Hi there, we’re Harisystems
"Unlock your potential and soar to new heights with our exclusive online courses! Ignite your passion, acquire valuable skills, and embrace limitless possibilities. Don't miss out on our limited-time sale - invest in yourself today and embark on a journey of personal and professional growth. Enroll now and shape your future with knowledge that lasts a lifetime!".
For corporate trainings, projects, and real world experience reach us. We believe that education should be accessible to all, regardless of geographical location or background.
1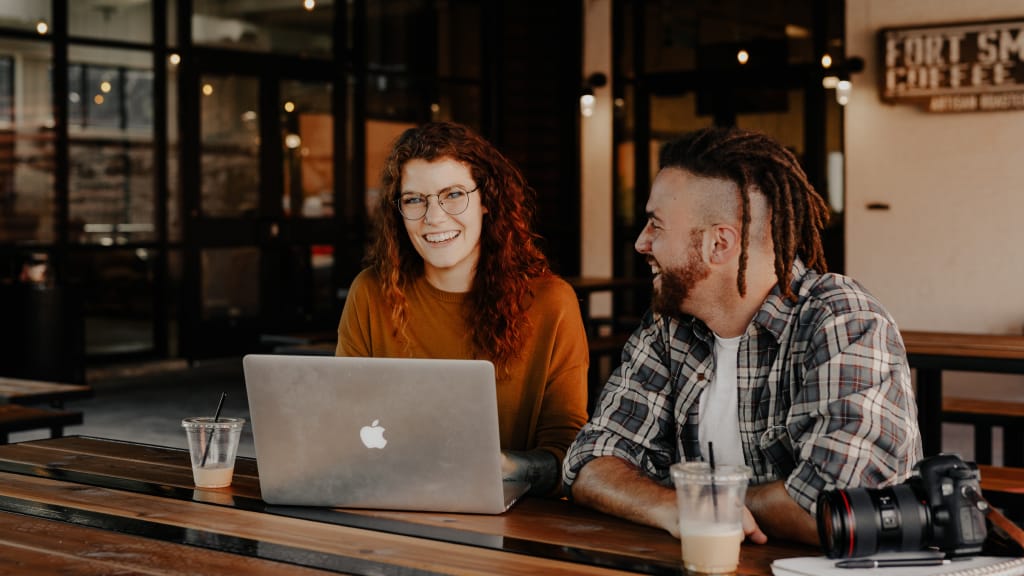
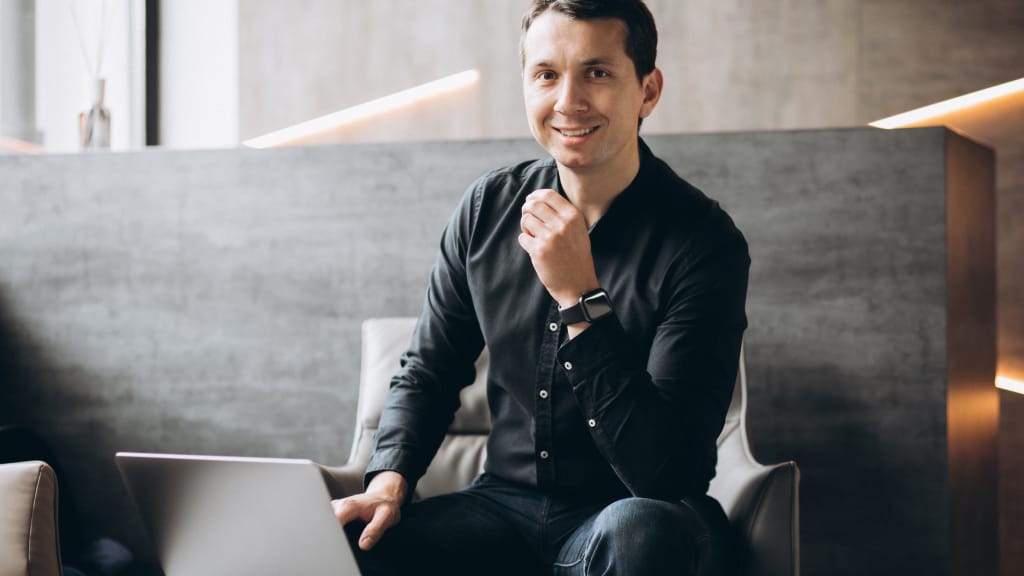
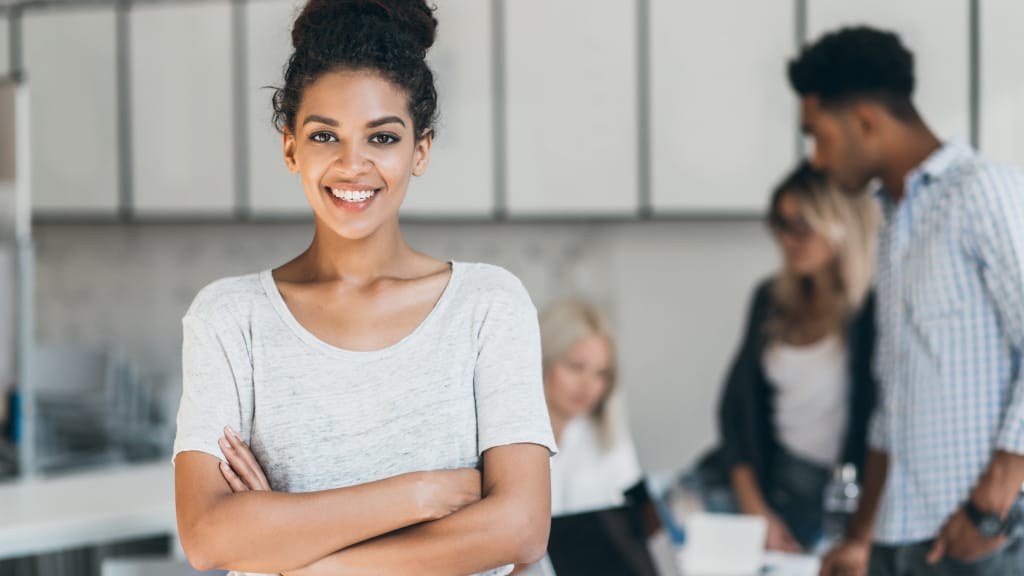
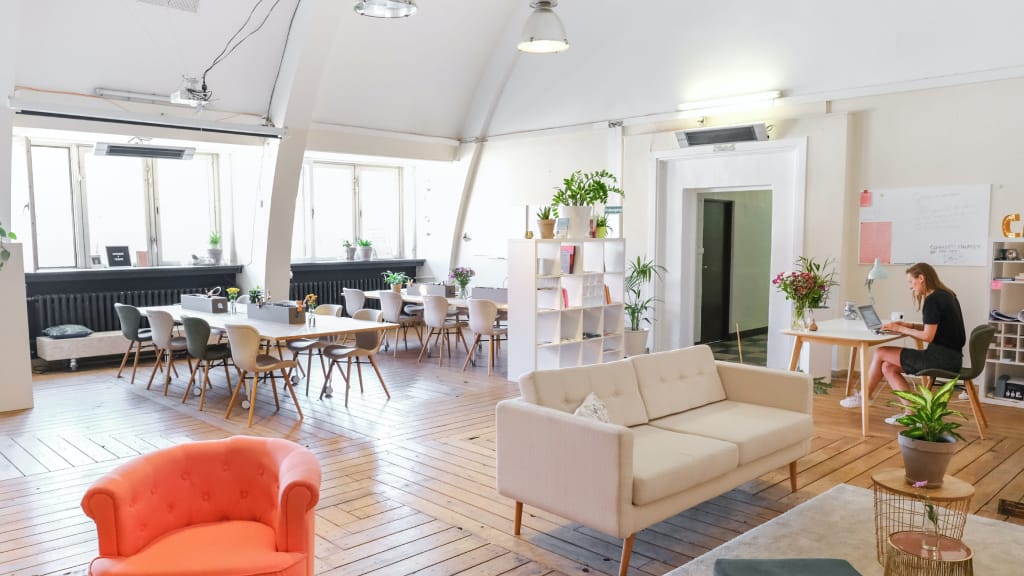
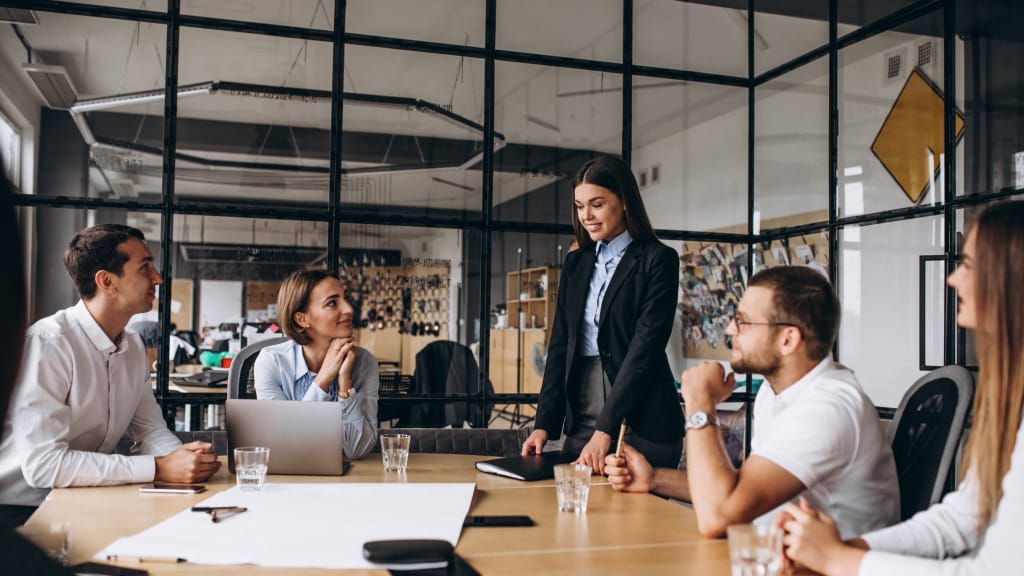
