Hi there, we’re Harisystems
"Unlock your potential and soar to new heights with our exclusive online courses! Ignite your passion, acquire valuable skills, and embrace limitless possibilities. Don't miss out on our limited-time sale - invest in yourself today and embark on a journey of personal and professional growth. Enroll now and shape your future with knowledge that lasts a lifetime!".
For corporate trainings, projects, and real world experience reach us. We believe that education should be accessible to all, regardless of geographical location or background.
1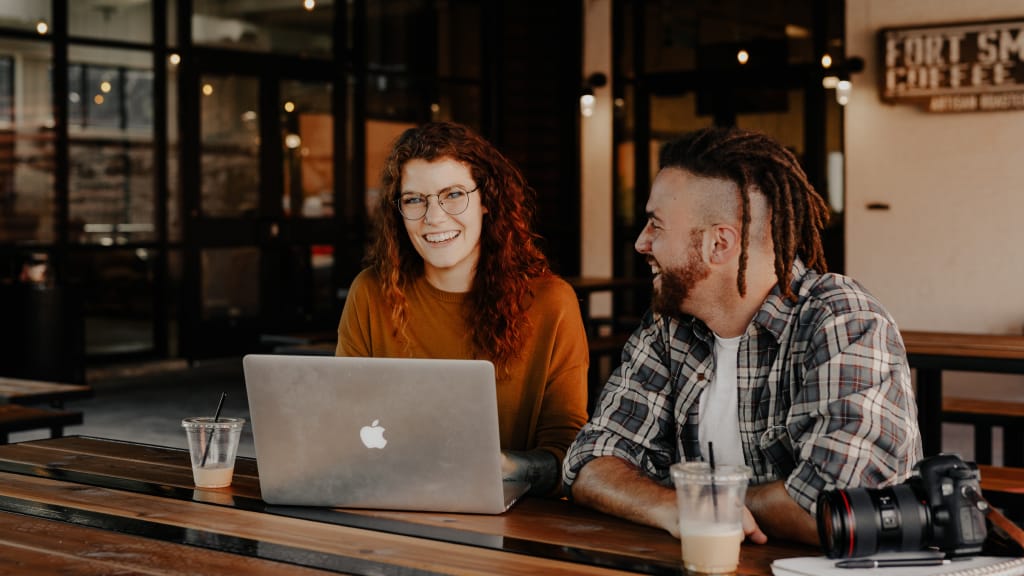
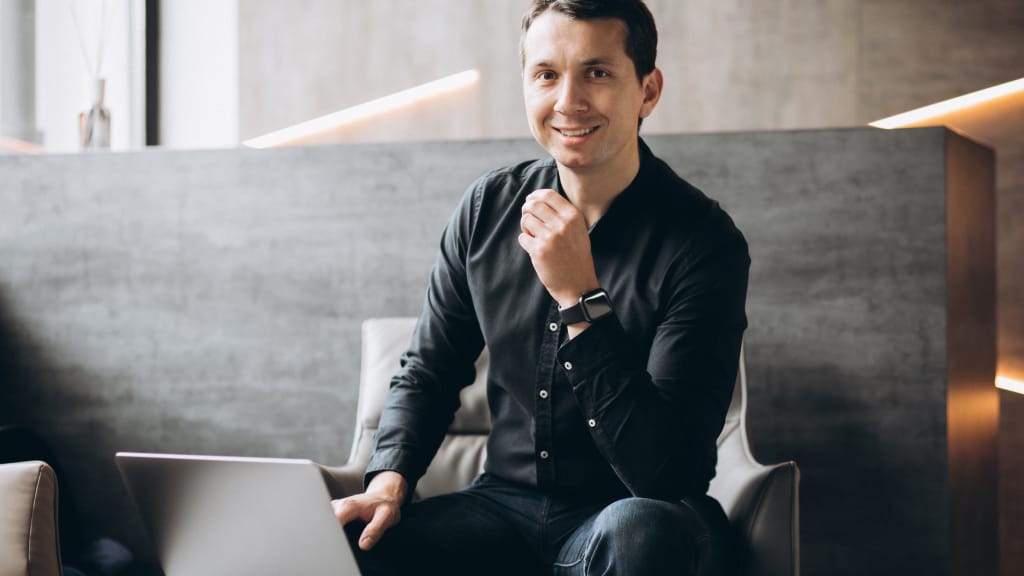
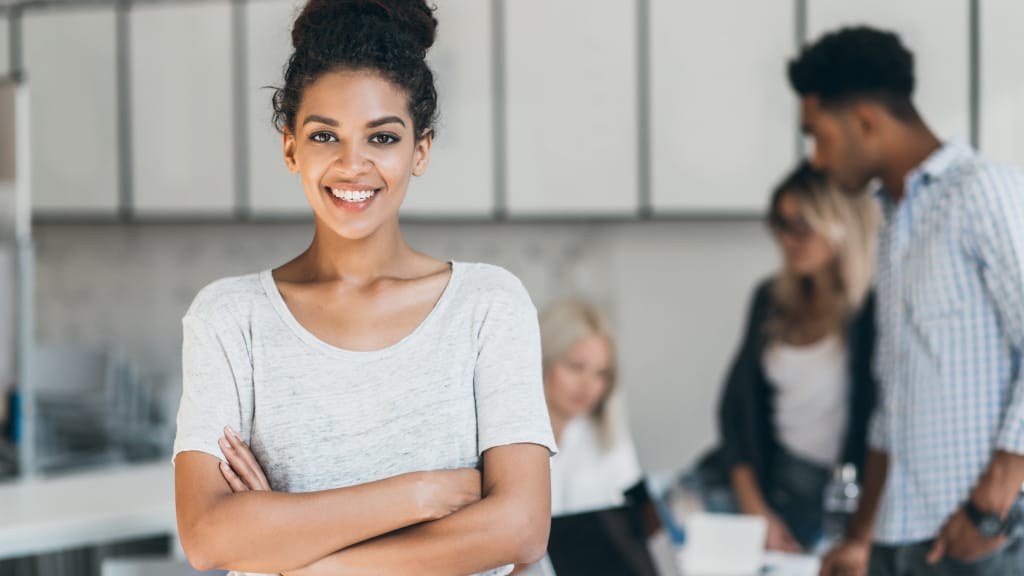
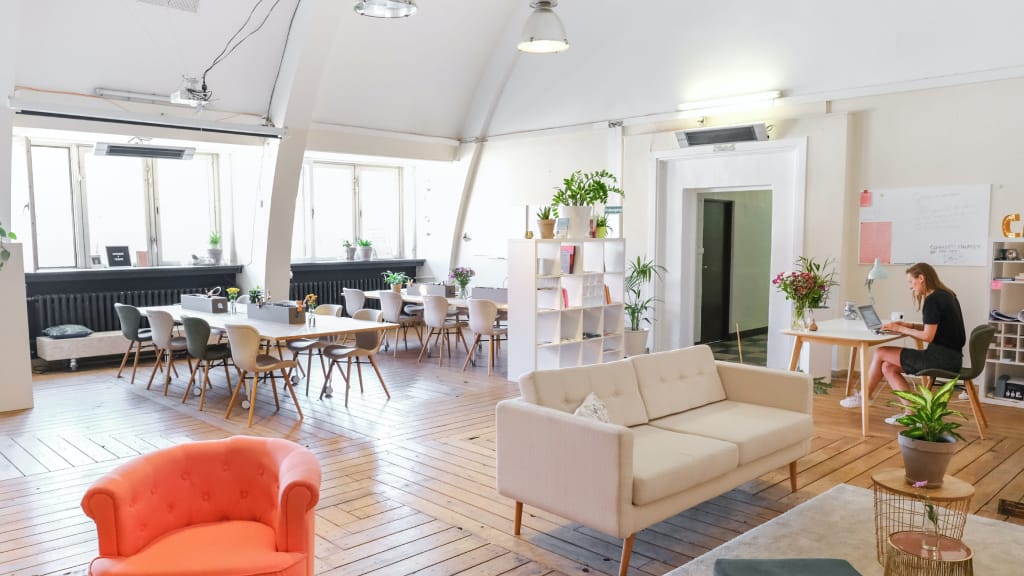
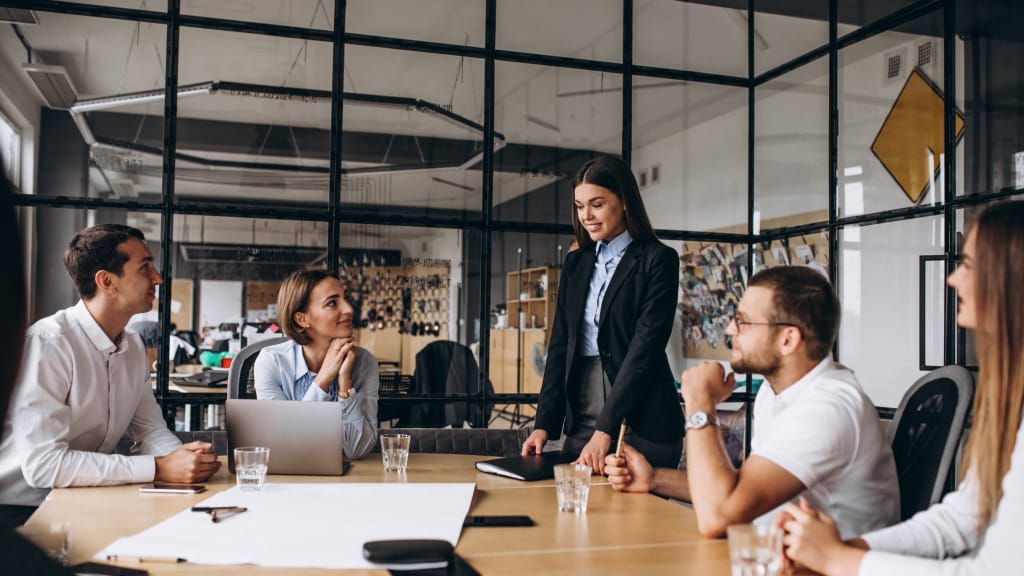
