Hi there, we’re Harisystems
"Unlock your potential and soar to new heights with our exclusive online courses! Ignite your passion, acquire valuable skills, and embrace limitless possibilities. Don't miss out on our limited-time sale - invest in yourself today and embark on a journey of personal and professional growth. Enroll now and shape your future with knowledge that lasts a lifetime!".
For corporate trainings, projects, and real world experience reach us. We believe that education should be accessible to all, regardless of geographical location or background.
1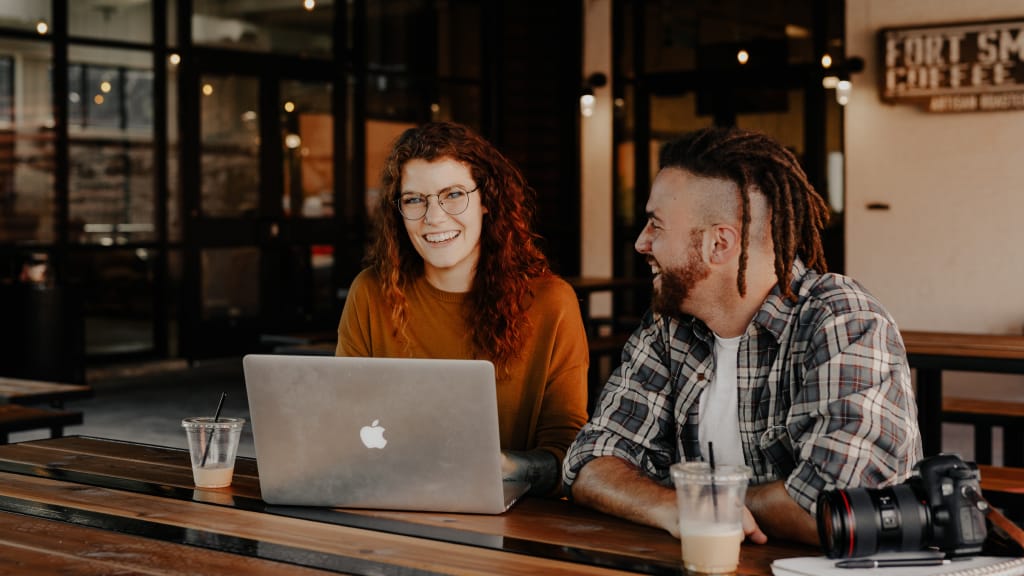
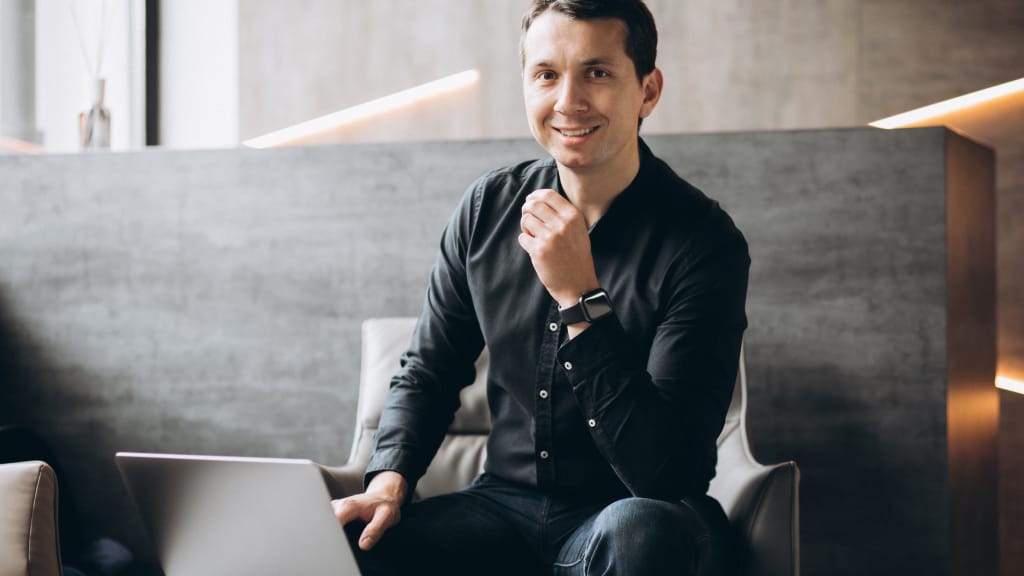
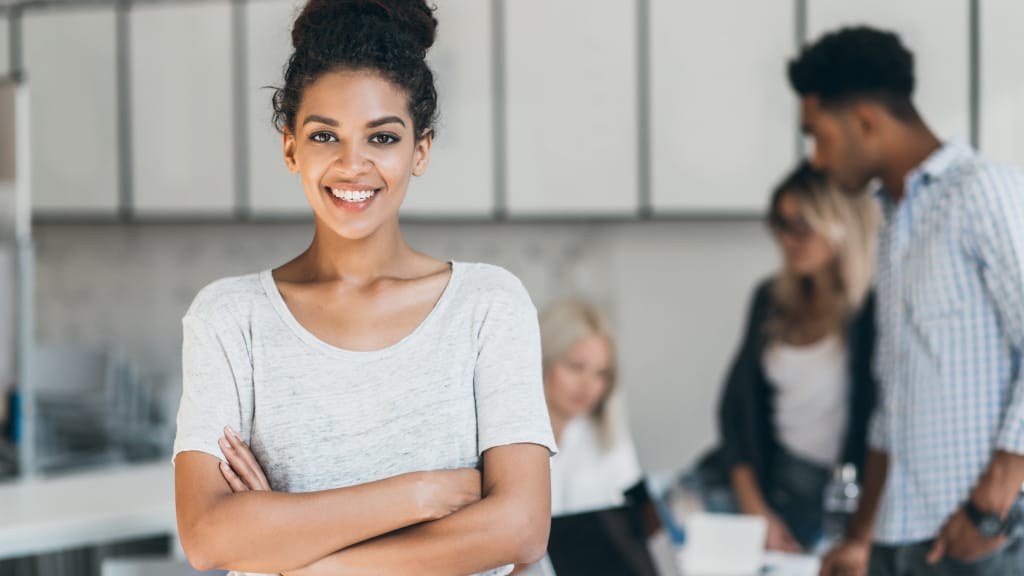
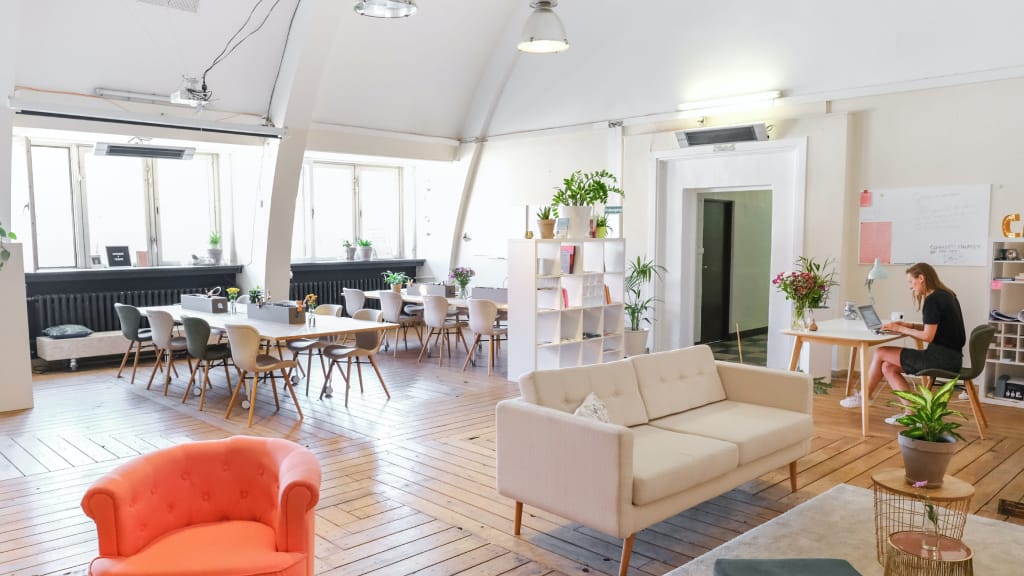
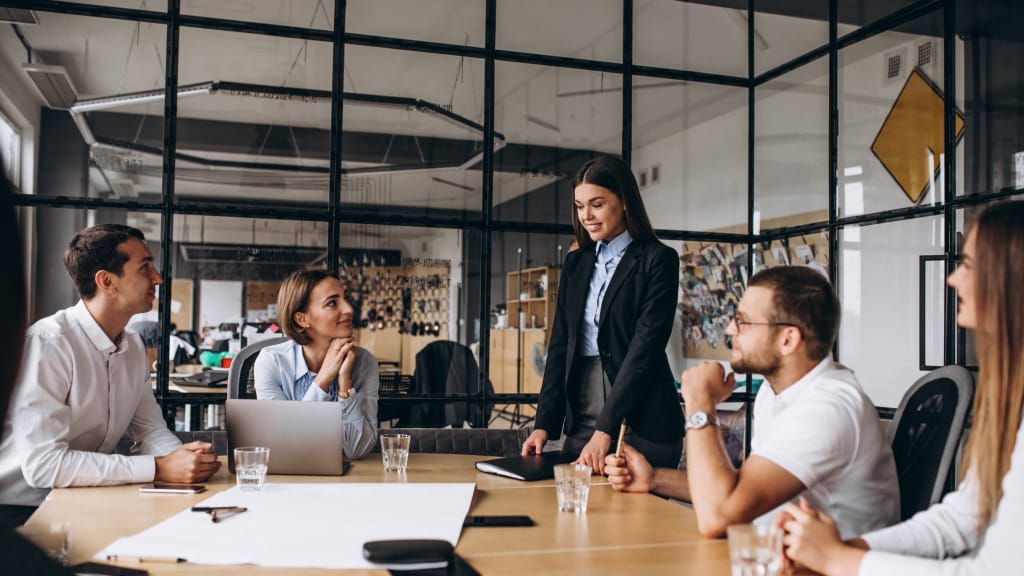
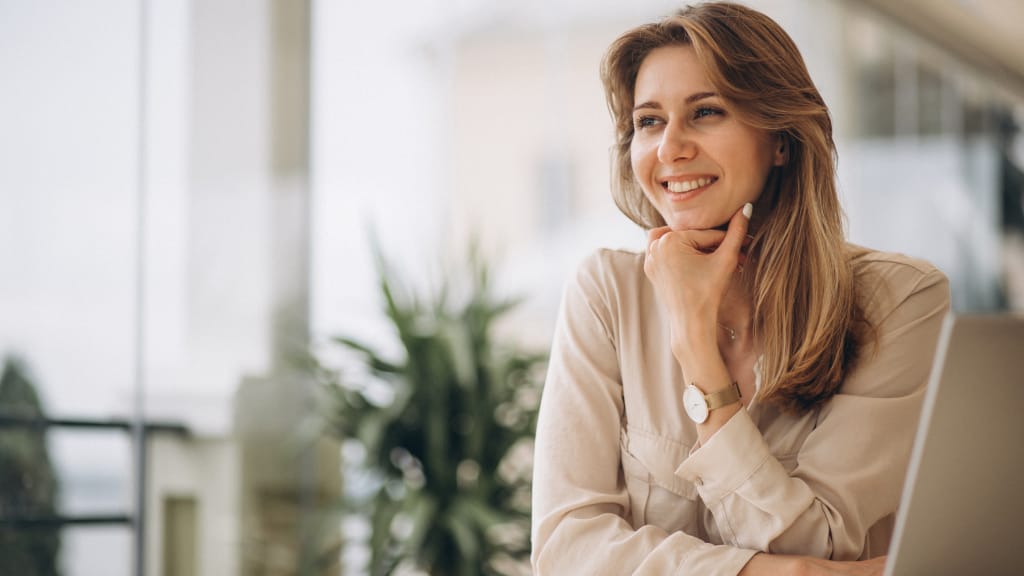
JavaScript Frameworks: Enhancing Web Development with Power and Efficiency
JavaScript frameworks have revolutionized the way web applications are built, allowing developers to streamline their development process and create powerful and efficient web solutions. In this article, we will explore some popular JavaScript frameworks and their key features, along with examples of how they can be used to enhance web development.