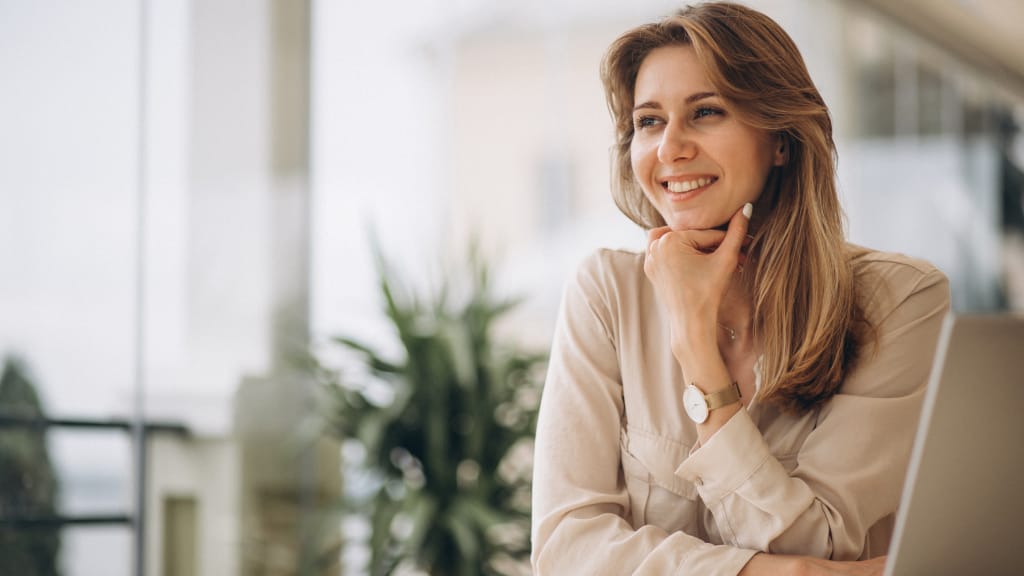
Internal JavaScript: Explained with Examples
JavaScript is a powerful scripting language that enables developers to add interactivity and dynamic functionality to web pages. One common approach to including JavaScript code in an HTML document is by using internal JavaScript. In this article, we will explore internal JavaScript and how it can be used within an HTML file.
