Hi there, we’re Harisystems
"Unlock your potential and soar to new heights with our exclusive online courses! Ignite your passion, acquire valuable skills, and embrace limitless possibilities. Don't miss out on our limited-time sale - invest in yourself today and embark on a journey of personal and professional growth. Enroll now and shape your future with knowledge that lasts a lifetime!".
For corporate trainings, projects, and real world experience reach us. We believe that education should be accessible to all, regardless of geographical location or background.
1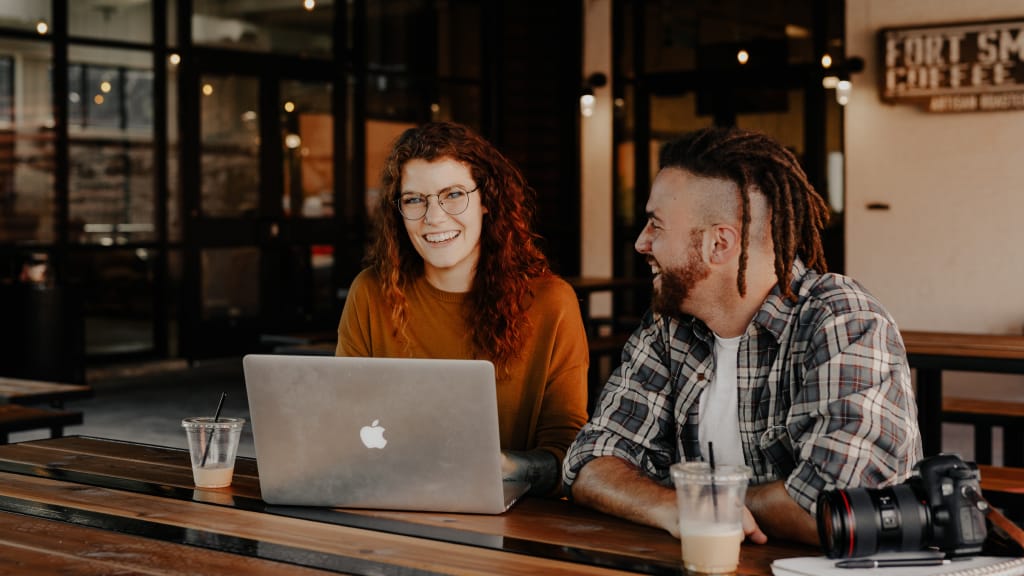
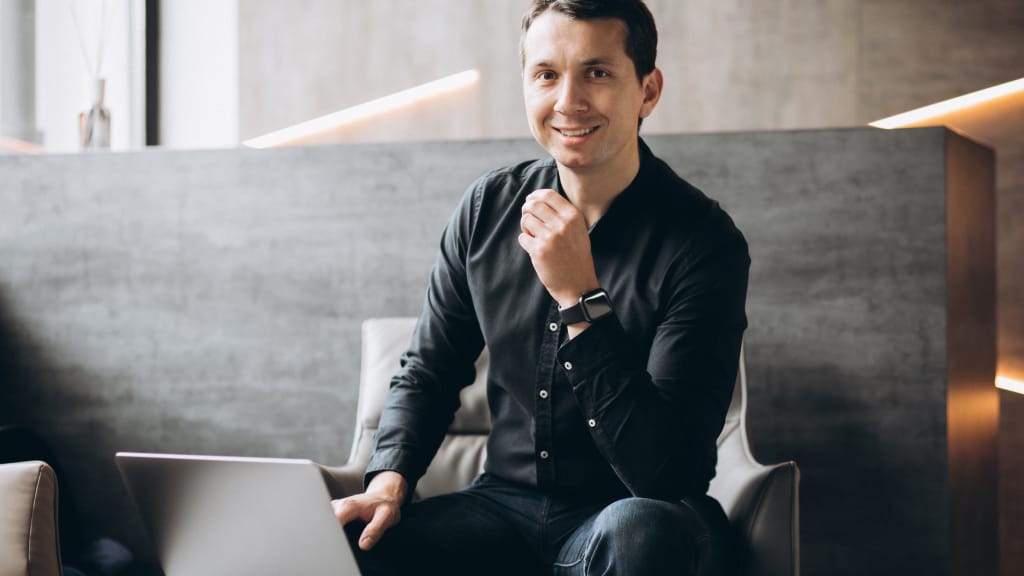
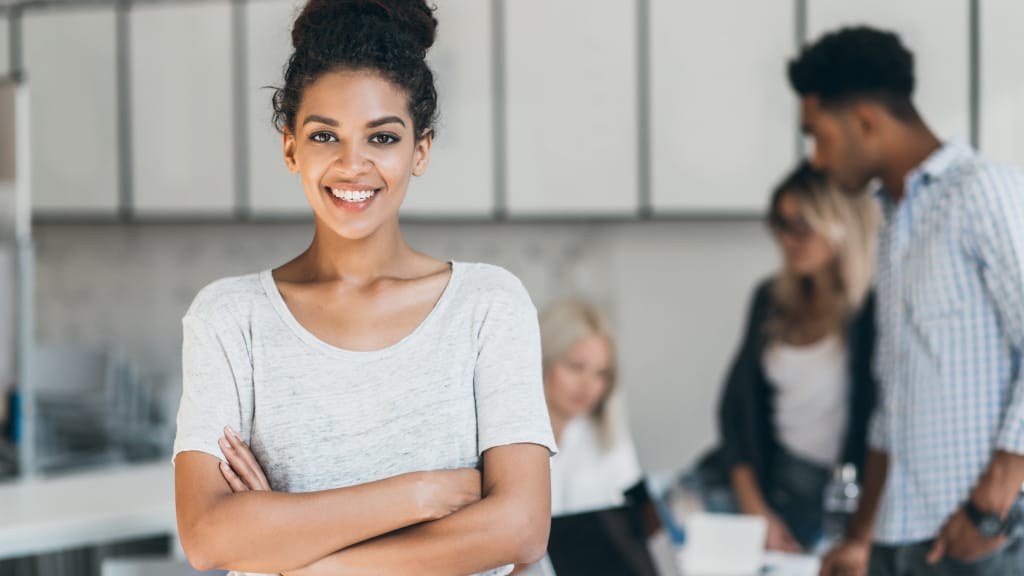
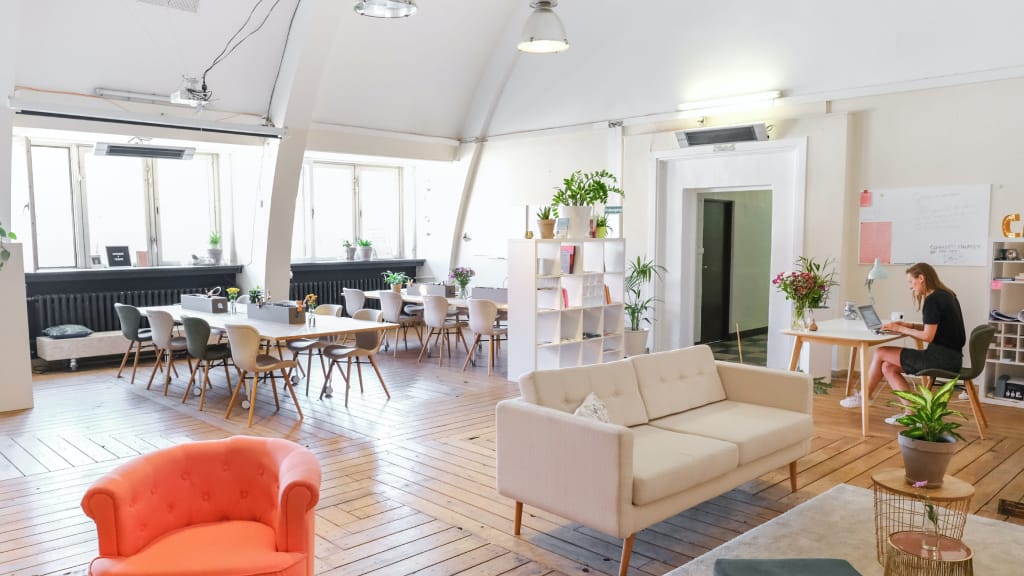
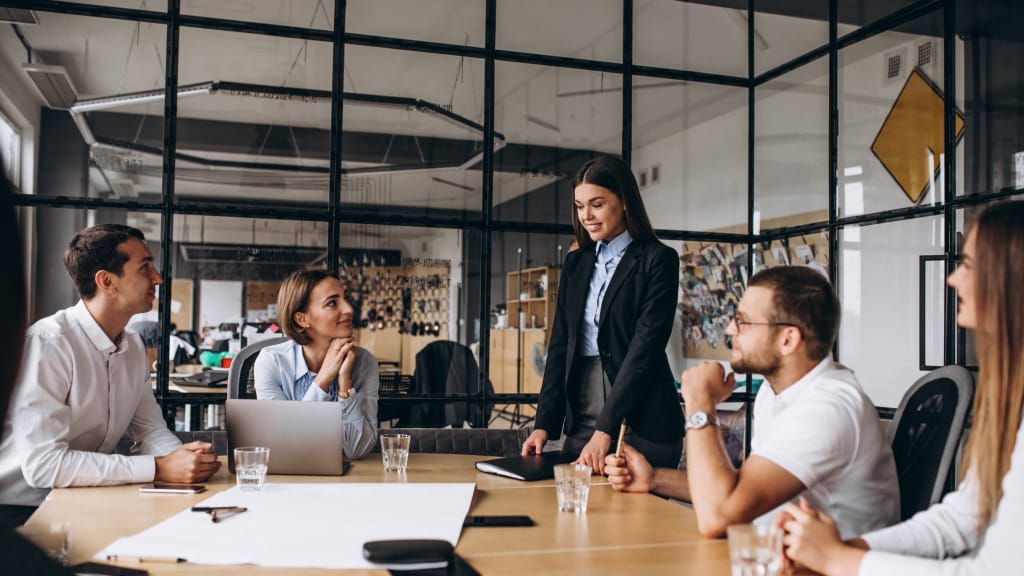
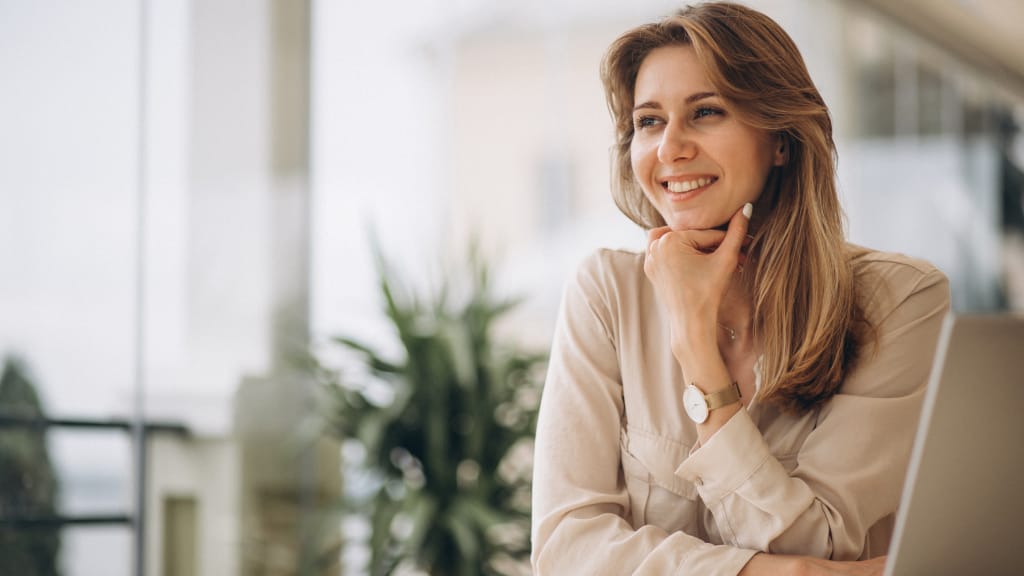
Understanding JavaScript Encapsulation: Examples and Usage
Encapsulation is a fundamental principle of object-oriented programming that allows you to bundle data and related methods together into a single unit, known as a class. JavaScript, being a flexible and dynamic language, provides several techniques to achieve encapsulation. In this article, we will explore JavaScript encapsulation and provide examples of its usage.