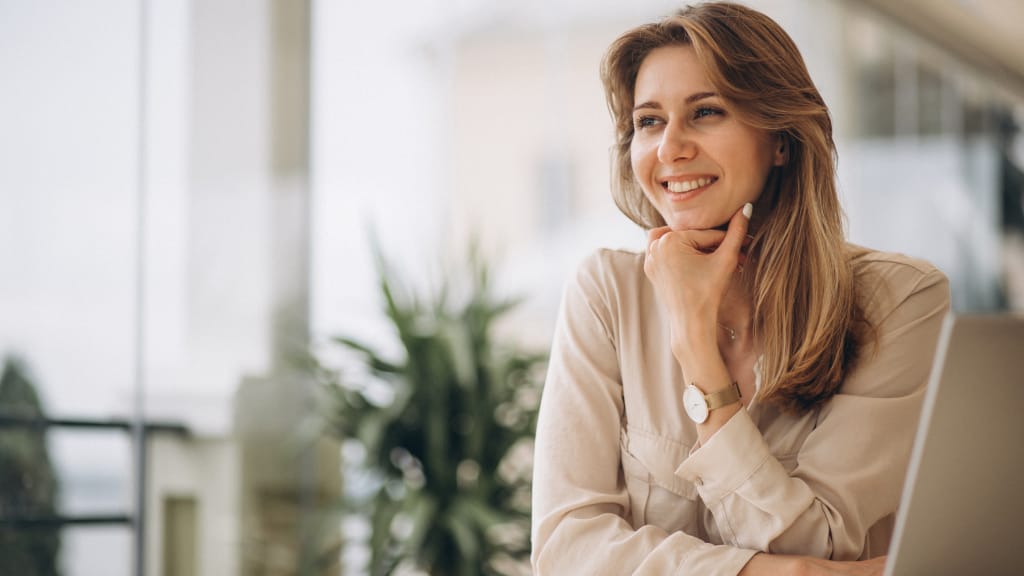
Django Models: Building Database-backed Web Applications
Introduction
In Django, models are the essential building blocks for creating database-backed web applications. Models define the structure and behavior of your data, allowing you to interact with the database through Python code. In this guide, we will explore Django models with examples.