What is Internal JavaScript?
Internal JavaScript refers to the placement of JavaScript code within an HTML document using the <script>
tag. The code is written directly within the HTML file, typically within the <head>
or <body>
section.
Usage and Syntax
To include internal JavaScript in an HTML document, you can use the <script>
tag with the JavaScript code placed between the opening and closing tags. Here's an example:
<html>
<head>
<title>Internal JavaScript Example</title>
<script>
function greet() {
console.log('Hello, World!');
}
</script>
</head>
<body>
<button onclick="greet()">Click Me</button>
</body>
</html>
In this example, the JavaScript code defining the greet()
function is placed within the <script>
tags in the <head>
section. The function is then called when the button is clicked using the onclick
event attribute.
Benefits of Internal JavaScript
Internal JavaScript offers several advantages for developers:
- Simplicity: The JavaScript code is directly embedded within the HTML file, making it easy to write and understand.
- Accessibility: Internal JavaScript code is accessible to other elements within the HTML document, allowing for seamless interaction.
- Quick Execution: The browser can load and execute the JavaScript code without making additional HTTP requests, resulting in faster rendering and performance.
- Code Organization: Internal JavaScript allows developers to keep the code related to a specific page within the same HTML file, promoting better code organization and maintenance.
Considerations
While internal JavaScript has its benefits, it's important to be mindful of potential drawbacks:
- Code Reusability: Internal JavaScript code cannot be easily reused across multiple HTML files, leading to code duplication.
- Maintainability: As the codebase grows, managing and updating internal JavaScript within HTML files can become challenging and prone to errors.
- Separation of Concerns: Placing JavaScript code directly in the HTML file may violate the principle of separating HTML, CSS, and JavaScript into separate layers.
As your JavaScript codebase expands, you may want to consider using external JavaScript files or adopting a modular approach using JavaScript frameworks and libraries.
In conclusion, internal JavaScript allows developers to include JavaScript code directly within an HTML file. It provides simplicity, accessibility, and quick execution. However, it's important to carefully consider code reusability, maintainability, and separation of concerns as your project evolves.
Inline JavaScript: Explained with Examples
JavaScript is a powerful scripting language that allows developers to add interactivity and dynamic behavior to web pages. One common way to include JavaScript code directly within an HTML document is through inline JavaScript. In this article, we will explore inline JavaScript and its usage within HTML files.
What is Inline JavaScript?
Inline JavaScript refers to the practice of embedding JavaScript code directly within an HTML element using event attributes such as onclick
or onload
. This approach allows for the execution of JavaScript code when a specific event occurs on an element.
Usage and Syntax
To include inline JavaScript in an HTML document, you can use event attributes such as onclick
, onload
, or others directly within HTML elements. Here's an example:
<button onclick="alert('Hello, World!')">Click Me</button>
In this example, the onclick
event attribute is used to execute the inline JavaScript code. When the button is clicked, the JavaScript code within the attribute is triggered, displaying an alert with the message 'Hello, World!'.
Benefits of Inline JavaScript
Inline JavaScript offers several advantages for developers:
- Quick Implementation: Inline JavaScript is easy to implement as it doesn't require separate script tags or external files.
- Element-specific Behavior: Inline JavaScript allows you to define element-specific behaviors, making it ideal for simple, isolated functionality.
- Immediate Feedback: Inline JavaScript provides immediate feedback to user actions by executing code directly within the event attribute.
Considerations
While inline JavaScript has its benefits, it's important to be mindful of some considerations:
- Code Maintainability: As the JavaScript code is directly embedded within HTML elements, it can become difficult to manage and maintain as the codebase grows.