1. React.js
React.js, developed by Facebook, is a component-based JavaScript library used for building user interfaces. It offers a declarative approach to building UI components, making it easier to manage complex user interfaces. React.js utilizes a virtual DOM (Document Object Model) for efficient rendering and updates only the necessary components, resulting in better performance. Here's a simple example of a React component:
<script src="https://unpkg.com/react/umd/react.development.js"></script>
<script src="https://unpkg.com/react-dom/umd/react-dom.development.js"></script>
<div id="root"></div>
<script>
const App = () => {
return (
<div>
<h1>Hello, React!</h1>
<p>This is a React component.</p>
</div>
);
};
ReactDOM.render(<App />, document.getElementById('root'));
</script>
In this example, we define a simple React component called "App" that renders a heading and a paragraph. The ReactDOM.render function is used to render the component into the HTML element with the id "root". React.js's component-based architecture and efficient rendering make it a popular choice for building dynamic and interactive user interfaces.
2. Angular
Angular, developed by Google, is a comprehensive and feature-rich JavaScript framework for building web applications. It follows the Model-View-Controller (MVC) architectural pattern and provides a robust set of tools and features. Angular offers features like two-way data binding, dependency injection, and a powerful template system. Here's an example of an Angular component:
<script src="https://unpkg.com/angular/angular.js"></script>
<div ng-app="myApp">
<div ng-controller="MyController">
<h1>Hello, Angular!</h1>
<p>This is an Angular component.</p>
</div>
</div>
<script>
angular.module('myApp', [])
.controller('MyController', function($scope) {
// Controller logic goes here
});
</script>
In this example, we define an Angular module called "myApp" and a controller called "MyController". The ng-app and ng-controller directives are used to define the scope of the Angular application and the specific controller to be used. Angular's powerful features and extensive documentation make it a popular choice for large-scale web applications.
3. Vue.js
Vue.js is a lightweight and progressive JavaScript framework that is gaining popularity among developers. It focuses on the view layer and provides a simple and intuitive syntax for building user interfaces. Vue.js offers features like declarative rendering, component-based architecture, and two-way data binding. Here's an example of a Vue.js component:
<script src="https://unpkg.com/vue/dist/vue.js"></script>
<div id="app">
<h1>Hello, Vue.js!</h1>
<p>This is a Vue.js component.</p>
</div>
<script>
new Vue({
el: '#app',
template: `
<div>
<h1>Hello, Vue.js!</h1>
<p>This is a Vue.js component.</p>
</div>
`
});
</script>
In this example, we create a Vue instance and associate it with the HTML element with the id "app". The template property defines the structure and content of the component. Vue.js's simplicity and ease of integration make it a great choice for building smaller-scale applications or adding interactivity to existing projects.
Conclusion
JavaScript frameworks have significantly transformed the web development landscape, empowering developers to build sophisticated and efficient web applications. React.js, Angular, and Vue.js are just a few examples of the many JavaScript frameworks available today. By leveraging the power and features offered by these frameworks, developers can streamline their development process, create reusable components, and deliver exceptional user experiences. Whether you're building a simple website or a complex web application, exploring and utilizing JavaScript frameworks can greatly enhance your web development journey.
4.5L
Learners
20+
Instructors
50+
Courses
6.0L
Course enrollments
Future Trending Courses
When selecting, a course, Here are a few areas that are expected to be in demand in the future:.
Future Learning for all
If you’re passionate and ready to dive in, we’d love to join 1:1 classes for you. We’re committed to support our learners and professionals their development and well-being.
View Courses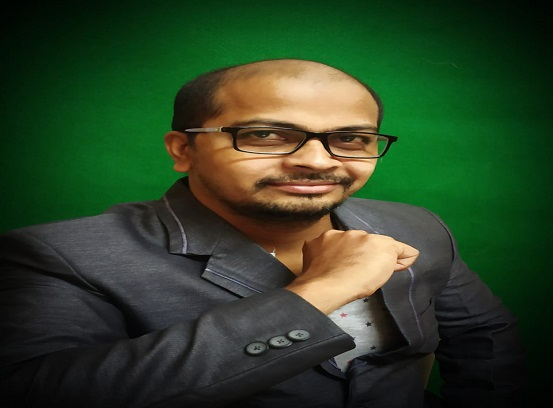
Most Popular Course topics
These are the most popular course topics among Software Courses for learners