Hi there, we’re Harisystems
"Unlock your potential and soar to new heights with our exclusive online courses! Ignite your passion, acquire valuable skills, and embrace limitless possibilities. Don't miss out on our limited-time sale - invest in yourself today and embark on a journey of personal and professional growth. Enroll now and shape your future with knowledge that lasts a lifetime!".
For corporate trainings, projects, and real world experience reach us. We believe that education should be accessible to all, regardless of geographical location or background.
1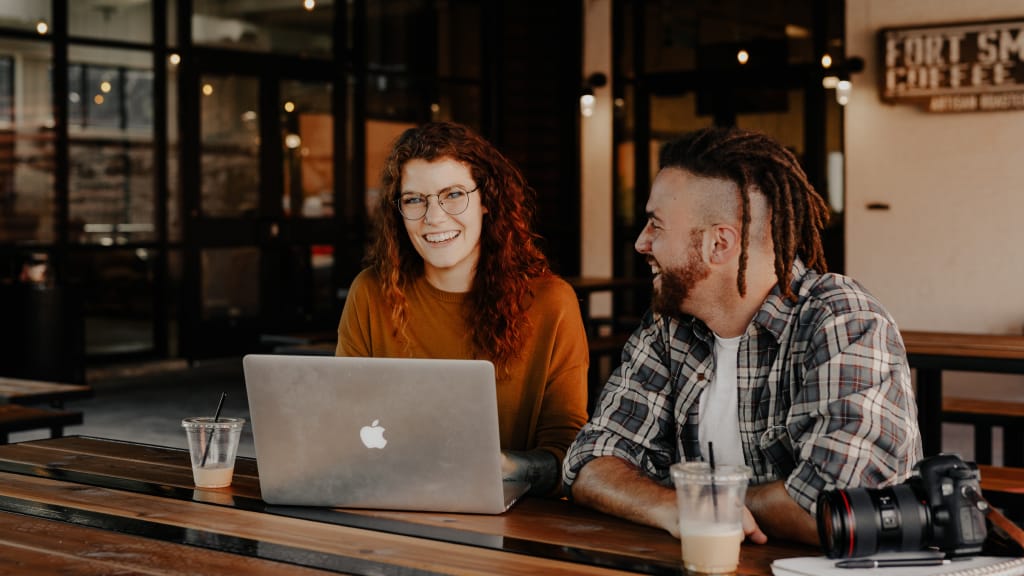
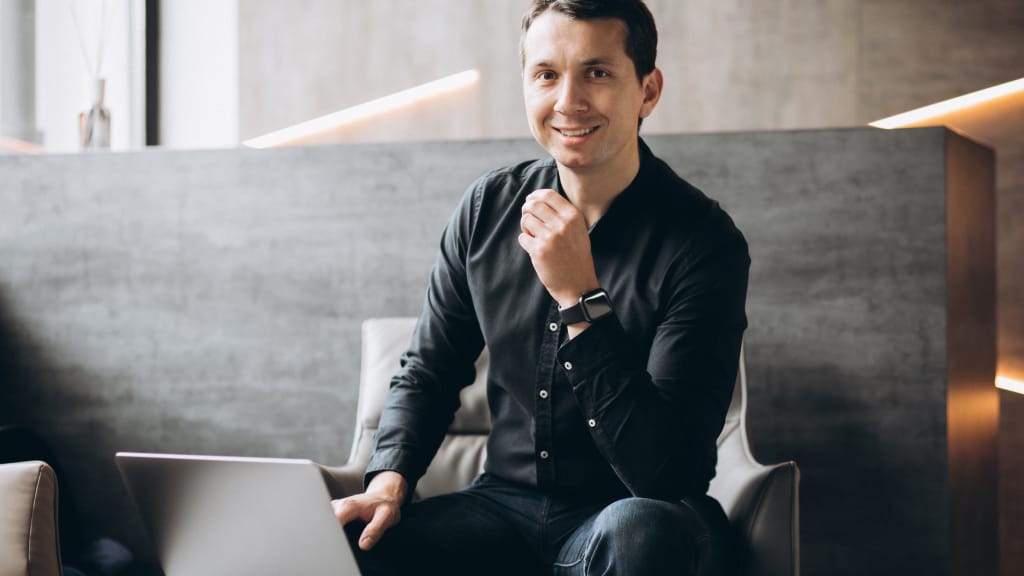
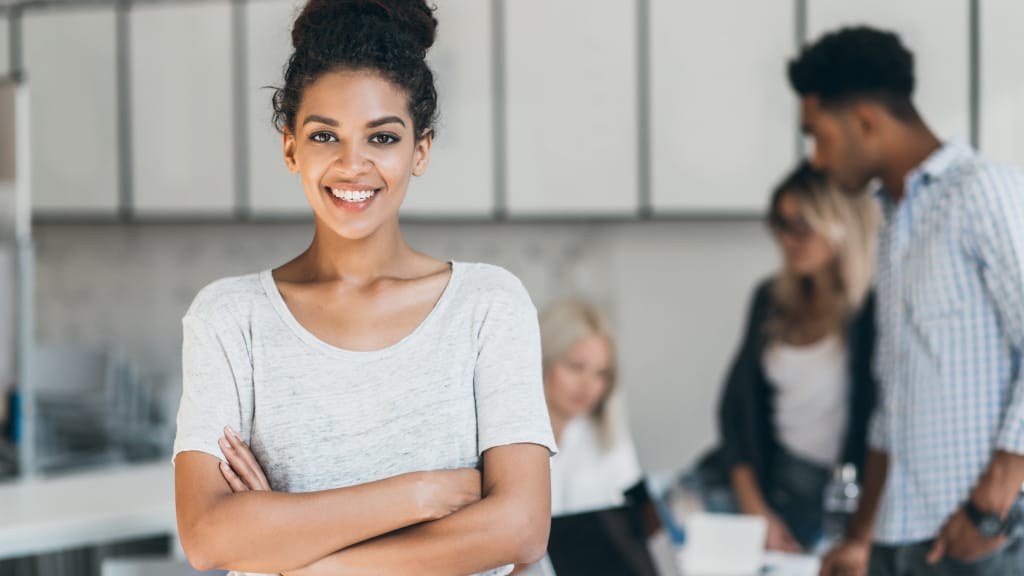
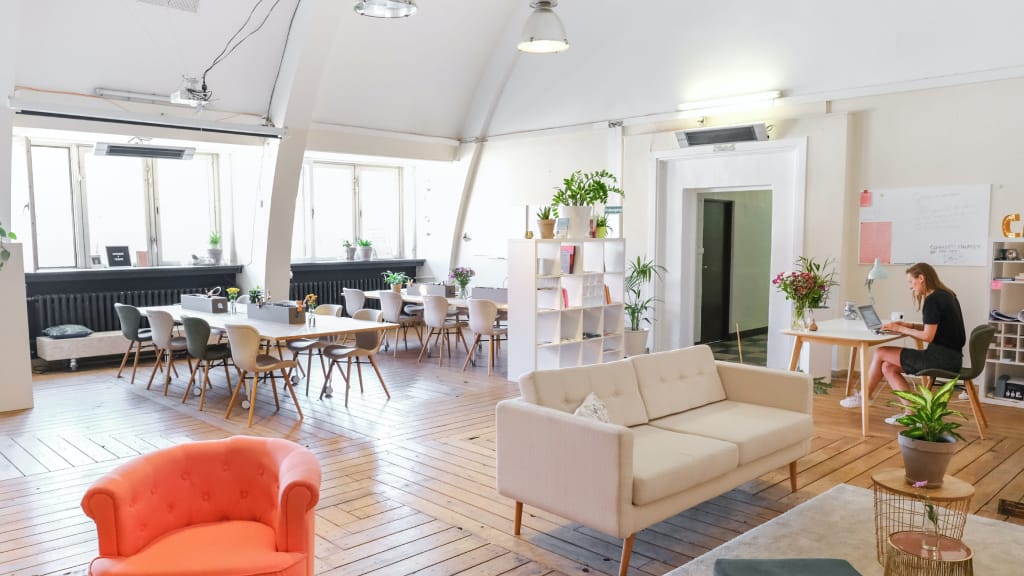
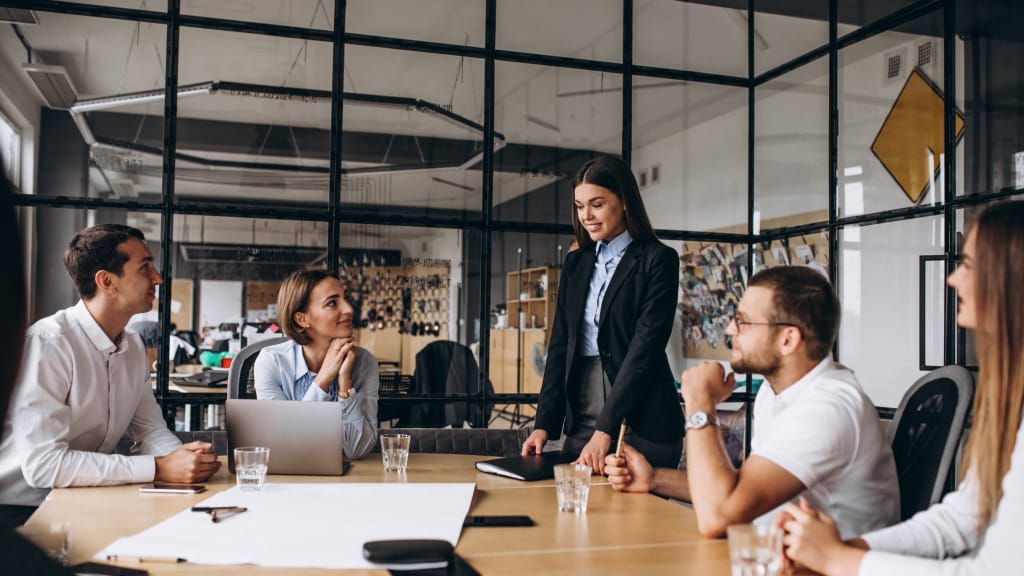
