Hi there, we’re Harisystems
"Unlock your potential and soar to new heights with our exclusive online courses! Ignite your passion, acquire valuable skills, and embrace limitless possibilities. Don't miss out on our limited-time sale - invest in yourself today and embark on a journey of personal and professional growth. Enroll now and shape your future with knowledge that lasts a lifetime!".
For corporate trainings, projects, and real world experience reach us. We believe that education should be accessible to all, regardless of geographical location or background.
1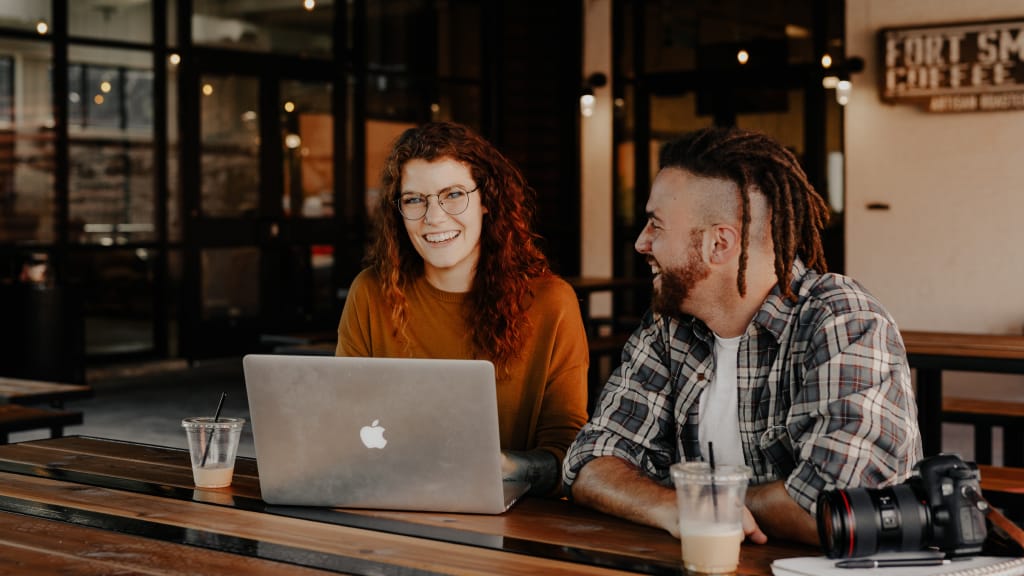
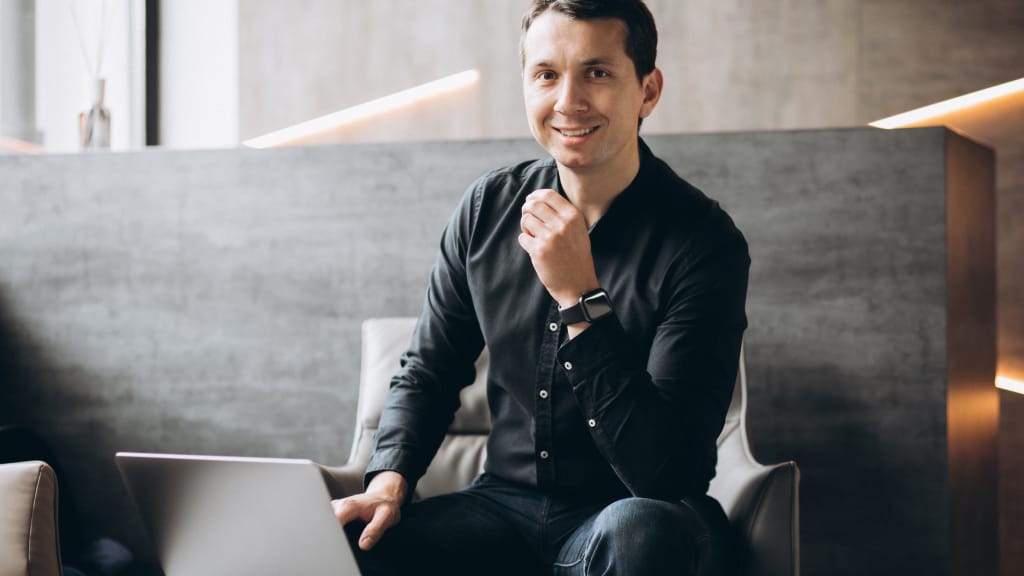
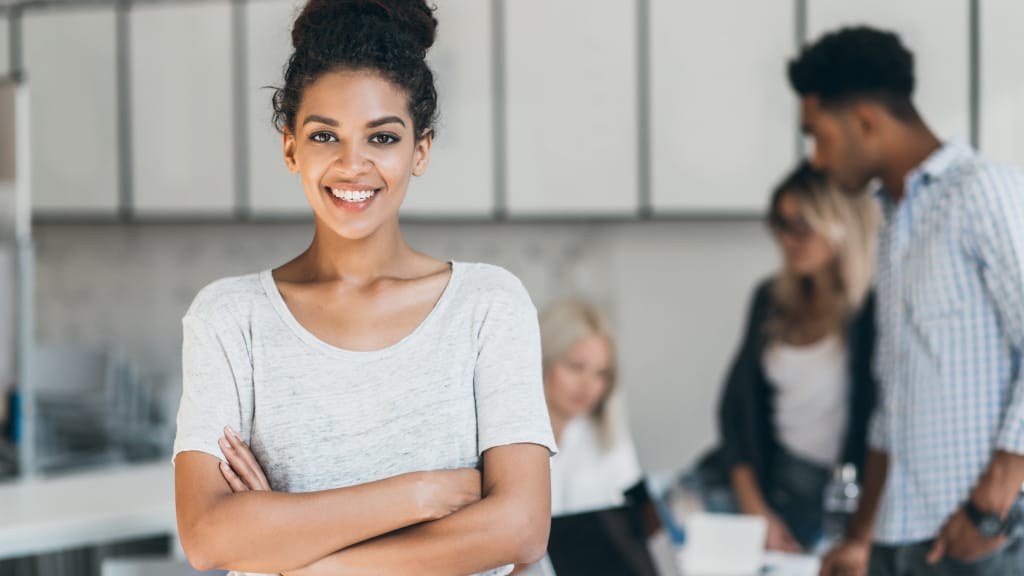
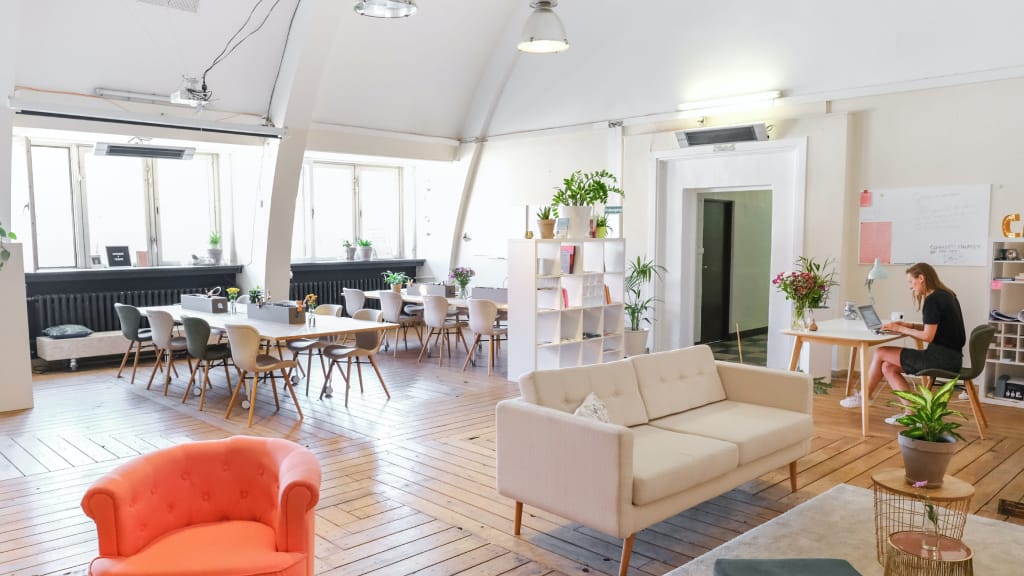
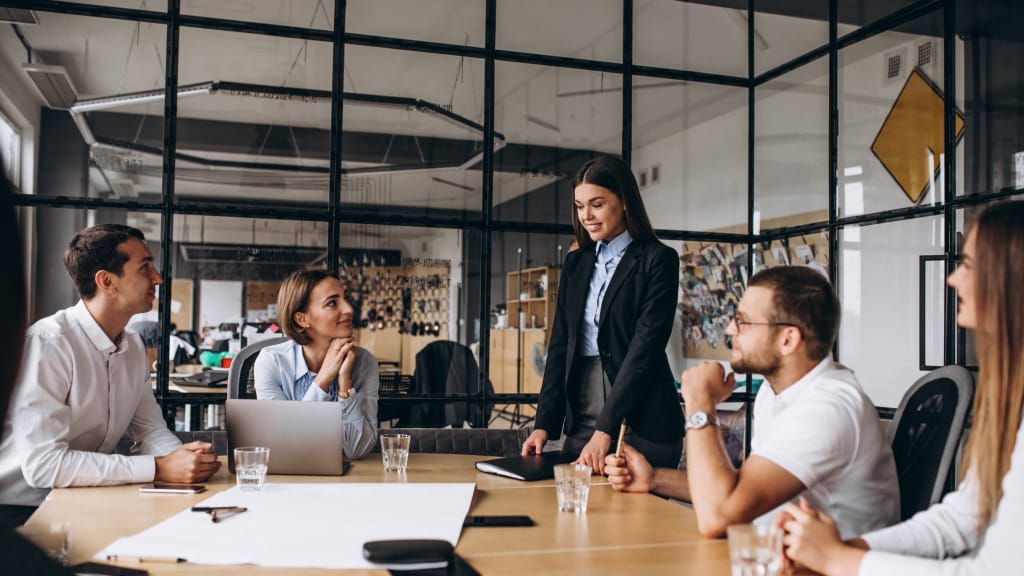
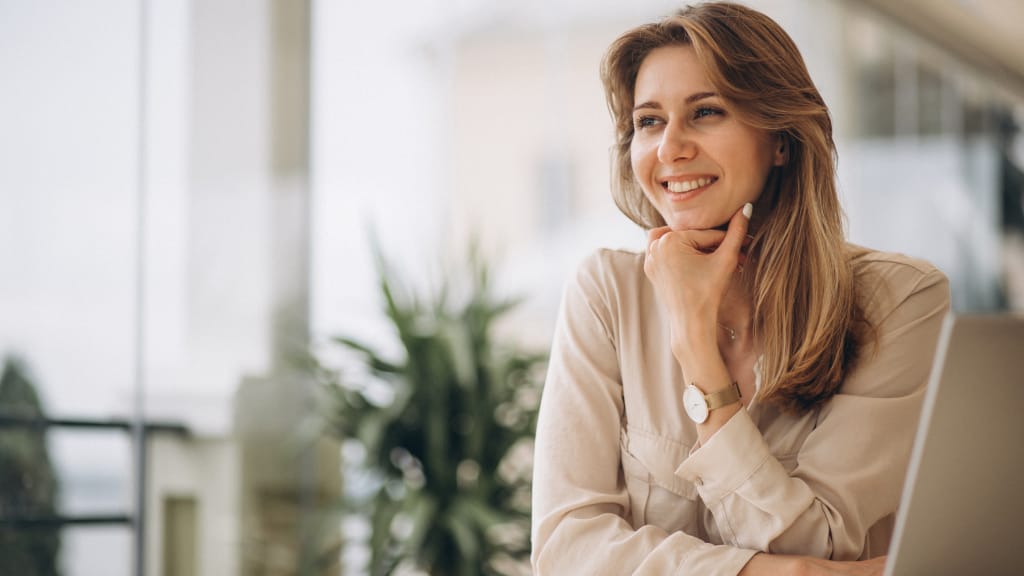
Web Development: Building Reactive UIs with Vue.js
Vue.js is a progressive JavaScript framework for building user interfaces. It focuses on the view layer of web applications and provides reactive and component-based features. Vue.js is known for its simplicity and ease of integration with existing projects. In this article, we will explore the basics of using Vue.js and provide examples of its implementation in web development projects.
