Hi there, we’re Harisystems
"Unlock your potential and soar to new heights with our exclusive online courses! Ignite your passion, acquire valuable skills, and embrace limitless possibilities. Don't miss out on our limited-time sale - invest in yourself today and embark on a journey of personal and professional growth. Enroll now and shape your future with knowledge that lasts a lifetime!".
For corporate trainings, projects, and real world experience reach us. We believe that education should be accessible to all, regardless of geographical location or background.
1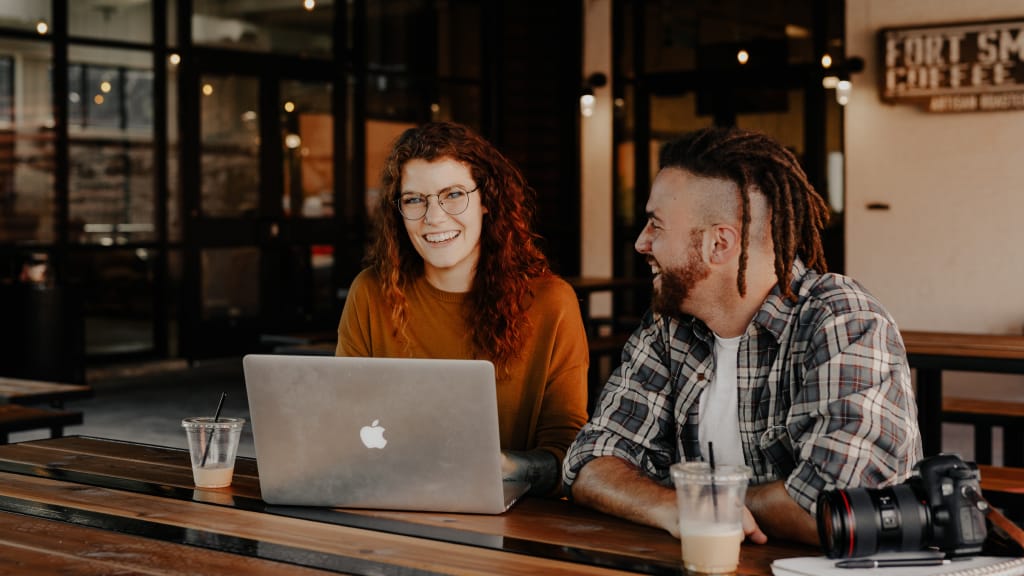
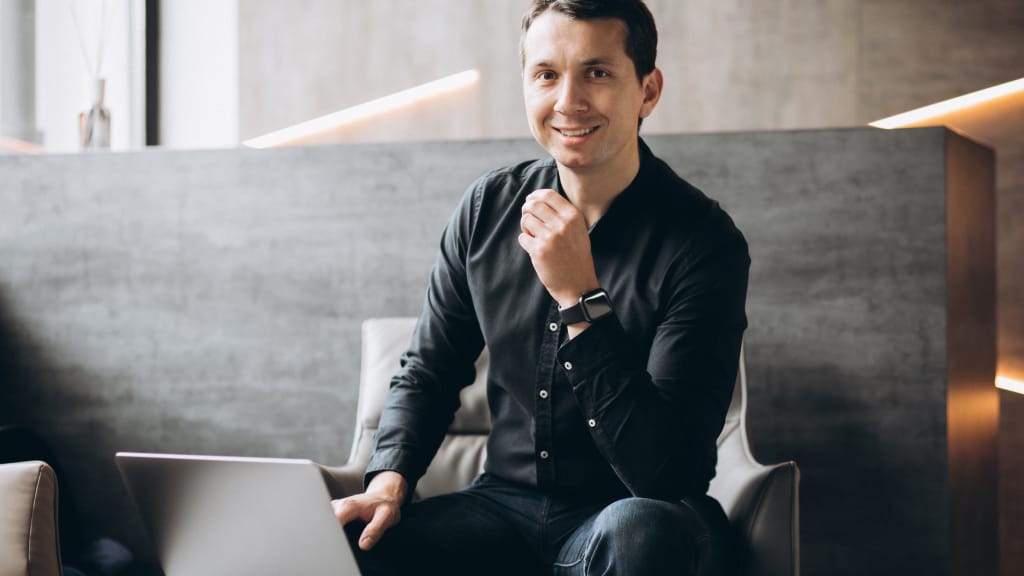
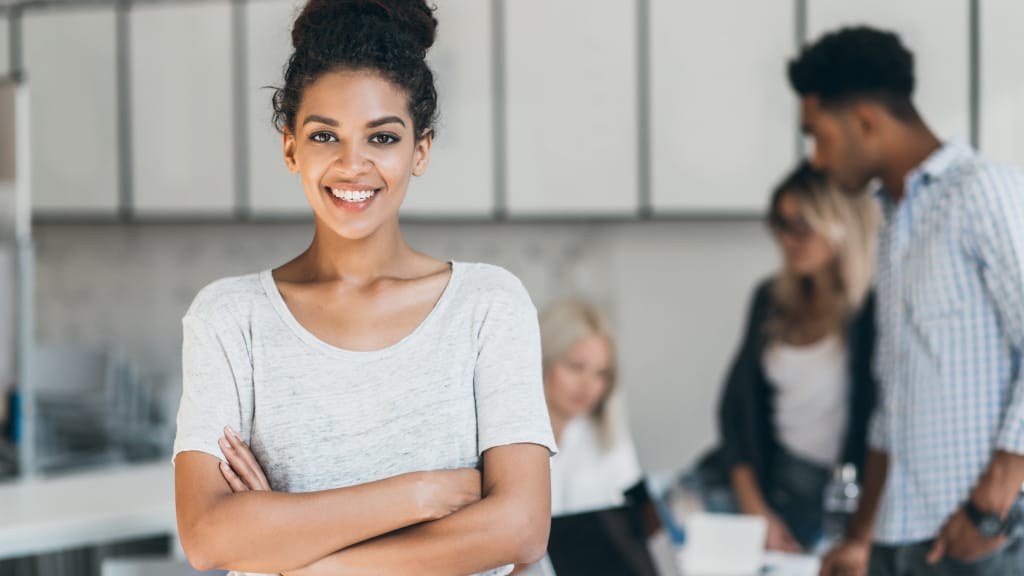
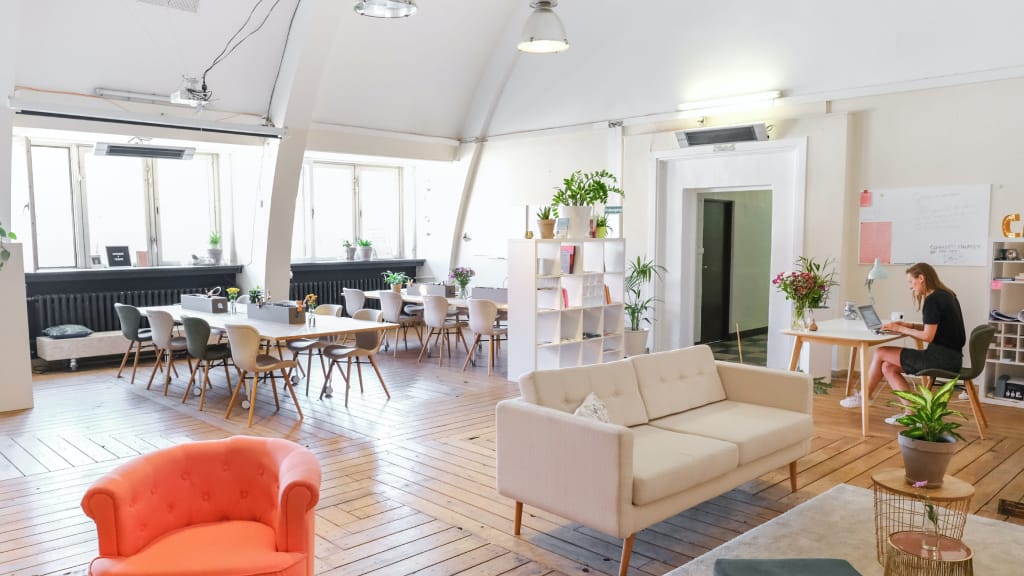