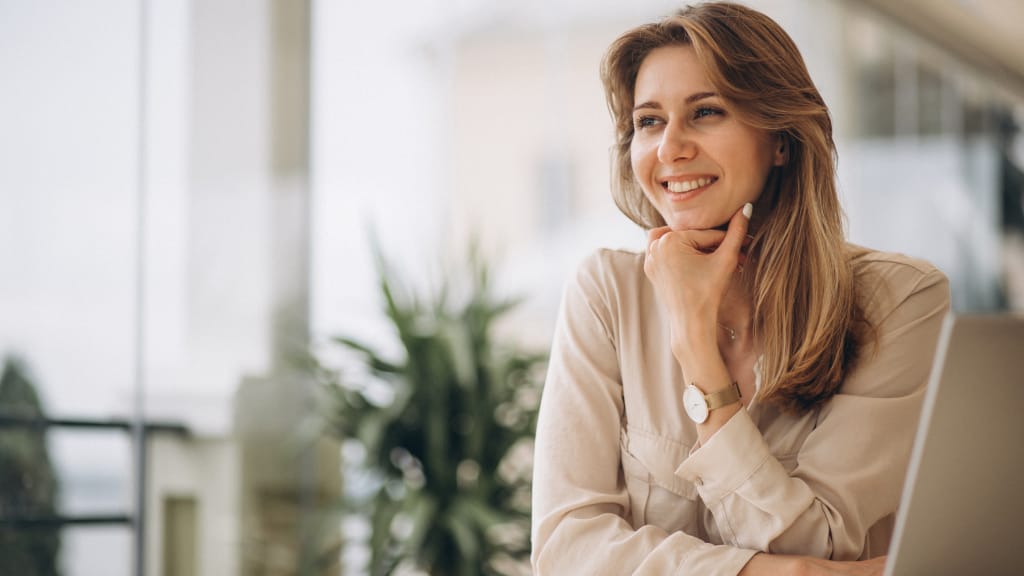
Web Development: Understanding HTML DOM
When it comes to web development, understanding the HTML Document Object Model (DOM) is crucial. The DOM represents the structure of an HTML document and allows developers to manipulate and interact with its elements. In this article, we will explore the basics of HTML DOM and provide examples to illustrate its functionality.