Step 1: Creating a Django Project
Before we dive into models, make sure you have a Django project set up. If you haven't created a Django project yet, you can refer to the previous guide on creating a Django project.
Step 2: Defining a Model
To define a model, open the models.py
file inside your app's directory. Here's an example of a simple model that represents a blog post:
from django.db import models
class BlogPost(models.Model):
title = models.CharField(max_length=200)
content = models.TextField()
pub_date = models.DateTimeField(auto_now_add=True)
In this example, we import the models
module from django.db
and define a class named BlogPost
that inherits from models.Model
. Inside the class, we define three fields: title
(a character field), content
(a text field), and pub_date
(a date and time field).
Step 3: Running Migrations
After defining a model, you need to create the corresponding database table. Django provides a migration system to handle database schema changes. In your terminal, run the following command:
python manage.py makemigrations
python manage.py migrate
The first command, makemigrations
, generates the necessary migration files based on the changes in your models. The second command, migrate
, applies those changes to the database.
Step 4: Interacting with Models
Once the database table is created, you can interact with your models using Django's database API. For example, to create a new blog post:
from myapp.models import BlogPost
post = BlogPost(title='Hello World', content='This is my first blog post.')
post.save()
In this example, we import the BlogPost
model from the myapp.models
module. We create a new instance of the BlogPost
model, set the title
and content
attributes, and call the save()
method to persist the object to the database.
Step 5: Querying Models
You can retrieve data from the database using Django's query API. For example, to retrieve all blog posts:
from myapp.models import BlogPost
posts = BlogPost.objects.all()
In this example, we use the objects.all()
method to retrieve all instances of the BlogPost
model from the database.
Step 6: Performing CRUD Operations
Django models provide convenient methods for performing CRUD (Create, Read, Update, Delete) operations. For example, to update an existing blog post:
post = BlogPost.objects.get(pk=1)
post.title = 'Updated Title'
post.save()
In this example, we retrieve a blog post with the primary key (id) of 1 using the objects.get()
method. We update the title
attribute and save the changes to the database using the save()
method.
Step 7: Adding Relationships
Django models support various types of relationships between entities. For example, to define a one-to-many relationship between a blog post and comments:
from django.db import models
class BlogPost(models.Model):
title = models.CharField(max_length=200)
content = models.TextField()
pub_date = models.DateTimeField(auto_now_add=True)
class Comment(models.Model):
post = models.ForeignKey(BlogPost, on_delete=models.CASCADE)
text = models.TextField()
pub_date = models.DateTimeField(auto_now_add=True)
In this example, we define a new model called Comment
that has a foreign key relationship with the BlogPost
model. The ForeignKey
field represents a one-to-many relationship, where each comment belongs to a specific blog post.
Conclusion
Django models provide a powerful and intuitive way to work with databases in web applications. By following this guide, you have learned how to define models, run migrations, interact with models using the database API, query data, perform CRUD operations, and establish relationships between entities. With Django models, you can build robust and data-driven web applications efficiently.
4.5L
Learners
20+
Instructors
50+
Courses
6.0L
Course enrollments
Future Trending Courses
When selecting, a course, Here are a few areas that are expected to be in demand in the future:.
Future Learning for all
If you’re passionate and ready to dive in, we’d love to join 1:1 classes for you. We’re committed to support our learners and professionals their development and well-being.
View Courses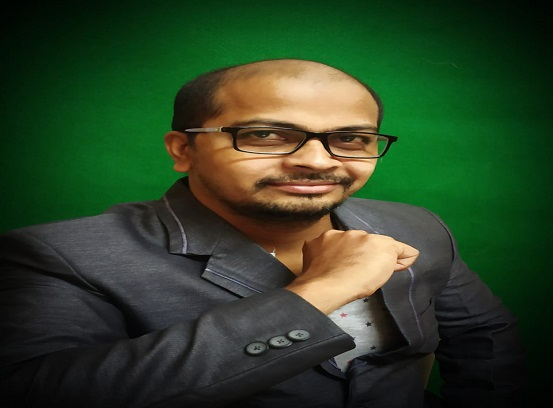
Most Popular Course topics
These are the most popular course topics among Software Courses for learners