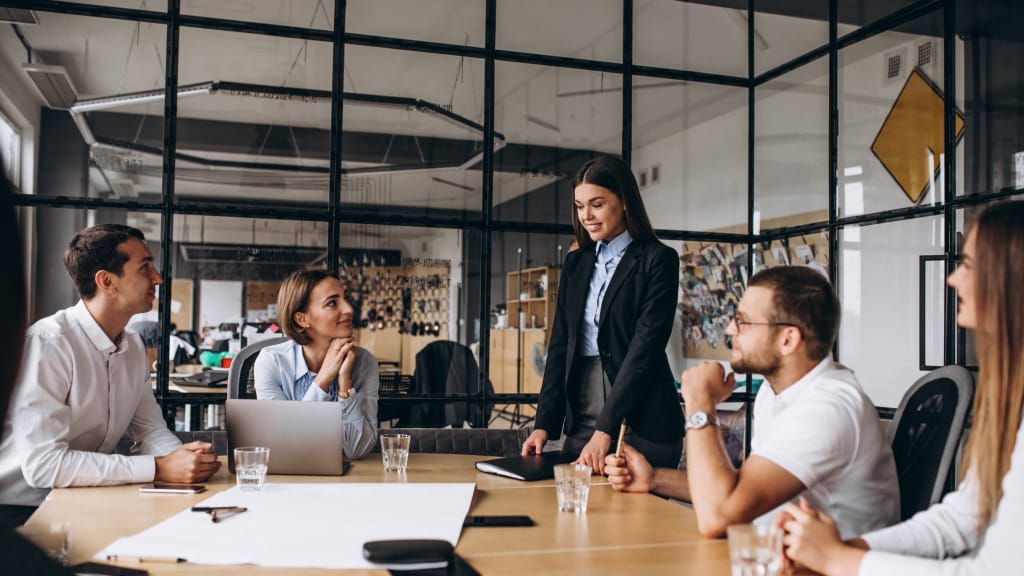
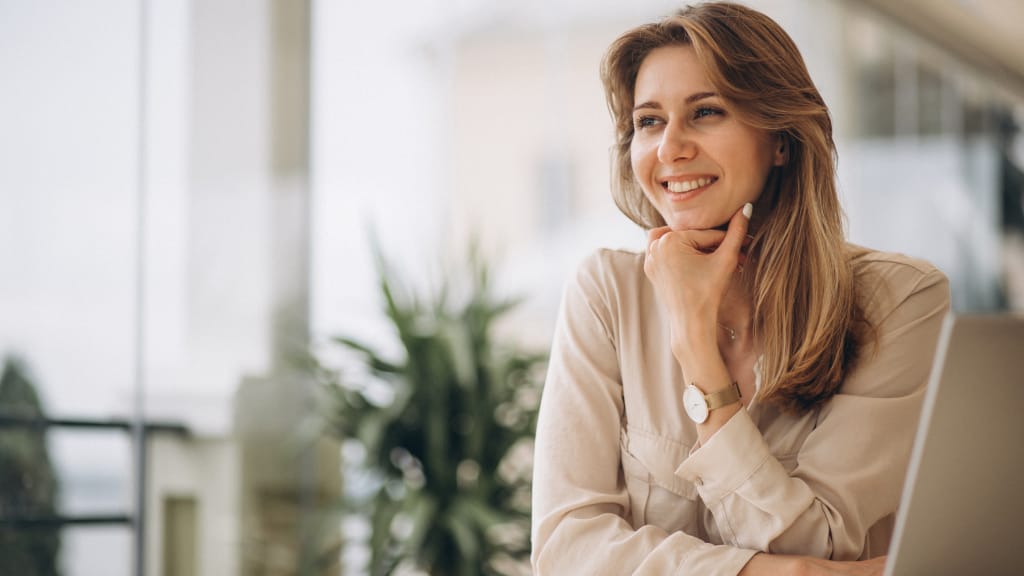
Java Strings: Manipulating Textual Data
In Java, strings are used to represent and manipulate textual data. Strings are a sequence of characters enclosed in double quotes. In this article, we will explore the concept of strings in Java and provide examples to help you understand their usage.