What is HTML DOM?
The HTML DOM is a programming interface for web documents. It represents the structure of an HTML document as a tree-like structure, where each element in the document is a node with properties and methods. The DOM allows developers to access, manipulate, and modify elements, attributes, and styles within an HTML document.
Accessing Elements
There are several methods to access elements in the HTML DOM:
getElementById()
: Retrieves an element by its unique ID attribute.getElementsByClassName()
: Retrieves elements by their class name.getElementsByTagName()
: Retrieves elements by their tag name.querySelector()
: Retrieves the first element that matches a CSS selector.querySelectorAll()
: Retrieves all elements that match a CSS selector.
Here's an example:
<div id="myDiv">Hello, World!</div>
<script>
var elementById = document.getElementById("myDiv");
var elementByClass = document.getElementsByClassName("myClass");
var elementsByTag = document.getElementsByTagName("p");
var elementByQuery = document.querySelector("#myDiv");
var elementsByQueryAll = document.querySelectorAll(".myClass");
</script>
Manipulating Elements
The DOM allows you to manipulate elements by changing their content, attributes, or styles. Here are some commonly used methods:
innerHTML
: Retrieves or sets the HTML content of an element.setAttribute()
: Sets the value of an attribute for an element.style
: Accesses or modifies the CSS properties of an element.appendChild()
: Appends a new child element to an existing element.
Here's an example:
<div id="myDiv">Hello, World!</div>
<script>
var element = document.getElementById("myDiv");
element.innerHTML = "New content";
element.setAttribute("class", "myClass");
element.style.color = "blue";
var newElement = document.createElement("p");
newElement.textContent = "This is a new paragraph.";
element.appendChild(newElement);
</script>
Handling Events
The DOM allows you to handle various events, such as mouse clicks, keyboard interactions, or form submissions. Here's an example of handling a button click event:
<button id="myButton">Click Me</button>
<script>
var button = document.getElementById("myButton");
button.addEventListener("click", function() {
alert("Button clicked!");
});
</script>
Conclusion
The HTML DOM is a powerful tool that enables web developers to interact with HTML elements, modify content, attributes, and styles, and handle events. By understanding how to access, manipulate, and interact with elements using the DOM, you can create dynamic and interactive web experiences. Explore the DOM API documentation for more in-depth information and continue to practice and experiment with different DOM methods to expand your web development skills.
4.5L
Learners
20+
Instructors
50+
Courses
6.0L
Course enrollments
Future Trending Courses
When selecting, a course, Here are a few areas that are expected to be in demand in the future:.
Future Learning for all
If you’re passionate and ready to dive in, we’d love to join 1:1 classes for you. We’re committed to support our learners and professionals their development and well-being.
View Courses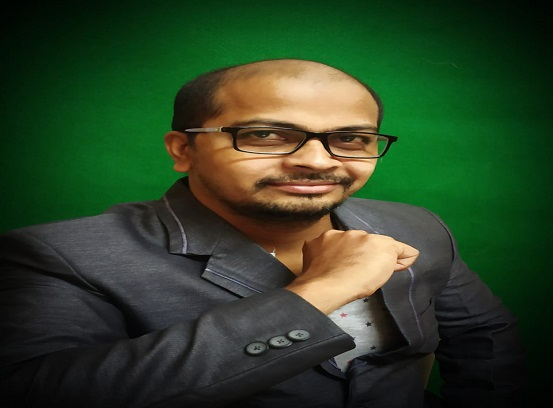
Most Popular Course topics
These are the most popular course topics among Software Courses for learners