1. Using Constructor Functions
One way to achieve encapsulation in JavaScript is by using constructor functions and the this
keyword to define and access object properties. Here's an example:
<script>
function Car(make, model) {
let _make = make; // private property
let _model = model; // private property
this.getMake = function() {
return _make;
};
this.getModel = function() {
return _model;
};
}
const myCar = new Car("Toyota", "Camry");
console.log(myCar.getMake()); // Output: "Toyota"
console.log(myCar.getModel()); // Output: "Camry"
</script>
In this example, we define a Car
constructor function that takes make
and model
as arguments. We use private variables (_make
and _model
) to encapsulate the data and create getter methods (getMake()
and getModel()
) to access the private properties. The encapsulated data is not directly accessible from outside the object, ensuring data privacy and encapsulation.
2. Using ES6 Classes
With the introduction of ES6, JavaScript provides a more declarative way to define classes and achieve encapsulation using the class
syntax. Here's an example:
<script>
class Car {
constructor(make, model) {
let _make = make; // private property
let _model = model; // private property
this.getMake = function() {
return _make;
};
this.getModel = function() {
return _model;
};
}
}
const myCar = new Car("Toyota", "Camry");
console.log(myCar.getMake()); // Output: "Toyota"
console.log(myCar.getModel()); // Output: "Camry"
</script>
In this example, we define a Car
class using the class
keyword. The constructor function is used to initialize the private properties (_make
and _model
) and create the getter methods (getMake()
and getModel()
). The encapsulated data remains hidden from outside the class, providing encapsulation and data privacy.
3. Using Closures
Another approach to achieve encapsulation in JavaScript is by utilizing closures. Here's an example:
<script>
function createCounter() {
let count = 0; // private variable
return {
increment() {
count++;
},
decrement() {
count--;
},
getCount() {
return count;
}
};
}
const counter = createCounter();
console.log(counter.getCount()); // Output: 0
counter.increment();
counter.increment();
console.log(counter.getCount()); // Output: 2
counter.decrement();
console.log(counter.getCount()); // Output: 1
</script>
In this example, we define a function createCounter()
that encapsulates a private variable count
and returns an object with methods to increment, decrement, and get the count. The count variable remains hidden from outside the function, and the returned object provides controlled access to it, achieving encapsulation.
Conclusion
JavaScript encapsulation is a powerful concept that allows you to hide and protect data within objects. By using techniques like constructor functions, ES6 classes, and closures, you can achieve encapsulation and ensure data privacy. In this article, we explored various methods to achieve encapsulation in JavaScript and provided examples of their usage. Understanding encapsulation in JavaScript can lead to more modular, maintainable, and secure code in your applications.
4.5L
Learners
20+
Instructors
50+
Courses
6.0L
Course enrollments
Future Trending Courses
When selecting, a course, Here are a few areas that are expected to be in demand in the future:.
Future Learning for all
If you’re passionate and ready to dive in, we’d love to join 1:1 classes for you. We’re committed to support our learners and professionals their development and well-being.
View Courses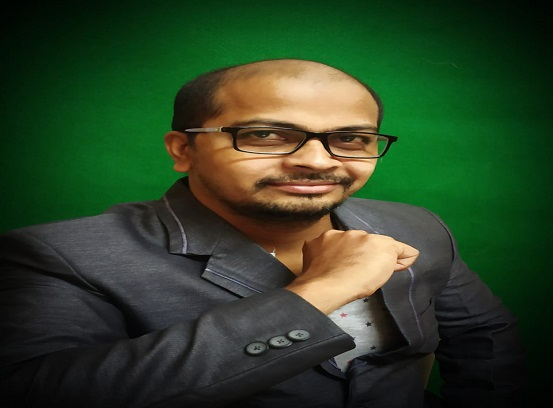
Most Popular Course topics
These are the most popular course topics among Software Courses for learners