Creating Strings
In Java, you can create strings using the String
class. Here's an example:
String message = "Hello, World!";
The above code creates a string variable named message
with the value "Hello, World!"
.
String Concatenation
You can concatenate strings using the +
operator. Here's an example:
String firstName = "John";
String lastName = "Doe";
String fullName = firstName + " " + lastName;
The above code concatenates the strings firstName
, a space, and lastName
to form the string fullName
with the value "John Doe"
.
String Length
You can determine the length of a string using the length()
method. Here's an example:
String message = "Hello, World!";
int length = message.length();
System.out.println(length); // Output: 13
In the above code, the length()
method returns the number of characters in the string message
.
String Comparison
You can compare strings using the equals()
method or the comparison operators (==
, !=
, >
, <
, >=
, <=
). Here's an example:
String str1 = "Hello";
String str2 = "World";
boolean isEqual = str1.equals(str2);
boolean isGreaterThan = str1.compareTo(str2) > 0;
System.out.println(isEqual); // Output: false
System.out.println(isGreaterThan); // Output: true
In the above code, the equals()
method compares the contents of str1
and str2
for equality. The compareTo()
method compares the two strings lexicographically and returns a positive integer if str1
is greater than str2
.
String Manipulation
The String
class provides various methods for manipulating strings. Here are a few commonly used methods:
- toUpperCase(): Converts the string to uppercase. Example:
String upperCase = "hello".toUpperCase();
- toLowerCase(): Converts the string to lowercase. Example:
String lowerCase = "WORLD".toLowerCase();
- substring(): Extracts a substring from the original string. Example:
String sub = "Hello, World!".substring(7, 12);
- replace(): Replaces a specified character or substring with another. Example:
String replaced = "Hello".replace("H", "J");
- split(): Splits the string into an array of substrings based on a delimiter. Example:
String[] parts = "Hello,World".split(",");
String Formatting
You can format strings using the String.format()
method or the %
symbol. Here's an example:
String name = "John";
int age = 30;
String formattedString = String.format("My name is %s and I am %d years old.", name, age);
The above code formats the string by replacing %s
with the value of name
and %d
with the value of age
.
Conclusion
Strings are essential for working with textual data in Java. In this article, we explored the concept of strings, including creating strings, concatenating strings, determining string length, comparing strings, manipulating strings, and formatting strings. By understanding string manipulation techniques, you can effectively work with textual data in your Java programs. Continuously practice using strings and explore more advanced topics, such as regular expressions, to enhance your Java programming skills.
4.5L
Learners
20+
Instructors
50+
Courses
6.0L
Course enrollments
Future Trending Courses
When selecting, a course, Here are a few areas that are expected to be in demand in the future:.
Future Learning for all
If you’re passionate and ready to dive in, we’d love to join 1:1 classes for you. We’re committed to support our learners and professionals their development and well-being.
View Courses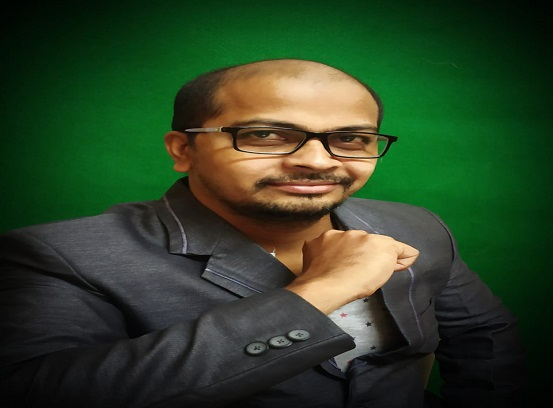
Most Popular Course topics
These are the most popular course topics among Software Courses for learners