What is Vue.js?
Vue.js is a lightweight JavaScript framework that enables developers to build reactive web applications. It follows the MVVM (Model-View-ViewModel) architectural pattern and provides a reactive data-binding system. Vue.js is designed to be incrementally adoptable, meaning you can integrate it into existing projects without any major overhaul.
Setting Up Vue.js
To start using Vue.js in your web development project, you need to include the Vue.js library in your HTML file. You can download Vue.js from the official Vue.js website or use a CDN (Content Delivery Network) to include it:
<script src="https://cdn.jsdelivr.net/npm/vue@2.6.14/dist/vue.js"></script>
Creating a Vue Instance
In Vue.js, you create a Vue instance that represents your application. Here's an example:
<div id="app">
{{ message }}
</div>
<script>
var app = new Vue({
el: '#app',
data: {
message: 'Hello, Vue!'
}
});
</script>
Data Binding
Vue.js provides a convenient way to bind data to the DOM. Here's an example:
<div id="app">
<input v-model="message">
<p>{{ message }}</p>
</div>
<script>
var app = new Vue({
el: '#app',
data: {
message: 'Hello, Vue!'
}
});
</script>
Directives
Vue.js uses directives to extend HTML with additional functionality. Directives are special attributes prefixed with "v-". Here's an example:
<div id="app">
<p v-if="showMessage">{{ message }}</p>
<button v-on:click="showMessage = true">Show Message</button>
</div>
<script>
var app = new Vue({
el: '#app',
data: {
message: 'Hello, Vue!',
showMessage: false
}
});
</script>
Computed Properties
Vue.js allows you to define computed properties that depend on other data properties. Computed properties are cached and updated only when their dependencies change. Here's an example:
<div id="app">
<input v-model="firstName">
<input v-model="lastName">
<p>Full Name: {{ fullName }}</p>
</div>
<script>
var app = new Vue({
el: '#app',
data: {
firstName: '',
lastName: ''
},
computed: {
fullName: function() {
return this.firstName + ' ' + this.lastName;
}
}
});
</script>
Handling Events
Vue.js provides event handling capabilities similar to regular HTML. Here's an example:
<div id="app">
<button v-on:click="increment">Increment</button>
<p>Counter: {{ counter }}</p>
</div>
<script>
var app = new Vue({
el: '#app',
data: {
counter: 0
},
methods: {
increment: function() {
this.counter++;
}
}
});
</script>
Conclusion
Vue.js is a powerful JavaScript framework that simplifies web development by providing reactive and component-based features. Its simplicity and ease of integration make it a popular choice for building user interfaces. Understanding the basics of Vue.js and its implementation in web development is essential for creating modern and dynamic web applications. Explore the Vue.js documentation, experiment with its features, and leverage the framework to build interactive and responsive user interfaces in your web development projects.
4.5L
Learners
20+
Instructors
50+
Courses
6.0L
Course enrollments
Future Trending Courses
When selecting, a course, Here are a few areas that are expected to be in demand in the future:.
Future Learning for all
If you’re passionate and ready to dive in, we’d love to join 1:1 classes for you. We’re committed to support our learners and professionals their development and well-being.
View Courses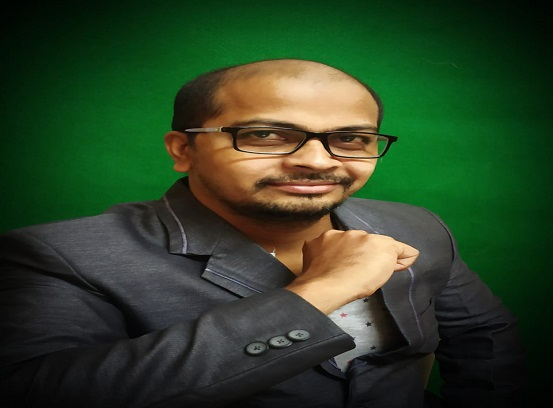
Most Popular Course topics
These are the most popular course topics among Software Courses for learners