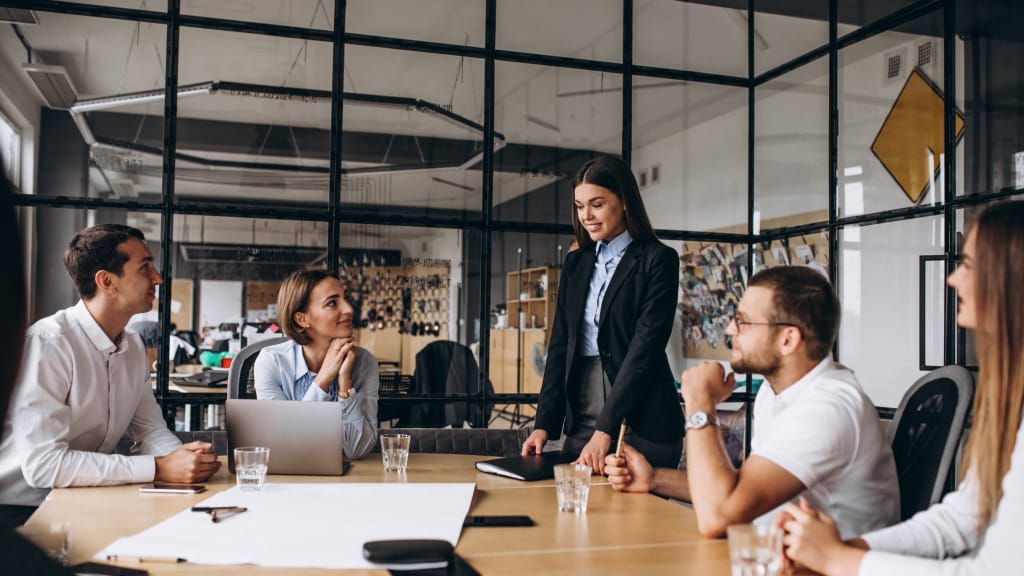
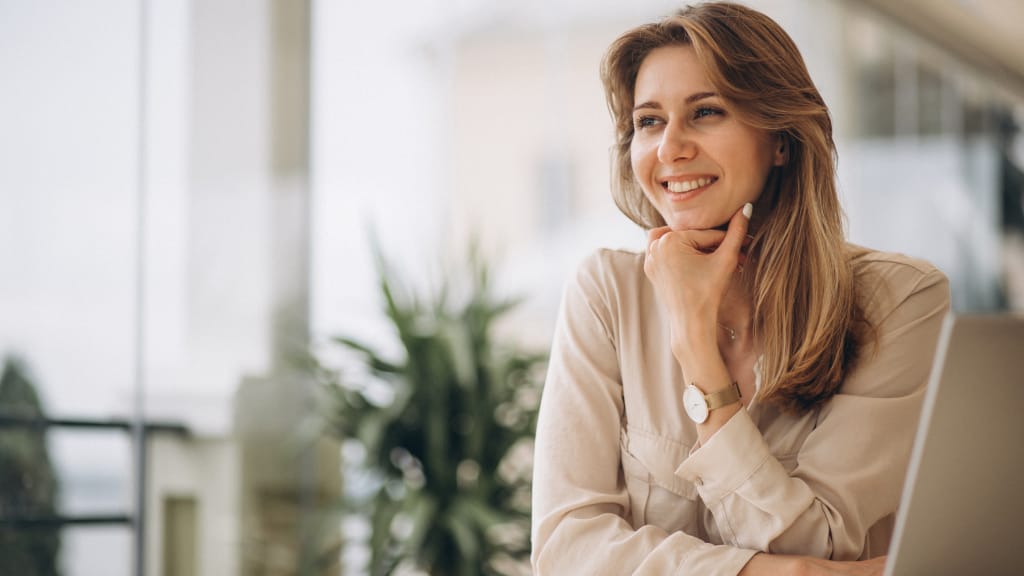
Exploring JavaScript Classes and Objects: Examples and Usage
JavaScript is an object-oriented programming language that supports the creation of classes and objects. Classes provide a blueprint for creating objects with properties and methods. In this article, we will explore the concept of classes and objects in JavaScript and provide examples of their usage.
1. Creating a Class
In JavaScript, you can create a class using the class
keyword. Here's an example of a simple class named Person
with a constructor and a method:
<script>
class Person {
constructor(name, age) {
this.name = name;
this.age = age;
}
sayHello() {
console.log("Hello, my name is " + this.name + " and I am " + this.age + " years old.");
}
}
const person = new Person("John", 30);
person.sayHello(); // Output: "Hello, my name is John and I am 30 years old."
</script>
In this example, we define a Person
class with a constructor that takes two parameters, name
and age
. The constructor assigns these values to the class properties. We also define a sayHello
method that logs a greeting to the console using the property values. We then create an instance of the Person
class using the new
keyword and call the sayHello
method on the instance.
2. Creating Objects
Objects are instances of classes and are created using the new
keyword. Here's an example of creating multiple Person
objects and accessing their properties:
<script>
class Person {
constructor(name, age) {
this.name = name;
this.age = age;
}
}
const person1 = new Person("John", 30);
const person2 = new Person("Jane", 25);
console.log(person1.name); // Output: "John"
console.log(person2.age); // Output: 25
</script>
In this example, we create two instances of the Person
class, person1
and person2
, with different values for the name
and age
properties. We then access and log the values of these properties using dot notation.
3. Class Inheritance
JavaScript supports class inheritance, allowing you to create a new class based on an existing class. The new class inherits the properties and methods of the base class and can add its own properties and methods. Here's an example of class inheritance:
<script>
class Animal {
constructor(name) {
this.name = name;
}
eat() {
console.log(this.name + " is eating.");
}
}
class Dog extends Animal {
bark() {
console.log(this.name + " is barking.");
}
}
const dog = new Dog("Max");
dog.eat(); // Output: "Max is eating."
dog.bark(); // Output: "Max is barking."
</script>
In this example, we have a base class Animal
with a name
property and an eat
method. We then define a derived class Dog
using the extends
keyword, which inherits from the Animal
class. The Dog
class adds its own method bark
. We create an instance of the Dog
class and call both the inherited eat
method and the bark
method.
Conclusion
JavaScript classes and objects provide a powerful way to structure and organize your code. In this article, we explored examples of creating classes, creating objects, and class inheritance. By utilizing classes and objects, you can create reusable and modular code, making your JavaScript applications more maintainable and scalable.
4.5L
Learners
20+
Instructors
50+
Courses
6.0L
Course enrollments
Future Trending Courses
When selecting, a course, Here are a few areas that are expected to be in demand in the future:.
Future Learning for all
If you’re passionate and ready to dive in, we’d love to join 1:1 classes for you. We’re committed to support our learners and professionals their development and well-being.
View Courses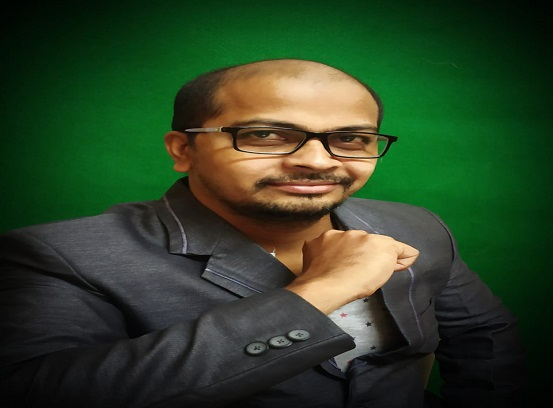
Most Popular Course topics
These are the most popular course topics among Software Courses for learners