- Code Reusability: Inline JavaScript code cannot be easily reused across different elements or HTML files, leading to code duplication.
- Separation of Concerns: Embedding JavaScript code within HTML elements can violate the principle of separating HTML, CSS, and JavaScript into distinct layers.
It's important to strike a balance and use inline JavaScript judiciously, considering the complexity and scalability of your project.
In conclusion, inline JavaScript is a convenient way to add small, specific behaviors to HTML elements. It offers quick implementation and element-specific functionality. However, it's important to consider code maintainability, reusability, and separation of concerns when using inline JavaScript in larger-scale projects.
External JavaScript: Explained with Examples
JavaScript is a powerful scripting language that enables developers to add interactivity and dynamic functionality to web pages. One common approach to including JavaScript code in an HTML document is by using external JavaScript files. In this article, we will explore external JavaScript and how it can be used within an HTML file.
What is External JavaScript?
External JavaScript involves storing JavaScript code in separate external files with a .js extension and linking them to an HTML document using the <script>
tag's src
attribute. By keeping the JavaScript code in separate files, it promotes code organization and separation of concerns.
Usage and Syntax
To include external JavaScript in an HTML document, you need to create a separate .js file and link it using the <script>
tag. Here's an example:
<html>
<head>
<title>External JavaScript Example</title>
<script src="script.js"></script>
</head>
<body>
<!-- HTML content goes here -->
</body>
</html>
In this example, the JavaScript code is stored in a separate file named script.js
. The <script>
tag's src
attribute is used to specify the location of the external JavaScript file. The JavaScript code within the file will be executed when the browser encounters the <script>
tag.
Benefits of External JavaScript
External JavaScript offers several advantages for developers:
- Code Reusability: External JavaScript files can be reused across multiple HTML files, promoting code reusability and reducing code duplication.
- Modularity: Keeping JavaScript code separate from HTML allows for better organization, modularity, and separation of concerns.
- Maintainability: External JavaScript files are easier to manage and update as they can be modified independently of the HTML structure.
- Improved Performance: Browsers can cache external JavaScript files, resulting in faster loading times for subsequent visits to the website.
Considerations
While external JavaScript has its benefits, it's important to consider a few points:
- File Management: Managing multiple external JavaScript files requires careful file organization and proper linking in HTML documents.
- Dependencies: Ensure that any external JavaScript files are properly included and loaded in the correct order to avoid dependency issues.
- HTTP Requests: Loading multiple external JavaScript files may result in additional HTTP requests, impacting initial page load time. Consider minifying and combining JavaScript files where possible.
It's important to strike a balance and use external JavaScript jud iciously, considering the project's size, complexity, and performance requirements.
In conclusion, external JavaScript files provide a modular and reusable approach to including JavaScript code in HTML documents. They offer code reusability, maintainability, and improved performance. By separating JavaScript code from HTML, developers can achieve better code organization and promote separation of concerns within their web applications.
4.5L
Learners
20+
Instructors
50+
Courses
6.0L
Course enrollments
Future Trending Courses
When selecting, a course, Here are a few areas that are expected to be in demand in the future:.
Future Learning for all
If you’re passionate and ready to dive in, we’d love to join 1:1 classes for you. We’re committed to support our learners and professionals their development and well-being.
View Courses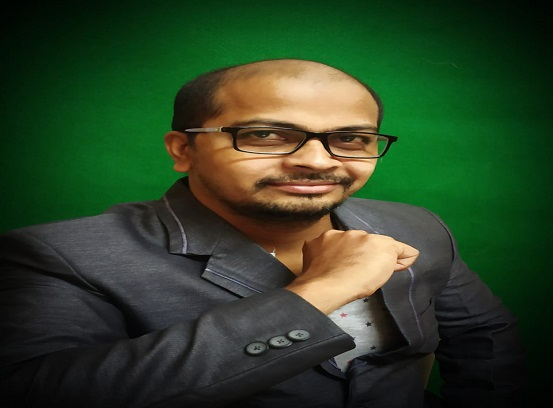
Most Popular Course topics
These are the most popular course topics among Software Courses for learners